[LeetCode]103. Binary Tree Zigzag Level Order Traversal--二叉树之字形遍历
2017-08-04 14:48
369 查看
103. Binary Tree Zigzag Level Order Traversal
Given a binary tree, return the zigzag level order traversal of its nodes’ values. (ie, from left to right, then right to left for the next level and alternate between).
For example:
Given binary tree [3,9,20,null,null,15,7],
3
/ \
9 20
/ \
15 7
return its zigzag level order traversal as:
[
[3],
[20,9],
[15,7]
]
分析:简单分析就可以发现,每行都是先进后出,所以很显然需要用到栈。但需要注意一点,对于每一行,左右孩子入栈的顺序不同,需要专门标记一下,奇数行先左孩子后右孩子入栈,偶数行先右孩子后左孩子入栈。
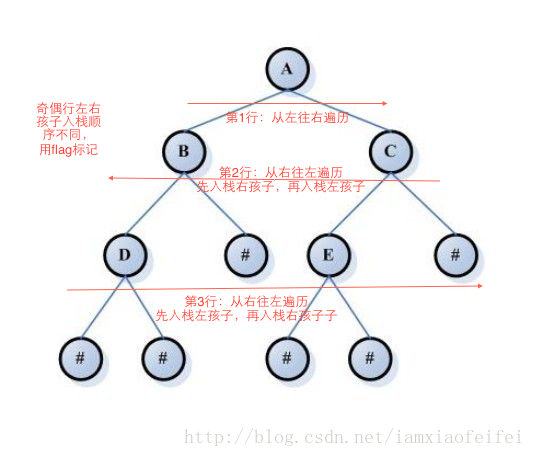
也可以使用《剑指offer》中解法,思想是一样的,代码略微不同。
Given a binary tree, return the zigzag level order traversal of its nodes’ values. (ie, from left to right, then right to left for the next level and alternate between).
For example:
Given binary tree [3,9,20,null,null,15,7],
3
/ \
9 20
/ \
15 7
return its zigzag level order traversal as:
[
[3],
[20,9],
[15,7]
]
分析:简单分析就可以发现,每行都是先进后出,所以很显然需要用到栈。但需要注意一点,对于每一行,左右孩子入栈的顺序不同,需要专门标记一下,奇数行先左孩子后右孩子入栈,偶数行先右孩子后左孩子入栈。
/** * Definition for a binary tree node. * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode(int x) : val(x), left(NULL), right(NULL) {} * }; */ class Solution { public: vector<vector<int>> zigzagLevelOrder(TreeNode* root) { vector<vector<int>> result; if(root == NULL) return result; stack<TreeNode*>current, next; current.push(root); vector<int> oneLayer; bool flag = true; while(!current.empty()){ TreeNode* pCur = current.top(); current.pop(); oneLayer.push_back(pCur->val); // 需要确定左右子树入栈的顺序 // 用flag标记 if(flag){ if(pCur->left) next.push(pCur->left); if(pCur->right) next.push(pCur->right); }else{ if(pCur->right) next.push(pCur->right); if(pCur->left) next.push(pCur->left); } if(current.empty()){ result.push_back(oneLayer); oneLayer.clear(); // 清空 swap(current, next); // 直接交换 flag = !flag; // flag切换 } } return result; } };
也可以使用《剑指offer》中解法,思想是一样的,代码略微不同。
/** * Definition for a binary tree node. * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode(int x) : val(x), left(NULL), right(NULL) {} * }; */ class Solution { public: vector<vector<int>> zigzagLevelOrder(TreeNode* root) { vector<vector<int>> result; if(!root) return result; stack<TreeNode*> s[2]; int current = 0; int next = 1; s[current].push(root); vector<int> v; while(!s[current].empty() || !s[next].empty()){ TreeNode* pCur = s[current].top(); s[current].pop(); v.push_back(pCur->val); if(current == 0){ if(pCur->left) s[next].push(pCur->left); if(pCur->right) s[next].push(pCur->right); }else{ if(pCur->right) s[next].push(pCur->right); if(pCur->left) s[next].push(pCur->left); } if(s[current].empty()){ current = 1 - current; next = 1 - next; result.push_back(v); v.clear(); } } return result; } };
相关文章推荐
- 【LeetCode笔记】Binary Tree Zigzag Level Order Traversal 二叉树Z字形遍历
- 【LeetCode-面试算法经典-Java实现】【103-Binary Tree Zigzag Level Order Traversal(二叉树分层Z字形遍历)】
- LeetCode 103. Binary Tree Zigzag Level Order Traversal(二叉树之字形遍历)
- [LeetCode] 103. Binary Tree Zigzag Level Order Traversal 二叉树的之字形层序遍历
- [LeetCode] Binary Tree Level Order Traversal 二叉树层序遍历
- leetcode中二叉树的遍历
- 已知前序(后序)遍历序列和中序遍历序列构建二叉树(Leetcode相关题目)
- LeetCode 314. Binary Tree Vertical Order Traversal(二叉树垂直遍历)
- [LeetCode]144. Binary Tree Preorder Traversal--二叉树前序遍历
- LeetCode基础--二叉树--ZigZag遍历
- leetCode 103.Binary Tree Zigzag Level Order Traversal (二叉树Z字形水平序) 解题思路和方法
- [LeetCode] Binary Tree Level Order Traversal II 二叉树层序遍历之二
- [leetcode]Minimum Depth of Binary Tree--二叉树层序遍历的应用
- leetcode 236. Lowest Common Ancestor of a Binary Tree 最近公告祖先LCA + 二叉树 + 深度优先遍历DFS
- [LeetCode] Binary Tree Level Order Traversal 二叉树层次遍历(DFS | BFS)
- LeetCode-106:Construct Binary Tree from Inorder and Postorder Traversal (利用中序和后序遍历构建二叉树) -- medium
- 根据前序中序,中序后序遍历构造二叉树 leetcode105 106
- LeetCode 145 Binary Tree Postorder Traversal (后序遍历二叉树)
- LeetCode Binary Tree Preorder Traversal 前序遍历二叉树 递归和非递归解法
- leetcode 94. Binary Tree Inorder Traversal 二叉树中序递归遍历