算法之二分查找(c++版实现+测试)
2017-07-10 19:39
525 查看
进阶版入口
递归版算法(deep变量是为了测试,实际生产可不需要):
核心算法:
测试专用代码:
main.cpp
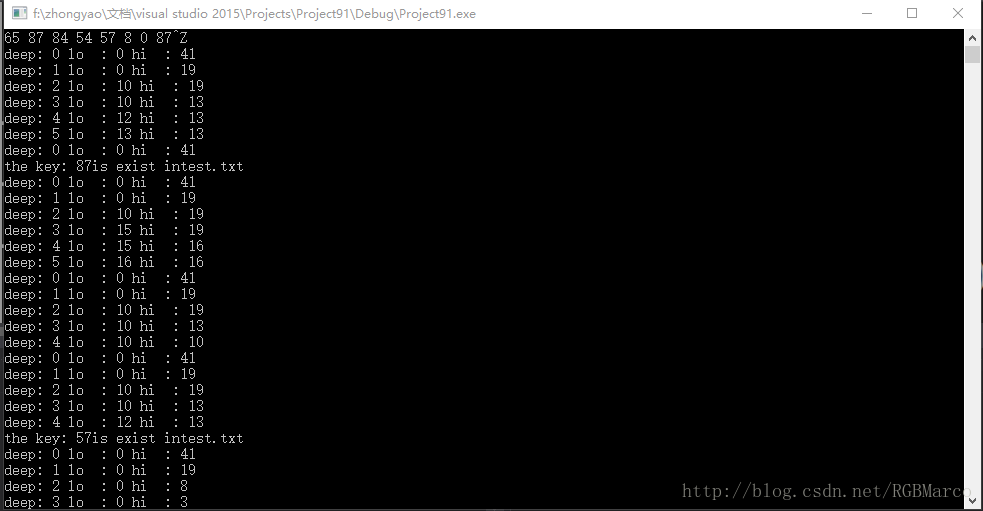
非递归算法:
测试代码:
main.cpp
递归版算法(deep变量是为了测试,实际生产可不需要):
核心算法:
#include <iostream> #include <vector> #include <fstream> #include <iterator> #include <algorithm> #include <string> using namespace std; template<typename T,typename Container> int Rank(const T& key, const Container& c, const int& lo,const int& hi,int& deep) { if (lo > hi) return -1; int mid = lo + (hi - lo) / 2; //use to test cout << "deep: " << deep << ends << "lo : " << lo << ends << "hi : " << hi << endl; if (key > c[mid]) { ++deep; return Rank(key, c, mid + 1, hi,deep); } else if (key < c[mid]) { ++deep; return Rank(key, c, lo, mid - 1,deep); } else return mid; } template<typename T,typename Container> int BinarySearch(const T& key,Container& c) { //deep表示 递归深度 int deep = 0; //必须要排序 可以把这步放在调用BinarySearch()之前避免对一个序列 //重复调用sort(); sort(begin(c), end(c)); return Rank(key, c, 0, static_cast<int>(end(c)-begin(c))-1,deep); }
测试专用代码:
void test(const string& mode, const string& file) { ifstream in(file); vector<int> c; copy(istream_iterator<int>(in), istream_iterator<int>(), back_inserter(c)); auto f = [=,&c](const int& key) { //从标准输入找出文件中存在的 if (mode == "exist") { if (BinarySearch(key, c) != -1) { cout << "the key: " << key << "is exist in" << file << endl; } } if (mode == "noexist") { if (BinarySearch(key, c) == -1) { cout << "the key: " << key << "is not exist in" << file << endl; } } }; for_each(istream_iterator<int>(cin), istream_iterator<int>(),f); }
main.cpp
int main() { test("exist", "test.txt"); system("pause"); return 0; }
非递归算法:
#include <iostream> #include <vector> #include <fstream> #include <iterator> #include <algorithm> #include <string> using namespace std; template<typename T,typename Container> int BinarySearch(const T& key, Container& c) { sort(begin(c), end(c)); int lo = 0; int hi = static_cast<int>(end(c) - begin(c))-1; while (lo <= hi) { int mid = lo + (hi - lo) / 2; if (c[mid] > key) hi = mid - 1; else if (c[mid] < key) lo = mid + 1; else return mid; } return -1; }
测试代码:
void test(const string& mode, const string& file) { ifstream in(file); vector<int> c; copy(istream_iterator<int>(in), istream_iterator<int>(), back_inserter(c)); auto f = [=,&c](const int& key) { //从标准输入找出文件中存在的 if (mode == "exist") { if (BinarySearch(key, c) != -1) { cout << "the key: " << key << " is exist in" << file << endl; } } if (mode == "noexist") { if (BinarySearch(key, c) == -1) { cout << "the key: " << key << "is not exist in" << file << endl; } } }; for_each(istream_iterator<int>(cin), istream_iterator<int>(),f); }
main.cpp
int main() { test("exist", "test.txt"); system("pause"); return 0; }
相关文章推荐
- 算法之二分查找(c语言版实现+测试)
- 算法之二分查找(java版实现加测试)
- 【算法和数据结构】分治思想之二分查找(C++实现)
- [C++] 测试硬件popcnt(位1计数)指令与各种软件算法,利用模板实现静态多态优化性能
- 顺序表创建以及查找排序算法(含有顺序查找算法、带哨兵站顺序查找、折半查找算法、冒泡排序)的C++实现在vs2013环境下实现
- c/c++算法之正确实现二分查找
- 算法:C++实现O(n)复杂度内查找第K大数
- 算法:C++实现二分查找
- C++实现查找中位数的O(N)算法和Kmin算法
- C++ 基础算法之二分查找
- 二分查找算法的C/C++实现
- 算法(第四版)学习笔记之二分查找的递归与非递归java实现
- 单词字典中对兄弟单词查找算法(C++实现)
- 算法-对分查找(二分查找)C++实现
- PHP实现常见算法之二分查找法
- C++实现的O(n)复杂度内查找第K大数算法示例
- 算法代码实现之二分法查找,C/C++实现
- 算法-对分查找(二分查找)C++实现
- 素数判断算法 - 拉宾-米勒测试定理(c++实现)
- 查找(顺序、二分、斐波那契和插值)算法的实现和测试