复杂单链表的复制
2017-07-04 17:00
218 查看
复杂单链表的复制:
一、何为复制单链表
就我们所知,单链表也就是一个结点包含一个数据域和一个指针域,这样若干个结点构成的链表。而复杂单链表和普通单链表差不多,唯一的不同就是多一个random。我们可以用一张图来表示:
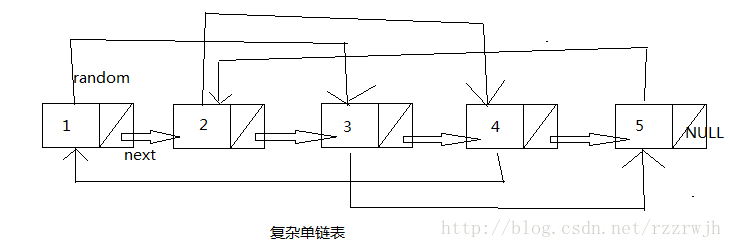
那我们怎么来进行复杂链表的复制呢,现在公认的最有效的方法只有一种,就是在原有单链表的每个元素后面添加一个结点,结点中的data是前一个结点的data,结点的next是原来结点的next。
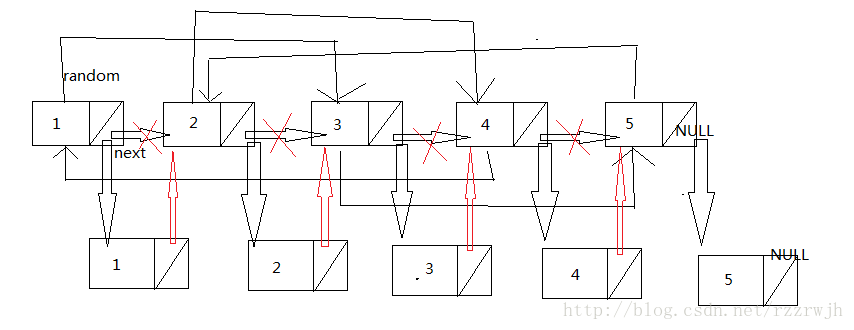
由上图可见我们已经将结点都插入到原有复杂单链表的后面去了,这样我们就已经搞定了复杂单链表的一个复制:复制next关系。接着要做的就是复制它的random关系,由图,可以很清楚地看到,要复制单链表的结点的random也就是原有结点的random的next。用一个例子来解释:
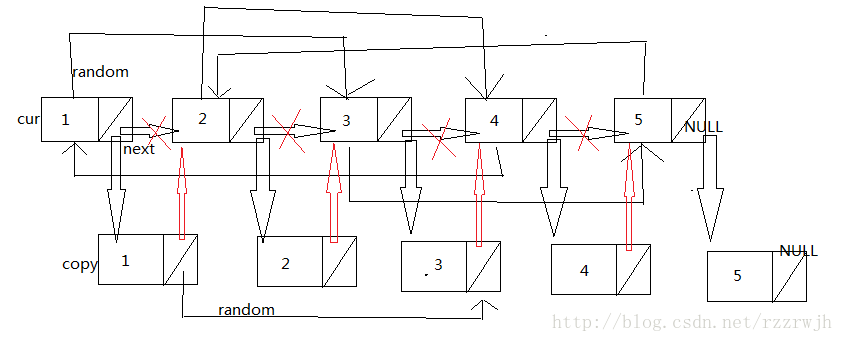
由图:cur的random的data为3,如果要进行复制,那么复制出来的链表的random的data也必须是3,且指向的位置应该和原来的位置是一样的。那么可以明显看到copy->random = cur->random->next。
这样也就完成了一次random的复制。如此重复,就能将所有的random关系都赋好。
最后也就剩一个拆分了,这步最为简单,将两个单链表从一个单链表中分离出来就行。
二、代码实现
头文件:
程序实现文件:
测试文件:
到此,复杂链表的复制完成。
一、何为复制单链表
就我们所知,单链表也就是一个结点包含一个数据域和一个指针域,这样若干个结点构成的链表。而复杂单链表和普通单链表差不多,唯一的不同就是多一个random。我们可以用一张图来表示:
那我们怎么来进行复杂链表的复制呢,现在公认的最有效的方法只有一种,就是在原有单链表的每个元素后面添加一个结点,结点中的data是前一个结点的data,结点的next是原来结点的next。
由上图可见我们已经将结点都插入到原有复杂单链表的后面去了,这样我们就已经搞定了复杂单链表的一个复制:复制next关系。接着要做的就是复制它的random关系,由图,可以很清楚地看到,要复制单链表的结点的random也就是原有结点的random的next。用一个例子来解释:
由图:cur的random的data为3,如果要进行复制,那么复制出来的链表的random的data也必须是3,且指向的位置应该和原来的位置是一样的。那么可以明显看到copy->random = cur->random->next。
这样也就完成了一次random的复制。如此重复,就能将所有的random关系都赋好。
最后也就剩一个拆分了,这步最为简单,将两个单链表从一个单链表中分离出来就行。
二、代码实现
头文件:
#ifndef __COMPLEXLIST_H__ #define __COMPLEXLIST_H__ #include <stdio.h> #include <stdlib.h> #include <assert.h> #include <string.h> typedef int DataType; typedef struct complexnode { DataType _data; struct complexnode* _next; struct complexnode* _random; }complexnode; complexnode* Buycomplexnode(DataType x);//创建一个结点 void display(complexnode* head);//打印链表 complexnode* copycomplexnode(complexnode* head);//复制链表 void destroylist(complexnode* head);//销毁链表 #endif
程序实现文件:
#include "complexlist.h" complexnode* Buycomplexnode(DataType x) { complexnode* newnode = NULL; newnode = (complexnode*)malloc(sizeof(complexnode)); if(newnode == NULL) { perror("complexnode()::malloc"); return NULL; } newnode->_data = x; newnode->_next = NULL; newnode->_random = NULL; return newnode; } void display(complexnode* head) { complexnode* cur = head; while(cur) { printf("%d-%d-->",cur->_data,cur->_random->_data); cur = cur->_next; } printf("NULL\n"); } complexnode* copycomplexnode(complexnode* head) { complexnode* cur = head; complexnode* copy =NULL; complexnode* prev = NULL; complexnode* random = NULL; complexnode* newhead = NULL; //在原有链表的结点之后插入结点 while(cur) { complexnode* ptr = Buycomplexnode(cur->_data); prev = cur; cur = cur->_next; ptr->_next = cur; prev->_next = ptr; } //将原有链表的random关系赋给将要复制的链表 cur = head; while(cur) { random = cur->_random; copy = cur->_next; copy->_random = random->_next; cur = cur->_next->_next; } //将复制完的链表拆分,形成两条链表 cur = head; newhead = cur->_next; copy = cur->_next; while(cur) { cur->_next = copy->_next; cur = cur->_next; if(cur != NULL) { copy->_next = cur->_next; copy = copy->_next; } } return newhead; } void destroylist(complexnode* head) { complexnode* cur =< 4000 /span> head; while(cur) { complexnode* del = cur; cur = cur->_next; free(del); } free(cur); head = NULL; }
测试文件:
#include "complexlist.h" void test() { complexnode* head; complexnode* newhead; complexnode* node1 = Buycomplexnode(1); complexnode* node2 = Buycomplexnode(2); complexnode* node3 = Buycomplexnode(3); complexnode* node4 = Buycomplexnode(4); complexnode* node5 = Buycomplexnode(5); node1->_next = node2; node2->_next = node3; node3->_next = node4; node4->_next = node5; node1->_random = node3; node2->_random = node4; node3->_random = node5; node4->_random = node1; node5->_random = node2; //构造复杂链表 head = node1; display(head);//打印链表 newhead = copycomplexnode(head);//复制复杂链表 display(newhead); destroylist(head);//销毁链表 destroylist(newhead);//销毁链表 } int main() { test(); return 0; }
到此,复杂链表的复制完成。
相关文章推荐
- 复杂链表的复制
- 软件设计艺术大师基本功--复杂链表的复制
- 剑指Offer24 复杂链表的复制
- 复杂链表的复制
- 复杂链表的复制
- 输入一个复杂链表(每个节点中有节点值,以及两个指针,一个指向下一个节点,另一个特殊指针指向任意一个节点),返回结果为复制后复杂链表的head。(注意,输出结果中请不要返回参数中的节点引用,否则判题程序
- 程序员面试题精选100题(49)-复杂链表的复制[算法]
- 复杂链表的复制
- 复杂链表的复制
- 复杂链表的复制
- 复杂链表的复制
- Q26复杂链表的复制
- 剑指offer--复杂链表的复制
- 程序员面试题精选---复杂链表的复制
- 面试题26:复杂链表的复制
- 复杂链表的复制、链表判环、无环单链表判相交
- 算法题:复杂链表的复制
- 【链表】复杂链表的复制
- 复杂链表复制