5 Hibernate:Java Persistence API (JPA) 入门
2017-07-02 00:00
375 查看
Java Persistence API (JPA),Java 持久层 API,是 JCP 组织发布的 java EE 标准规范,通过注解或 XML 描述实体对象和数据库表的映射关系。
Hibernate 实现了 JPA 规范,以下展示 Hibernate JPA 开发的完整步骤:
2 创建 JPA 配置文件
Hibernate JPA 开发不需要创建 Hibernate 特定配置文件
注意:
需要为
对比
4 通过 JPA API 编写访问数据库的代码
单元测试日志:
数据库查询结果:
Hibernate 实现了 JPA 规范,以下展示 Hibernate JPA 开发的完整步骤:
1 使用 Maven 管理 Hibernate 依赖
参看 4 Hibernate:使用注解(Annotation)2 创建 JPA 配置文件 persistence.xml
Hibernate JPA 开发不需要创建 Hibernate 特定配置文件 hibernate.cfg.xml,取而代之的是创建 JPA 自有配置文件
persistence.xml,JPA 规范定义的启动过程不同于 Hibernate 启动过程。
<persistence xmlns="http://java.sun.com/xml/ns/persistence" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/persistence http://java.sun.com/xml/ns/persistence/persistence_2_0.xsd" version="2.0"> <persistence-unit name="hibernate.jpa"> <description> Persistence unit for the JPA tutorial of the Hibernate Getting Started Guide </description> <class>hibernate.Person</class> <properties> <property name="javax.persistence.jdbc.driver" value="com.mysql.jdbc.Driver" /> <property name="javax.persistence.jdbc.url" value="jdbc:mysql://localhost:3306/test" /> <property name="javax.persistence.jdbc.user" value="root" /> <property name="javax.persistence.jdbc.password" value="123456" /> <property name="hibernate.show_sql" value="true" /> <property name="hibernate.hbm2ddl.auto" value="create" /> </properties> </persistence-unit> </persistence>
注意:
需要为
persistence-unit设置
name属性,此名称唯一;
对比
hibernate.cfg.xml,除数据库连接配置部分属性名称有所不同,其他配置保持一致。
3 使用注解创建持久化类
package hibernate; import java.util.Date; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; import javax.persistence.Table; import javax.persistence.Temporal; import javax.persistence.TemporalType; import org.hibernate.annotations.GenericGenerator; @Entity @Table(name = "person") public class Person { private int id; private String account; private String name; private Date birth; public Person() {} public Person(String account, String name, Date birth) { this.account = account; this.name = name; this.birth = birth; } @Id // 实体唯一标识 @GeneratedValue(generator = "increment") // 使用名为“increment”的生成器 @GenericGenerator(name = "increment", strategy = "increment") // 定义名为“increment”的生成器,使用Hibernate的"increment"生成策略,即自增 public int getId() { return id; } public void setId(int id) { this.id = id; } public String getAccount() { return account; } public void setAccount(String account) { this.account = account; } public String getName() { return name; } public void setName(String name) { this.name = name; } @Temporal(TemporalType.TIMESTAMP) public Date getBirth() { return birth; } public void setBirth(Date birth) { this.birth = birth; } @Override public String toString() { return "Person [id=" + id + ", account=" + account + ", name=" + name + ", birth=" + birth + "]"; } }
4 通过 JPA API 编写访问数据库的代码
package hibernate; import java.text.ParseException; import java.util.Date; import java.util.List; import javax.persistence.EntityManager; import javax.persistence.EntityManagerFactory; import javax.persistence.Persistence; import org.junit.After; import org.junit.Before; import org.junit.Test; public class EntityManagerIllustrationTest { private EntityManagerFactory entityManagerFactory; @Before public void setUp() throws Exception { entityManagerFactory = Persistence.createEntityManagerFactory("hibernate.jpa"); } @After public void tearDown() throws Exception { entityManagerFactory.close(); } @Test public void test() throws ParseException { EntityManager entityManager = entityManagerFactory.createEntityManager(); entityManager.getTransaction().begin(); entityManager.persist(new Person("tony", "Tony Stark", new Date())); entityManager.persist(new Person("hulk", "Bruce Banner", new Date())); entityManager.getTransaction().commit(); entityManager.close(); entityManager = entityManagerFactory.createEntityManager(); entityManager.getTransaction().begin(); List<Person> result = entityManager.createQuery("FROM Person", Person.class).getResultList(); for (Person person : result) { System.out.println(person); } entityManager.getTransaction().commit(); entityManager.close(); } }
单元测试日志:
六月 26, 2017 12:01:22 上午 org.hibernate.jpa.internal.util.LogHelper logPersistenceUnitInformation INFO: HHH000204: Processing PersistenceUnitInfo [ name: hibernate.jpa ...] 六月 26, 2017 12:01:23 上午 org.hibernate.Version logVersion INFO: HHH000412: Hibernate Core {5.2.10.Final} 六月 26, 2017 12:01:23 上午 org.hibernate.cfg.Environment <clinit> INFO: HHH000206: hibernate.properties not found 六月 26, 2017 12:01:23 上午 org.hibernate.annotations.common.reflection.java.JavaReflectionManager <clinit> INFO: HCANN000001: Hibernate Commons Annotations {5.0.1.Final} 六月 26, 2017 12:01:23 上午 org.hibernate.boot.jaxb.internal.stax.LocalXmlResourceResolver resolveEntity WARN: HHH90000012: Recognized obsolete hibernate namespace http://hibernate.sourceforge.net/hibernate-mapping. Use namespace http://www.hibernate.org/dtd/hibernate-mapping instead. Support for obsolete DTD/XSD namespaces may be removed at any time. 六月 26, 2017 12:01:28 上午 org.hibernate.engine.jdbc.connections.internal.DriverManagerConnectionProviderImpl configure WARN: HHH10001002: Using Hibernate built-in connection pool (not for production use!) 六月 26, 2017 12:01:28 上午 org.hibernate.engine.jdbc.connections.internal.DriverManagerConnectionProviderImpl buildCreator INFO: HHH10001005: using driver [com.mysql.jdbc.Driver] at URL [jdbc:mysql://localhost:3306/test] 六月 26, 2017 12:01:28 上午 org.hibernate.engine.jdbc.connections.internal.DriverManagerConnectionProviderImpl buildCreator INFO: HHH10001001: Connection properties: {user=root, password=****} 六月 26, 2017 12:01:28 上午 org.hibernate.engine.jdbc.connections.internal.DriverManagerConnectionProviderImpl buildCreator INFO: HHH10001003: Autocommit mode: false 六月 26, 2017 12:01:28 上午 org.hibernate.engine.jdbc.connections.internal.PooledConnections <init> INFO: HHH000115: Hibernate connection pool size: 20 (min=1) Mon Jun 26 00:01:29 CST 2017 WARN: Establishing SSL connection without server's identity verification is not recommended. According to MySQL 5.5.45+, 5.6.26+ and 5.7.6+ requirements SSL connection must be established by default if explicit option isn't set. For compliance with existing applications not using SSL the verifyServerCertificate property is set to 'false'. You need either to explicitly disable SSL by setting useSSL=false, or set useSSL=true and provide truststore for server certificate verification. 六月 26, 2017 12:01:30 上午 org.hibernate.dialect.Dialect <init> INFO: HHH000400: Using dialect: org.hibernate.dialect.MySQL5Dialect Hibernate: drop table if exists PERSON 六月 26, 2017 12:01:32 上午 org.hibernate.resource.transaction.backend.jdbc.internal.DdlTransactionIsolatorNonJtaImpl getIsolatedConnection INFO: HHH10001501: Connection obtained from JdbcConnectionAccess [org.hibernate.engine.jdbc.env.internal.JdbcEnvironmentInitiator$ConnectionProviderJdbcConnectionAccess@73511076] for (non-JTA) DDL execution was not in auto-commit mode; the Connection 'local transaction' will be committed and the Connection will be set into auto-commit mode. Hibernate: create table PERSON (ID integer not null auto_increment, ACCOUNT varchar(255), NAME varchar(255), BIRTH datetime, primary key (ID)) engine=MyISAM 六月 26, 2017 12:01:32 上午 org.hibernate.resource.transaction.backend.jdbc.internal.DdlTransactionIsolatorNonJtaImpl getIsolatedConnection INFO: HHH10001501: Connection obtained from JdbcConnectionAccess [org.hibernate.engine.jdbc.env.internal.JdbcEnvironmentInitiator$ConnectionProviderJdbcConnectionAccess@42257bdd] for (non-JTA) DDL execution was not in auto-commit mode; the Connection 'local transaction' will be committed and the Connection will be set into auto-commit mode. 六月 26, 2017 12:01:33 上午 org.hibernate.tool.schema.internal.SchemaCreatorImpl applyImportSources INFO: HHH000476: Executing import script 'org.hibernate.tool.schema.internal.exec.ScriptSourceInputNonExistentImpl@4b629f13' Hibernate: insert into PERSON (ACCOUNT, NAME, BIRTH) values (?, ?, ?) Hibernate: insert into PERSON (ACCOUNT, NAME, BIRTH) values (?, ?, ?) 六月 26, 2017 12:01:33 上午 org.hibernate.hql.internal.QueryTranslatorFactoryInitiator initiateService INFO: HHH000397: Using ASTQueryTranslatorFactory Hibernate: select person0_.ID as ID1_0_, person0_.ACCOUNT as ACCOUNT2_0_, person0_.NAME as NAME3_0_, person0_.BIRTH as BIRTH4_0_ from PERSON person0_ Person [id=1, account=tony, name=Tony Stark, birth=2017-06-26 00:01:33.0] Person [id=2, account=hulk, name=Bruce Banner, birth=2017-06-26 00:01:34.0] 六月 26, 2017 12:01:34 上午 org.hibernate.engine.jdbc.connections.internal.DriverManagerConnectionProviderImpl stop INFO: HHH10001008: Cleaning up connection pool [jdbc:mysql://localhost:3306/test]
数据库查询结果:
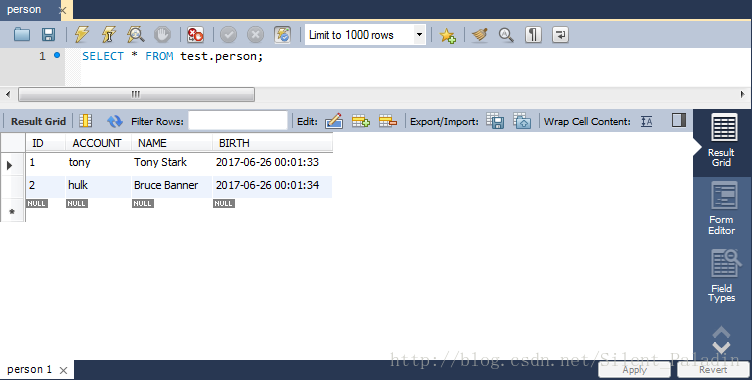
相关文章推荐
- 5 Hibernate:Java Persistence API (JPA) 入门
- JBoss 系列六十三:JBoss 7/WildFly 集群之 Java Persistence API (JPA) - II(Hibernate查询缓存和二级缓存示例)
- JBoss 系列六十三:JBoss 7/WildFly 集群之 Java Persistence API (JPA) - II(Hibernate查询缓存和二级缓存示例)
- JBoss 系列六十三:JBoss 7/WildFly 集群之 Java Persistence API (JPA) - II(Hibernate查询缓存和二级缓存示例)
- Spring3.0 JPA(hibernate3.6实现)整合问题之:java.lang.NoSuchMethodError: javax.persistence.spi.PersistenceUnitInfo.getValidationMode()Lja
- JPA(Java Persistence API,Java持久化API)
- JBoss 系列五十六:JBoss 7/WildFly 集群之 Java Persistence API (JPA) - I(基本理论)
- JPA(Java Persistence API,Java持久化API)
- JAVA PERSISTENCE API (JPA)
- JPA入门例子(采用JPA的hibernate实现版本) --- 会伴随 配置文件:persistence.xml
- Java Persistence API、Hibernate、Toplink、Entity Enterprise Java bean之间的优缺点
- Spring3.0 JPA(hibernate3.6实现)整合问题之:java.lang.NoSuchMethodError: javax.persistence.spi.PersistenceUni
- fastDFS+java api + sping mvc +JPA+Hibernate
- JPA-Java Persistence API
- 【Java基础】JPA入门例子(采用JPA的hibernate实现版本)
- 第6章 JPA-Java Persistence API
- JPA(Java Persistence API)学习总结
- Java Persistence API (JPA) 的陷阱
- 使用 EJB 3.0 Java Persistence API 设计企业应用程序
- JDBC、Hibernate、Java Persistence