vue2.0 之列表渲染-v-for
2017-06-24 22:46
639 查看
v-for 数组渲染
App.vue代码<template> <div> <ul> <li v-for="item in list"> {{ item.name }} - {{ item.price }}</li> </ul> <ul> <li v-for="item in list" v-text="item.name + '-' + item.price"></li> </ul> <ul> <li v-for="(item, index) in list"> {{ index }} - {{ item.name }} - {{ item.price }}</li> </ul> </div> </template> <script> export default { data () { return { list: [ { name: 'apple 7S', price: 6188 }, { name: 'huawei P10', price: 4288 }, { name: 'mi6', price: 2999 } ] } } } </script> <style> html { height: 100%; } </style>
页面效果

v-for 对象渲染
App.vue代码<template> <div> <ul> <li v-for="value in objlist"> {{ value }}</li> </ul> <ul> <li v-for="(key, value) in objlist"> {{ key + ':' + value }}</li> </ul> </div> </template> <script> export default { data () { return { objlist: { name: 'apple 7S', price: 6188, color: 'red', size: 6.0 } } } } </script> <style> html { height: 100%; } </style>
页面显示
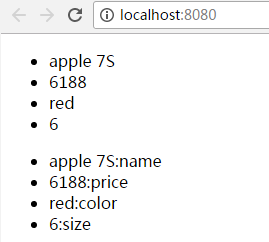
v-for 子组件渲染
创建./components/hello.vue文件<template> <div> {{ hello }} </div> </template> <script> export default { data () { return { hello: 'i am component hello' } } } </script> <style scoped>/**/ h1 { height: 100px; } </style>
App.vue代码
<template> <div> <componenthello v-for="(key, value) in objlist"></componenthello> </div> </template> <script> import componenthello from './components/hello' export default { components: { componenthello }, data () { return { objlist: { name: 'apple 7S', price: 6188, color: 'red', size: 6.0 } } } } </script> <style> html { height: 100%; } </style>
页面显示
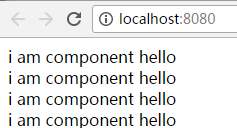
列表数据的同步更新
案例一:<template> <div> <ul> <li v-for="item in list"> {{ item.name }} - {{ item.price }}</li> </ul> <button v-on:click="addItem">addItem</button> </div> </template> <script> export default { data () { return { list: [ { name: 'apple 7S', price: 6188 }, { name: 'huawei P10', price: 4288 }, { name: 'mi6', price: 2999 } ] } }, methods: { addItem () { this.list.push({ name: 'meizu', price: 2499 }) } } } </script> <style> html { height: 100%; } </style>

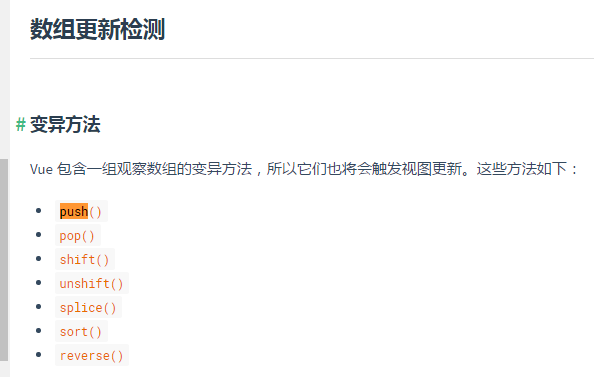
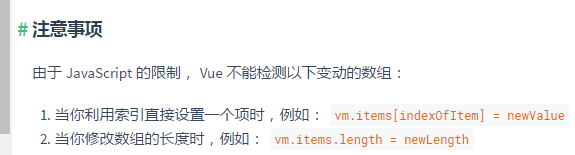
案例二
<template> <div> <ul> <li v-for="item in list"> {{ item.name }} - {{ item.price }}</li> </ul> <button v-on:click="addItem">addItem</button> </div> </template> <script> import Vue from 'vue' export default { data () { return { list: [ { name: 'apple 7S', price: 6188 }, { name: 'huawei P10', price: 4288 }, { name: 'mi6', price: 2999 } ] } }, methods: { addItem () { Vue.set(this.list, 1, { name: 'meizu', price: 2499 }) } } } </script> <style> html { height: 100%; } </style>
点击按钮前
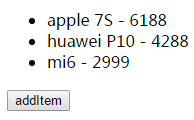
点击按钮后
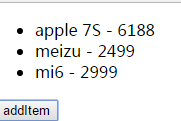
相关文章推荐
- VUE2.0全套demo讲解 基础2(列表渲染)
- Vue------第三天(条件渲染:v-if、v-show;列表渲染:v-for)
- Vue列表渲染指令v-for的索引$index报错Uncaught ReferenceError: $index is not defined
- vue2.0 渲染列表在苹果手机加载不出来的问题
- React(9)列表渲染(对标Vue的 v-for)
- VUE指令-列表渲染v-for
- vuejsLearn--- v-for列表渲染
- 8-Vue指令之列表渲染 V-for
- vue替换data里面for渲染的列表或者是循环以及替换数组的内容
- Vue.js入门学习--列表渲染--v-for遍历数组生成元素(四)
- 列表渲染 Vue2.0与Vue1.0的区别
- VUE指令-列表渲染v-for
- vue.js中的列表渲染(循环渲染)(v-for)
- vue使用v-for渲染列表属性需要:="items.attribute"绑定
- VUE2.0全套demo讲解 基础2(列表渲染)
- Vue.js中的列表渲染:v-for
- vue2.0 可折叠列表 v-for循环展示
- Vue.js学习 Item7 -- 条件渲染与列表渲染
- 在v-for中利用index来对第一项添加class(vue2.0)
- Vue.2.0.5-列表渲染