Unity调用Win32的打开和保存文件对话框
2017-06-20 16:10
676 查看
在window平台下,有时我们需要打开或则保存一些东西到指定目录下,这时我们不需要再重复造轮子,完全可以调用系统的文件窗口,具体的调用如下:
第一步:创建打开窗口和保存窗口的类。
第二步:调用刚才创建的类,使用事件系统绑定以下两个方法。
最终效果如下:
打开文件对话框
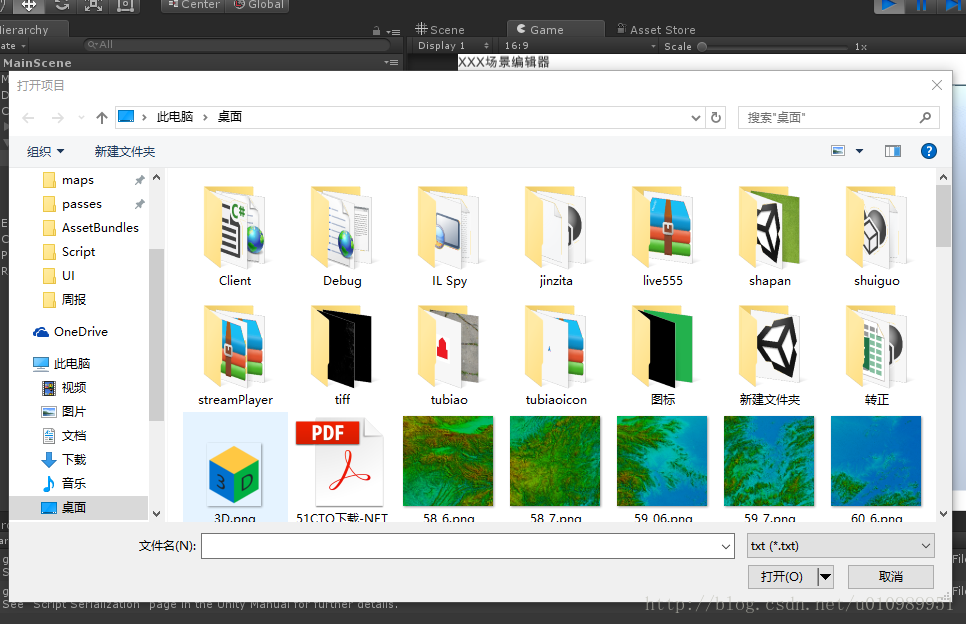
保存文件对话框:
第一步:创建打开窗口和保存窗口的类。
using UnityEngine; using System.Collections; using System.Runtime.InteropServices; using System; namespace Common { [StructLayout(LayoutKind.Sequential, CharSet = CharSet.Auto)] public class FileDlg { public int structSize = 0; public IntPtr dlgOwner = IntPtr.Zero; public IntPtr instance = IntPtr.Zero; public String filter = null; public String customFilter = null; public int maxCustFilter = 0; public int filterIndex = 0; public String file = null; public int maxFile = 0; public String fileTitle = null; public int maxFileTitle = 0; public String initialDir = null; public String title = null; public int flags = 0; public short fileOffset = 0; public short fileExtension = 0; public String defExt = null; public IntPtr custData = IntPtr.Zero; public IntPtr hook = IntPtr.Zero; public String templateName = null; public IntPtr reservedPtr = IntPtr.Zero; public int reservedInt = 0; public int flagsEx = 0; } [StructLayout(LayoutKind.Sequential, CharSet = CharSet.Auto)] public class OpenFileDlg : FileDlg { } public class OpenFileDialog { [DllImport("Comdlg32.dll", SetLastError = true, ThrowOnUnmappableChar = true, CharSet = CharSet.Auto)] public static extern bool GetOpenFileName([In, Out] OpenFileDlg ofd); } public class SaveFileDialog { [DllImport("Comdlg32.dll", SetLastError = true, ThrowOnUnmappableChar = true, CharSet = CharSet.Auto)] public static extern bool GetSaveFileName([In, Out] SaveFileDlg ofd); } [StructLayout(LayoutKind.Sequential, CharSet = CharSet.Auto)] public class SaveFileDlg: FileDlg { } }
第二步:调用刚才创建的类,使用事件系统绑定以下两个方法。
using UnityEngine; using System.Collections; using System.Collections.Generic; using System.IO; using System.Text; using Common; public class FileControllor : MonoBehaviour { public void OpenProject() { OpenFileDlg pth = new OpenFileDlg(); pth.structSize = System.Runtime.InteropServices.Marshal.SizeOf(pth); pth.filter = "txt (*.txt)"; pth.file = new string(new char[256]); pth.maxFile = pth.file.Length; pth.fileTitle = new string(new char[64]); pth.maxFileTitle = pth.fileTitle.Length; pth.initialDir = Application.dataPath; // default path pth.title = "打开项目"; pth.defExt = "txt"; pth.flags = 0x00080000 | 0x00001000 | 0x00000800 | 0x00000200 | 0x00000008; if (OpenFileDialog.GetOpenFileName(pth)) { string filepath = pth.file;//选择的文件路径; Debug.Log(filepath); } } public void SaveProject() { SaveFileDlg pth = new SaveFileDlg(); pth.structSize = System.Runtime.InteropServices.Marshal.SizeOf(pth); pth.filter = "txt (*.txt)"; pth.file = new string(new char[256]); pth.maxFile = pth.file.Length; pth.fileTitle = new string(new char[64]); pth.maxFileTitle = pth.fileTitle.Length; pth.initialDir = Application.dataPath; // default path pth.title = "保存项目"; pth.defExt = "txt"; pth.flags = 0x00080000 | 0x00001000 | 0x00000800 | 0x00000200 | 0x00000008; if (SaveFileDialog.GetSaveFileName(pth)) { string filepath = pth.file;//选择的文件路径; Debug.Log(filepath); } } }
最终效果如下:
打开文件对话框
保存文件对话框:
相关文章推荐
- 一个Win32SDK的通用的打开、保存文件的对话框的调用
- 打开和保存文件对话框 调用动态库
- Win32 api使用中调用GetOpenFileName打开文件对话框无响应的解决方法
- 调用IE内部命令实现文件打开、保存对话框
- win32 api 调用浏览文件 保存文件对话框 以及浏览文件夹对话框
- VC++中打开文件和保存文件对话框的调用
- VC++中打开文件和保存文件对话框的调用
- win32api调用打开/保存文件对话框
- win32 SDK开发中打开 保存文件对话框的使用
- win32 api 调用浏览文件 保存文件对话框 以及浏览文件夹对话框
- [Win32]打开文件/保存文件/选择文件夹对话框
- 打开和保存文件对话框 调用动态库
- vba使用win32 API(GetOpenFileName )实现打开文件对话框
- vba使用win32 API(GetOpenFileName )实现打开文件对话框
- Vb中不用控件调用文件打开对话框
- vba使用win32 API(GetOpenFileName )实现打开文件对话框
- C#学习笔记(十六):使用打开保存文件对话框
- vba使用win32 API(GetOpenFileName )实现打开文件对话框
- vba使用win32 API(GetOpenFileName )实现打开文件对话框
- 一个WinForm记事本程序(包含主/下拉/弹出菜单/打开文件/保存文件/打印/页面设置/字体/颜色对话框/剪切版操作等等控件用法以及记事本菜单事件/按键事件的具体代码)