Android单击通知栏后返回正在运行的程序并跳转到指定fragment页面
2017-05-27 15:44
826 查看
今天写通知栏的时候遇到了这样的一个问题:在fragment中发送通知,点击通知后跳转到指定的fragment。
第一思路就是创建一个通知,点击通知后启动activity,在activity接受传入过来的值来判断启动那个fragment。
既然有思路了,就开始写吧。代码如下:
activity_main.xml:
MainActivity.class:
NotifyFragment.class:
fragment_notify.xml:
跳转后的fragment
xml:
运行结果:
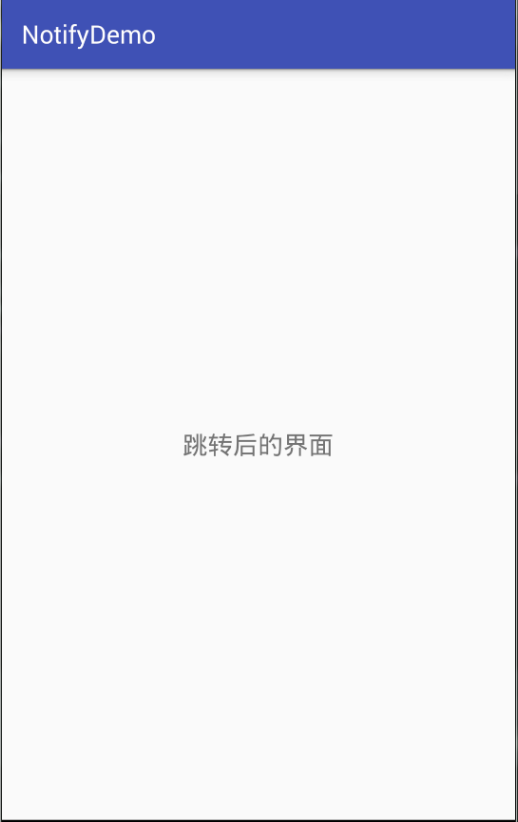
成功接受到结果,页面也跳转了,但是单击返回键的时候会返回上个activity。正常的思路应该是在当前程序里点击通知直接跳转到指定fragment上,这样写不符合要求。想了下,是不是没有设置flag的原因?
结合网上资料后代码如下:
运行效果如下:
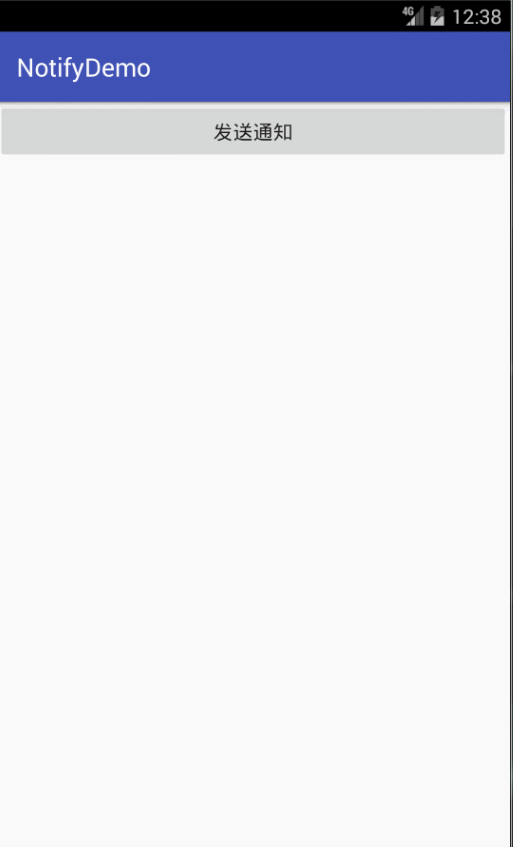
但是这样又出现一个问题:在软件界面上点击是没有问题,但是无法接受到传过来的参数,退出去后点通知能够接受到参数。也就是说onResume()方法没有被执行。
在网上看到这篇文章:http://blog.csdn.net/nihaoqiulinhe/article/details/50697301
通过设置Activity启动模式解决。上代码:
manifest文件添加 android:launchMode=”singleTask”
当使用Intent来启动Activity时会调用onNewIntent()方法。
MainActivity:
NotifyFragment的Intent修改如下
最后运行情况:
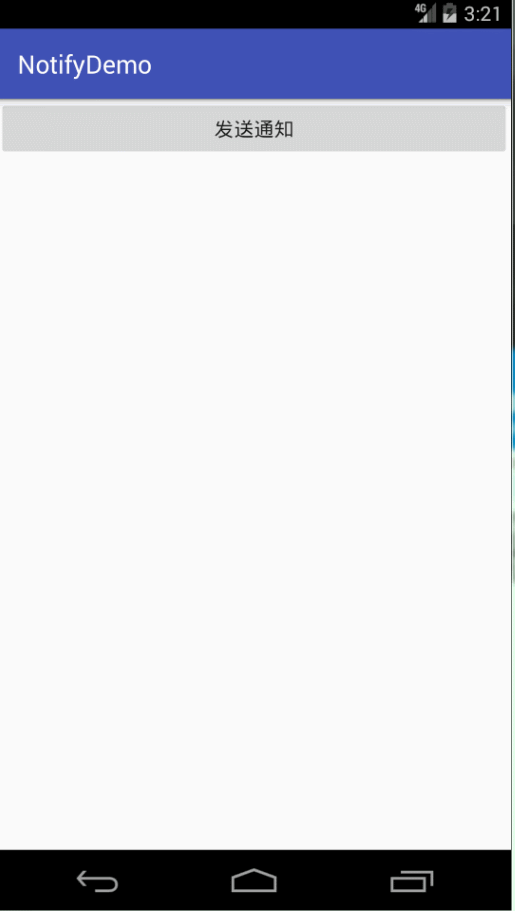
ok,三种打开方式都能进入进入页面。问题解决。
完整代码下载:
https://github.com/HOBERHUNTER/NotifyStartIntentDemo
第一思路就是创建一个通知,点击通知后启动activity,在activity接受传入过来的值来判断启动那个fragment。
既然有思路了,就开始写吧。代码如下:
activity_main.xml:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <FrameLayout android:layout_width="match_parent" android:layout_height="match_parent" android:id="@+id/fragment_index"/> </LinearLayout>
MainActivity.class:
public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); getSupportFragmentManager().beginTransaction().replace(R.id.fragment_index,new NotifyFragment()).commit(); } @Override protected void onResume() { Intent intent = getIntent(); String value = intent.getStringExtra("toValue"); Log.i("TAG", "onResume: " + value); if(!TextUtils.isEmpty(value)) { switch (value) { case "href": getSupportFragmentManager().beginTransaction().replace(R.id.fragment_index, new HrefFragment()).commit(); break; } } super.onResume(); } }
NotifyFragment.class:
public class NotifyFragment extends Fragment { private View view; private Button btnNotify; @Nullable @Override public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { view = inflater.inflate(R.layout.fragment_content,container,false); btnNotify = (Button) view.findViewById(R.id.btn_notify); btnNotify.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN) { Intent intent = new Intent(getContext(),MainActivity.class); intent.putExtra("toValue","href"); PendingIntent pend = PendingIntent.getActivity(getContext(),201,intent,PendingIntent.FLAG_UPDATE_CURRENT); Notification notify = new Notification.Builder(getContext()) .setWhen(System.currentTimeMillis()) .setSmallIcon(R.mipmap.ic_launcher) .setContentTitle("这是标题") .setContentText("这是内容") .setContentIntent(pend) .build(); notify.flags = Notification.FLAG_AUTO_CANCEL; NotificationManager manager = (NotificationManager) getContext().getSystemService(Context.NOTIFICATION_SERVICE); manager.notify(1,notify); } } }); return view; } }
fragment_notify.xml:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/btn_notify" android:textSize="16sp" android:text="发送通知" /> </LinearLayout>
跳转后的fragment
public class HrefFragment extends Fragment{ private View view; @Nullable @Override public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { view = inflater.inflate(R.layout.fragment_href,container,false); return view; } }
xml:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:id="@+id/text_href" android:textSize="20sp" android:text="跳转后的界面" android:gravity="center" /> </LinearLayout>
运行结果:
05-27 00:15:38.040 17430-17430/com.example.administrator.notifydemo I/TAG: onResume: href
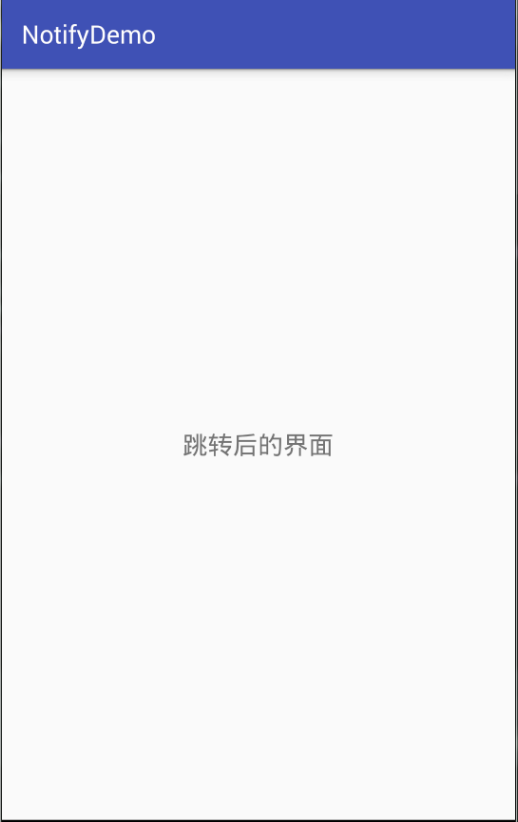
成功接受到结果,页面也跳转了,但是单击返回键的时候会返回上个activity。正常的思路应该是在当前程序里点击通知直接跳转到指定fragment上,这样写不符合要求。想了下,是不是没有设置flag的原因?
结合网上资料后代码如下:
btnNotify.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN) { //Intent intent = new Intent(getContext(),MainActivity.class); //判断当前activity是否创建 Intent intent = new Intent(Intent.ACTION_MAIN); intent.putExtra("toValue","href"); //====增加部分 intent.addCategory(Intent.CATEGORY_LAUNCHER); intent.setComponent(new ComponentName(getContext(), MainActivity.class)); intent.setFlags( Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_RESET_TASK_IF_NEEDED); PendingIntent pend = PendingIntent.getActivity(getContext(),201,intent,PendingIntent.FLAG_UPDATE_CURRENT); Notification notify = new Notification.Builder(getContext()) .setWhen(System.currentTimeMillis()) .setSmallIcon(R.mipmap.ic_launcher) .setContentTitle("这是标题") .setContentText("这是内容") .setContentIntent(pend) .build(); notify.flags = Notification.FLAG_AUTO_CANCEL; NotificationManager manager = (NotificationManager) getContext().getSystemService(Context.NOTIFICATION_SERVICE); manager.notify(1,notify); } } });
运行效果如下:
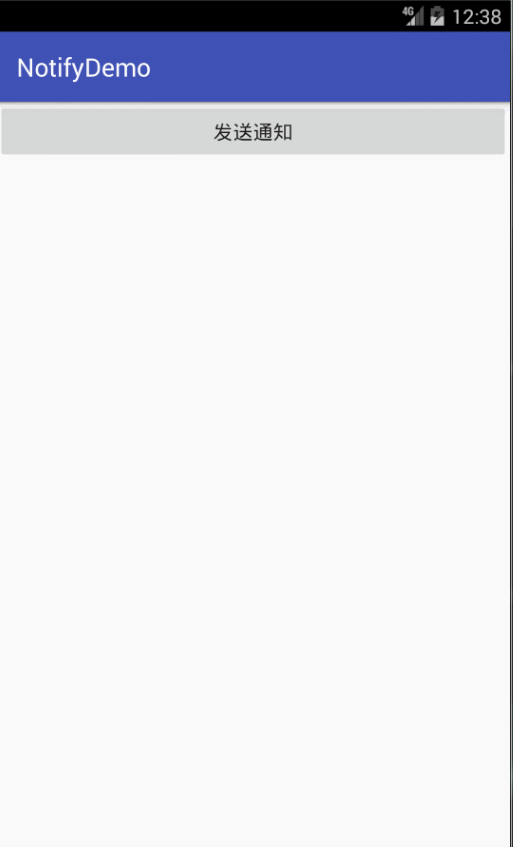
但是这样又出现一个问题:在软件界面上点击是没有问题,但是无法接受到传过来的参数,退出去后点通知能够接受到参数。也就是说onResume()方法没有被执行。
在网上看到这篇文章:http://blog.csdn.net/nihaoqiulinhe/article/details/50697301
通过设置Activity启动模式解决。上代码:
manifest文件添加 android:launchMode=”singleTask”
<activity android:name=".MainActivity" android:launchMode="singleTask"> <intent-filter> <action android:name="android.intent.action.MAIN"/> <category android:name="android.intent.category.LAUNCHER"/> </intent-filter> </activity>
当使用Intent来启动Activity时会调用onNewIntent()方法。
MainActivity:
@Override protected void onResume() { //退出应用后点击通知栏进去是进行这个方法 getNotify(getIntent()); super.onResume(); } @Override protected void onNewIntent(Intent intent) { //在应用上点击通知栏进去是执行这个方法 getNotify(intent); setIntent(intent); } private void getNotify(Intent intent){ String value = intent.getStringExtra("toValue"); Log.i("TAG", "onNewIntent: " + value); if(!TextUtils.isEmpty(value)) { switch (value) { case "href": getSupportFragmentManager().beginTransaction().replace(R.id.fragment_index, new HrefFragment()).commitAllowingStateLoss(); //这里不是用的commit提交,用的commitAllowingStateLoss方式。commit不允许后台执行,不然会报Deferring update until onResume 错误 break; } } super.onNewIntent(intent); }
NotifyFragment的Intent修改如下
Intent intent = new Intent(getContext(),MainActivity.class); intent.putExtra("toValue","href");
最后运行情况:
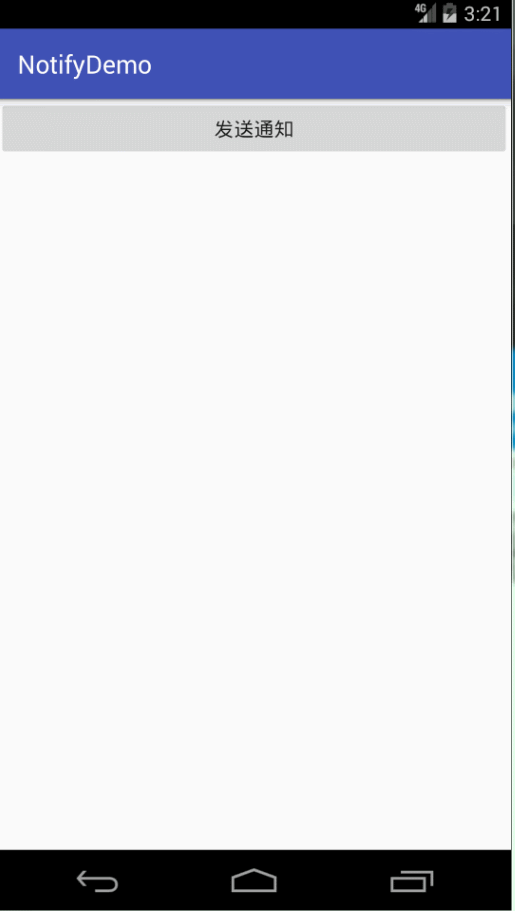
ok,三种打开方式都能进入进入页面。问题解决。
完整代码下载:
https://github.com/HOBERHUNTER/NotifyStartIntentDemo
相关文章推荐
- android开发之Intent.setFlags()_让Android点击通知栏信息后返回正在运行的程序
- android开发之Intent.setFlags()_让Android点击通知栏信息后返回正在运行的程序
- Android通知之通知栏点击通知后返回正在运行的程序,而不是一个新Activity
- Android点击通知栏信息后返回正在运行的程序,而不是一个新Activity
- android开发之让Android点击通知栏信息后返回正在运行的程序
- android服务里生成通知点击后返回正在运行的程序和当前的Activity
- android开发之Intent.setFlags()_让Android点击通知栏信息后返回正在运行的程序
- 让Android点击通知栏信息后返回正在运行的程序,并完全退出所有activity
- android开发之让Android点击通知栏信息后返回正在运行的程序
- Android点击通知栏信息后返回正在运行的程序,而不是一个新Activity
- Android中实现“程序前后台切换效果”和“返回正在运行的程序,而不是一个新Activity”
- Android中实现“程序前后台切换效果”和“返回正在运行的程序,而不是一个新Activity”
- Android卸载程序之后跳转到指定的反馈页面
- Android中实现“程序前后台切换效果”和“返回正在运行的程序,而不是一个新Activity”
- Android中实现“程序前后台切换效果”和“返回正在运行的程序,而不是一个新Activity”
- Android卸载程序之后跳转到指定的反馈页面
- Android卸载程序之后跳转到指定的反馈页面
- Android activity跳转到另一个activity的指定fragment页面
- Android 点击通知栏消息 跳转到指定的Fragment界面
- Android中实现“程序前后台切换效果”和“返回正在运行的程序,而不是一个新Activity”