设计模式之解释器模式
2017-05-05 17:48
127 查看
转自:http://blog.csdn.net/lovelion/article/details/7713593
概述:解释器模式是一种使用频率相对较低但学习难度较大的设计模式,它用于描述如何使用面向对象语言构成一个简单的语言解释器。在某些情况下,为了更好地描述某一些特定类型的问题,我们可以创建一种新的语言,这种语言拥有自己的表达式和结构,即文法规则,这些问题的实例将对应为该语言中的句子。此时,可以使用解释器模式来设计这种新的语言。对解释器模式的学习能够加深我们对面向对象思想的理解,并且掌握编程语言中文法规则的解释过程。
角色:
AbstractExpression(抽象表达式):在抽象表达式中声明了抽象的解释操作,它是所有终结符表达式和非终结符表达式的公共父类。
TerminalExpression(终结符表达式):终结符表达式是抽象表达式的子类,它实现了与文法中的终结符相关联的解释操作,在句子中的每一个终结符都是该类的一个实例。通常在一个解释器模式中只有少数几个终结符表达式类,它们的实例可以通过非终结符表达式组成较为复杂的句子。
NonterminalExpression(非终结符表达式):非终结符表达式也是抽象表达式的子类,它实现了文法中非终结符的解释操作,由于在非终结符表达式中可以包含终结符表达式,也可以继续包含非终结符表达式,因此其解释操作一般通过递归的方式来完成。
Context(环境类):环境类又称为上下文类,它用于存储解释器之外的一些全局信息,通常它临时存储了需要解释的语句。
类图:
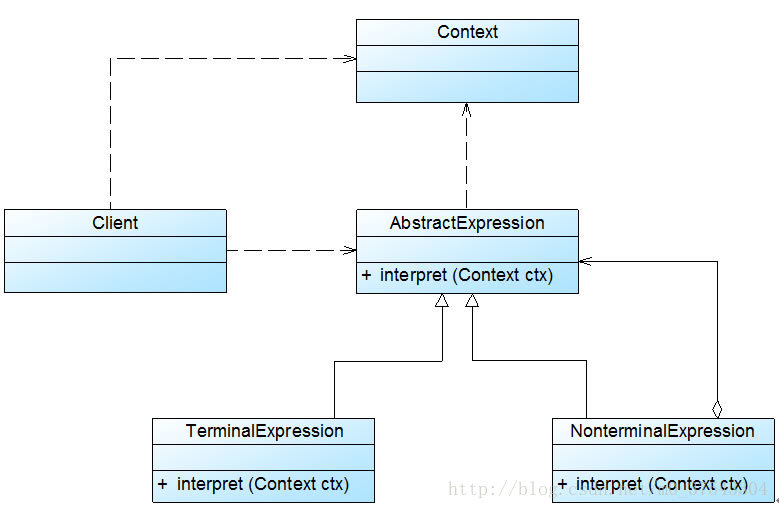
实现:
概述:解释器模式是一种使用频率相对较低但学习难度较大的设计模式,它用于描述如何使用面向对象语言构成一个简单的语言解释器。在某些情况下,为了更好地描述某一些特定类型的问题,我们可以创建一种新的语言,这种语言拥有自己的表达式和结构,即文法规则,这些问题的实例将对应为该语言中的句子。此时,可以使用解释器模式来设计这种新的语言。对解释器模式的学习能够加深我们对面向对象思想的理解,并且掌握编程语言中文法规则的解释过程。
角色:
AbstractExpression(抽象表达式):在抽象表达式中声明了抽象的解释操作,它是所有终结符表达式和非终结符表达式的公共父类。
TerminalExpression(终结符表达式):终结符表达式是抽象表达式的子类,它实现了与文法中的终结符相关联的解释操作,在句子中的每一个终结符都是该类的一个实例。通常在一个解释器模式中只有少数几个终结符表达式类,它们的实例可以通过非终结符表达式组成较为复杂的句子。
NonterminalExpression(非终结符表达式):非终结符表达式也是抽象表达式的子类,它实现了文法中非终结符的解释操作,由于在非终结符表达式中可以包含终结符表达式,也可以继续包含非终结符表达式,因此其解释操作一般通过递归的方式来完成。
Context(环境类):环境类又称为上下文类,它用于存储解释器之外的一些全局信息,通常它临时存储了需要解释的语句。
类图:
实现:
//注:本实例对机器人控制指令的输出结果进行模拟,将英文指令翻译为中文指令,实际情况是调用不同的控制程序进行机器人的控制,包括对移动方向、方式和距离的控制等 import java.util.*; //抽象表达式 abstract class AbstractNode { public abstract String interpret(); } //And解释:非终结符表达式 class AndNode extends AbstractNode { private AbstractNode left; //And的左表达式 private AbstractNode right; //And的右表达式 public AndNode(AbstractNode left, AbstractNode right) { this.left = left; this.right = right; } //And表达式解释操作 public String interpret() { return left.interpret() + "再" + right.interpret(); } } //简单句子解释:非终结符表达式 class SentenceNode extends AbstractNode { private AbstractNode direction; private AbstractNode action; private AbstractNode distance; public SentenceNode(AbstractNode direction,AbstractNode action,AbstractNode distance) { this.direction = direction; this.action = action; this.distance = distance; } //简单句子的解释操作 public String interpret() { return direction.interpret() + action.interpret() + distance.interpret(); } } //方向解释:终结符表达式 class DirectionNode extends AbstractNode { private String direction; public DirectionNode(String direction) { this.direction = direction; } //方向表达式的解释操作 public String interpret() { if (direction.equalsIgnoreCase("up")) { return "向上"; } else if (direction.equalsIgnoreCase("down")) { return "向下"; } else if (direction.equalsIgnoreCase("left")) { return "向左"; } else if (direction.equalsIgnoreCase("right")) { return "向右"; } else { return "无效指令"; } } } //动作解释:终结符表达式 class ActionNode extends AbstractNode { private String action; public ActionNode(String action) { this.action = action; } //动作(移动方式)表达式的解释操作 public String interpret() { if (action.equalsIgnoreCase("move")) { return "移动"; } else if (action.equalsIgnoreCase("run")) { return "快速移动"; } else { return "无效指令"; } } } //距离解释:终结符表达式 class DistanceNode extends AbstractNode { private String distance; public DistanceNode(String distance) { this.distance = distance; } //距离表达式的解释操作 public String interpret() { return this.distance; } } //指令处理类:工具类 class InstructionHandler { private String instruction; private AbstractNode node; public void handle(String instruction) { AbstractNode left = null, right = null; AbstractNode direction = null, action = null, distance = null; Stack stack = new Stack(); //声明一个栈对象用于存储抽象语法树 String[] words = instruction.split(" "); //以空格分隔指令字符串 for (int i = 0; i < words.length; i++) { //本实例采用栈的方式来处理指令,如果遇到“and”,则将其后的三个单词作为三个终结符表达式连成一个简单句子SentenceNode作为“and”的右表达式,而将从栈顶弹出的表达式作为“and”的左表达式,最后将新的“and”表达式压入栈中。 if (words[i].equalsIgnoreCase("and")) { left = (AbstractNode)stack.pop(); //弹出栈顶表达式作为左表达式 String word1= words[++i]; direction = new DirectionNode(word1); String word2 = words[++i]; action = new ActionNode(word2); String word3 = words[++i]; distance = new DistanceNode(word3); right = new SentenceNode(direction,action,distance); //右表达式 stack.push(new AndNode(left,right)); //将新表达式压入栈中 } //如果是从头开始进行解释,则将前三个单词组成一个简单句子SentenceNode并将该句子压入栈中 else { String word1 = words[i]; direction = new DirectionNode(word1); String word2 = words[++i]; action = new ActionNode(word2); String word3 = words[++i]; distance = new DistanceNode(word3); left = new SentenceNode(direction,action,distance); stack.push(left); //将新表达式压入栈中 } } this.node = (AbstractNode)stack.pop(); //将全部表达式从栈中弹出 } public String output() { String result = node.interpret(); //解释表达式 return result; } }
工具类InstructionHandler用于对输入指令进行处理,将输入指令分割为字符串数组,将第1个、第2个和第3个单词组合成一个句子,并存入栈中;如果发现有单词“and”,则将“and”后的第1个、第2个和第3个单词组合成一个新的句子作为“and”的右表达式,并从栈中取出原先所存句子作为左表达式,然后组合成一个And节点存入栈中。依此类推,直到整个指令解析结束。 编写如下客户端测试代码:
class Client { public static void main(String args[]) { String instruction = "up move 5 and down run 10 and left move 5"; InstructionHandler handler = new InstructionHandler(); handler.handle(instruction); String outString; outString = handler.output(); System.out.println(outString); } }
相关文章推荐
- .NET设计模式(20):解释器模式(Interpreter Pattern)
- 设计模式之解释器模式(interpreter)
- 设计模式笔记(16)---解释器模式(行为型)
- 设计模式笔记(16)---解释器模式(行为型)
- 设计模式--解释器模式(Interpreter)
- 设计模式——解释器模式
- 设计模式笔记(二十三) —— 解释器模式
- 设计模式笔记--行为型模式-之三 解释器
- 设计模式--解释器模式(Interpreter)
- 设计模式学习(十二)职责链模式-命令模式-解释器模式
- 深入浅出基于Java的解释器设计模式
- 设计模式之Interpreter(解释器)
- 设计模式之Interpreter(解释器)
- 设计模式 - Interpreter Pattern(解释器模式)
- 设计模式袖珍版 连续转载之 - Interpreter(解释器)
- 深入浅出基于Java的解释器设计模式
- 乐在其中设计模式(C#) - 解释器模式(Interpreter Pattern)
- [导入]C#面向对象设计模式纵横谈(16):(行为型模式) Interpreter 解释器模式.zip(9.14 MB)
- 设计模式--解释器模式(Interpreter)
- 设计模式(22)-解释器模式(Interpreter)