Android收缩控件,展开,收缩
2017-05-05 17:28
357 查看
今天项目需要实现一个点击展开收缩的功能,网上搜索找了个适合自己的,稍微修改了下适合项目本身需求的。
只是做记录,需要的可以参考。
不废话直接上代码。
首先main布局:
2.收缩控件布局:
3.Main代码:
4.收缩控件代码:
效果图:
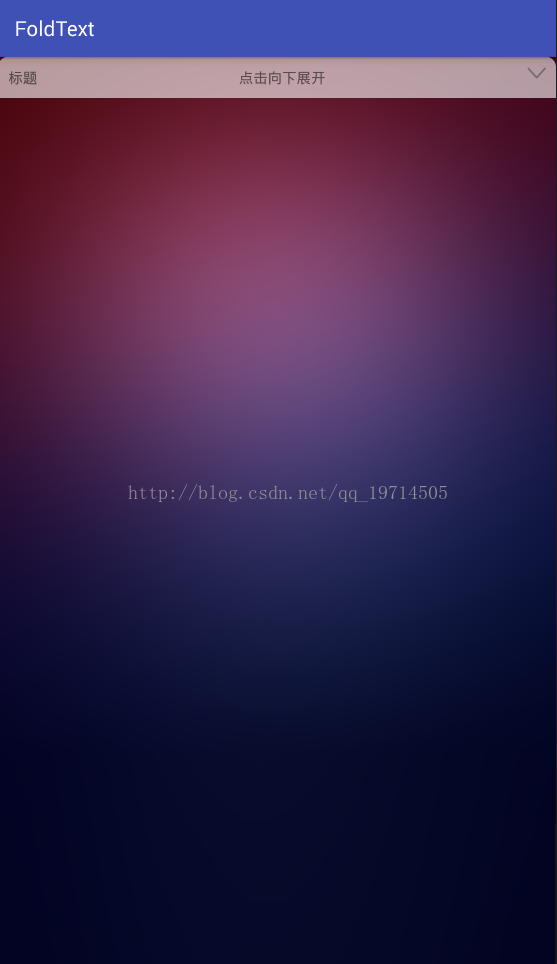
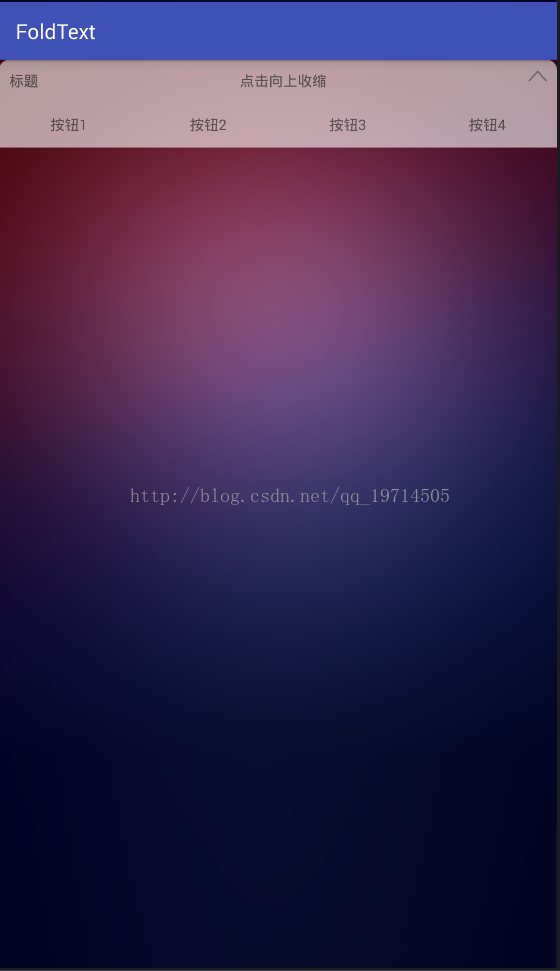
就这样完啦!
只是做记录,需要的可以参考。
不废话直接上代码。
首先main布局:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@mipmap/head" android:orientation="vertical" tools:context="com.foldtext.MainActivity"> <LinearLayout android:id="@+id/lin_tv" android:layout_width="match_parent" android:layout_height="wrap_content" android:background="@drawable/backdrop_top_style" android:orientation="horizontal"> <TextView android:id="@+id/hello1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:maxLines="2" android:padding="10dp" android:text="标题" /> <TextView android:id="@+id/textview_title" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="1" android:gravity="center" android:text="点击向下展开" /> <ImageView android:id="@+id/img_shrink" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentRight="true" android:padding="10dp" android:src="@mipmap/bottom" /> </LinearLayout> <com.foldtext.Utils.ExpandView android:id="@+id/ex_expandview" android:layout_width="match_parent" android:layout_height="wrap_content" android:visibility="gone" /> </LinearLayout>
2.收缩控件布局:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#99ffffff" android:gravity="center_horizontal" android:orientation="horizontal"> <TextView android:layout_width="match_parent" android:layout_height="45dp" android:layout_weight="1" android:gravity="center" android:text="按钮1" android:textSize="14sp" /> <TextView android:layout_width="match_parent" android:layout_height="45dp" android:layout_weight="1" android:gravity="center" android:text="按钮2" android:textSize="14sp" /> <TextView android:layout_width="match_parent" android:layout_height="45dp" android:layout_weight="1" android:gravity="center" android:text="按钮3" android:textSize="14sp" /> <TextView android:layout_width="match_parent" android:layout_height="45dp" android:layout_weight="1" android:gravity="center" android:text="按钮4" android:textSize="14sp" /> </LinearLayout>
3.Main代码:
package com.foldtext; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.ImageView; import android.widget.LinearLayout; import android.widget.TextView; import com.foldtext.Utils.ExpandView; public class MainActivity extends AppCompatActivity { private LinearLayout lin_tv; private ImageView img_shrink; private ExpandView expandView; private TextView textview_title; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); oncreateview(); ExpandView(); } /** * 初始化控件 */ private void oncreateview() { lin_tv = (LinearLayout) findViewById(R.id.lin_tv); img_shrink = (ImageView) findViewById(R.id.img_shrink); expandView = (ExpandView) findViewById(R.id.ex_expandview); textview_title = (TextView) findViewById(R.id.textview_title); } /** * 初始化调用 */ public void ExpandView() { expandView.setContentView(); lin_tv.setClickable(true); lin_tv.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (expandView.isExpand()) { expandView.collapse(); textview_title.setText("点击向下展开"); img_shrink.setImageDrawable(getResources().getDrawable(R.mipmap.bottom)); } else { expandView.expand(); textview_title.setText("点击向上收缩"); img_shrink.setImageDrawable(getResources().getDrawable(R.mipmap.shrink)); } } }); } }
4.收缩控件代码:
package com.foldtext.Utils; import android.content.Context; import android.util.AttributeSet; import android.view.LayoutInflater; import android.view.View; import android.view.animation.Animation; import android.view.animation.AnimationUtils; import android.widget.FrameLayout; import com.foldtext.R; /** * 收缩控件 */ public class ExpandView extends FrameLayout implements View.OnClickListener { private Animation mExpandAnimation; private Animation mCollapseAnimation; private boolean mIsExpand; public ExpandView(Context context) { this(context, null); } public ExpandView(Context context, AttributeSet attrs) { this(context, attrs, 0); } public ExpandView(Context context, AttributeSet attrs, int defStyle) { super(context, attrs, defStyle); initExpandView(); } private void initExpandView() { LayoutInflater.from(getContext()).inflate(R.layout.layout_expand, this, true); mExpandAnimation = AnimationUtils.loadAnimation(getContext(), R.anim.expand); mExpandAnimation.setAnimationListener(new Animation.AnimationListener() { @Override public void onAnimationStart(Animation animation) { } @Override public void onAnimationRepeat(Animation animation) { } @Override public void onAnimationEnd(Animation animation) { setVisibility(View.VISIBLE); } }); mCollapseAnimation = AnimationUtils.loadAnimation(getContext(), R.anim.collapse); mCollapseAnimation.setAnimationListener(new Animation.AnimationListener() { @Override public void onAnimationStart(Animation animation) { } @Override public void onAnimationRepeat(Animation animation) { } @Override public void onAnimationEnd(Animation animation) { setVisibility(View.GONE); } }); } public void collapse() { if (mIsExpand) { mIsExpand = false; clearAnimation(); startAnimation(mCollapseAnimation); } } public void expand() { if (!mIsExpand) { mIsExpand = true; clearAnimation(); startAnimation(mExpandAnimation); } } public boolean isExpand() { return mIsExpand; } public void setContentView() { View view = null; view = LayoutInflater.from(getContext()).inflate(R.layout.layout_expand, null); removeAllViews(); addView(view); } @Override public void onClick(View v) { switch (v.getId()) { } } }
效果图:
就这样完啦!
相关文章推荐
- Android更多条目收缩展开控件ExpandView的示例代码
- Android支持展开/收缩功能的列表控件
- Android更多条目收缩展开控件ExpandView
- Android支持展开/收缩功能的列表控件
- android 支持展开/收缩功能的列表控件
- 转载 android 支持展开/收缩功能的列表控件
- Android支持展开/收缩功能的列表控件
- Android ExpandableListView 展开列表控件
- Android列表收缩与展开仿QQ好友列表(非常详细,附源码)
- Android之可收缩展开列表ExpandableList
- Android之可收缩展开列表Expandabl…
- 使用CollapsiblePanelExtender实现展开和收缩效果以及Accordion控件
- Android之可收缩展开列表ExpandableList
- Android ExpandableListView的特殊使用——始终展开不收缩
- StretchPanel可以根据需要收缩或者展开视图的控件,使用简单方便!
- android 伸缩控件ExpandableListView 展开失败的可能原因。
- android列表收缩与展开仿QQ好友列表(非常详细,附源码)
- 实现dhtmlxTree树型控件单击展开收缩功能
- C#使用splitContainer控件制作收缩展开面板
- android 可展开(收缩)的列表ListView(ExpandableListView)