如何编写自己的JavaScript组件
2017-05-03 21:40
459 查看
相信大家在开发过程中一定简介或直接的引用了很多各种类库的组件。但是,有时候因为需求的缘故,网上的组件并不能满足我们的需求,这时候就需要我们自己来编写组件。本文以一个简单的例子,来了解一下一个组件搭建的大致流程。
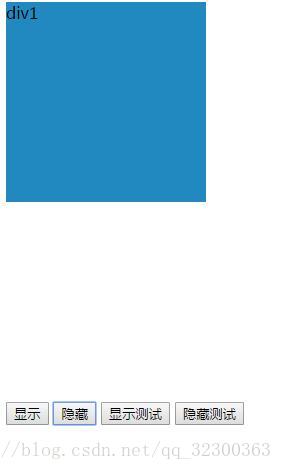
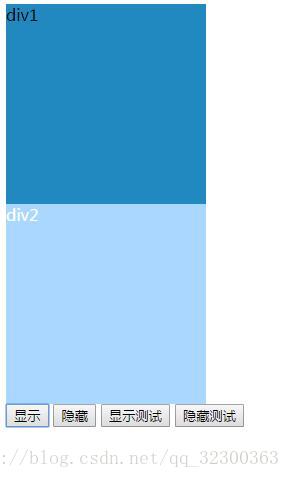
图中的隐藏和显示按钮控制div2的显示和隐藏,显示测试和隐藏测试按钮则是采用应用组件中的方法来达到和隐藏和显示按钮一样的效果。
组件的基本结构
jquery的做法是把$挂在window对象上,将其他组件挂在
$上,进一步的避免了全局变量的污染。本例只有一个组件,所以采用的做法是直接将组件挂在了window对象上。
(function(window, undefined) { //将组件的方法封装在一个类中 function JsClassName(cfg) { var config = cfg || {}; this.get = function(n) { return config ; } this.set = function(n, v) { config = v; } this.init(); } JsClassName.prototype = { init: function(){}, otherMethod: function(){} }; //将组件绑定到window对象上 window.JsClassName = window.JsClassName || JsClassName; })(window);
示例
<html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Tip</title> <style type="text/css"> #div1{ background-color: #2189c0; width: 200px; height: 200px; } #div2{ background-color: #aad7ff; color: white; width: 200px; height: 200px; visibility: hidden; } </style> </head> <body> <div id="div1">div1</div> <div id="div2">div2</div> <button id="show">显示</button> <button id="hide">隐藏</button> <button id="test1">显示测试</button> <button id="test2">隐藏测试</button> <script type="text/javascript"> (function(window, undefined) { function JsClassName(cfg) { var config = cfg || {}; this.get = function(n) { return config ; } this.set = function(n, v) { config = v; } this.init(); } JsClassName.prototype = { init: function(){ this.createDom(); this.bindEvent(); }, createDom: function(){ var div1=document.getElementById('div1'); var div2=document.getElementById('div2'); var showButton=document.getElementById('show'); var hideButton=document.getElementById('hide'); this.set("div1",div1); this.set("div2",div2); this.set("showButton",showButton); this.set("hideButton",hideButton); }, bindEvent:function(){ var that=this; var div1=that.get("div1"); var showButton=that.get("showButton"); var hideButton=that.get("hideButton"); showButton.onclick=function(){ that.show(); }; hideButton.onclick=function(){ that.hide(); }; }, show:function(){ var that=this; var div2=that.get("div2"); div2.style.visibility='visible'; }, hide:function(){ var that=this; var div2=that.get("div2"); div2.style.visibility='hidden'; } }; var obj=new JsClassName(); window.show=function(){ obj.show.call(obj) } window.hide=function(){ obj.hide.call(obj) } })(window); var button1=document.getElementById("test1"); button1.onclick=show; var button2=document.getElementById("test2"); button2.onclick=hide; </script> </body> </html>
示例图
图中的隐藏和显示按钮控制div2的显示和隐藏,显示测试和隐藏测试按钮则是采用应用组件中的方法来达到和隐藏和显示按钮一样的效果。
总结
组件就是将控制一些操作的方法封装在一起,并提供接口调用,同时也一定程度上避免了全局变量的污染。相关文章推荐
- 如何建立自己的上传组件的编程思路
- Delphi组件如何放到自己的页上
- 如何在自己编写的Plugin中使用第三方jar
- 编写组件,使用JavaScript更新UpdatePanel
- 编写组件,使用JavaScript更新UpdatePanel
- 自己编写JAVA环境下的文件上传组件
- [转帖]如何在C#中加载自己编写的DLL
- 如何编写自己的Windows登录认证模块Gina
- 如何在VC++ 编写的组件中使用 ADO
- 如何编写自己的缓冲区overflow程序
- Delph组件如何使用自己的图标
- 如何在C#中加载自己编写的动态链接库(DLL)
- 如何在C#中加载自己编写的动态链接库(DLL)
- 如何为live.com编写并添加自己的Gadget (一)
- 如何在C#中加载自己编写的动态链接库(DLL)
- DELPHI7 如何在编写可视组件中传递一个事件到组件外部?
- 如何用Delphi编写自己的可视化控件 选择自 chenbin165 的 Blog
- 如何为live.com编写并添加自己的Gadget
- 自己动手编写devExpress组件自动安装程序
- 如何在linux下编写自己得rpc调用