c++ 标准输入输出流基础
2017-04-12 10:33
155 查看
有三个重要的对象
cin cout cerr
以及一些manipulator有的带参数,有的不带参数。
Default format:
prec | pi | speed of light | fine-structure
-------------------------------------------------------
0 | 3 | 3E+08 | 0.007
2 | 3.1 | 3E+08 | 0.0073
4 | 3.142 | 2.998E+08 | 0.007257
6 | 3.14159 | 2.99792E+08 | 0.00725735
Fixed format:
prec | pi | speed of light | fine-structure
-------------------------------------------------------
0 | 3 | 299792458 | 0
2 | 3.14 | 299792458.00 | 0.01
4 | 3.1416 | 299792458.0000 | 0.0073
6 | 3.141593 | 299792458.000000 | 0.007257
Scientific format:
prec | pi | speed of light | fine-structure
-------------------------------------------------------
0 | 3E+00 | 3E+08 | 7E-03
2 | 3.14E+00 | 3.00E+08 | 7.26E-03
4 | 3.1416E+00 | 2.9979E
4000
+08 | 7.2574E-03
6 | 3.141593E+00 | 2.997925E+08 | 7.257352E-03
file操作
Reading or writing a file in C++ requires the following steps.
1. Declare a stream variable to refer to the file.
2. Open the file.
If however, the name of the file is stored in a string variable named filename, you will need to open the file like this:
infile.open(filename.c_str());
3. Transfer the data.
4. Close the file.
infile.close();
单个字符操作
Input stream in the C++ library support reading a single charactor using a method called get, which exists in two forms.
char ch;
infile.get(ch);
The general pattern for reading all the characters in a file looks like this:
另外一种写法
还有一种写法
For output streams, the put method takes a char values as its argument and writes that character to the stream.
文本需要放到生成可执行程序相同的目录下,它是读取整个字符串,并没有解析的功能。
Input file: test.txt
Die gyre and gimble in the wabe;
All misy were the borogoves,
And the mome raths outgrabe.
The open call, for example, need to use c_str to convert the C++ string stored in filename to the old-style C string that the stream library requires. Similarly, the call to clear inside the while loop is necessary to ensure that the failure status indicator
in the stream is reset before the user enters a new file name.
The effect of this call is to "push" the most recent character back into the input stream so that it is returned on the text call to get.
Calling
copies the next line of the file into the variable str, up to but not including the newline character that signals the end of the line.
从文件中获取数值
The crux of the problem is that the extraction operator in the expression
will set the failure indicator in either of two case.
1. Reaching the end of the file, at which point there are no more value to read.
2. Trying to read data from the file that cannot be convert to an integer.
可以进行错误检查
The error doesn't provide the user with much guidance as to the source of the data error, but at least it's better than nothing.
字符串流 string streams
The existence of the class makes it possible to implement the stringToInteger method described in the last section as follows:
The following function, for example, converts an integer into a string of decimal digits:
string integerToString(int n) [
ostringstream stream;
stream << n;
return stream.str();
}
The most effective way to ensure that user input is valid is to read an entire line as a string and then convert that string to an integer.
int getInteger(sting prompt) {
int value;
string line;
while (true) {
cout << prompt;
getline(cin, line);
istringstream stream(line);
stream >> value >> ws;
if (!stream.fail() && stream.eof()) break;
cout << "Illegal integer format. Try again." << endl;
}
return value;
}
Class hierarchies
类继承
封装和继承
Of these, the most important is that classes provide a framework for encapsulation, which is the process of combining the data representation and the associated operations into a coherent whole that reveals as few details as possible about the underlying
structure.
Classes in an object-oriented language from a hierarchy in which each class automatically acquires the characteristics of the classes that precede it in the hierarchy.
The original system contained only the plant and animal kingdoms.
Each kingdom is then further broken down into the hierarchical categories of phylum, class, order, family, genus, and species.
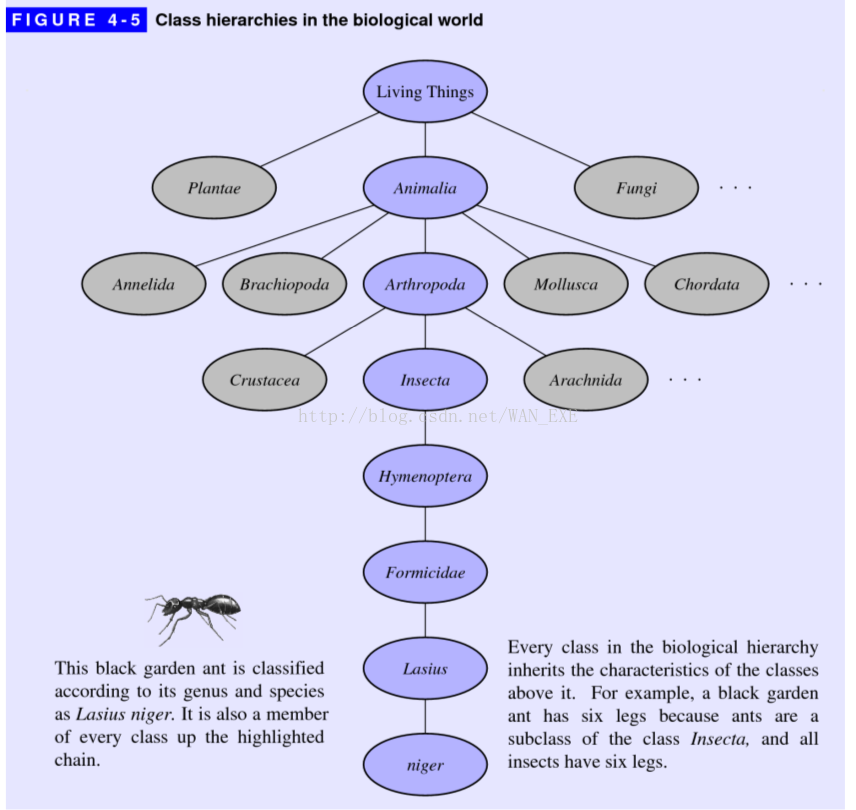
The classes in the stream libraries form hierarchies that are in many ways similar to the biological introduced in the preceding section.
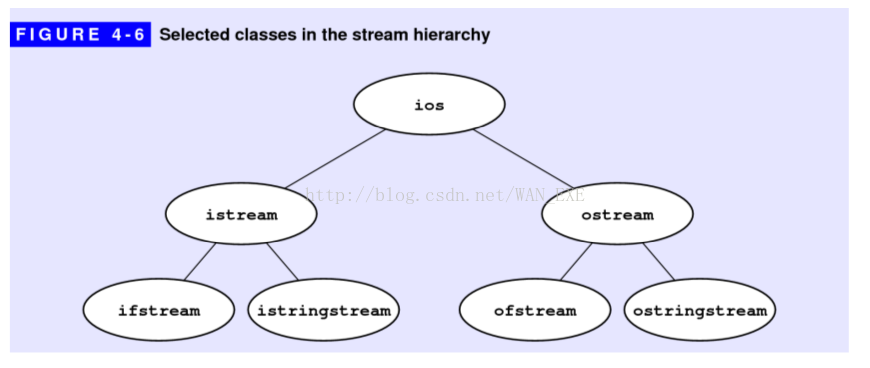
Thus, if the istream class exports a particular method, that method is automatically available to any ifstream or istringstream object.
This enhanced diagram adopts parts of a standard methodology for illustrating class hierarchies called the Universal Modeling Language, or UML for short.
Any ifstream object has access to the following methods:
The open and close methods from the ifstream class itself.
The get and upget methods and the >> operator from the istream class.
The clear, fail, and eof methods from the ios class.
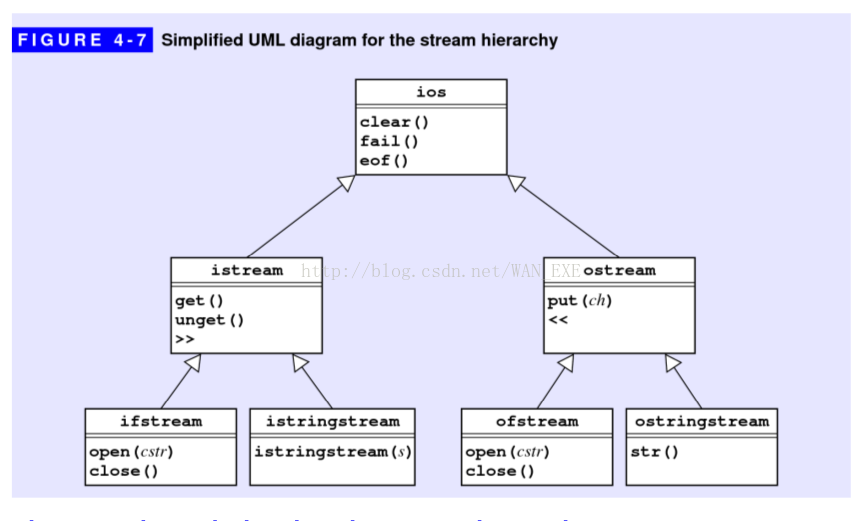
void copyStream(istream &is, ostream &os) {
char ch;
while (is.get()) {
os.put(ch);
}
}
The advantage of the new coding is that you can use this version of copyStream with any stream types.
介绍stanford引用的两个库,
simpio.h 和 filelib.h 两个库。
the simpio.h interface you have already seen and a filelib.h interface for the method that are more closely related to files.
With the expansion of the web, programmers use online reference materials more often than printed ones.
In any event, reading .h file is a programming skill that you will need to cultivate if you want to become proficient in C++.
The <iomanip> library makes it possible to control the output format. This library exports a set of manipulators.
Review Question.
问答题
cin cout cerr
以及一些manipulator有的带参数,有的不带参数。
/* * This program demonstrates various options for floating-point output * by displaying three different constants (pi, the speed of light in meters/second, * and the fine-structure constant). These constants * are chosen because they illustrate a range of exponent scales. */ #include <iostream> #include <iomanip> #include <cmath> using namespace std; const double PI = 3.1415926535897; const double SPEED_OF_LIGHT = 2.99792458e+8; const double FINE_STRUCTURE = 7.2573525e-3; void printPrecisionTable(); int main() { cout << uppercase << right; cout << "Default format:" << endl << endl; printPrecisionTable(); cout << endl << "Fixed format:" << fixed << endl << endl; printPrecisionTable(); cout << endl << "Scientific format:" << scientific << endl << endl; printPrecisionTable(); return 0; } void printPrecisionTable() { cout << "prec | pi | speed of light | fine-structure" << endl; cout << "-------------------------------------------------------" << endl; for (int prec = 0; prec <= 6; prec +=2) { cout << setw(4) << prec << " |"; cout << " " << setw(12) << setprecision(prec) << PI << " |"; cout << " " << setw(16) << setprecision(prec) << SPEED_OF_LIGHT << " |"; cout << " " << setw(14) << setprecision(prec) << FINE_STRUCTURE << endl; } }
Default format:
prec | pi | speed of light | fine-structure
-------------------------------------------------------
0 | 3 | 3E+08 | 0.007
2 | 3.1 | 3E+08 | 0.0073
4 | 3.142 | 2.998E+08 | 0.007257
6 | 3.14159 | 2.99792E+08 | 0.00725735
Fixed format:
prec | pi | speed of light | fine-structure
-------------------------------------------------------
0 | 3 | 299792458 | 0
2 | 3.14 | 299792458.00 | 0.01
4 | 3.1416 | 299792458.0000 | 0.0073
6 | 3.141593 | 299792458.000000 | 0.007257
Scientific format:
prec | pi | speed of light | fine-structure
-------------------------------------------------------
0 | 3E+00 | 3E+08 | 7E-03
2 | 3.14E+00 | 3.00E+08 | 7.26E-03
4 | 3.1416E+00 | 2.9979E
4000
+08 | 7.2574E-03
6 | 3.141593E+00 | 2.997925E+08 | 7.257352E-03
file操作
Reading or writing a file in C++ requires the following steps.
1. Declare a stream variable to refer to the file.
ifstream infile; ofstream outfile;
2. Open the file.
infile.open("Jabberwocky.txt");
If however, the name of the file is stored in a string variable named filename, you will need to open the file like this:
infile.open(filename.c_str());
3. Transfer the data.
4. Close the file.
infile.close();
单个字符操作
Input stream in the C++ library support reading a single charactor using a method called get, which exists in two forms.
char ch;
infile.get(ch);
The general pattern for reading all the characters in a file looks like this:
char ch; while (infile.get(ch)) { // perform some operation on the character. }
另外一种写法
while (true) { int ch = infile.get(); if (ch == EOF) break; }
还有一种写法
int ch; while ((ch = infile.get()) != EOF) { // Perform some operation on the character. }
For output streams, the put method takes a char values as its argument and writes that character to the stream.
文本需要放到生成可执行程序相同的目录下,它是读取整个字符串,并没有解析的功能。
/* * This program displays the contents of a file chosen by the user. */ #include <iostream> #include <fstream> #include <string> #include "console.h" using namespace std; string promptUserForFile(ifstream &infile, string prompt = ""); int main() { ifstream infile; promptUserForFile(infile, "Input file: "); char ch; while (infile.get(ch)) { cout.put(ch); } infile.close(); return 0; } string promptUserForFile(ifstream &infile, string prompt) { while (true) { cout << prompt; string filename; getline(cin, filename); infile.open(filename.c_str()); if (!infile.fail()) return filename; infile.clear(); cout << "Unable to open that file. Try again." << endl; if (prompt == "") prompt = "Input file: "; } }
Input file: test.txt
Die gyre and gimble in the wabe;
All misy were the borogoves,
And the mome raths outgrabe.
The open call, for example, need to use c_str to convert the C++ string stored in filename to the old-style C string that the stream library requires. Similarly, the call to clear inside the while loop is necessary to ensure that the failure status indicator
in the stream is reset before the user enters a new file name.
infile.unget();
The effect of this call is to "push" the most recent character back into the input stream so that it is returned on the text call to get.
Calling
getline(infile, str);
copies the next line of the file into the variable str, up to but not including the newline character that signals the end of the line.
string line; while (getline(infile, line)) { cout << line << endl; }
从文件中获取数值
int value; while (infile >> value) { total += value; }
The crux of the problem is that the extraction operator in the expression
infile >> value;
will set the failure indicator in either of two case.
1. Reaching the end of the file, at which point there are no more value to read.
2. Trying to read data from the file that cannot be convert to an integer.
可以进行错误检查
if (!infile.eof()) { error("Data error in file"); }
The error doesn't provide the user with much guidance as to the source of the data error, but at least it's better than nothing.
字符串流 string streams
The existence of the class makes it possible to implement the stringToInteger method described in the last section as follows:
int stringToInteger(string str) { istringstream stream(str); int value; stream >> value >> ws; if (stream.fail() || !stream.eof()) { error("stringToInteger: Illegal integer format"); } return value; }
The following function, for example, converts an integer into a string of decimal digits:
string integerToString(int n) [
ostringstream stream;
stream << n;
return stream.str();
}
The most effective way to ensure that user input is valid is to read an entire line as a string and then convert that string to an integer.
int getInteger(sting prompt) {
int value;
string line;
while (true) {
cout << prompt;
getline(cin, line);
istringstream stream(line);
stream >> value >> ws;
if (!stream.fail() && stream.eof()) break;
cout << "Illegal integer format. Try again." << endl;
}
return value;
}
Class hierarchies
类继承
封装和继承
Of these, the most important is that classes provide a framework for encapsulation, which is the process of combining the data representation and the associated operations into a coherent whole that reveals as few details as possible about the underlying
structure.
Classes in an object-oriented language from a hierarchy in which each class automatically acquires the characteristics of the classes that precede it in the hierarchy.
The original system contained only the plant and animal kingdoms.
Each kingdom is then further broken down into the hierarchical categories of phylum, class, order, family, genus, and species.
The classes in the stream libraries form hierarchies that are in many ways similar to the biological introduced in the preceding section.
Thus, if the istream class exports a particular method, that method is automatically available to any ifstream or istringstream object.
This enhanced diagram adopts parts of a standard methodology for illustrating class hierarchies called the Universal Modeling Language, or UML for short.
Any ifstream object has access to the following methods:
The open and close methods from the ifstream class itself.
The get and upget methods and the >> operator from the istream class.
The clear, fail, and eof methods from the ios class.
void copyStream(istream &is, ostream &os) {
char ch;
while (is.get()) {
os.put(ch);
}
}
The advantage of the new coding is that you can use this version of copyStream with any stream types.
介绍stanford引用的两个库,
simpio.h 和 filelib.h 两个库。
the simpio.h interface you have already seen and a filelib.h interface for the method that are more closely related to files.
With the expansion of the web, programmers use online reference materials more often than printed ones.
In any event, reading .h file is a programming skill that you will need to cultivate if you want to become proficient in C++.
The <iomanip> library makes it possible to control the output format. This library exports a set of manipulators.
Review Question.
问答题
相关文章推荐
- 黑马程序员——JAVA基础之标准输入输出流
- C++的输入和输出与标准输出流
- Java基础-22总结登录注册IO版,数据操作流,内存操作流,打印流,标准输入输出流,转换流,随机访问流,合并流,序列化流,Properties
- C++提高 10(标准输入,输出流,文件io流)
- 黑马程序员——JAVA基础——IO(一)---流概述,节点流、处理流、转换流与标准输入输出流、打印流、File文件对象、合并流
- Java_基础—标准输入输出流概述和输出语句
- C++基础学习系列--1、1的简陋版本--输入输出流与字符串变量的使用
- C++语言基础 例程 标准输入流
- c++用标准输入输出流测试数据
- C++基础:标准输入输出
- C++标准输入输出流
- C++语法基础--标准IO库--文件的输入和输出(fstream,ifstream,ofstream)
- Java基础知识强化之IO流笔记61:标准输入输出流的本质
- Java基础-22总结登录注册IO版,数据操作流,内存操作流,打印流,标准输入输出流,转换流,随机访问流,合并流,序列化流,Properties
- java语言基础(85)——标准输入输出流 和 随机访问流
- C++ 关于标准输入输出流 20180317 day8
- C++的标准输入输出流
- C++基础系列:输入输出流条件状态问题
- 【Java基础知识】IO流--标准输入输出流、打印流PrintStream
- c++的标准输入输出流