读取本地json数据实现省市区三级联动PickerView
2017-03-31 18:48
836 查看
这个功能在应用中还是用的比较多的,这里我将之前的一个电商项目中使用到的省市区三级联动选择器的代码粘出来,需要的自取。实现UI如图:
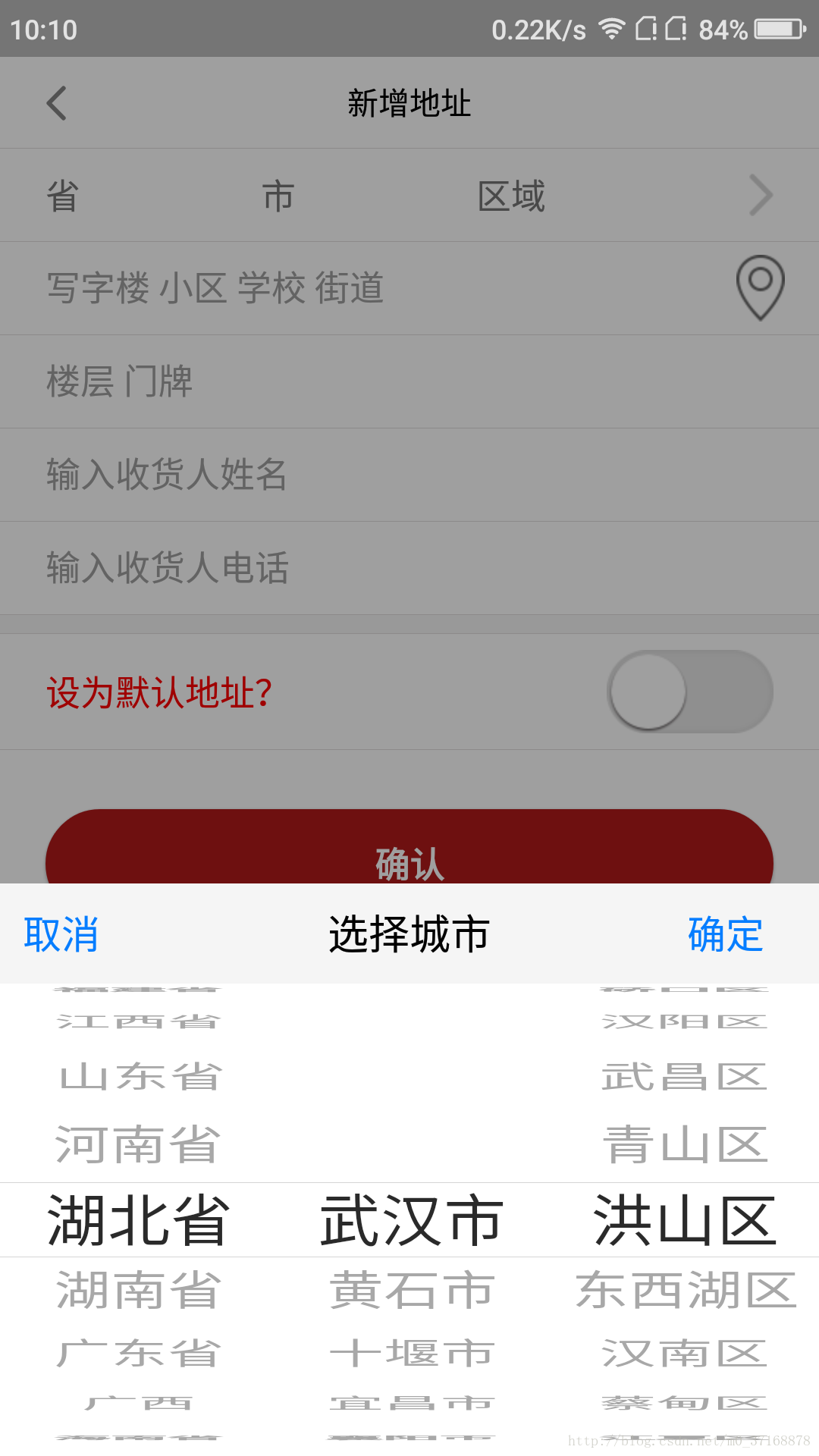
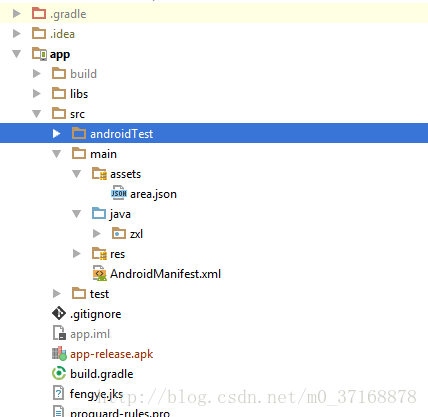
在Activity的onCreat()方法中初始化
获取json数据
将json数据赋值给PickerView
实体对象,满足伸手党《哈哈》
1、Area实体类
2、ProvinceBean
输入流的available方法和read方法
available
public int available() throws IOException
返回此输入流下一个方法调用可以不受阻塞地从此输入流读取(或跳过)的估计字节数。下一个调用可能是同一个线程,也可能是另一个线程。一次读取或跳过此估计数个字节不会受阻塞,但读取或跳过的字节数可能小于该数。
注意,有些 InputStream 的实现将返回流中的字节总数,但也有很多实现不会这样做。试图使用此方法的返回值分配缓冲区,以保存此流所有数据的做法是不正确的。
如果已经调用 close() 方法关闭了此输入流,那么此方法的子类实现可以选择抛出IOException。
类 InputStream 的 available 方法总是返回 0。
此方法应该由子类重写。
返回:
可以不受阻塞地从此输入流读取(或跳过)的估计字节数;如果到达输入流末尾,则返回 0。
抛出:
IOException - 如果发生 I/O 错误。
read
read(bytes)返回的是个整数,是每次填充给bytes数组的长度。
这个方法在按bytes数组读取文件。不等于-1是因为出现-1就说明文件已经读取结束了。
可以通过这个方法,进行循环读取文件内容,当read返回值为-1的时候,表示文件读取完毕,就可以显示文件内容,进行相应的操作。
首先是省市区的json数据
资源已经上传,在我的资源页,文件名area.json,链接如下:http://download.csdn.net/download/m0_37168878/9800543项目目录结构
使用代码
导入jar包compile 'com.bigkoo:pickerview:2.1.0'
在Activity的onCreat()方法中初始化
OptionsPickerView pvOptions = new OptionsPickerView(this);
获取json数据
private ArrayList<ProvinceBean> options1Items = new ArrayList<>(); private ArrayList<ArrayList<String>> options2Items = new ArrayList<>(); private ArrayList<ArrayList<ArrayList<String>>> options3Items = new ArrayList<>(); private void hasJsonData() { Gson gson = new Gson(); try { InputStream in = getResources().getAssets().open("area.json"); int available = in.available(); byte[] b = new byte[available]; in.read(b); String json = new String(b, "UTF-8"); //地址类 area = gson.fromJson(json, Area.class); ArrayList<Area.RootBean> citylist = area.getRoot(); for (Area.RootBean province : citylist ) { String provinceName = province.getProvince(); ArrayList<Area.RootBean.CitiesBean> c = province.getCities(); options1Items.add(new ProvinceBean(0, provinceName, "", "")); ArrayList<ArrayList<String>> options3Items_01 = new ArrayList<ArrayList<String>>(); ArrayList<String> options2Items_01 = new ArrayList<String>(); if (c != null) { for (Area.RootBean.CitiesBean area : c ) { options2Items_01.add(area.getCity()); ArrayList<Area.RootBean.CitiesBean.CountiesBean> a = area.getCounties(); ArrayList<String> options3Items_01_01 = new ArrayList<String>(); if (a != null) { for (Area.RootBean.CitiesBean.CountiesBean street : a ) { options3Items_01_01.add(street.getCounty()); } options3Items_01.add(options3Items_01_01); } else { options3Items_01_01.add(""); options3Items_01.add(options3Items_01_01); } } options2Items.add(options2Items_01); } else { options2Items_01.add(""); } options3Items.add(options3Items_01); ArrayList<String> options3Items_01_01 = new ArrayList<String>(); options3Items_01_01.add(""); options3Items_01.add(options3Items_01_01); } } catch (IOException e) { e.printStackTrace(); } }
将json数据赋值给PickerView
private void initPickerView(BDLocation mlocation) { pvOptions.setPicker(options1Items, options2Items, options3Items, true); pvOptions.setTitle("选择城市"); pvOptions.setCyclic(false, false, false); int p1 = 0; int p2 = 0; int p3 = 0; if (mlocation != null) { for (int i1 = 0; i1 < options1Items.size(); i1++) { if (options1Items.get(i1).getName().equals(mlocation.getProvince())) { p1 = i1; } for (int i2 = 0; i2 < options2Items.get(i1).size(); i2++) { if (options2Items.get(i1).get(i2).equals(mlocation.getCity())) { p2 = i2; } for (int i3 = 0; i3 < options3Items.get(i1).get(i2).size(); i3++) { if (options3Items.get(i1).get(i2).get(i3).equals(mlocation.getDistrict())) { p3 = i3; } } } } } pvOptions.setSelectOptions(p1, p2, p3); //--选中当前位置 pvOptions.setCancelable(true); pvOptions.setOnoptionsSelectListener(new OptionsPickerView.OnOptionsSelectListener() { @Override public void onOptionsSelect(int options1, int option2, int options3) { String p1 = options1Items.get(options1).getPickerViewText(); String address; if ("北京市".equals(p1) || "上海市".equals(p1) || "天津市".equals(p1) || "重庆市".equals(p1)) { //--------------baidu地理编码定位------------------------ cityLocation = options1Items.get(options1).getPickerViewText(); addressLocation = options3Items.get(options1).get(option2).get(options3); //------------------------------------------------------ address = options1Items.get(options1).getPickerViewText() + " " + options3Items.get(options1).get(option2).get(options3); provinceCode = area.getRoot().get(options1).getProvinceCode(); cityCode = area.getRoot().get(options1).getCities().get(option2).getCityCode(); countyCode = area.getRoot().get(options1).getCities().get(option2).getCounties().get(options3).getCountyCode(); } else if ("澳门".contains(p1) || "香港".contains(p1) || "台湾省".equals(p1)) { //--------------baidu地理编码定位------------------------ cityLocation = options1Items.get(options1).getPickerViewText(); addressLocation = options2Items.get(options1).get(option2); //------------------------------------------------------ address = options1Items.get(options1).getPickerViewText() + " " + options2Items.get(options1).get(option2); provinceCode = area.getRoot().get(options1).getProvinceCode(); cityCode = area.getRoot().get(options1).getCities().get(option2).getCityCode(); } else { //--------------baidu地理编码定位------------------------ // cityLocation = options1Items.get(options1).getPickerViewText()+options2Items.get(options1).get(option2); cityLocation = options2Items.get(options1).get(option2); addressLocation = options3Items.get(options1).get(option2).get(options3); //------------------------------------------------------ address = options1Items.get(options1).getPickerViewText() + " " + options2Items.get(options1).get(option2) + " " + options3Items.get(options1).get(option2).get(options3); provinceCode = area.getRoot().get(options1).getProvinceCode(); cityCode = area.getRoot().get(options1).getCities().get(option2).getCityCode(); countyCode = area.getRoot().get(options1).getCities().get(option2).getCounties().get(options3).getCountyCode(); } hintInfo.setVisibility(View.INVISIBLE); showAddress.setVisibility(View.VISIBLE); showAddress.setText(address); } }); }
实体对象,满足伸手党《哈哈》
1、Area实体类
public class Area implements Parcelable { private ArrayList<RootBean> root; protected Area(Parcel in) { } public static final Creator<Area> CREATOR = new Creator<Area>() { @Override public Area createFromParcel(Parcel in) { return new Area(in); } @Override public Area[] newArray(int size) { return new Area[size]; } }; public ArrayList<RootBean> getRoot() { return root; } public void setRoot(ArrayList<RootBean> root) { this.root = root; } @Override public int describeContents() { return 0; } @Override public void writeToParcel(Parcel dest, int flags) { } public static class RootBean { private int provinceCode; private String province; private ArrayList<CitiesBean> cities; public int getProvinceCode() { return provinceCode; } public void setProvinceCode(int provinceCode) { this.provinceCode = provinceCode; } public String getProvince() { return province; } public void setProvince(String province) { this.province = province; } public ArrayList<CitiesBean> getCities() { return cities; } public void setCities(ArrayList<CitiesBean> cities) { this.cities = cities; } /** *使用这个方法可以获取文字,并判断文字是否超长,比如“新疆维吾尔自治区”,这肯定显示不完,我们就可以截取后再显示 */ public String getPickerViewText() { return province; } public static class CitiesBean { private int cityCode; private String city; private int superCode; private ArrayList<CountiesBean> counties; public int getCityCode() { return cityCode; } public void setCityCode(int cityCode) { this.cityCode = cityCode; } public String getCity() { return city; } public void setCity(String city) { this.city = city; } public int getSuperCode() { return superCode; } public void setSuperCode(int superCode) { this.superCode = superCode; } public ArrayList<CountiesBean> getCounties() { return counties; } public void setCounties(ArrayList<CountiesBean> counties) { this.counties = counties; } public static class CountiesBean { private int countyCode; private String county; private int superCode; public int getCountyCode() { return countyCode; } public void setCountyCode(int countyCode) { this.countyCode = countyCode; } public String getCounty() { return county; } public void setCounty(String county) { this.county = county; } public int getSuperCode() { return superCode; } public void setSuperCode(int superCode) { this.superCode = superCode; } } } } }
2、ProvinceBean
public class ProvinceBean implements IPickerViewData { private long id; private String name; private String description; private String others; public ProvinceBean(long id,String name,String description,String others){ this.id = id; this.name = name; this.description = description; this.others = others; } public long getId() { return id; } public void setId(long id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getDescription() { return description; } public void setDescription(String description) { this.description = description; } public String getOthers() { return others; } public void setOthers(String others) { this.others = others; } //这个用来显示在PickerView上面的字符串,PickerView会通过反射获取getPickerViewText方法显示出来。 public String getPickerViewText() { //这里还可以判断文字超长截断再提供显示 if (name.length()>4){ return name.substring(0, 2);//字段太长截取 } return name; } }
附上项目中使用到的完整类
public class AddAddressActivity extends BaseActivity {
@Bind(R.id.iv_title_left)
ImageView ivTitleLeft;
@Bind(R.id.tv_title)
TextView tvTitle;
@Bind(R.id.iv_title_right)
ImageView ivTitleRight;
@Bind(R.id.tv_score2award)
TextView tvScore2award;
@Bind(R.id.province_address)
TextView provinceAddress;
@Bind(R.id.city_address)
TextView cityAddress;
@Bind(R.id.county_address)
TextView countyAddress;
@Bind(R.id.add_address_third_iv)
ImageView addAddressThirdIv;
@Bind(R.id.floor_door_num)
EditText floorDoorNum;
@Bind(R.id.has_detail_for_map)
ImageView hasDetailForMap;
@Bind(R.id.user_name_et)
EditText userNameEt;
@Bind(R.id.detail_address)
EditText detailAddress;
@Bind(R.id.user_tel_et)
EditText userTelEt;
@Bind(R.id.set_default_address)
CheckBox setDefaultAddress;
@Bind(R.id.address_isok_btn)
Button addressIsok;
@Bind(R.id.hint_info)
LinearLayout hintInfo;
@Bind(R.id.show_address)
TextView showAddress;
OptionsPickerView pvOptions;
private ArrayList<ProvinceBean> options1Items = new ArrayList<>();
private ArrayList<ArrayList<String>> options2Items = new ArrayList<>();
private ArrayList<ArrayList<ArrayList<String>>> options3Items = new ArrayList<>();
private Area area;
private int provinceCode;
private int cityCode;
private int countyCode;
private String addressType;
private int addressId;
private String latitude;
private String longitude;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_add_address);
ButterKnife.bind(this);
pvOptions = new OptionsPickerView(this);
//--检测是否有定位权限
if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
//去请求权限
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, 111);
} else {
initLocation();
}
hasJsonData();
initView();
}
private void initPickerView(BDLocation mlocation) { pvOptions.setPicker(options1Items, options2Items, options3Items, true); pvOptions.setTitle("选择城市"); pvOptions.setCyclic(false, false, false); int p1 = 0; int p2 = 0; int p3 = 0; if (mlocation != null) { for (int i1 = 0; i1 < options1Items.size(); i1++) { if (options1Items.get(i1).getName().equals(mlocation.getProvince())) { p1 = i1; } for (int i2 = 0; i2 < options2Items.get(i1).size(); i2++) { if (options2Items.get(i1).get(i2).equals(mlocation.getCity())) { p2 = i2; } for (int i3 = 0; i3 < options3Items.get(i1).get(i2).size(); i3++) { if (options3Items.get(i1).get(i2).get(i3).equals(mlocation.getDistrict())) { p3 = i3; } } } } } pvOptions.setSelectOptions(p1, p2, p3); //--选中当前位置 pvOptions.setCancelable(true); pvOptions.setOnoptionsSelectListener(new OptionsPickerView.OnOptionsSelectListener() { @Override public void onOptionsSelect(int options1, int option2, int options3) { String p1 = options1Items.get(options1).getPickerViewText(); String address; if ("北京市".equals(p1) || "上海市".equals(p1) || "天津市".equals(p1) || "重庆市".equals(p1)) { //--------------baidu地理编码定位------------------------ cityLocation = options1Items.get(options1).getPickerViewText(); addressLocation = options3Items.get(options1).get(option2).get(options3); //------------------------------------------------------ address = options1Items.get(options1).getPickerViewText() + " " + options3Items.get(options1).get(option2).get(options3); provinceCode = area.getRoot().get(options1).getProvinceCode(); cityCode = area.getRoot().get(options1).getCities().get(option2).getCityCode(); countyCode = area.getRoot().get(options1).getCities().get(option2).getCounties().get(options3).getCountyCode(); } else if ("澳门".contains(p1) || "香港".contains(p1) || "台湾省".equals(p1)) { //--------------baidu地理编码定位------------------------ cityLocation = options1Items.get(options1).getPickerViewText(); addressLocation = options2Items.get(options1).get(option2); //------------------------------------------------------ address = options1Items.get(options1).getPickerViewText() + " " + options2Items.get(options1).get(option2); provinceCode = area.getRoot().get(options1).getProvinceCode(); cityCode = area.getRoot().get(options1).getCities().get(option2).getCityCode(); } else { //--------------baidu地理编码定位------------------------ // cityLocation = options1Items.get(options1).getPickerViewText()+options2Items.get(options1).get(option2); cityLocation = options2Items.get(options1).get(option2); addressLocation = options3Items.get(options1).get(option2).get(options3); //------------------------------------------------------ address = options1Items.get(options1).getPickerViewText() + " " + options2Items.get(options1).get(option2) + " " + options3Items.get(options1).get(option2).get(options3); provinceCode = area.getRoot().get(options1).getProvinceCode(); cityCode = area.getRoot().get(options1).getCities().get(option2).getCityCode(); countyCode = area.getRoot().get(options1).getCities().get(option2).getCounties().get(options3).getCountyCode(); } hintInfo.setVisibility(View.INVISIBLE); showAddress.setVisibility(View.VISIBLE); showAddress.setText(address); } }); }
//---------1011zuo---------
public LocationClient mLocationClient = null;
private void initLocation() {
mLocationClient = new LocationClient(this); // 声明LocationClient类
mLocationClient.registerLocationListener(new MyLocationListener()); // 注册监听函数
LocationClientOption option = new LocationClientOption();
option.setLocationMode(LocationClientOption.LocationMode.Hight_Accuracy);// 可选,默认高精度,设置定位模式,高精度,低功耗,仅设备
// option.setCoorType("bd09ll");// 可选,默认gcj02,设置返回的定位结果坐标系-----百度使用的坐标系
option.setCoorType("gcj02");// 可选,默认gcj02,设置返回的定位结果坐标系-----高德使用的就是gcj02的坐标系
int span = 100;
option.setScanSpan(span);// 可选,默认0,即仅定位一次,设置发起定位请求的间隔需要大于等于1000ms才是有效的
option.setIsNeedAddress(true);// 可选,设置是否需要地址信息,默认不需要
option.setOpenGps(true);// 可选,默认false,设置是否使用gps
option.setLocationNotify(true);// 可选,默认false,设置是否当gps有效时按照1S1次频率输出GPS结果
option.setIsNeedLocationDescribe(true);// 可选,默认false,设置是否需要位置语义化结果,可以在BDLocation.getLocationDescribe里得到,结果类似于“在北京天安门附近”
mLocationClient.setLocOption(option);
mLocationClient.start();
}
/**
* 定位的回调
*/
private class MyLocationListener implements BDLocationListener {
@Override
public void onReceiveLocation(BDLocation location) {
initPickerView(location);
}
}
//---------1011zuo---------
private void initView() {
tvScore2award.setVisibility(View.GONE);
ivTitleRight.setVisibility(View.INVISIBLE);
Intent intent = getIntent();
addressType = intent.getStringExtra("type");
if ("add".equals(addressType)) {
tvTitle.setText("新增地址");
return;
}
if ("update".equals(addressType)) {
tvTitle.setText("修改地址");
AddressListBean addressBean = intent.getParcelableExtra("addressBean");
addressId = addressBean.getAddressId();
hintInfo.setVisibility(View.INVISIBLE);
showAddress.setVisibility(View.VISIBLE);
cityLocation = addressBean.getArea().getCity();
addressLocation = addressBean.getArea().getCounty();
showAddress.setText("" + addressBean.getArea().getProvince() + addressBean.getArea().getCity() + addressBean.getArea().getCounty());
provinceCode = addressBean.getArea().getProvinceCode();
cityCode = addressBean.getArea().getCityCode();
countyCode = addressBean.getArea().getCountyCode();
detailAddress.setText(addressBean.getAddress());
floorDoorNum.setText(addressBean.getAreas()); //门牌号
userNameEt.setText(addressBean.getContactName());
userTelEt.setText(addressBean.getContactPhone());
setDefaultAddress.setChecked(addressBean.isDefault());
return;
}
}
//从地图页面返回的位置信息
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
switch (resultCode) {
case RESULT_OK:
if (data == null) {
return;
}
Bundle bundle = data.getExtras();
String address = bundle.getString("name");
DecimalFormat df = new DecimalFormat("######0.000000");
latitude = df.format(bundle.getDouble("latitude"));
longitude = df.format(bundle.getDouble("longitude"));
detailAddress.setText(address);
break;
default:
break;
}
}
@OnClick({R.id.has_detail_for_map, R.id.iv_title_left, R.id.add_address_third_iv, R.id.set_default_address, R.id.address_isok_btn})
public void onClick(View view) {
switch (view.getId()) {
case R.id.has_detail_for_map:
//--检测是否有定位权限
if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
//去请求权限
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, 112);
} else {
chooseLocationWay();
}
break;
case R.id.iv_title_left:
hintUserSave();
// toAddressManger();
break;
case R.id.add_address_third_iv:
pvOptions.show();
break;
case R.id.address_isok_btn:
addAddress();
break;
}
}
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
switch (requestCode) {
case 112:
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
//同意
chooseLocationWay();
} else {
//拒绝
Toast.makeText(AddAddressActivity.this, "亲,定位权限被拒绝,\n请在设置中打开", Toast.LENGTH_LONG).show();
}
break;
case 111:
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
//同意
initLocation();
} else {
//拒绝
Toast.makeText(AddAddressActivity.this, "亲,定位权限被拒绝,\n请在设置中打开", Toast.LENGTH_LONG).show();
}
break;
default:
break;
}
}
//选择定位的方式---用的V7包的
private String cityLocation = "";
private String addressLocation = "";
private void chooseLocationWay() {
String[] choose = {"定位到指定的省市区", "定位到当前位置"};
final AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("选择定位方式");
builder.setItems(choose, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Intent intent = new Intent(AddAddressActivity.this, LocationMapActivity.class);
switch (which) {
case 0:
String text = (String) showAddress.getText();
if (TextUtils.isEmpty(text)) {
CommUtils.showSafeToast(AddAddressActivity.this, "请选择省市区");
return;
}
//--把地址传过去,定位
intent.putExtra("city", cityLocation);
intent.putExtra("address", addressLocation);
break;
case 1:
intent.putExtra("ctiy", "");
intent.putExtra("address", "");
break;
}
startActivityForResult(intent, 10010);
dialog.dismiss();
}
});
builder.create().show();
}
private int userId = UserInfoUtils.getUserInfoBean().getUserId();
private String address;
private String doorNum;
private String contactName;
private String contactPhone;
private boolean isDefaul;
private void addAddress() {
if (!CommUtils.isNetWorkConnected(AddAddressActivity.this)) {
ToastUtil.showShort(AddAddressActivity.this, "请检查网络", Gravity.CENTER);
return;
}
String area = showAddress.getText().toString();
address = detailAddress.getText().toString();
doorNum = floorDoorNum.getText().toString();
contactName = userNameEt.getText().toString();
contactPhone = userTelEt.getText().toString();
isDefaul = setDefaultAddress.isChecked();
if (TextUtils.isEmpty(area)) {
CommUtils.showSafeToast(this, "请选择省市区");
return;
}
if (TextUtils.isEmpty(doorNum)) {
CommUtils.showSafeToast(this, "门牌号为空");
return;
}
if (TextUtils.isEmpty(address)) {
CommUtils.showSafeToast(this, "详细地址为空");
return;
}
if (TextUtils.isEmpty(contactName)) {
CommUtils.showSafeToast(this, "收货人为空");
return;
}
if (TextUtils.isEmpty(contactPhone)) {
CommUtils.showSafeToast(this, "联系电话为空");
return;
}
if (!CommUtils.isPhoneValid(contactPhone)) {
CommUtils.showSafeToast(this, "请输入有效手机号码");
return;
}
//--------------------------
Map<String, String> options = new HashMap<>();
if ("update".equals(addressType)) {
options.put("addressId", addressId + "");
}
options.put("method", "addOrUpdate");
options.put("userId", userId + "");
options.put("provinceCode", provinceCode + "");
options.put("cityCode", cityCode + "");
options.put("countyCode", countyCode + "");
try {
URLEncoder.encode(address, "UTF-8");
URLEncoder.encode(doorNum, "UTF-8");
URLEncoder.encode(contactName, "UTF-8");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
options.put("address", address);
options.put("areas", doorNum);
options.put("contactName", contactName);
options.put("contactPhone", contactPhone);
options.put("isDefault", setDefaultAddress.isChecked() + "");
//---加上经纬度-------
options.put("longitude", longitude);
options.put("latitude", latitude);
RestClient.api().resetAddress(options).enqueue(new Callback<ReceiveData.ResetAddressResponse>() {
@Override
public void onResponse(Call<ReceiveData.ResetAddressResponse> call, Response<ReceiveData.ResetAddressResponse> response) {
if (response.body() == null) {
ToastUtil.showShort(AddAddressActivity.this, getResources().getString(R.string.response_error), Gravity.CENTER);
return;
}
if (response.body().code == 0) {
if ("add".equals(addressType)) {
CommUtils.showSafeToast(AddAddressActivity.this, "添加地址成功");
}
if ("update".equals(addressType)) {
CommUtils.showSafeToast(AddAddressActivity.this, "修改地址成功");
}
toAddressManger();
} else {
}
}
@Override
public void onFailure(Call<ReceiveData.ResetAddressResponse> call, Throwable t) {
ToastUtil.showShort(AddAddressActivity.this, getResources().getString(R.string.server_fail), Gravity.CENTER);
}
});
}
//---提示用户保存修改--不要的话就去掉吧
private void hintUserSave() {
AlertDialog chooseDialog = new AlertDialog.Builder(this).setItems(
new String[]{"不做修改,就此离开", "留下,再想想"},
new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface arg0, int arg1) {
switch (arg1) {
case 0:
toAddressManger();
break;
case 1:
default:
break;
}
}
}).create();
chooseDialog.show();
}
private void toAddressManger() {
Intent intent = new Intent();
intent.putExtra("doing", "refresh");
setResult(RESULT_OK, intent);
finish();
}
private void hasJsonData() {
Gson gson = new Gson();
try {
InputStream in = getResources().getAssets().open("area.json");
int available = in.available();
byte[] b = new byte[available];
in.read(b);
String json = new String(b, "UTF-8");
//地址类
area = gson.fromJson(json, Area.class);
ArrayList<Area.RootBean> citylist = area.getRoot();
for (Area.RootBean province : citylist
) {
String provinceName = province.getProvince();
ArrayList<Area.RootBean.CitiesBean> c = province.getCities();
options1Items.add(new ProvinceBean(0, provinceName, "", ""));
ArrayList<ArrayList<String>> options3Items_01 = new ArrayList<ArrayList<String>>();
ArrayList<String> options2Items_01 = new ArrayList<>();
if (c != null) {
for (Area.RootBean.CitiesBean area : c
) {
options2Items_01.add(area.getCity());
ArrayList<Area.RootBean.CitiesBean.CountiesBean> a = area.getCounties();
ArrayList<String> options3Items_01_01 = new ArrayList<>();
if (a != null) {
for (Area.RootBean.CitiesBean.CountiesBean street : a
) {
options3Items_01_01.add(street.getCounty());
}
options3Items_01.add(options3Items_01_01);
} else {
options3Items_01_01.add("");
options3Items_01.add(options3Items_01_01);
}
}
options2Items.add(options2Items_01);
} else {
options2Items_01.add("");
}
options3Items.add(options3Items_01);
ArrayList<String> options3Items_01_01 = new ArrayList<String>();
options3Items_01_01.add("");
options3Items_01.add(options3Items_01_01);
}
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void onBackPressed() {
hintUserSave();
}
}
输入流的available方法和read方法
available
public int available() throws IOException
返回此输入流下一个方法调用可以不受阻塞地从此输入流读取(或跳过)的估计字节数。下一个调用可能是同一个线程,也可能是另一个线程。一次读取或跳过此估计数个字节不会受阻塞,但读取或跳过的字节数可能小于该数。
注意,有些 InputStream 的实现将返回流中的字节总数,但也有很多实现不会这样做。试图使用此方法的返回值分配缓冲区,以保存此流所有数据的做法是不正确的。
如果已经调用 close() 方法关闭了此输入流,那么此方法的子类实现可以选择抛出IOException。
类 InputStream 的 available 方法总是返回 0。
此方法应该由子类重写。
返回:
可以不受阻塞地从此输入流读取(或跳过)的估计字节数;如果到达输入流末尾,则返回 0。
抛出:
IOException - 如果发生 I/O 错误。
read
read(bytes)返回的是个整数,是每次填充给bytes数组的长度。
这个方法在按bytes数组读取文件。不等于-1是因为出现-1就说明文件已经读取结束了。
可以通过这个方法,进行循环读取文件内容,当read返回值为-1的时候,表示文件读取完毕,就可以显示文件内容,进行相应的操作。
相关文章推荐
- WheelView实现省市区三级联动(数据库实现版本号附带完整SQL及数据)
- Android使用开源框架Citypickerview实现省市区三级联动效果
- Android中使用开源框架Citypickerview实现省市区三级联动选择
- Android中使用开源框架citypickerview实现省市区三级联动选择
- 安卓学习笔记---Android-PickerView实现 3D滚轮效果(时间选择器、省市区三级联动,单项选择效果)
- javascript实现省市区三级联动选择的代码(数据为模拟json数据)
- WheelView实现省市区三级联动(数据库实现版本附带完整SQL及数据)
- jQuery 读取XML数据实现省市区三级联动
- Android中使用开源框架citypickerview实现省市区三级联动选择
- WheelView实现省市区三级联动(数据库实现版本号附带完整SQL及数据)
- Android中使用开源框架Citypickerview实现省市区三级联动选择
- 用js读取XML数据实现省市区的三级联动
- BaseAdapter,这篇博客讲深入一些,实现从本地的JSON文件读取数据
- jquery从数据库中获取数据装换成json数据实现三级联动
- jQuery读取json文件,实现省市区/县(国标)三级联动
- 怎样使用pickerview来实现地址菜单的三级联动效果
- JSON 数据,实现省市县三级联动下拉菜单
- 省市区三级联动下拉菜单的实现(含最新行政区划数据)
- Android省市区三级联动滚轮控件,使用本地数据库数据
- 用DropDownList控件绑定XML数据实现省市区三级联动