ListFragment 使用ListView and 自定义Adapter
2017-03-25 00:00
381 查看
在开发过程中经常使用Tabs + ListFragment 作为表现形式。
ListFragment 中加入ListView显示方式很容易。
[java]
view plain
copy


package project.animalsound;
import android.app.ListFragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ListView;
public class TabFirst extends ListFragment {
private AnimalListAdapter adapter = null;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
adapter = new AnimalListAdapter (getActivity());
setListAdapter(adapter);
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View v = inflater.inflate(R.layout.tab_first, container, false);
return v;
}
@Override
public void onListItemClick(ListView l, View v, int position, long id) {
System.out.println("Click On List Item!!!");
super.onListItemClick(l, v, position, id);
}
}
只要在onCreateView中增加
[java]
view plain
copy


View v = inflater.inflate(R.layout.tab_first, container, false);
就可以完成。
对应的R.layout.tab_first为:
[html]
view plain
copy


<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<ListView
android:id="@+id/android:list"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
</RelativeLayout>
在这里我们对于ListView上的每个Item的布局使用下面布局
user.xml
[java]
view plain
copy


<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="horizontal" >
<ImageView
android:id="@+id/animal"
android:layout_width="wrap_content"
android:layout_height="80dp"
android:layout_alignParentLeft="true"
android:layout_centerVertical="true"
android:layout_margin="1dp" />
<LinearLayout
android:layout_height="fill_parent"
android:layout_width="wrap_content"
android:layout_marginLeft="20dp"
android:layout_toRightOf="@id/animal"
android:layout_centerVertical="true"
android:orientation="vertical" >
<TextView
android:id="@+id/cn_word"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:textColor="#191970"
android:textSize="30sp" />
<TextView
android:id="@+id/en_word"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:textColor="#800080"
android:textSize="30sp"/>
</LinearLayout>
<ImageView
android:id="@+id/speaker"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentRight="true"
android:layout_margin="15dp"
android:layout_centerVertical="true"
android:src="@drawable/speaker"
/>
</RelativeLayout>
但是在实现的时候常会遇到一个问题,如果相对其中的一个ImageView增加OnClickListener的时候会出现问题。
我们无法在下句获得的View对象中使用findViewById(R.id.speaker)去获得对应的ID对象。
[java]
view plain
copy


View v = inflater.inflate(R.layout.tab_first, container, false);
原因是这句只是获得了R.layout.tab_first对应的View对象。
所以需要从user.xml获得对象信息。
这里可以使用Adapter轻松完成注册Listener的过程。我们继承BaseAdapter,然后在getView中实现整个初始化的过程。
[java]
view plain
copy


package project.animalsound;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.TextView;
class ViewHolder {
public ImageView animal;
public TextView cn_word;
public TextView en_word;
public ImageView speaker;
}
public class AnimalListAdapter extends BaseAdapter {
private LayoutInflater mInflater = null;
public AnimalListAdapter(Context context){
super();
mInflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
}
@Override
public int getCount() {
// TODO Auto-generated method stub
return 50;
}
@Override
public Object getItem(int position) {
// TODO Auto-generated method stub
return null;
}
@Override
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder = null;
if (convertView == null) {
holder = new ViewHolder();
convertView = mInflater.inflate(R.layout.user, null);
holder.animal = (ImageView) convertView.findViewById(R.id.animal);
holder.cn_word = (TextView) convertView.findViewById(R.id.cn_word);
holder.en_word = (TextView) convertView.findViewById(R.id.en_word);
holder.speaker = (ImageView) convertView.findViewById(R.id.speaker);
convertView.setTag(holder);
} else {
holder = (ViewHolder) convertView.getTag();
}
holder.animal.setImageResource(R.drawable.ic_launcher);
holder.cn_word.setText("xxxxx");
holder.en_word.setText("ssssss");
holder.speaker.setImageResource(R.drawable.speaker);
holder.speaker.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View v) {
System.out.println("Click on the speaker image on ListItem ");
}
});
return convertView;
}
}
ListFragment 中加入ListView显示方式很容易。
[java]
view plain
copy

package project.animalsound;
import android.app.ListFragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ListView;
public class TabFirst extends ListFragment {
private AnimalListAdapter adapter = null;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
adapter = new AnimalListAdapter (getActivity());
setListAdapter(adapter);
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View v = inflater.inflate(R.layout.tab_first, container, false);
return v;
}
@Override
public void onListItemClick(ListView l, View v, int position, long id) {
System.out.println("Click On List Item!!!");
super.onListItemClick(l, v, position, id);
}
}
只要在onCreateView中增加
[java]
view plain
copy

View v = inflater.inflate(R.layout.tab_first, container, false);
就可以完成。
对应的R.layout.tab_first为:
[html]
view plain
copy

<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<ListView
android:id="@+id/android:list"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
</RelativeLayout>
在这里我们对于ListView上的每个Item的布局使用下面布局
user.xml
[java]
view plain
copy

<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="horizontal" >
<ImageView
android:id="@+id/animal"
android:layout_width="wrap_content"
android:layout_height="80dp"
android:layout_alignParentLeft="true"
android:layout_centerVertical="true"
android:layout_margin="1dp" />
<LinearLayout
android:layout_height="fill_parent"
android:layout_width="wrap_content"
android:layout_marginLeft="20dp"
android:layout_toRightOf="@id/animal"
android:layout_centerVertical="true"
android:orientation="vertical" >
<TextView
android:id="@+id/cn_word"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:textColor="#191970"
android:textSize="30sp" />
<TextView
android:id="@+id/en_word"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:textColor="#800080"
android:textSize="30sp"/>
</LinearLayout>
<ImageView
android:id="@+id/speaker"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentRight="true"
android:layout_margin="15dp"
android:layout_centerVertical="true"
android:src="@drawable/speaker"
/>
</RelativeLayout>
但是在实现的时候常会遇到一个问题,如果相对其中的一个ImageView增加OnClickListener的时候会出现问题。
我们无法在下句获得的View对象中使用findViewById(R.id.speaker)去获得对应的ID对象。
[java]
view plain
copy

View v = inflater.inflate(R.layout.tab_first, container, false);
原因是这句只是获得了R.layout.tab_first对应的View对象。
所以需要从user.xml获得对象信息。
这里可以使用Adapter轻松完成注册Listener的过程。我们继承BaseAdapter,然后在getView中实现整个初始化的过程。
[java]
view plain
copy

package project.animalsound;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.TextView;
class ViewHolder {
public ImageView animal;
public TextView cn_word;
public TextView en_word;
public ImageView speaker;
}
public class AnimalListAdapter extends BaseAdapter {
private LayoutInflater mInflater = null;
public AnimalListAdapter(Context context){
super();
mInflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
}
@Override
public int getCount() {
// TODO Auto-generated method stub
return 50;
}
@Override
public Object getItem(int position) {
// TODO Auto-generated method stub
return null;
}
@Override
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder = null;
if (convertView == null) {
holder = new ViewHolder();
convertView = mInflater.inflate(R.layout.user, null);
holder.animal = (ImageView) convertView.findViewById(R.id.animal);
holder.cn_word = (TextView) convertView.findViewById(R.id.cn_word);
holder.en_word = (TextView) convertView.findViewById(R.id.en_word);
holder.speaker = (ImageView) convertView.findViewById(R.id.speaker);
convertView.setTag(holder);
} else {
holder = (ViewHolder) convertView.getTag();
}
holder.animal.setImageResource(R.drawable.ic_launcher);
holder.cn_word.setText("xxxxx");
holder.en_word.setText("ssssss");
holder.speaker.setImageResource(R.drawable.speaker);
holder.speaker.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View v) {
System.out.println("Click on the speaker image on ListItem ");
}
});
return convertView;
}
}
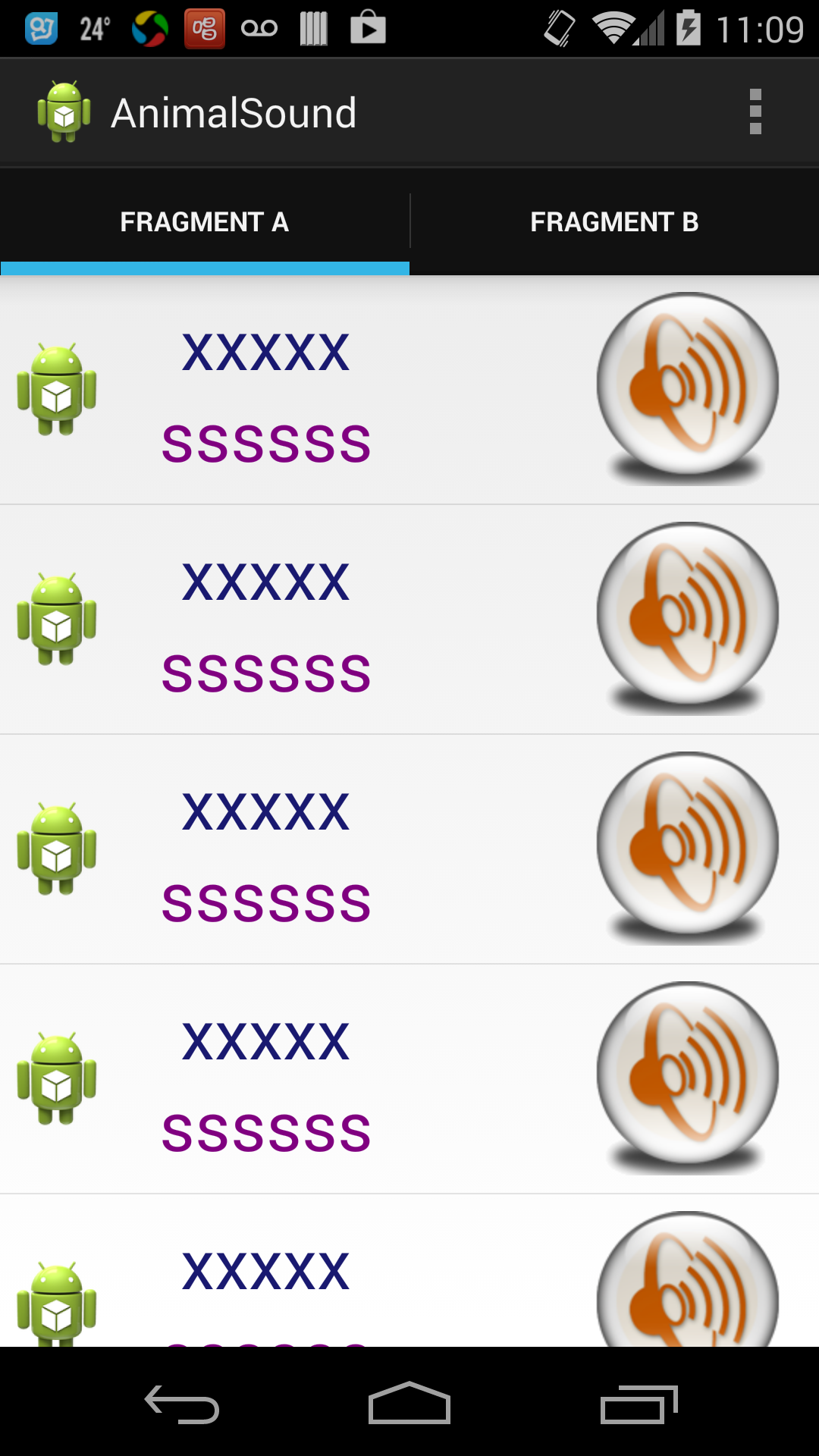
相关文章推荐
- ListFragment 使用ListView and 自定义Adapter
- ListFragment 使用ListView and 自定义Adapter
- ListFragment 使用ListView and 自定义Adapter(转载)
- ListFragment使用ListView和自定义Adapter
- fragment、ListFragment使用ListView及自定义Listview等初始化操作
- ListFragment 使用ListView and 自定义Adapter
- fragment、ListFragment使用ListView及自定义Listview等初始化操作
- fragment、ListFragment使用ListView及自定义Listview等初始化操作
- fragment、ListFragment使用ListView及自定义Listview等初始化操作
- fragment、ListFragment使用ListView及自定义Listview等初始化操作
- fragment、ListFragment使用ListView及自定义Listview等初始化操作
- 在ListView上使用CheckBox的自定义Adapter写法
- ListView的Adapter使用(绑定数据) 之 自定义每一项的布局去绑定数据
- ListView的Adapter使用(绑定数据) 之 自定义每一项的布局去绑定数据(一)
- android ListView布局之三(使用自定义的Adapter绑定数据,通过contextView.setTag绑定数据)有按钮的ListView
- HowTo: ListView, Adapter, getView and different list items’ layouts in one ListView
- ListView中使用自定义Adapter及时更新数据
- 使用自定义适配器实现ListView中的每一个list的不同显示风格
- ListView使用自定义Adapter
- HowTo: ListView, Adapter, getView and different list items’ layouts in one ListView