剑指offer--从尾到头打印链表
2017-03-22 20:57
344 查看
4000
题目:输入一个链表,从尾到头打印链表每个节点的值。
分析如下:
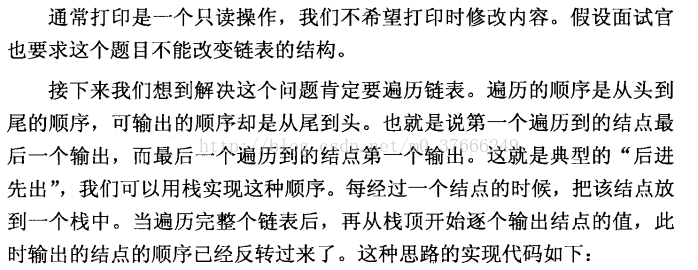
剑指offer上给出如下的代码以及测试用例:
// ReverseList.cpp : Defines the entry point for the console application.
//
#include "stdafx.h"
#include "..\Utilities\List.h"
ListNode* ReverseList(ListNode* pHead)
{
ListNode* pReversedHead = NULL;
ListNode* pNode = pHead;
ListNode* pPrev = NULL;
while(pNode != NULL)
{
ListNode* pNext = pNode->m_pNext;
if(pNext == NULL)
pReversedHead = pNode;
pNode->m_pNext = pPrev;
pPrev = pNode;
pNode = pNext;
}
return pReversedHead;
}
// ====================测试代码====================
ListNode* Test(ListNode* pHead)
{
printf("The original list is: \n");
PrintList(pHead);
ListNode* pReversedHead = ReverseList(pHead);
printf("The reversed list is: \n");
PrintList(pReversedHead);
return pReversedHead;
}
// 输入的链表有多个结点
void Test1()
{
ListNode* pNode1 = CreateListNode(1);
ListNode* pNode2 = CreateListNode(2);
ListNode* pNode3 = CreateListNode(3);
ListNode* pNode4 = CreateListNode(4);
ListNode* pNode5 = CreateListNode(5);
ConnectListNodes(pNode1, pNode2);
ConnectListNodes(pNode2, pNode3);
ConnectListNodes(pNode3, pNode4);
ConnectListNodes(pNode4, pNode5);
ListNode* pReversedHead = Test(pNode1);
DestroyList(pReversedHead);
}
// 输入的链表只有一个结点
void Test2()
{
ListNode* pNode1 = CreateListNode(1);
ListNode* pReversedHead = Test(pNode1);
DestroyList(pReversedHead);
}
// 输入空链表
void Test3()
{
Test(NULL);
}
int _tmain(int argc, _TCHAR* argv[])
{
Test1();
Test2();
Test3();
return 0;
}
书上还给出了递归的解法,本人对递归总是一知半解,所以直接把代码给大家看看哈:
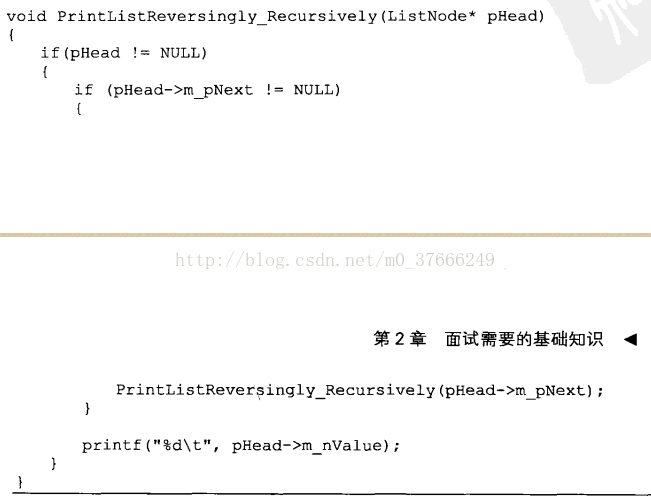
当链表非常长的时候,会导致函数调用的层级很深,可能会导致溢出等错误。
今天小编在刷牛客网上的题时候,写的代码也给大家参考参考,函数原型不一样:
/**
* struct ListNode {
* int val;
* struct ListNode *next;
* ListNode(int x) :
* val(x), next(NULL) {
* }
* };
*/
class Solution {
public:
vector<int> printListFromTailToHead(ListNode* head) {
stack<ListNode*> nodes;
vector<int> vec;
ListNode*pHead=head;
while(pHead!=NULL)
{
nodes.push(pHead);
pHead=pHead->next;
}
while(!nodes.empty())
{
pHead=nodes.top();
//printf("%d\t",pHead->val);
vec.push_back(pHead->val);
nodes.pop();
}
return vec;
}
};关于 C++中的vector、stack以及queue小编在下一篇文章中给大家更新。
题目:输入一个链表,从尾到头打印链表每个节点的值。
分析如下:
剑指offer上给出如下的代码以及测试用例:
// ReverseList.cpp : Defines the entry point for the console application.
//
#include "stdafx.h"
#include "..\Utilities\List.h"
ListNode* ReverseList(ListNode* pHead)
{
ListNode* pReversedHead = NULL;
ListNode* pNode = pHead;
ListNode* pPrev = NULL;
while(pNode != NULL)
{
ListNode* pNext = pNode->m_pNext;
if(pNext == NULL)
pReversedHead = pNode;
pNode->m_pNext = pPrev;
pPrev = pNode;
pNode = pNext;
}
return pReversedHead;
}
// ====================测试代码====================
ListNode* Test(ListNode* pHead)
{
printf("The original list is: \n");
PrintList(pHead);
ListNode* pReversedHead = ReverseList(pHead);
printf("The reversed list is: \n");
PrintList(pReversedHead);
return pReversedHead;
}
// 输入的链表有多个结点
void Test1()
{
ListNode* pNode1 = CreateListNode(1);
ListNode* pNode2 = CreateListNode(2);
ListNode* pNode3 = CreateListNode(3);
ListNode* pNode4 = CreateListNode(4);
ListNode* pNode5 = CreateListNode(5);
ConnectListNodes(pNode1, pNode2);
ConnectListNodes(pNode2, pNode3);
ConnectListNodes(pNode3, pNode4);
ConnectListNodes(pNode4, pNode5);
ListNode* pReversedHead = Test(pNode1);
DestroyList(pReversedHead);
}
// 输入的链表只有一个结点
void Test2()
{
ListNode* pNode1 = CreateListNode(1);
ListNode* pReversedHead = Test(pNode1);
DestroyList(pReversedHead);
}
// 输入空链表
void Test3()
{
Test(NULL);
}
int _tmain(int argc, _TCHAR* argv[])
{
Test1();
Test2();
Test3();
return 0;
}
书上还给出了递归的解法,本人对递归总是一知半解,所以直接把代码给大家看看哈:
当链表非常长的时候,会导致函数调用的层级很深,可能会导致溢出等错误。
今天小编在刷牛客网上的题时候,写的代码也给大家参考参考,函数原型不一样:
/**
* struct ListNode {
* int val;
* struct ListNode *next;
* ListNode(int x) :
* val(x), next(NULL) {
* }
* };
*/
class Solution {
public:
vector<int> printListFromTailToHead(ListNode* head) {
stack<ListNode*> nodes;
vector<int> vec;
ListNode*pHead=head;
while(pHead!=NULL)
{
nodes.push(pHead);
pHead=pHead->next;
}
while(!nodes.empty())
{
pHead=nodes.top();
//printf("%d\t",pHead->val);
vec.push_back(pHead->val);
nodes.pop();
}
return vec;
}
};关于 C++中的vector、stack以及queue小编在下一篇文章中给大家更新。
相关文章推荐
- 剑指offer 从尾到头打印链表
- 【剑指offer】面试题5:从尾到头打印链表 java
- 剑指offer - 面试题5:从尾到头打印链表
- 【剑指offer】从尾到头打印链表
- 【剑指offer】从尾到头打印链表
- 牛客网剑指offer-从尾到头打印链表
- 【剑指Offer学习】【面试题5 : 从尾到头打印链表】
- 剑指offer--从尾到头打印链表
- 剑指offer-从尾到头打印链表
- 《剑指 Offer》学习(3)—— 6_从尾到头打印链表
- 【练习笔记】剑指offer-面试题5 :从尾到头打印链表
- 【剑指offer-java版】3、从尾到头打印链表
- 剑指offer 5 -从尾到头打印链表
- 剑指Offer:从尾到头打印链表
- 剑指offer-面试题5-从尾到头打印链表
- 剑指Offer——从尾到头打印链表
- 剑指Offer----从尾到头打印链表
- 剑指offer-3.从尾到头打印链表
- 剑指offer:从尾到头打印链表
- 剑指offer: 从尾到头打印链表(链表)