Android进阶之路 - Circleimageview的使用姿势
2017-03-19 18:41
351 查看
大牛经常说站在巨人的肩膀上摸月亮,所以我并没有去看这个控件内部如何实现,而是5-10分钟简单的集成到自己的项目中进行使用
Circleimageview在我认为是非常实用的一个第三方控件,快速的实现了我们经常会使用到的圆形图标,如用户的头像或是一些item的图片展示
效果图(手机截图出来的,显示比较大,大家凑乎看吧,CreamView包名已经改为Circleimageview-对了,展示大小自己设置,我只是为了让大家看的清楚 - -~):
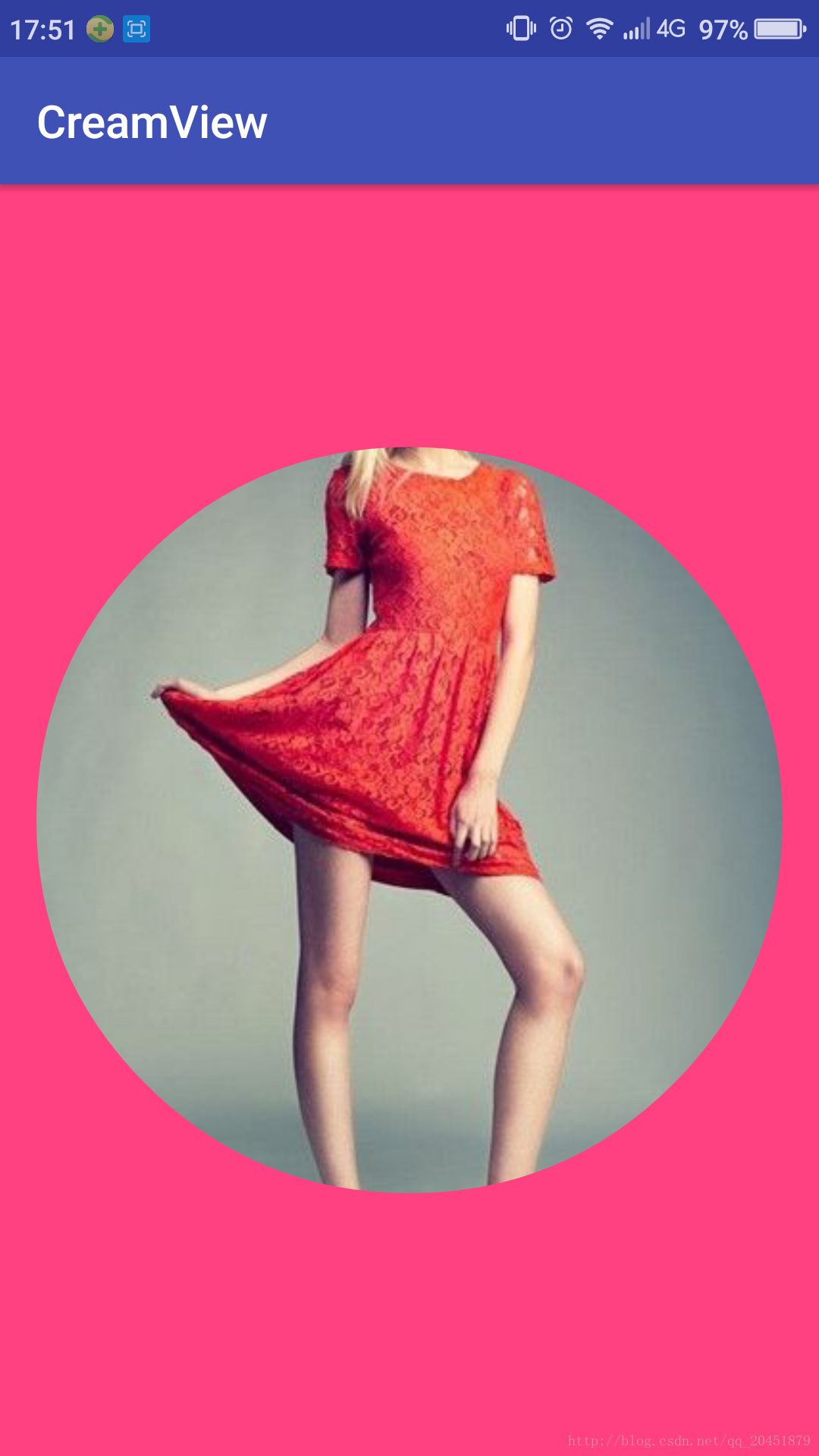
废话不多说,开始摸月亮吧~
前提-添加依赖:
valuse下新建attrs.xml-用于存储自定义属性
如没有实现圆形化,尝试换位下面的代码:
这里注意如果报错,是因为自定义属性的问题,可以合在一起!
如果想让你的实现更加完美-Xml引用命名控件,实现自定义属性使用:
简单的来说,我们使用的是他的自定义控件,所以我们要把这个类直接Copy过来,至于这个类的出处可以在github搜索circleimageview,之后下载一下就OK,当然也可以直接复制我下面的这个,但是记得改一下包名
CircleImageView(这个类是最重要的):
Mainactivity Xml(重要程度-次之-新手朋友要注意,在你需要圆形化的Xml中使用,并不是必须在Mainactivity的Xml中使用):
MainActivity:
Circleimageview在我认为是非常实用的一个第三方控件,快速的实现了我们经常会使用到的圆形图标,如用户的头像或是一些item的图片展示
效果图(手机截图出来的,显示比较大,大家凑乎看吧,CreamView包名已经改为Circleimageview-对了,展示大小自己设置,我只是为了让大家看的清楚 - -~):
废话不多说,开始摸月亮吧~
前提-添加依赖:
compile 'de.hdodenhof:circleimageview:2.1.0'
valuse下新建attrs.xml-用于存储自定义属性
<?xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name="CircleImageView"> <attr name="border_width" format="dimension" /> <attr name="border_color" format="color" /> <attr name="border_overlay" format="boolean" /> </declare-styleable> </resources>
如没有实现圆形化,尝试换位下面的代码:
<?xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name="CircleImageView"> //外边的宽度 <attr name="civ_border_width" format="dimension" /> //外边的颜色 <attr name="civ_border_color" format="color" /> <attr name="civ_border_overlay" format="boolean" /> <attr name="civ_fill_color" format="color" /> </declare-styleable> </resources>
这里注意如果报错,是因为自定义属性的问题,可以合在一起!
<?xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name="CircleImageView"> <attr name="civ_border_width" format="dimension" /> <attr name="civ_border_color" format="color" /> <attr name="civ_border_overlay" format="boolean" /> <attr name="civ_fill_color" format="color" /> <attr name="border_width" format="dimension" /> <attr name="border_color" format="color" /> <attr name="border_overlay" format="boolean" /> </declare-styleable> </resources>
如果想让你的实现更加完美-Xml引用命名控件,实现自定义属性使用:
xmlns:app="http://schemas.android.com/apk/res-auto"
简单的来说,我们使用的是他的自定义控件,所以我们要把这个类直接Copy过来,至于这个类的出处可以在github搜索circleimageview,之后下载一下就OK,当然也可以直接复制我下面的这个,但是记得改一下包名
CircleImageView(这个类是最重要的):
/* * Copyright 2014 - 2017 Henning Dodenhof * * Licensed under the Apache License, Version 2.0 (the "License"); * you may not use this file except in compliance with the License. * You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software * distributed under the License is distributed on an "AS IS" BASIS, * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. * See the License for the specific language governing permissions and * limitations under the License. */ package com.example.circleimageview; import android.content.Context; import android.content 4000 .res.TypedArray; import android.graphics.Bitmap; import android.graphics.BitmapShader; import android.graphics.Canvas; import android.graphics.Color; import android.graphics.ColorFilter; import android.graphics.Matrix; import android.graphics.Paint; import android.graphics.RectF; import android.graphics.Shader; import android.graphics.drawable.BitmapDrawable; import android.graphics.drawable.ColorDrawable; import android.graphics.drawable.Drawable; import android.net.Uri; import android.support.annotation.ColorInt; import android.support.annotation.ColorRes; import android.support.annotation.DrawableRes; import android.util.AttributeSet; import android.widget.ImageView; public class CircleImageView extends ImageView { private static final ScaleType SCALE_TYPE = ScaleType.CENTER_CROP; private static final Bitmap.Config BITMAP_CONFIG = Bitmap.Config.ARGB_8888; private static final int COLORDRAWABLE_DIMENSION = 2; private static final int DEFAULT_BORDER_WIDTH = 0; private static final int DEFAULT_BORDER_COLOR = Color.BLACK; private static final int DEFAULT_FILL_COLOR = Color.TRANSPARENT; private static final boolean DEFAULT_BORDER_OVERLAY = false; private final RectF mDrawableRect = new RectF(); private final RectF mBorderRect = new RectF(); private final Matrix mShaderMatrix = new Matrix(); private final Paint mBitmapPaint = new Paint(); private final Paint mBorderPaint = new Paint(); private final Paint mFillPaint = new Paint(); private int mBorderColor = DEFAULT_BORDER_COLOR; private int mBorderWidth = DEFAULT_BORDER_WIDTH; private int mFillColor = DEFAULT_FILL_COLOR; private Bitmap mBitmap; private BitmapShader mBitmapShader; private int mBitmapWidth; private int mBitmapHeight; private float mDrawableRadius; private float mBorderRadius; private ColorFilter mColorFilter; private boolean mReady; private boolean mSetupPending; private boolean mBorderOverlay; private boolean mDisableCircularTransformation; public CircleImageView(Context context) { super(context); init(); } public CircleImageView(Context context, AttributeSet attrs) { this(context, attrs, 0); } public CircleImageView(Context context, AttributeSet attrs, int defStyle) { super(context, attrs, defStyle); TypedArray a = context.obtainStyledAttributes(attrs, R.styleable.CircleImageView, defStyle, 0); mBorderWidth = a.getDimensionPixelSize(R.styleable.CircleImageView_civ_border_width, DEFAULT_BORDER_WIDTH); mBorderColor = a.getColor(R.styleable.CircleImageView_civ_border_color, DEFAULT_BORDER_COLOR); mBorderOverlay = a.getBoolean(R.styleable.CircleImageView_civ_border_overlay, DEFAULT_BORDER_OVERLAY); mFillColor = a.getColor(R.styleable.CircleImageView_civ_fill_color, DEFAULT_FILL_COLOR); a.recycle(); init(); } private void init() { super.setScaleType(SCALE_TYPE); mReady = true; if (mSetupPending) { setup(); mSetupPending = false; } } @Override public ScaleType getScaleType() { return SCALE_TYPE; } @Override public void setScaleType(ScaleType scaleType) { if (scaleType != SCALE_TYPE) { throw new IllegalArgumentException(String.format("ScaleType %s not supported.", scaleType)); } } @Override public void setAdjustViewBounds(boolean adjustViewBounds) { if (adjustViewBounds) { throw new IllegalArgumentException("adjustViewBounds not supported."); } } @Override protected void onDraw(Canvas canvas) { if (mDisableCircularTransformation) { super.onDraw(canvas); return; } if (mBitmap == null) { return; } if (mFillColor != Color.TRANSPARENT) { canvas.drawCircle(mDrawableRect.centerX(), mDrawableRect.centerY(), mDrawableRadius, mFillPaint); } canvas.drawCircle(mDrawableRect.centerX(), mDrawableRect.centerY(), mDrawableRadius, mBitmapPaint); if (mBorderWidth > 0) { canvas.drawCircle(mBorderRect.centerX(), mBorderRect.centerY(), mBorderRadius, mBorderPaint); } } @Override protected void onSizeChanged(int w, int h, int oldw, int oldh) { super.onSizeChanged(w, h, oldw, oldh); setup(); } @Override public void setPadding(int left, int top, int right, int bottom) { super.setPadding(left, top, right, bottom); setup(); } @Override public void setPaddingRelative(int start, int top, int end, int bottom) { super.setPaddingRelative(start, top, end, bottom); setup(); } public int getBorderColor() { return mBorderColor; } public void setBorderColor(@ColorInt int borderColor) { if (borderColor == mBorderColor) { return; } mBorderColor = borderColor; mBorderPaint.setColor(mBorderColor); invalidate(); } /** * @deprecated Use {@link #setBorderColor(int)} instead */ @Deprecated public void setBorderColorResource(@ColorRes int borderColorRes) { setBorderColor(getContext().getResources().getColor(borderColorRes)); } /** * Return the color drawn behind the circle-shaped drawable. * * @return The color drawn behind the drawable * * @deprecated Fill color support is going to be removed in the future */ @Deprecated public int getFillColor() { return mFillColor; } /** * Set a color to be drawn behind the circle-shaped drawable. Note that * this has no effect if the drawable is opaque or no drawable is set. * * @param fillColor The color to be drawn behind the drawable * * @deprecated Fill color support is going to be removed in the future */ @Deprecated public void setFillColor(@ColorInt int fillColor) { if (fillColor == mFillColor) { return; } mFillColor = fillColor; mFillPaint.setColor(fillColor); invalidate(); } /** * Set a color to be drawn behind the circle-shaped drawable. Note that * this has no effect if the drawable is opaque or no drawable is set. * * @param fillColorRes The color resource to be resolved to a color and * drawn behind the drawable * * @deprecated Fill color support is going to be removed in the future */ @Deprecated public void setFillColorResource(@ColorRes int fillColorRes) { setFillColor(getContext().getResources().getColor(fillColorRes)); } public int getBorderWidth() { return mBorderWidth; } public void setBorderWidth(int borderWidth) { if (borderWidth == mBorderWidth) { return; } mBorderWidth = borderWidth; setup(); } public boolean isBorderOverlay() { return mBorderOverlay; } public void setBorderOverlay(boolean borderOverlay) { if (borderOverlay == mBorderOverlay) { return; } mBorderOverlay = borderOverlay; setup(); } public boolean isDisableCircularTransformation() { return mDisableCircularTransformation; } public void setDisableCircularTransformation(boolean disableCircularTransformation) { if (mDisableCircularTransformation == disableCircularTransformation) { return; } mDisableCircularTransformation = disableCircularTransformation; initializeBitmap(); } @Override public void setImageBitm ea44 ap(Bitmap bm) { super.setImageBitmap(bm); initializeBitmap(); } @Override public void setImageDrawable(Drawable drawable) { super.setImageDrawable(drawable); initializeBitmap(); } @Override public void setImageResource(@DrawableRes int resId) { super.setImageResource(resId); initializeBitmap(); } @Override public void setImageURI(Uri uri) { super.setImageURI(uri); initializeBitmap(); } @Override public void setColorFilter(ColorFilter cf) { if (cf == mColorFilter) { return; } mColorFilter = cf; applyColorFilter(); invalidate(); } @Override public ColorFilter getColorFilter() { return mColorFilter; } private void applyColorFilter() { if (mBitmapPaint != null) { mBitmapPaint.setColorFilter(mColorFilter); } } private Bitmap getBitmapFromDrawable(Drawable drawable) { if (drawable == null) { return null; } if (drawable instanceof BitmapDrawable) { return ((BitmapDrawable) drawable).getBitmap(); } try { Bitmap bitmap; if (drawable instanceof ColorDrawable) { bitmap = Bitmap.createBitmap(COLORDRAWABLE_DIMENSION, COLORDRAWABLE_DIMENSION, BITMAP_CONFIG); } else { bitmap = Bitmap.createBitmap(drawable.getIntrinsicWidth(), drawable.getIntrinsicHeight(), BITMAP_CONFIG); } Canvas canvas = new Canvas(bitmap); drawable.setBounds(0, 0, canvas.getWidth(), canvas.getHeight()); drawable.draw(canvas); return bitmap; } catch (Exception e) { e.printStackTrace(); return null; } } private void initializeBitmap() { if (mDisableCircularTransformation) { mBitmap = null; } else { mBitmap = getBitmapFromDrawable(getDrawable()); } setup(); } private void setup() { if (!mReady) { mSetupPending = true; return; } if (getWidth() == 0 && getHeight() == 0) { return; } if (mBitmap == null) { invalidate(); return; } mBitmapShader = new BitmapShader(mBitmap, Shader.TileMode.CLAMP, Shader.TileMode.CLAMP); mBitmapPaint.setAntiAlias(true); mBitmapPaint.setShader(mBitmapShader); mBorderPaint.setStyle(Paint.Style.STROKE); mBorderPaint.setAntiAlias(true); mBorderPaint.setColor(mBorderColor); mBorderPaint.setStrokeWidth(mBorderWidth); mFillPaint.setStyle(Paint.Style.FILL); mFillPaint.setAntiAlias(true); mFillPaint.setColor(mFillColor); mBitmapHeight = mBitmap.getHeight(); mBitmapWidth = mBitmap.getWidth(); mBorderRect.set(calculateBounds()); mBorderRadius = Math.min((mBorderRect.height() - mBorderWidth) / 2.0f, (mBorderRect.width() - mBorderWidth) / 2.0f); mDrawableRect.set(mBorderRect); if (!mBorderOverlay && mBorderWidth > 0) { mDrawableRect.inset(mBorderWidth - 1.0f, mBorderWidth - 1.0f); } mDrawableRadius = Math.min(mDrawableRect.height() / 2.0f, mDrawableRect.width() / 2.0f); applyColorFilter(); updateShaderMatrix(); invalidate(); } private RectF calculateBounds() { int availableWidth = getWidth() - getPaddingLeft() - getPaddingRight(); int availableHeight = getHeight() - getPaddingTop() - getPaddingBottom(); int sideLength = Math.min(availableWidth, availableHeight); float left = getPaddingLeft() + (availableWidth - sideLength) / 2f; float top = getPaddingTop() + (availableHeight - sideLength) / 2f; return new RectF(left, top, left + sideLength, top + sideLength); } private void updateShaderMatrix() { float scale; float dx = 0; float dy = 0; mShaderMatrix.set(null); if (mBitmapWidth * mDrawableRect.height() > mDrawableRect.width() * mBitmapHeight) { scale = mDrawableRect.height() / (float) mBitmapHeight; dx = (mDrawableRect.width() - mBitmapWidth * scale) * 0.5f; } else { scale = mDrawableRect.width() / (float) mBitmapWidth; dy = (mDrawableRect.height() - mBitmapHeight * scale) * 0.5f; } mShaderMatrix.setScale(scale, scale); mShaderMatrix.postTranslate((int) (dx + 0.5f) + mDrawableRect.left, (int) (dy + 0.5f) + mDrawableRect.top); mBitmapShader.setLocalMatrix(mShaderMatrix); } }
Mainactivity Xml(重要程度-次之-新手朋友要注意,在你需要圆形化的Xml中使用,并不是必须在Mainactivity的Xml中使用):
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:background="@color/colorAccent" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.creamview.MainActivity"> <com.example.circleimageview.CircleImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/woman" android:layout_centerInParent="true" app:border_color="#f000" app:border_width="2dp" /> </RelativeLayout>
MainActivity:
package com.example.circleimageview; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } }
相关文章推荐
- 【Android开源项目分析】自定义圆形头像CircleImageView的使用和源码分析
- Android CircleImageView自定义圆环头像控件的使用
- android开源系列:CircleImageView自定义圆形控件的使用
- 【Android开源项目分析】自定义圆形头像CircleImageView的使用和源码分析
- Android中自定义圆形图片的CircleImageView和RoundedImageView基本使用效果
- Android ImageView 正确使用姿势
- 【Android开源项目分析】自定义圆形头像CircleImageView的使用和源码分析
- 【腾讯Bugly干货分享】Android ImageView 正确使用姿势
- Android ImageView 正确使用姿势
- android开源系列:CircleImageView自定义圆形控件的使用
- android开源系列:CircleImageView自定义圆形控件的使用
- Android中使用CircleImageView和Cardview制作圆形头像的方法
- [转]Android ImageView 正确使用姿势
- Android开发(二)——自定义圆形控件的使用CircleImageView
- android开源系列:CircleImageView自定义圆形控件的使用
- 【Android开源项目分析】自定义圆形头像CircleImageView的使用和源码分析
- Android开源组件---CircleImageView的使用
- Android ImageView 正确使用姿势
- Android ImageView 正确使用姿势
- 【腾讯Bugly干货分享】Android ImageView 正确使用姿势