《unix/linux编程实践教程》学习笔记:第七章 事件驱动编程:编写一个视频游戏
2017-03-12 18:09
555 查看
本章以一个弹珠游戏为例,学习了
屏幕编程的curses库(Ubuntu中需要另外安装软件)
时间编程,使用计时器
信号处理
临界区
注意编译的命令为
游戏代码
运行截图
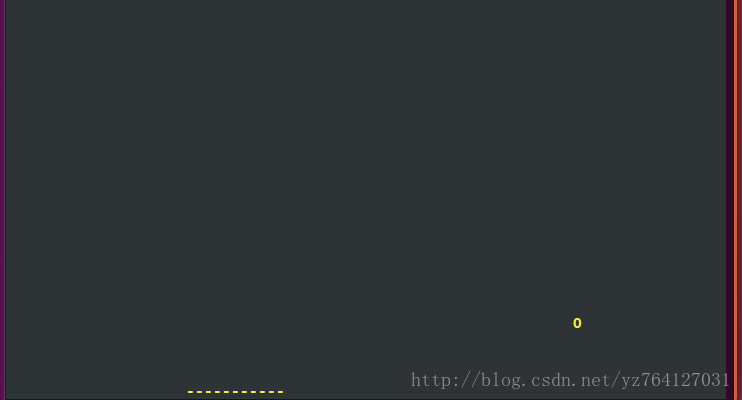
函数调用关系:
main——》control——》init——》moveball
屏幕编程的curses库(Ubuntu中需要另外安装软件)
时间编程,使用计时器
信号处理
临界区
注意编译的命令为
gcc filename.c - o gamename -l curses
游戏代码
运行截图
函数调用关系:
main——》control——》init——》moveball
#include <curses.h> #include <sys/time.h> #include <signal.h> #define RIGHT COLS-1 /*屏幕最右*/ #define BOTTOM LINES-1 /*屏幕底部*/ #define BOARD_LENGTH 10 /*挡板长度*/ #define LEFT 0 #define TOP 0 char BALL= 'O'; /*小球图像*/ char BLANK= ' '; /*清除小球*/ int left_board; /*挡板最左*/ int right_board; /*挡板最右*/ int is_lose=0; int hdir; /*横向速度*/ int vdir; /*纵向速度*/ int pos_X; /*小球横坐标*/ int pos_Y; /*小球纵坐标*/ int delay=100; void moveBall(); void init(); void control(); int main() { initscr(); /*初始化curses库和tty*/ crmode(); noecho(); move(6,28); /*打印主菜单*/ attron(A_BOLD); addstr("Welcome to the BallGame!"); move(8,20); attroff(A_BOLD); addstr("Help:"); move(9,23); addstr("'N':Start a new game."); move(10,23); addstr("'Q':Quit game."); move(11,23); addstr("'KEY_LEFT' :Control baffle left shift."); move(12,23); addstr("'KEY_RIGHT':Control baffle right shift."); move(13,23); addstr("'KEY_UP' :Control of the ball speed."); move(14,23); addstr("'KEY_DOWN' :Control of the ball reducer."); int flag=1; char choice; move(16,24); addstr("Please choose your choice!(n/q):"); refresh(); choice=getch(); /*阻塞等待输入*/ while(flag){ if(choice=='q'||choice=='Q'||choice=='n'||choice=='N') flag=0; else choice=getch(); } if(choice=='n'||choice=='N'){ /*输入N游戏开始*/ clear(); move(10,25); addstr("BallGame will start! Are you read?"); refresh(); sleep(3); control(); } else if(choice=='q'||choice=='Q'){ /*输入Q游戏退出*/ clear(); move(10,25); addstr("You quit the game successfully!"); refresh(); sleep(3); endwin(); } endwin(); /*关闭curses库并重置tty*/ return 0; } void init(){ int i,j; clear(); if(start_color()==OK){ attron(A_BOLD); init_pair(1,COLOR_YELLOW,COLOR_BLACK); attron(COLOR_PAIR(1)); } //小球位置 pos_X =22; pos_Y = BOTTOM-1; //小球速度与方向¯ hdir=1; vdir=-1; //画挡板 left_board=20; right_board=left_board+BOARD_LENGTH; for(i=left_board;i<=right_board;i++){ move(BOTTOM,i); addch('-'); } //定时刷新 signal(SIGALRM,moveBall); set_ticker(delay); keypad(stdscr,TRUE); attroff(A_BLINK); is_lose=0; move(pos_Y,pos_X); addch(BALL); move(LINES-1, COLS-1); refresh(); usleep(100000); move(LINES-1,COLS-1); refresh(); } void moveBall(){ if(is_lose) return; signal(SIGALRM,moveBall); move(pos_Y,pos_X); addch(BLANK); pos_X += hdir; pos_Y += vdir; //边界控制 if(pos_X >= RIGHT) { hdir = -1; beep(); } if(pos_X <= LEFT) { hdir = 1; beep(); } if(pos_Y <= TOP) { vdir = 1; beep(); } //碰撞判断 if(pos_Y >= BOTTOM-1){ if(pos_X>=left_board&&pos_X<=right_board) vdir=-1; else{ is_lose=1; move(pos_Y,pos_X); addch(BALL); move(LINES-1, COLS-1); refresh(); usleep(delay*1000); move(pos_Y,pos_X); addch(BLANK); pos_X += hdir; pos_Y += vdir; move(pos_Y,pos_X); addch(BALL); move(LINES-1, COLS-1); refresh(); } } move(pos_Y,pos_X); addch(BALL); move(LINES-1, COLS-1); refresh(); } void control(){ init(); //初始化屏幕 int cmd; while (1) { if(!is_lose){ cmd=getch(); if(cmd=='q'||cmd=='Q'||cmd==27) break; //挡板左移 if(cmd==KEY_LEFT){ if(left_board>0){ move(BOTTOM,right_board); addch(' '); right_board--; left_board--; move(BOTTOM,left_board); addch('-'); move(BOTTOM,RIGHT); refresh(); } } //挡板右移 else if(cmd==KEY_RIGHT){ if(right_board<RIGHT){ move(BOTTOM,left_board); addch(' '); right_board++; left_board++; move(BOTTOM,right_board); addch('-'); move(BOTTOM,RIGHT); refresh(); } } //小球速度加快 else if(cmd==KEY_UP){ delay/=2; set_ticker(delay); } //小球速度减慢 else if(cmd==KEY_DOWN){ delay*=2; set_ticker(delay); } } else{ //游戏失败页面 int flag=1; char choice; move(8,15); addstr("Game Over!try again?(y/n):"); refresh(); choice=getch(); while(flag){ if(choice=='y'||choice=='Y'||choice=='n'||choice=='N') flag=0; else choice=getch(); } if(choice=='y'||choice=='Y'){ /*重新开始*/ delay=100; init(); continue; } else if(choice=='n'||choice=='N'){ /*结束游戏*/ break; } } } } //设置定时器 int set_ticker(int n_msecs){ struct itimerval new_timeset; long n_sec,n_usecs; n_sec=n_msecs/1000; n_usecs=(n_msecs%1000)*1000L; new_timeset.it_interval.tv_sec=n_sec; new_timeset.it_interval.tv_usec=n_usecs; new_timeset.it_value.tv_sec=n_sec; new_timeset.it_value.tv_usec=n_usecs; return setitimer(ITIMER_REAL,&new_timeset,NULL); }
相关文章推荐
- Understanding Unix/Linux Programming 笔记:chapter 7:事件驱动编程:编写一个视频游戏
- Understanding Unix/Linux Programming-事件驱动编程:编写一个视频游戏
- 第7章 事件驱动编程:编写一个视频游戏
- 三. 学习Unix/Linux编程之事件驱动编程:编写一个视频游戏
- 采用事件驱动编程以及GUI组件开发一个贷款计算器
- 韩顺平 javascript教学视频_学习笔记27_dom对象(window对象3.history.location.navigator.screen.event)_js事件驱动编程
- 一个最简单的事件驱动的IO libevent编程例子
- 【视频】半小时内编写一个贪吃蛇游戏
- 【编程游戏】编写一个会划拳的机器人参加擂台赛,规则内详。路过有分。
- 通过一个游戏编程熟悉事件处理
- 一个使用面向对象方式编写的简单游戏框架
- 这几天我写了一个类库,可以提供office编程时使用Word, Excel,PowerPoint等的保存后事件DocumentAfterSave
- [导入]视频游戏开发系列课程(2):基础编程概念及C#简介
- 用DS播放一个视频时,如何通过编程知道GRAPH加载的FILTER的组件所在的路径??
- 事件驱动的JScript面对象编程
- 事件驱动的JScript面对象编程
- 最近正在计划编写一个麻将游戏
- C#编写的一个俄罗斯方块游戏
- ASP.net组件编程中的两种事件编写方法
- 介绍一个PHP5事件驱动的好工程