spring cloud netflix eureka ribbon 示例
2017-03-03 18:28
721 查看
示例分三部分,
eureka-server 服务注册中心
eureka-provider 服务提供者
eureka-consumer 服务调用者
eureka-server 服务注册中心
简单的 boot
配置文件
eureka-provider 服务提供者
服务功能
配置
eureka-consumer 服务调用者
boot 和 调用服务
配置文件
效果图
注册中心,看到注册的应用
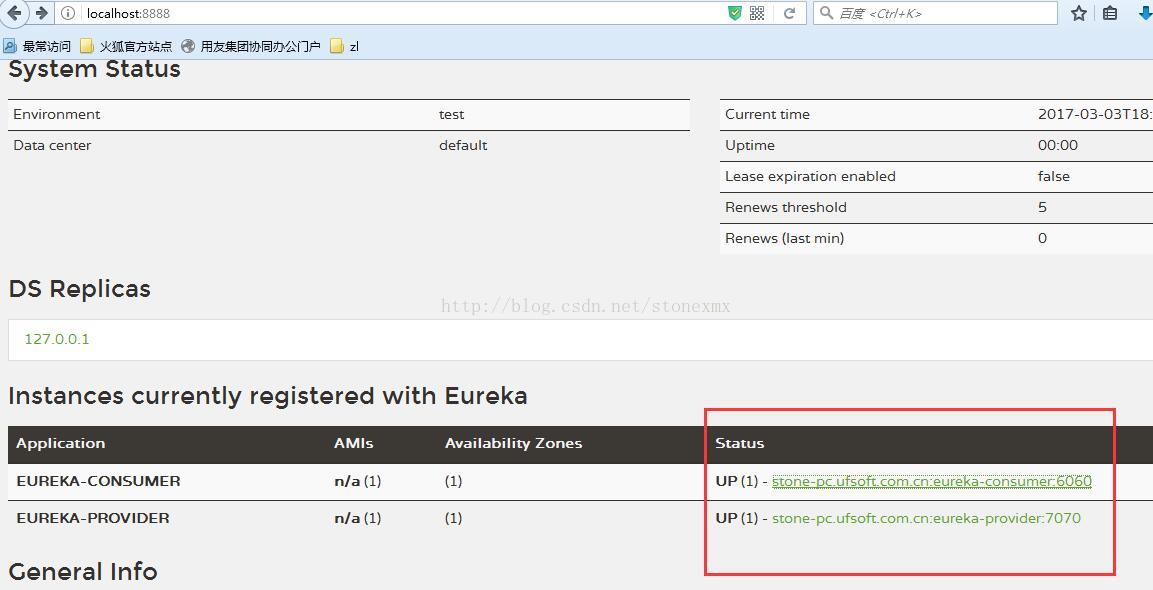
查看provider 自身的服务

使用consumer 调用 provider的服务

示例下载地址:
http://download.csdn.net/detail/stonexmx/9769755
eureka-server 服务注册中心
eureka-provider 服务提供者
eureka-consumer 服务调用者
eureka-server 服务注册中心
简单的 boot
package com.yonyou; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer; @EnableEurekaServer //开启eureka服务 @SpringBootApplication //springBoot注解,spring在springBoot基础之上来构建项目 public class EurekaServiceApplication { //spirng boot的标准入口 public static void main(String[] args) { SpringApplication.run(EurekaServiceApplication.class, args); } }
配置文件
spring.application.name=eureka-server server.port=8888 eureka.client.register-with-eureka=false eureka.client.fetch-registry=false logging.level.com.netflix.eureka=OFF logging.level.com.netflix.discovery=OFF eureka.client.serviceUrl.defaultZone=http://127.0.0.1:${server.port}/eureka/
eureka-provider 服务提供者
package com.yonyou; import java.util.List; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.client.ServiceInstance; import org.springframework.cloud.client.discovery.DiscoveryClient; import org.springframework.cloud.client.discovery.EnableDiscoveryClient; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import org.springframework.web.client.RestTemplate; /** * @author Administrator * */ @EnableDiscoveryClient //通过该注解,实现服务发现,注册 @SpringBootApplication public class EurekaClientApplication { public static void main(String[] args) { SpringApplication.run(EurekaClientApplication.class, args); } } @RestController class ServiceInstanceRestController { @Autowired private DiscoveryClient discoveryClient; @RequestMapping("/service-instances/{applicationName}") public List<ServiceInstance> serviceInstancesByApplicationName( @PathVariable String applicationName) { return this.discoveryClient.getInstances(applicationName); } @RequestMapping("/info") public String sayhello() { return "client-student"; } }
服务功能
package com.yonyou.student.web; import java.util.ArrayList; import java.util.List; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.client.ServiceInstance; import org.springframework.cloud.client.discovery.DiscoveryClient; import org.springframework.cloud.client.discovery.EnableDiscoveryClient; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import org.springframework.web.client.RestTemplate; import com.yonyou.student.Student; @RestController @RequestMapping("/student") class StudentController { @RequestMapping("list") public List<Student> list() { List<Student> list = new ArrayList<Student>(); list.add(new Student(111,"yaoming","156787787823","china shanghai")); list.add(new Student(222,"chenglong","13898687682","china hongkong")); list.add(new Student(333,"lilianjie","187923420023","china beijing")); return list; } }
配置
spring.application.name=eureka-provider server.port=7070 logging.level.com.netflix.eureka=OFF logging.level.com.netflix.discovery=OFF eureka.client.serviceUrl.defaultZone=http://127.0.0.1:8888/eureka/
eureka-consumer 服务调用者
boot 和 调用服务
package com.yonyou; import java.net.URI; import java.util.List; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Value; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.client.ServiceInstance; import org.springframework.cloud.client.discovery.DiscoveryClient; import org.springframework.cloud.client.discovery.EnableDiscoveryClient; import org.springframework.cloud.client.loadbalancer.LoadBalanced; import org.springframework.cloud.client.loadbalancer.LoadBalancerClient; import org.springframework.context.annotation.Bean; import org.springframework.http.client.SimpleClientHttpRequestFactory; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import org.springframework.web.client.RestTemplate; /** * @author Administrator * */ @EnableDiscoveryClient //通过该注解,实现服务发现,注册 @SpringBootApplication public class EurekaClientApplication { /** * LoadBalanced 注解表明restTemplate使用LoadBalancerClient执行请求 */ @Bean @LoadBalanced public RestTemplate restTemplate() { RestTemplate template = new RestTemplate(); SimpleClientHttpRequestFactory factory = (SimpleClientHttpRequestFactory) template.getRequestFactory(); factory.setConnectTimeout(3000); factory.setReadTimeout(3000); return template; } public static void main(String[] args) { SpringApplication.run(EurekaClientApplication.class, args); } } @RestController class ServiceInstanceRestController { @Autowired @LoadBalanced RestTemplate restTemplate; @Autowired private DiscoveryClient discoveryClient; @RequestMapping("/service-instances/{applicationName}") public List<ServiceInstance> serviceInstancesByApplicationName( @PathVariable String applicationName) { return this.discoveryClient.getInstances(applicationName); } @RequestMapping("/info") public String sayhello() { return "client-teacher"; } // Restful服务对应的url地址 @Value("${rest.student.list}") private String restStudentList; @RequestMapping("/students") public String test() { // ServiceInstance instance = loadBalancer.choose("eureka-client-student"); // URI uri = instance.getUri(); List list = restTemplate.getForObject(restStudentList, List.class); return list.toString(); } }
配置文件
spring.application.name=eureka-consumer server.port=6060 logging.level.com.netflix.eureka=OFF logging.level.com.netflix.discovery=OFF eureka.client.serviceUrl.defaultZone=http://127.0.0.1:8888/eureka/ rest.student=http://eureka-provider:7070 rest.student.list=${rest.student}/student/list
效果图
注册中心,看到注册的应用
查看provider 自身的服务
使用consumer 调用 provider的服务
示例下载地址:
http://download.csdn.net/detail/stonexmx/9769755
相关文章推荐
- Spring Cloud Netflix的3大组件应用 Eureka&Ribbon&Hystrix
- Spring Cloud实战(三)-Spring Cloud Netflix Ribbon
- 【SpringCloud】Netflix源码解析之Ribbon:负载均衡策略的定义和实现
- Spring Cloud Netflix Eureka: 多网卡环境下Eureka服务注册IP选择问题
- 《Spring Cloud Netflix》-- 服务注册和服务发现-Eureka的服务认证和集群
- Spring Cloud Netflix负载均衡组件Ribbon介绍
- Spring Cloud Netflix 教程(Feign+Ribbon+Hystrix)
- 微服务框架Spring Cloud介绍 Part4: 使用Eureka, Ribbon, Feign实现REST服务客户端
- Spring Cloud Netflix Eureka - 揭秘手册
- Spring-cloud & Netflix 源码解析:Eureka 服务注册发现接口 ****
- 《Spring Cloud Netflix》--服务注册和服务发现-Eureka的深入了解
- Spring Cloud Netflix之Eureka上篇
- Spring Cloud Netflix之Eureka Doc Translation
- spring-cloud-netflix eureka服务添加基本用户验证
- Spring Cloud Netflix Eureka client源码分析
- Spring Cloud Netflix之Eureka 相关概念
- 《Spring Cloud Netflix》 -- 服务注册和服务发现-Eureka 的使用
- 服务注册和服务发现-Eureka的服务认证和集群--Spring Cloud Netflix
- Spring Cloud Netflix之Eureka Client Configuration
- 四、Spring Cloud - Netflix(Eureka注册中心集群构建代码层面)