Python Numpy
2017-02-19 14:44
148 查看
一些Numpy常用的概念和方法
1.数组
2.数组切片
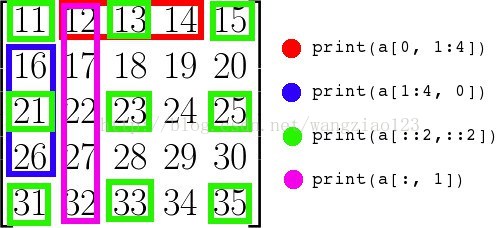
这里具体说一下reshape
numpy.reshape(a, newshape, order='C')#a就是要被reshape的数组,newshape是新数组形状int
or tuple of ints.
例子:
1.数组
# 1D Array a = np.array([0, 1, 2, 3, 4]) b = np.array((0, 1, 2, 3, 4)) c = np.arange(5) d = np.linspace(0, 2*np.pi, 5) #在0到2*np.pi之间平均生成5个数 print(a) # >>>[0 1 2 3 4] print(b) # >>>[0 1 2 3 4] print(c) # >>>[0 1 2 3 4] print(d) # >>>[ 0. 1.57079633 3.14159265 4.71238898 6.28318531] print(a[3]) # >>>3下面是二维数组
# MD Array, a = np.array([[11, 12, 13, 14, 15], [16, 17, 18, 19, 20], [21, 22, 23, 24, 25], [26, 27, 28 ,29, 30], [31, 32, 33, 34, 35]]) print(a[2,4]) # >>>25
2.数组切片
print(a[0, 1:4]) # >>>[12 13 14] print(a[1:4, 0]) # >>>[16 21 26] print(a[::2,::2]) # >>>[[11 13 15] # [21 23 25] # [31 33 35]] print(a[:, 1]) # >>>[12 17 22 27 32]这幅图很好的解释了切片操作
3.数组操作
a = np.arange(25) a = a.reshape((5, 5)) b = np.array([10, 62, 1, 14, 2, 56, 79, 2, 1, 45, 4, 92, 5, 55, 63, 43, 35, 6, 53, 24, 56, 3, 56, 44, 78]) b = b.reshape((5,5)) print(a + b) #两个数组对应元素进行+-*/ print(a - b) print(a * b) print(a / b) print(a ** 2) print(a < b) print(a > b)#返回布尔值 print(a.dot(b))#点积
a = np.arange(10) print(a.sum()) print(a.min()) print(a.max())
a = np.arange(0, 100, 10) b = a[:5] c = a[a >= 50] print(b) # >>>[ 0 10 20 30 40] print(c) # >>>[50 60 70 80 90]
这里具体说一下reshape
numpy.reshape(a, newshape, order='C')#a就是要被reshape的数组,newshape是新数组形状int
or tuple of ints.
例子:
>>> a = np.arange(6).reshape((3, 2)) >>> a array([[0, 1], [2, 3], [4, 5]])
>>> a = np.array([[1,2,3], [4,5,6]]) >>> np.reshape(a, 6) array([1, 2, 3, 4, 5, 6]) >>> np.reshape(a, 6, order='F') array([1, 4, 2, 5, 3, 6])
>>> np.reshape(a, (3,-1)) # the unspecified value is inferred to be 2 array([[1, 2], [3, 4], [5, 6]])
相关文章推荐
- Python画图库 matplotlib, 数值计算库 numpy, 科学计算库 scipy 的安装
- Python之路——numpy各函数简介之生成数组函数(Array creation routines)
- Ubuntu-Python2.7安装 scipy,numpy,matplotlib
- python中numpy介绍
- windows下python安装Numpy和Scipy模块
- ubuntu 下 python numpy,scipy,matplotlib安装
- Python绘图和数值工具:matplotlib 和 numpy下载与使用
- ParaView - Mixing NumPy and VTK APIs with high efficient using Python Programmable filter
- Python库numpy中的svd分解和Matlab中的svd的一点区别
- 使用python和numpy,scipy做FIR带通滤波实验
- Python Numpy数组保存
- 为python安装numpy和scipy(federo)
- 【python】python path,macports,easy-install,numpy,scipy,ipython,matplotlib,集成工具
- yablog: calculate cosine with python numpy
- Python、VPython、NumPy、MatPlotLib简介
- Linux(Fedora)下python “no module named numpy” 解决方案
- 使用numpy很容易在python中将数据读入内存
- python numpy使用
- 比较一下numpy.math和Python标准库的math.sin的计算速度
- python学习--numpy的数组