MyBatis源码剖析 - MyBatis 插件之拦截器(Interceptor)实现原理
2017-02-18 12:18
726 查看
在上一篇文章:MyBatis 教程 - MyBatis插件(Plugins)开发 中已经介绍了如何去开发一个MyBatis 插件,本文将结合MyBatis 源码来揭秘MyBatis Plugins内部实现原理。
Mybatis3 插件采用责任链模式,通过动态代理组织多个拦截器(插件),通过这些拦截器可以改变Mybatis的默认行为(诸如SQL重写之类的)。
本文分析使用的MyBatis 源代码版本为3.4.1
先来看看上一篇文章中实现的插件,代码如下:
下面以Executor接口为例,org.apache.ibatis.executor.SimpleExecutor 在执行doUpdate、doQuery、doQueryCursor方法时会执行如下代码:
顺藤摸瓜,我们来看看org.apache.ibatis.session.Configuration 类,其代码如下:
我们重点关注这行代码:
上面代码功能是:对statementHandler 插入所有的Interceptor以便进行拦截,InterceptorChain里保存了所有的拦截器,它在Configuration 对象被构造出来的时候创建。
org.apache.ibatis.plugin.InterceptorChain 源代码如下:
org.apache.ibatis.plugin.Interceptor 类的代码如下:
org.apache.ibatis.plugin.Invocation 代码如下:
org.apache.ibatis.plugin.Plugin 源代码如下:
责任链模式在面向对象程式设计里是一种软件设计模式,它包含了一些命令对象和一系列的处理对象。每一个处理对象决定它能处理哪些命令对象,它也知道如何将它不能处理的命令对象传递给该链中的下一个处理对象。该模式还描述了往该处理链的末尾添加新的处理对象的方法。
23种设计模式中非常经典的一个设计模式。
Mybatis3 插件采用责任链模式,通过动态代理组织多个拦截器(插件),通过这些拦截器可以改变Mybatis的默认行为(诸如SQL重写之类的)。
本文分析使用的MyBatis 源代码版本为3.4.1
先来看看上一篇文章中实现的插件,代码如下:
package com.bytebeats.mybatis3.interceptor; import org.apache.ibatis.executor.statement.StatementHandler; import org.apache.ibatis.mapping.BoundSql; import org.apache.ibatis.plugin.*; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import java.sql.Connection; import java.util.Properties; /** * ${DESCRIPTION} * * @author Ricky Fung * @date 2017-02-17 11:52 */ @Intercepts({ @Signature(type = StatementHandler.class, method = "prepare", args = { Connection.class, Integer.class}) }) public class SQLStatsInterceptor implements Interceptor { private final Logger logger = LoggerFactory.getLogger(this.getClass()); @Override public Object intercept(Invocation invocation) throws Throwable { StatementHandler statementHandler = (StatementHandler) invocation.getTarget(); BoundSql boundSql = statementHandler.getBoundSql(); String sql = boundSql.getSql(); logger.info("mybatis intercept sql:{}", sql); return invocation.proceed(); } @Override public Object plugin(Object target) { return Plugin.wrap(target, this); } @Override public void setProperties(Properties properties) { String dialect = properties.getProperty("dialect"); logger.info("mybatis intercept dialect:{}", dialect); } }
实现原理分析
Mybatis支持对Executor、StatementHandler、PameterHandler和ResultSetHandler 接口进行拦截,也就是说会对这4种对象进行代理。下面以Executor接口为例,org.apache.ibatis.executor.SimpleExecutor 在执行doUpdate、doQuery、doQueryCursor方法时会执行如下代码:
Configuration configuration = ms.getConfiguration(); StatementHandler handler = configuration.newStatementHandler(this, ms, parameter, RowBounds.DEFAULT, null, null);
顺藤摸瓜,我们来看看org.apache.ibatis.session.Configuration 类,其代码如下:
public class Configuration { protected final InterceptorChain interceptorChain = new InterceptorChain(); /**对ParameterHandler 进行拦截**/ public ParameterHandler newParameterHandler(MappedStatement mappedStatement, Object parameterObject, BoundSql boundSql) { ParameterHandler parameterHandler = mappedStatement.getLang().createParameterHandler(mappedStatement, parameterObject, boundSql); parameterHandler = (ParameterHandler) interceptorChain.pluginAll(parameterHandler); return parameterHandler; } /**对ResultSetHandler 进行拦截**/ public ResultSetHandler newResultSetHandler(Executor executor, MappedStatement mappedStatement, RowBounds rowBounds, ParameterHandler parameterHandler, ResultHandler resultHandler, BoundSql boundSql) { ResultSetHandler resultSetHandler = new DefaultResultSetHandler(executor, mappedStatement, parameterHandler, resultHandler, boundSql, rowBounds); resultSetHandler = (ResultSetHandler) interceptorChain.pluginAll(resultSetHandler); return resultSetHandler; } /**对StatementHandler 进行拦截**/ public StatementHandler newStatementHandler(Executor executor, MappedStatement mappedStatement, Object parameterObject, RowBounds rowBounds, ResultHandler resultHandler, BoundSql boundSql) { StatementHandler statementHandler = new RoutingStatementHandler(executor, mappedStatement, parameterObject, rowBounds, resultHandler, boundSql); statementHandler = (StatementHandler) interceptorChain.pluginAll(statementHandler); return statementHandler; } /**对Executor 进行拦截**/ public Executor newExecutor(Transaction transaction) { return newExecutor(transaction, defaultExecutorType); } /**对Executor 进行拦截**/ public Executor newExecutor(Transaction transaction, ExecutorType executorType) { executorType = executorType == null ? defaultExecutorType : executorType; executorType = executorType == null ? ExecutorType.SIMPLE : executorType; Executor executor; if (ExecutorType.BATCH == executorType) { executor = new BatchExecutor(this, transaction); } else if (ExecutorType.REUSE == executorType) { executor = new ReuseExecutor(this, transaction); } else { executor = new SimpleExecutor(this, transaction); } if (cacheEnabled) { executor = new CachingExecutor(executor); } executor = (Executor) interceptorChain.pluginAll(executor); return executor; } public void addInterceptor(Interceptor interceptor) { interceptorChain.addInterceptor(interceptor); } }
我们重点关注这行代码:
statementHandler = (StatementHandler) interceptorChain.pluginAll(statementHandler);
上面代码功能是:对statementHandler 插入所有的Interceptor以便进行拦截,InterceptorChain里保存了所有的拦截器,它在Configuration 对象被构造出来的时候创建。
org.apache.ibatis.plugin.InterceptorChain 源代码如下:
public class InterceptorChain { private final List<Interceptor> interceptors = new ArrayList<Interceptor>(); public Object pluginAll(Object target) { for (Interceptor interceptor : interceptors) { target = interceptor.plugin(target); } return target; } public void addInterceptor(Interceptor interceptor) { interceptors.add(interceptor); } public List<Interceptor> getInterceptors() { return Collections.unmodifiableList(interceptors); } }
org.apache.ibatis.plugin.Interceptor 类的代码如下:
public interface Interceptor { Object intercept(Invocation invocation) throws Throwable; Object plugin(Object target); void setProperties(Properties properties); }
org.apache.ibatis.plugin.Invocation 代码如下:
public class Invocation { private Object target; private Method method; private Object[] args; public Invocation(Object target, Method method, Object[] args) { this.target = target; this.method = method; this.args = args; } public Object getTarget() { return target; } public Method getMethod() { return method; } public Object[] getArgs() { return args; } public Object proceed() throws InvocationTargetException, IllegalAccessException { return method.invoke(target, args); } }
org.apache.ibatis.plugin.Plugin 源代码如下:
public class Plugin implements InvocationHandler { private Object target; private Interceptor interceptor; private Map<Class<?>, Set<Method>> signatureMap; private Plugin(Object target, Interceptor interceptor, Map<Class<?>, Set<Method>> signatureMap) { this.target = target; this.interceptor = interceptor; this.signatureMap = signatureMap; } public static Object wrap(Object target, Interceptor interceptor) { Map<Class<?>, Set<Method>> signatureMap = getSignatureMap(interceptor); Class<?> type = target.getClass(); Class<?>[] interfaces = getAllInterfaces(type, signatureMap); if (interfaces.length > 0) { return Proxy.newProxyInstance( type.getClassLoader(), interfaces, new Plugin(target, interceptor, signatureMap)); } return target; } @Override public Object invoke(Object proxy, Method method, Object[] args) throws Throwable { try { Set<Method> methods = signatureMap.get(method.getDeclaringClass()); if (methods != null && methods.contains(method)) { return interceptor.intercept(new Invocation(target, method, args)); } return method.invoke(target, args); } catch (Exception e) { throw ExceptionUtil.unwrapThrowable(e); } } }
责任链模式
责任链模式(Chain Of Responsibility Pattern )在 Wiki 上定义如下:责任链模式在面向对象程式设计里是一种软件设计模式,它包含了一些命令对象和一系列的处理对象。每一个处理对象决定它能处理哪些命令对象,它也知道如何将它不能处理的命令对象传递给该链中的下一个处理对象。该模式还描述了往该处理链的末尾添加新的处理对象的方法。
23种设计模式中非常经典的一个设计模式。
责任链模式应用
1、Servlet FilterChain
package com.bytebeats.mario.web; import java.io.IOException; import javax.servlet.Filter; import javax.servlet.FilterChain; import javax.servlet.FilterConfig; import javax.servlet.ServletException; import javax.servlet.ServletRequest; import javax.servlet.ServletResponse; public class CharacterEncodingFilter implements Filter { public void init(FilterConfig config) throws ServletException { } public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) { chain.doFilter(request, response); } public void destroy() { } }
2、OkHttp Interceptors**
OkHttp Interceptors:https://github.com/square/okhttp/wiki/Interceptors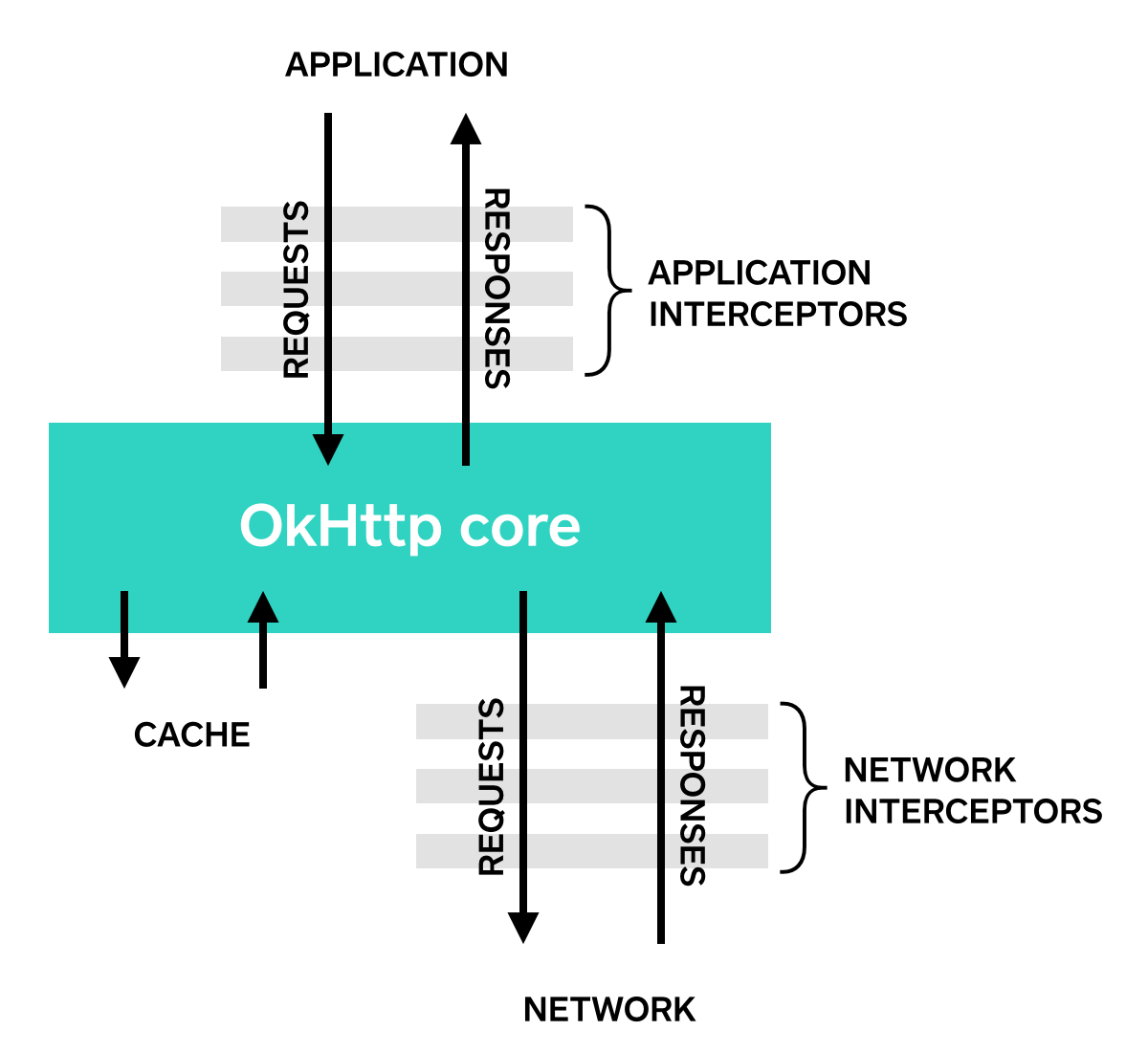
相关文章推荐
- MyBatis 插件之拦截器(Interceptor)实现原理
- Mybatis Interceptor 拦截器原理 源码分析
- struts2拦截器的实现原理及源码剖析
- mybatis -- 由浅入深分析mybatis通过动态代理实现拦截器(插件)的原理
- struts2拦截器的实现原理及源码剖析
- struts2拦截器的实现原理及源码剖析
- struts2拦截器的实现原理及源码剖析
- struts2拦截器的实现原理及源码剖析
- 由浅入深分析mybatis通过动态代理实现拦截器(插件)的原理
- 由浅入深分析mybatis通过动态代理实现拦截器(插件)的原理
- struts2拦截器的实现原理及源码剖析
- struts2拦截器的实现原理及源码剖析
- struts2(八)拦截器的实现原理及源码剖析
- structs2拦截器的实现原理和源码剖析
- 由浅入深分析mybatis通过动态代理实现拦截器(插件)的原理
- Java程序员从笨鸟到菜鸟之(四十六)细谈struts2(八)拦截器的实现原理及源码剖析
- struts2拦截器的实现原理及源码剖析
- struts2拦截器的实现原理及源码剖析
- struts2拦截器的实现原理及源码剖析
- struts2拦截器的实现原理及源码剖析