JDBC:通过 Statement 执行更新操作
2017-01-01 20:22
597 查看
本文内容大多基于官方文档和网上前辈经验总结,经过个人实践加以整理积累,仅供参考。
(2) 通过 Connection 的 createStatement() 方法获取
(3) 通过 executeUpdate(sql) 执行 SQL 语句
(4) 传入的 SQL 可以是 INSERT,UPDATE 和 DELETE,但是不能是 SELECT
(5) Statement 和 Connection 都是应用程序和数据库服务器的连接资源,使用后一定要关闭,需要在 finally 块中关闭,关闭的顺序是先关闭后获取的,即先关闭 Statement 后关闭 Connection
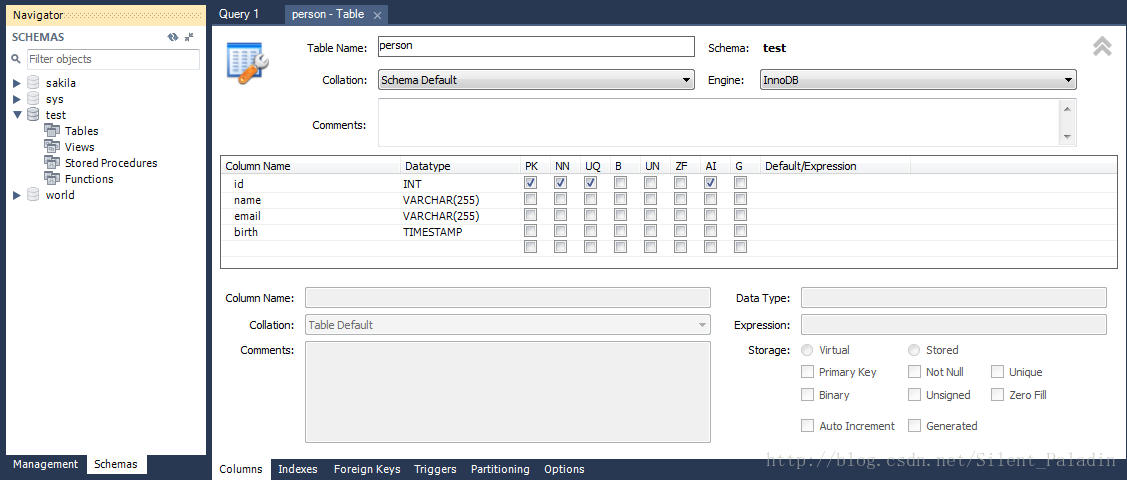
生成的 pom 文件:
(2) 在工程 src/main/resources 目录下新建 JDBC 配置文件:jdbc.properties
(3) 编写测试用例
(4) JDBCTool getConnection()
(5) 运行测试用例

(6) 查询数据库
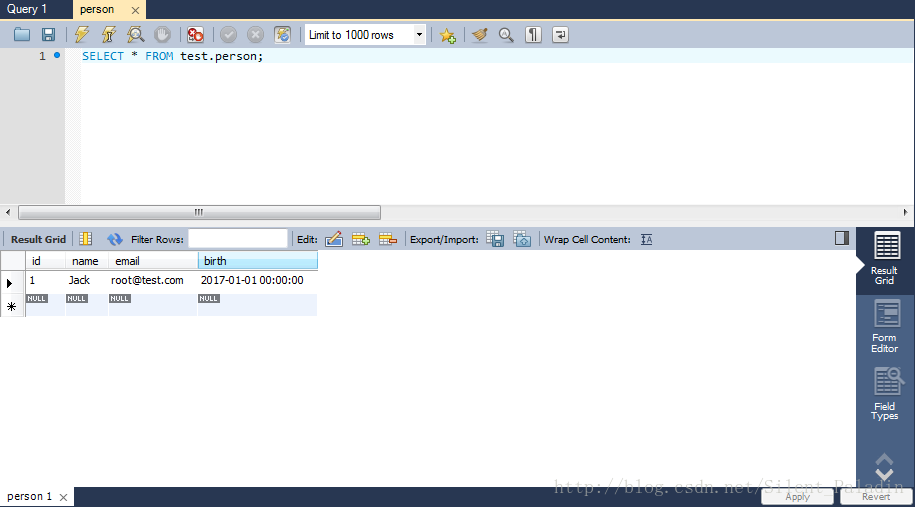
1 Statement 简介
(1) Statement:用于执行 SQL 语句的对象(2) 通过 Connection 的 createStatement() 方法获取
(3) 通过 executeUpdate(sql) 执行 SQL 语句
(4) 传入的 SQL 可以是 INSERT,UPDATE 和 DELETE,但是不能是 SELECT
(5) Statement 和 Connection 都是应用程序和数据库服务器的连接资源,使用后一定要关闭,需要在 finally 块中关闭,关闭的顺序是先关闭后获取的,即先关闭 Statement 后关闭 Connection
2 准备测试数据库
使用 MySQL 作为测试用数据库,新建 Database Test,在 Test 数据库中新建表 person3 在 Eclipse 中创建测试工程
(1) 在 Eclipse 中新建 Maven Project,添加 JUnit 和 MySQL JDBC 驱动依赖(参看:JDBC:通过 DriverManager 获取数据库连接)生成的 pom 文件:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>lesson</groupId> <artifactId>lesson</artifactId> <version>0.0.1-SNAPSHOT</version> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.40</version> </dependency> </dependencies> </project>
(2) 在工程 src/main/resources 目录下新建 JDBC 配置文件:jdbc.properties
jdbc.driver=com.mysql.jdbc.Driver jdbc.url=jdbc:mysql://127.0.0.1:3306/test jdbc.user=root jdbc.password=123456
(3) 编写测试用例
@Test public void testStatement() { java.sql.Connection conn = null; java.sql.Statement stmt = null; try { // 获取数据库连接 conn = JDBCTool.getConnection("jdbc.properties"); // 准备插入的SQL String sql = "INSERT INTO person(name, email, birth) VALUES ('Jack', 'root@test.com', '2017-1-1 00:00:00')"; // 获取操作SQL语句的Statement对象,调用Connection的createStatement方法来获取 stmt = conn.createStatement(); // 调用Statement对象的executeUpdate(sql)执行SQL语句进行插入 stmt.executeUpdate(sql); } catch (Exception e) { e.printStackTrace(); } finally { try { if (stmt != null) { stmt.close(); } } catch (java.sql.SQLException e) { e.printStackTrace(); } try { if (conn != null) { conn.close(); } } catch (java.sql.SQLException e) { e.printStackTrace(); } } }
(4) JDBCTool getConnection()
import java.io.IOException; import java.io.InputStream; import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.util.Properties; public class JDBCTool { /** * 重载的 getConnection()方法 * @throws IOException * @throws ClassNotFoundException * @throws SQLException */ public static Connection getConnection() throws IOException, ClassNotFoundException, SQLException { return getConnection("jdbc.properties"); } /** * @throws ClassNotFoundException * @throws SQLException * */ public static Connection getConnection(String driverClass, String jdbcUrl, String user, String password) throws ClassNotFoundException, SQLException { /* * 注册驱动:加载数据库驱动程序 * 实际上执行:DriverManager.registerDriver(new Driver()) */ Class.forName(driverClass); return DriverManager.getConnection(jdbcUrl, user, password); } /** * @throws IOException * @throws ClassNotFoundException * @throws SQLException * */ public static Connection getConnection(String propertiesFile) throws IOException, ClassNotFoundException, SQLException { // 读取类路径下的jdbc.properties文件 InputStream in = JDBCTool.class.getClassLoader().getResourceAsStream(propertiesFile); Properties info = new Properties(); info.load(in); String driverClass = info.getProperty("jdbc.driver"); String jdbcUrl = info.getProperty("jdbc.url"); String user = info.getProperty("jdbc.user"); String password = info.getProperty("jdbc.password"); return getConnection(driverClass, jdbcUrl, user, password); } }
(5) 运行测试用例
(6) 查询数据库
4 提取通用更新数据库方法
/** * 通用更新方法:包括INSERT,UPDATE,DELETE * @param sql */ public static void update(String sql) { Connection conn = null; Statement stmt = null; try { conn = getConnection("jdbc.properties"); stmt = conn.createStatement(); stmt.executeUpdate(sql); } catch (Exception e) { e.printStackTrace(); } finally { if (stmt != null) { try { stmt.close(); } catch (Exception e) { e.printStackTrace(); } } if (conn != null) { try { conn.close(); } catch (Exception e) { e.printStackTrace(); } } } }
5 提取释放数据库资源的方法
/** * 关闭数据库连接资源 */ public static void release(Statement stmt, Connection conn) { if (stmt != null) { try { stmt.close(); } catch (Exception e) { e.printStackTrace(); } } if (conn != null) { try { conn.close(); } catch (Exception e) { e.printStackTrace(); } } }
6 使用 JDBCTool 工具类重写单元测试代码
@Test public void testStatement() { java.sql.Connection conn = null; java.sql.Statement stmt = null; try { // 准备插入的SQL String sql = "INSERT INTO person(name, email, birth) VALUES ('Jack', 'root@test.com', '2017-1-1 00:00:00')"; // 调用JDBCTool工具类的update(sql)执行SQL语句进行插入 JDBCTool.update(sql); } catch (Exception e) { e.printStackTrace(); } finally { JDBCTool.release(stmt, conn); } }
附:JDBC 工具类
import java.io.IOException;
import java.io.InputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.Properties;
public class JDBCTool {
/**
* 重载的 getConnection()方法
* @throws IOException
* @throws ClassNotFoundException
* @throws SQLException
*/
public static Connection getConnection() throws IOException, ClassNotFoundException, SQLException {
return getConnection("jdbc.properties");
}
/**
* @throws ClassNotFoundException
* @throws SQLException
*
*/
public static Connection getConnection(String driverClass, String jdbcUrl, String user, String password) throws ClassNotFoundException, SQLException {
/*
* 注册驱动:加载数据库驱动程序
* 实际上执行:DriverManager.registerDriver(new Driver())
*/
Class.forName(driverClass);
return DriverManager.getConnection(jdbcUrl, user, password);
}
/**
* @throws IOException
* @throws ClassNotFoundException
* @throws SQLException
*
*/
public static Connection getConnection(String propertiesFile) throws IOException, ClassNotFoundException, SQLException {
// 读取类路径下的jdbc.properties文件
InputStream in = JDBCTool.class.getClassLoader().getResourceAsStream(propertiesFile);
Properties info = new Properties();
info.load(in);
String driverClass = info.getProperty("jdbc.driver");
String jdbcUrl = info.getProperty("jdbc.url");
String user = info.getProperty("jdbc.user");
String password = info.getProperty("jdbc.password");
return getConnection(driverClass, jdbcUrl, user, password);
}
/** * 通用更新方法:包括INSERT,UPDATE,DELETE * @param sql */ public static void update(String sql) { Connection conn = null; Statement stmt = null; try { conn = getConnection("jdbc.properties"); stmt = conn.createStatement(); stmt.executeUpdate(sql); } catch (Exception e) { e.printStackTrace(); } finally { if (stmt != null) { try { stmt.close(); } catch (Exception e) { e.printStackTrace(); } } if (conn != null) { try { conn.close(); } catch (Exception e) { e.printStackTrace(); } } } }
/** * 关闭数据库连接资源 */ public static void release(Statement stmt, Connection conn) { if (stmt != null) { try { stmt.close(); } catch (Exception e) { e.printStackTrace(); } } if (conn != null) { try { conn.close(); } catch (Exception e) { e.printStackTrace(); } } }
}
相关文章推荐
- jdbc中的Statement和PreparedStatement接口对象
- JDBC 数据库常用连接 链接字符串
- JSP中使用JDBC连接MySQL数据库的详细步骤
- java jdbc连接和使用详细介绍
- 使用JDBC4.0操作Oracle中BLOB类型的数据方法
- JDBC连接Access数据库的几种方式介绍
- 基于JDBC封装的BaseDao(实例代码)
- JDBC程序更新数据库中记录的方法
- jdbc链接远程数据库进行修改url操作
- JDBC 程序的常见错误及调试方法
- MyBatis通过JDBC数据驱动生成的执行语句问题
- 在Java的JDBC使用中设置事务回滚的保存点的方法
- JDBC连接数据库的方法汇总
- Java中使用JDBC操作数据库简单实例
- Java加载JDBC驱动程序实例详解
- JDBC数据库连接过程及驱动加载与设计模式详解
- 使用JDBC在MySQL数据库中如何快速批量插入数据
- JSP使用JDBC完成动态验证及采用MVC完成数据查询的方法
- JSP基于JDBC的数据库连接类实例
- JSP中使用JDBC访问SQL Server 2008数据库示例