案例三————健康栏目
2016-12-16 22:16
274 查看
健康栏目的视线分析
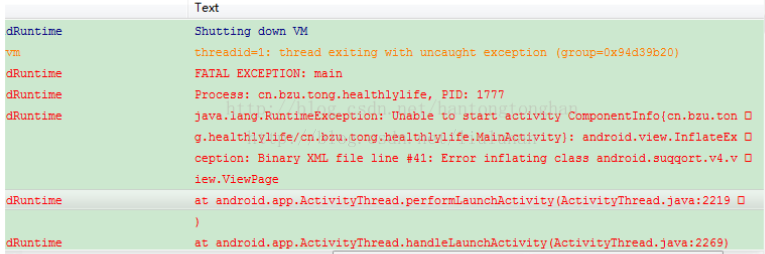
一.预期效果:
二.主要代码:
1.MainActivity.java
public class MainActivity extends Activity { private int currIndex;// 当前页卡编号 private TextView tvCursor; private TextView tvHealthNews; private TextView tvIllnessDefense; private ViewPager vpHealth; private ImageView ivHealthBack; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); requestWindowFeature(Window.FEATURE_NO_TITLE); setContentView(R.layout.activity_main); initViews(); initCursor(); initViewPager(); setListeners(); } private void initViews() { ivHealthBack = (ImageView) findViewById(R.id.icons_health_back); tvCursor = (TextView) findViewById(R.id.cursor); tvHealthNews = (TextView) findViewById(R.id.tv_healthnews); tvIllnessDefense = (TextView) findViewById(R.id.tv_healthill); vpHealth = (ViewPager) findViewById(R.id.viewpager); } private void initCursor() { Display display = getWindow().getWindowManager().getDefaultDisplay(); DisplayMetrics metrics = new DisplayMetrics(); display.getMetrics(metrics); // 取得手机屏幕宽度的一半 int tabLineLength = metrics.widthPixels / 2; // 设置游标的宽度为屏幕宽度的一半 LayoutParams lp = (LayoutParams) tvCursor.getLayoutParams(); lp.width = tabLineLength; tvCursor.setLayoutParams(lp); } private void initViewPager() { vpHealth = (ViewPager) findViewById(R.id.viewpager); List<View> views = new ArrayList<View>(); views.add(new HealthWebView(this) .getView("http://cms.hxky.cn/wap/jkxz/")); views.add(new HealthWebView(this) .getView("http://cms.hxky.cn/wap/jbfz/")); vpHealth.setAdapter(new HealthViewPagerAdapter(views)); // 给 ViewPager 设置适配器 vpHealth.setCurrentItem(0);// 设置当前显示标签页为第一页 } private void setListeners() { // 返回按钮 ivHealthBack.setOnClickListener(new OnClickListener() { @Override public void onClick(View arg0) { finish(); } }); // 点击健康须知 tvHealthNews.setOnClickListener(new OnClickListener() { @Override public void onClick(View view) { vpHealth.setCurrentItem(0); } }); // 点击疾病防治 tvIllnessDefense.setOnClickListener(new OnClickListener() { @Override public void onClick(View view) { vpHealth.setCurrentItem(1); } }); vpHealth.setOnPageChangeListener(new OnPageChangeListener() { @Override public void onPageSelected(int position) { currIndex = position; } @Override public void onPageScrolled(int position, float percent, int ag2) { LinearLayout.LayoutParams ll = (android.widget.LinearLayout.LayoutParams) tvCursor .getLayoutParams(); if (currIndex == position) { ll.leftMargin = (int) (currIndex * tvCursor.getWidth() + percent * tvCursor.getWidth()); } else if (currIndex > position) { ll.leftMargin = (int) (currIndex * tvCursor.getWidth() - (1 - percent) * tvCursor.getWidth()); } tvCursor.setLayoutParams(ll); } @Override public void onPageScrollStateChanged(int position) { } }); } }
2.titlebar.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="wrap_content" android:background="@drawable/titlebar_bg" > <ImageView android:id="@+id/icons_health_back" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:gravity="center" android:paddingLeft="6dp" android:src="@drawable/icons_health_back" /> <TextView android:id="@+id/title" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_gravity="center" android:layout_weight="1" android:gravity="center" android:text="健康" android:textColor="#ffffff" android:textSize="15sp" /> </LinearLayout>
3.pagerofhealth.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" > <WebView android:id="@+id/wvHealth" android:layout_width="match_parent" android:layout_height="match_parent" /> </RelativeLayout>
4.activity_main.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity" > <include layout="@layout/health_layout_titlebar"/> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content"> <!-- 健康新知 --> <TextView android:id="@+id/tv_healthnews" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:layout_weight="1" android:gravity="center" android:padding="6dp" android:text="@string/healthnews" android:textSize="18dp"/> <!-- 疾病防治 --> <TextView android:id="@+id/tv_healthill" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:layout_weight="1" android:gravity="center" android:padding="6dp" android:text="@string/illdefense" android:textSize="18dp"/> </LinearLayout> <TextView android:id="@+id/cursor" android:layout_width="125dp" android:layout_height="5dp" android:layout_marginLeft="20dp" android:background="#990033"/> <android.suqqort.v4.view.ViewPage android:id="@+id/viewpager" android:layout_width="match_parent" android:layout_height="match_parent"/> </LinearLayout>
5.HealthWebView.java
public class HealthWebView { private Context context; public HealthWebView(Context context) { this.context = context; } public View getView(String url) { View view = LayoutInflater.from(context).inflate( R.layout.pagerofhealth, null); WebView webView = (WebView) view.findViewById(R.id.wvHealth); webView.loadUrl(url); // 设置支持 JavaScript 脚本 webView.getSettings().setJavaScriptEnabled(true); /** * 禁止系统浏览器打开页面 */ webView.setWebViewClient(new WebViewClient() { @Override public boolean shouldOverrideUrlLoading(WebView view, String url) { view.loadUrl(url); return true; } }); return view; } }
6.HealthViewPagerAdapter.java
public class HealthViewPagerAdapter extends PagerAdapter{ private List<View> viewList; public HealthViewPagerAdapter(List<View> viewList) { this.viewList = viewList; } public int getCount(){ return viewList.size(); } public boolean isViewFromObject(View view, Object object) { return view == object; } public Object instantiateItem(ViewGroup container, int position) { container.addView(viewList.get(position)); return viewList.get(position); } public void destroyItem(ViewGroup container, int position, Object object) { container.removeView(viewList.get(position)); } }
相关文章推荐
- 在Qt Creator 和在 vs2012 里添加信号和槽
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析
- Docker之Linux UnionFS
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析
- 天池体验(二)——新人离线赛数据可视化分析