spring-cloud客户端负载均衡(初试)
2016-12-14 16:30
357 查看
目录结构
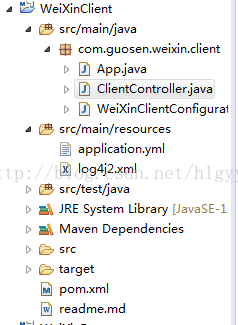
maven
application.yml
log4j2.xml
APP.java
WeiXinClientConfiguration.java
ClientController.java
maven
<groupId>WeiXinClient</groupId> <artifactId>WeiXinClient</artifactId> <version>1.0</version> <packaging>jar</packaging> <name>WeiXinClient</name> <description>weixin client of loadbalance</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.4.2.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> <exclusions> <exclusion> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-logging</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-log4j2</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-ribbon</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>Brixton.BUILD-SNAPSHOT</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <build> <finalName>weixinclient</finalName> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> <repositories> <repository> <id>spring-snapshots</id> <name>Spring Snapshots</name> <url>https://repo.spring.io/snapshot</url> <snapshots> <enabled>true</enabled> </snapshots> </repository> <repository> <id>spring-milestones</id> <name>Spring Milestones</name> <url>https://repo.spring.io/milestone</url> <snapshots> <enabled>false</enabled> </snapshots> </repository> </repositories>
application.yml
spring: application: name: WeiXinClient server: port: 8999 logging: config: classpath:log4j2.xml WeiXinClient: ribbon: eureka: enabled: false listOfServers: 172.24.3.64:8080,172.24.3.64:9092 ServerListRefreshInterval: 15000
log4j2.xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE xml> <!-- Log4j 2.x 配置文件。每30秒自动检查和应用配置文件的更新; --> <Configuration status="warn" monitorInterval="30" strict="true" schema="Log4J-V2.2.xsd"> <Appenders> <!-- 输出到控制台 --> <Console name="Console" target="SYSTEM_OUT"> <PatternLayout pattern="%d{yyyy-MM-dd HH:mm:ss,SSS}:%4p %t (%F:%L) - %m%n" /> </Console> <!-- 输出到文件,按天或者超过80MB分割 --> <RollingFile name="InfoRollingFile" fileName="/root/logs/weixinclient/info.log" filePattern="/root/logs/weixinclient/$${date:yyyy-MM-dd}/info-%d{yyyy-MM-dd}-%i.log"> <!-- 需要记录的级别 --> <ThresholdFilter level="INFO"/> <PatternLayout pattern="%d{yyyy-MM-dd HH:mm:ss,SSS}:%4p %t (%F:%L) - %m%n" /> <Policies> <OnStartupTriggeringPolicy /> <TimeBasedTriggeringPolicy /> <SizeBasedTriggeringPolicy size="80 MB" /> </Policies> </RollingFile> <!-- 输出到文件,按天或者超过80MB分割 --> <RollingFile name="ErrorRollingFile" fileName="/root/logs/weixinclient/error.log" filePattern="/root/logs/weixinclient/$${date:yyyy-MM-dd}/error-%d{yyyy-MM-dd}-%i.log"> <!-- 需要记录的级别 --> <ThresholdFilter level="ERROR"/> <PatternLayout pattern="%d{yyyy-MM-dd HH:mm:ss,SSS}:%4p %t (%F:%L) - %m%n" /> <Policies> <OnStartupTriggeringPolicy /> <TimeBasedTriggeringPolicy /> <SizeBasedTriggeringPolicy size="80 MB" /> </Policies> </RollingFile> </Appenders> <Loggers> <Root level="info"> <!-- 全局配置 --> <AppenderRef ref="Console" /> <AppenderRef ref="InfoRollingFile"/> <AppenderRef ref="ErrorRollingFile"/> </Root> </Loggers> </Configuration>
APP.java
package com.guosen.weixin.client; import java.nio.charset.Charset; import java.util.List; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.client.loadbalancer.LoadBalanced; import org.springframework.cloud.netflix.ribbon.RibbonClient; import org.springframework.context.annotation.Bean; import org.springframework.http.converter.HttpMessageConverter; import org.springframework.http.converter.StringHttpMessageConverter; import org.springframework.web.client.RestTemplate; /** * 发送微信client端 * * 创建于2016年12月12日 * @author guosen * */ @SpringBootApplication @RibbonClient(name = "WeiXinClient", configuration = WeiXinClientConfiguration.class) public class App { @LoadBalanced @Bean RestTemplate restTemplate() { RestTemplate restTemplate = new RestTemplate(); List<HttpMessageConverter<?>> converterList = restTemplate.getMessageConverters(); int count = -1; for (int i = 0; i < converterList.size(); i++) { HttpMessageConverter<?> message = converterList.get(i); if (message.getClass() == StringHttpMessageConverter.class) { count = i; break; } } StringHttpMessageConverter stringConverter = new StringHttpMessageConverter(Charset.forName("UTF-8")); converterList.set(count, stringConverter); return restTemplate; } public static void main( String[] args ){ SpringApplication.run(App.class, args); } }
WeiXinClientConfiguration.java
package com.guosen.weixin.client; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.annotation.Bean; import com.netflix.client.config.IClientConfig; import com.netflix.loadbalancer.AvailabilityFilteringRule; import com.netflix.loadbalancer.IPing; import com.netflix.loadbalancer.IRule; import com.netflix.loadbalancer.PingUrl; public class WeiXinClientConfiguration { @Autowired IClientConfig ribbonClientConfig; @Bean public IPing ribbonPing(IClientConfig config) { return new PingUrl(); } @Bean public IRule ribbonRule(IClientConfig config) { return new AvailabilityFilteringRule(); } }
ClientController.java
package com.guosen.weixin.client; import java.util.HashMap; import java.util.Map; import javax.servlet.http.HttpServletRequest; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RestController; import org.springframework.web.client.RestTemplate; import com.fasterxml.jackson.core.JsonProcessingException; import com.fasterxml.jackson.databind.ObjectMapper; @RestController public class ClientController { private static final Logger logger = LoggerFactory.getLogger(ClientController.class); @Autowired RestTemplate restTemplate; /** * 通过get方法发送微信,get请求信息必须包括(touser、toparty、totag)中的一种、agentid、content * @param request * @return */ @RequestMapping(value = "/send/text/byget", method = RequestMethod.GET) public String sendTextByGet(HttpServletRequest request){ // 消息 Map<String, Object> map = new HashMap<String, Object>(); ObjectMapper om = new ObjectMapper(); Map<String, Object> results = new HashMap<String, Object>(); String result = ""; String touser = request.getParameter("touser"); String toparty = request.getParameter("toparty"); String totag = request.getParameter("totag"); String agentid = request.getParameter("agentid"); String content = request.getParameter("content"); if ((null == touser || "".equals(touser)) && (null == toparty || "".equals(toparty)) && (null == totag || "".equals(totag))) { results.put("errcode", 9999); results.put("errmsg", "touser、toparty、totag必须选填一个"); try { result = om.writeValueAsString(results); } catch (JsonProcessingException e) { e.printStackTrace(); } return result; } boolean formatFlag = false; int agent = 0; try { agent = Integer.parseInt(agentid); formatFlag = true; } catch (NumberFormatException e2) { e2.printStackTrace(); } if (null == agentid || "".equals(agentid) || !formatFlag) { results.put("errcode", 9999); results.put("errmsg", "agentid不能为空,并且为数字"); try { result = om.writeValueAsString(results); } catch (JsonProcessingException e) { e.printStackTrace(); } return result; } if (null == content || "".equals(content)) { results.put("errcode", 9999); results.put("errmsg", "content不能为空,并且必填"); try { result = om.writeValueAsString(results); } catch (JsonProcessingException e) { e.printStackTrace(); } return result; } Map<String, String> cmap = new HashMap<String, String>(); cmap.put("content", content); map.put("safe", 0); map.put("msgtype", "text"); map.put("agentid", agent); map.put("text", cmap); if (null != touser && !"".equals(touser)) { map.put("touser", touser); } if (null != toparty && !"".equals(toparty)) { map.put("toparty", toparty); } if (null != totag && !"".equals(totag)) { map.put("totag", totag); } // 将参数map转换为json对象并且请求发送服务 try { String data = om.writeValueAsString(map); logger.info(data); result = this.restTemplate.postForObject("http://WeiXinClient/sendText", data, String.class); } catch (Exception e) { logger.error("发送微信信息异常", e); results.put("errcode", 9999); results.put("errmsg", e.getMessage()); try { result = om.writeValueAsString(results); } catch (JsonProcessingException e1) { e1.printStackTrace(); } } return result; } /** * 通过post json字符串的方式发送微信,post内容为{"touser":"xxx|xxx","content":"xxx","agentid":xx} 其中touser可以改为toparty、totag * @param requestData * @return */ @SuppressWarnings("unchecked") @RequestMapping(value = "/send/text/bypost", method = RequestMethod.POST) public String sendTextByPost(@RequestBody String requestData){ // 消息 ObjectMapper om = new ObjectMapper(); Map<String, Object> results = new HashMap<String, Object>(); String result = ""; // 将参数map转换为json对象并且请求发送服务 try { Map<String, Object> requestMap = om.readValue(requestData, Map.class); requestMap.put("safe", 0); requestMap.put("msgtype", "text"); Map<String, String> cmap = new HashMap<String, String>(); cmap.put("content", (String)requestMap.get("content")); requestMap.put("text", cmap); requestMap.remove("content"); String data = om.writeValueAsString(requestMap); result = this.restTemplate.postForObject("http://WeiXinClient/sendText", data, String.class); } catch (Exception e) { logger.error("发送微信信息异常", e); results.put("errcode", 9999); results.put("errmsg", e.getMessage()); try { result = om.writeValueAsString(results); } catch (JsonProcessingException e1) { e1.printStackTrace(); } } return result; } /** * 通过post字符串的方式发送微信,post内容为content=xxxx&agentid=xxx&touser=xxx * @param content * @param touser * @param agentid * @return */ @RequestMapping(value = "/entp", method = RequestMethod.POST) public String sendText(String content, String touser, String agentid){ // 消息 ObjectMapper om = new ObjectMapper(); Map<String, Object> results = new HashMap<String, Object>(); String result = ""; // 将参数map转换为json对象并且请求发送服务 try { Map<String, Object> requestMap = new HashMap<String, Object>(); requestMap.put("safe", 0); requestMap.put("msgtype", "text"); Map<String, String> cmap = new HashMap<String, String>(); cmap.put("content", content); requestMap.put("text", cmap); requestMap.put("touser", touser); requestMap.put("agentid", agentid); String data = om.writeValueAsString(requestMap); result = this.restTemplate.postForObject("http://WeiXinClient/sendText", data, String.class); } catch (Exception e) { logger.error("发送微信信息异常", e); results.put("errcode", 9999); results.put("errmsg", e.getMessage()); try { result = om.writeValueAsString(results); } catch (JsonProcessingException e1) { e1.printStackTrace(); } } return result; } }
相关文章推荐
- spring cloud Ribbon 2 (客户端负载均衡)
- Spring Cloud入门教程-Ribbon实现客户端负载均衡
- Spring Cloud微服务(4)之Ribbon客户端负载均衡
- springcloud使用ribbon实现客户端负载均衡
- Spring Cloud Learning | 第三篇:客户端负载均衡(Ribbon)
- SpringCloud实战2-Ribbon客户端负载均衡
- 客户端负载均衡Ribbon之一:Spring Cloud Netflix负载均衡组件Ribbon介绍
- 服务注册发现Eureka之三:Spring Cloud Ribbon实现客户端负载均衡(客户端负载均衡Ribbon之三:使用Ribbon实现客户端的均衡负载)
- Spring Cloud入门教程(二):客户端负载均衡(Ribbon)
- Spring Cloud Ribbon实现客户端负载均衡
- Spring Cloud入门教程-Ribbon实现客户端负载均衡
- Spring Cloud Ribbon 客户端负载均衡
- spring cloud中使用Ribbon实现客户端的软负载均衡
- Spring Cloud Ribbon——客户端负载均衡
- 笔记:Spring Cloud Ribbon 客户端负载均衡
- Spring Cloud 负载均衡后,某个服务挂掉后保证数据一致性
- Spring Cloud -- Ribbon负载均衡
- SpringCloud的服务注册中心(二)注册中心服务端和两个微服务应用客户端
- SpringCloud微服务搭建教程有负载均衡
- 使用Spring Cloud Feign作为HTTP客户端调用远程HTTP服务