Android实现加载富文本以及SpannableString、SpannableStringBuilder实现部分文字可以点击,更换颜色
2016-12-13 15:41
549 查看
最近项目中要实现部分文字变颜色,并且是可点击的。网上找了一下,实现的方式是android端加载富文本,如果你会js的话,那就方便了,表示本人不怎么会,而且项目框架和界面已经确定了,不可能再改,所以只能试一试其他的方式。
SpannableString、SpannableStringBuilder的详细具体用法可以参考这个博客:
http://blog.csdn.net/qq_24530405/article/details/50506519
第一种方式:
如果文字是固定不变的,可以在String.xml中配置的,可以采用网上最普遍的方式。
我们先来看看效果:
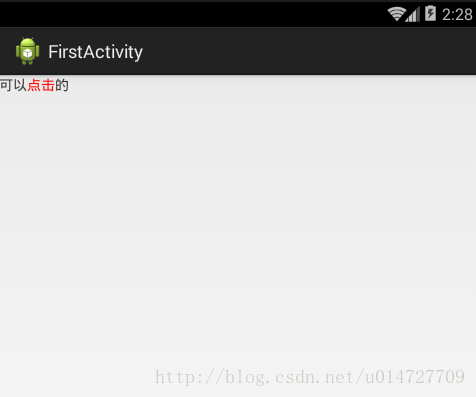
文字的颜色改变了,并且变红的两个字是有点击事件的。
下面直接看代码,布局文件就只有一个TextView,非常简单。
注意:这里使用的是SpannableStringBuilder,这和java里面StringBuilder性质是一样的,有append方法,可以不断的追加。
第二种方式:
通过正则表达式。
还是先看效果,颜色改变了,并且是可点击的。
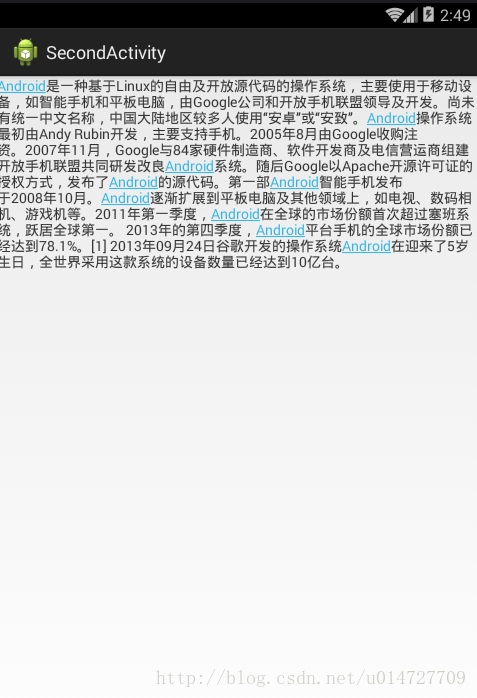
这里,我使用的是自定义TextView,下面贴上代码。
然后是在activity中使用的代码:
第三种方式:
是在github上看到的,具体是那儿,忘了,不好意思。
这个和第二个是类似的,这个也是自定义TextView,只不过这个是在xml文件中进行关键字的配置。
先看效果:
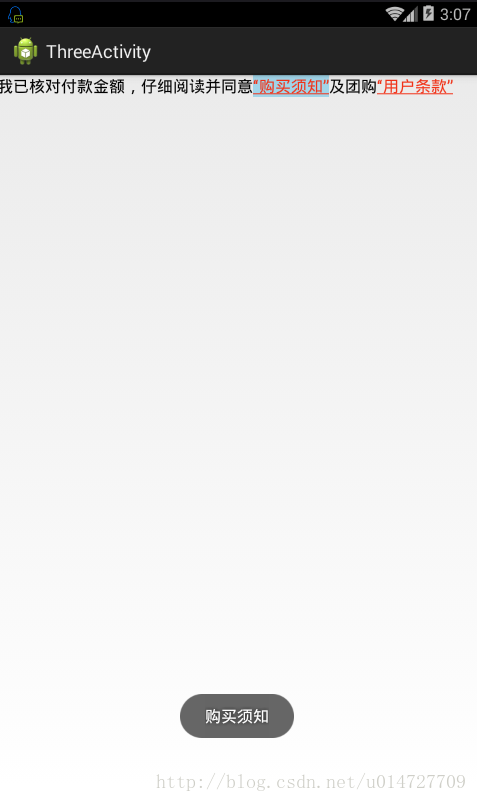
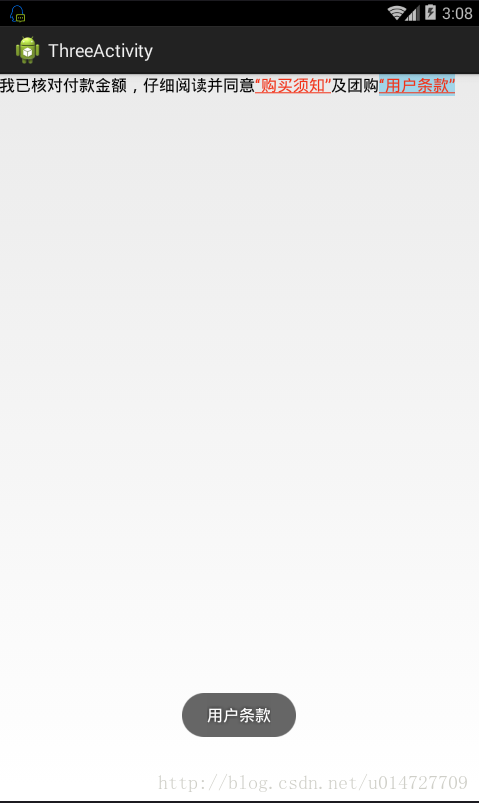
贴上代码:
然后是在activity里面的调用:
第四种方式:
使用Html.fromHtml来加载富文本。
先看效果,第一次录制gif文件,效果有点差哈,不要介意。
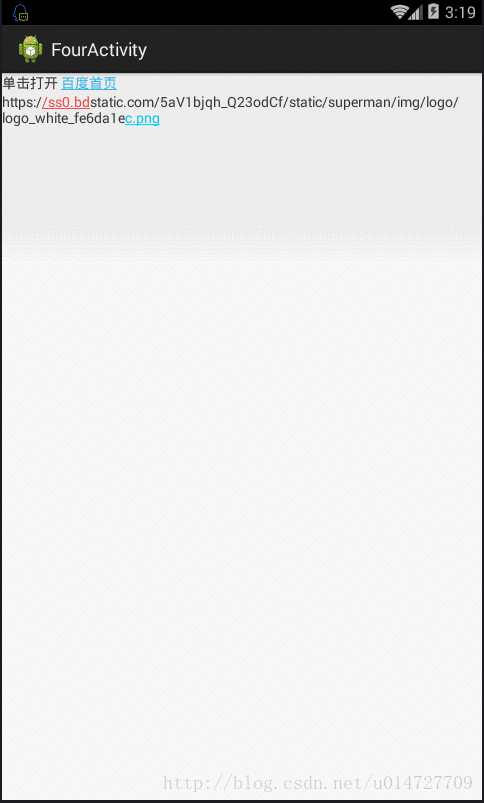
下面看见代码:
布局文件就两个TextView。
最后给出下载链接:
http://download.csdn.net/detail/u014727709/9710194
转载自: http://blog.csdn.net/u014727709/article/details/53610381
欢迎start,欢迎评论,欢迎指正
SpannableString、SpannableStringBuilder的详细具体用法可以参考这个博客:
http://blog.csdn.net/qq_24530405/article/details/50506519
第一种方式:
如果文字是固定不变的,可以在String.xml中配置的,可以采用网上最普遍的方式。
我们先来看看效果:
文字的颜色改变了,并且变红的两个字是有点击事件的。
下面直接看代码,布局文件就只有一个TextView,非常简单。
import android.app.Activity; import android.graphics.Color; import android.os.Bundle; import android.text.Spannable; import android.text.SpannableStringBuilder; import android.text.TextPaint; import android.text.method.LinkMovementMethod; import android.text.style.ClickableSpan; import android.text.style.ForegroundColorSpan; import android.util.Log; import android.view.View; import android.widget.TextView; public class FirstActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_first); TextView textView = (TextView) findViewById(R.id.text1); SpannableStringBuilder spannable = new SpannableStringBuilder("可以点击的"); //设置文字的前景色 spannable.setSpan(new ForegroundColorSpan(Color.RED),2,4,Spannable.SPAN_EXCLUSIVE_EXCLUSIVE); //这个一定要记得设置,不然点击不生效 textView.setMovementMethod(LinkMovementMethod.getInstance()); spannable.setSpan(new TextClick(),2,4 , Spannable.SPAN_EXCLUSIVE_EXCLUSIVE); textView.setText(spannable); } private class TextClick extends ClickableSpan{ @Override public void onClick(View widget) { //在此处理点击事件 Log.e("------->", "点击了"); } @Override public void updateDrawState(TextPaint ds) { // ds.setColor(ds.linkColor); // ds.setUnderlineText(true); } } }
注意:这里使用的是SpannableStringBuilder,这和java里面StringBuilder性质是一样的,有append方法,可以不断的追加。
第二种方式:
通过正则表达式。
还是先看效果,颜色改变了,并且是可点击的。
这里,我使用的是自定义TextView,下面贴上代码。
import java.util.ArrayList; import java.util.List; import android.content.Context; import android.text.Spannable; import android.text.SpannableStringBuilder; import android.text.TextPaint; import android.text.method.LinkMovementMethod; import android.text.style.ClickableSpan; import android.text.style.ForegroundColorSpan; import android.util.AttributeSet; import android.util.Log; import android.view.View; import android.widget.TextView; public class MyTextView extends TextView { public MyTextView(Context context, AttributeSet attrs) { super(context, attrs); } public void setSpecifiedTextsColor(String text, String specifiedTexts, int color) { List<Integer> sTextsStartList = new ArrayList<>(); int sTextLength = specifiedTexts.length(); String temp = text; int lengthFront = 0;//记录被找出后前面的字段的长度 int start = -1; do { start = temp.indexOf(specifiedTexts); if(start != -1) { start = start + lengthFront; sTextsStartList.add(start); lengthFront = start + sTextLength; temp = text.substring(lengthFront); } }while(start != -1); SpannableStringBuilder styledText = new SpannableStringBuilder(text); for(Integer i : sTextsStartList) { styledText.setSpan( new ForegroundColorSpan(color), i, i + sTextLength, Spannable.SPAN_EXCLUSIVE_EXCLUSIVE); //这个一定要记得设置,不然点击不生效 setMovementMethod(LinkMovementMethod.getInstance()); styledText.setSpan(new TextClick(),i,i + sTextLength , Spannable.SPAN_EXCLUSIVE_EXCLUSIVE); } setText(styledText); } private class TextClick extends ClickableSpan{ @Override public void onClick(View widget) { //在此处理点击事件 Log.e("------->", "点击了"); // widget.seth } @Override public void updateDrawState(TextPaint ds) { ds.setColor(ds.linkColor); ds.setUnderlineText(true); } } }
然后是在activity中使用的代码:
import java.util.regex.Matcher; import java.util.regex.Pattern; import android.app.Activity; import android.graphics.Color; import android.os.Bundle; import android.text.method.LinkMovementMethod; public class SecondActivity extends Activity { private MyTextView textView; public String text = "Android是一种基于Linux的自由及开放源代码的操作系统,主要使用于移动设备,如智能手机和平板电脑,由Google公司和开放手机联盟领导及开发。尚未有统一中文名称,中国大陆地区较多人使用“安卓”或“安致”。Android操作系统最初由Andy Rubin开发,主要支持手机。2005年8月由Google收购注资。2007年11月,Google与84家硬件制造商、软件开发商及电信营运商组建开放手机联盟共同研发改良Android系统。随后Google以Apache开源许可证的授权方式,发布了Android的源代码。第一部Android智能手机发布于2008年10月。Android逐渐扩展到平板电脑及其他领域上,如电视、数码相机、游戏机等。2011年第一季度,Android在全球的市场份额首次超过塞班系统,跃居全球第一。 2013年的第四季度,Android平台手机的全球市场份额已经达到78.1%。[1] 2013年09月24日谷歌开发的操作系统Android在迎来了5岁生日,全世界采用这款系统的设备数量已经达到10亿台。"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_second); // 正则表达式。表示连续6位的数字,可以在这边修改成自己所要的格式 Pattern pattern = Pattern.compile("[1-9]\\d*[TBU][1-9]\\d*"); String code = ""; // 匹配短信内容 Matcher matcher = pattern.matcher(text); if (matcher.find()) { code = matcher.group(0); } textView = (MyTextView)findViewById(R.id.tv); textView.setMovementMethod(LinkMovementMethod.getInstance()); textView.setSpecifiedTextsColor(text, "Android", Color.parseColor("#FF0000")); textView.setHighlightColor(getResources().getColor(android.R.color.transparent));//设置点击后的颜色 } }
第三种方式:
是在github上看到的,具体是那儿,忘了,不好意思。
这个和第二个是类似的,这个也是自定义TextView,只不过这个是在xml文件中进行关键字的配置。
先看效果:
贴上代码:
import android.content.Context; import android.content.res.TypedArray; import android.graphics.Color; import android.text.SpannableString; import android.text.Spanned; import android.text.TextPaint; import android.text.TextUtils; import android.text.method.LinkMovementMethod; import android.text.style.ClickableSpan; import android.util.AttributeSet; import android.view.View; import android.widget.TextView; /** * Created by Shaolei on 2016/10/10. */ public class AutoLinkStyleTextView extends TextView { private static String DEFAULT_TEXT_VALUE = null; private static int DEFAULT_COLOR = Color.parseColor("#f23218"); private static boolean HAS_UNDER_LINE = true; private ClickCallBack mClickCallBack; public AutoLinkStyleTextView(Context context) { this(context, null); } public AutoLinkStyleTextView(Context context, AttributeSet attrs) { this(context, attrs, 0); } public AutoLinkStyleTextView(Context context, AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); init(context, attrs, defStyleAttr); } private void init(Context context, AttributeSet attrs, int defStyleAttr) { TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.AutoLinkStyleTextView, defStyleAttr, 0); DEFAULT_TEXT_VALUE = typedArray.getString(R.styleable.AutoLinkStyleTextView_AutoLinkStyleTextView_text_value); DEFAULT_COLOR = typedArray.getColor(R.styleable.AutoLinkStyleTextView_AutoLinkStyleTextView_default_color, DEFAULT_COLOR); HAS_UNDER_LINE = typedArray.getBoolean(R.styleable.AutoLinkStyleTextView_AutoLinkStyleTextView_has_under_line, HAS_UNDER_LINE); // setAutoLinkStyleTextView_text_value("“购买”,“须知”"); addStyle(); typedArray.recycle(); } private void addStyle(){ if (!TextUtils.isEmpty(DEFAULT_TEXT_VALUE) && DEFAULT_TEXT_VALUE.contains(",")) { String[] values = DEFAULT_TEXT_VALUE.split(","); // Log.e("---------->值", DEFAULT_TEXT_VALUE); SpannableString spannableString = new SpannableString(getText().toString().trim()); for (int i = 0; i < values.length; i++){ final int position = i; spannableString.setSpan(new ClickableSpan() { @Override public void onClick(View widget) { if (mClickCallBack != null) mClickCallBack.onClick(position); } @Override public void updateDrawState(TextPaint ds) { super.updateDrawState(ds); ds.setColor(DEFAULT_COLOR); ds.setUnderlineText(HAS_UNDER_LINE); } }, getText().toString().trim().indexOf(values[i]), getText().toString().trim().indexOf(values[i]) + values[i].length(), Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); } setText(spannableString); setMovementMethod(LinkMovementMethod.getInstance()); } } // public void setAutoLinkStyleTextView_text_value(String text) { // DEFAULT_TEXT_VALUE = text; // Log.e("-------------", "调用几次"+DEFAULT_TEXT_VALUE); // addStyle(); // }; public void setOnClickCallBack(ClickCallBack clickCallBack){ this.mClickCallBack = clickCallBack; } public interface ClickCallBack{ void onClick(int position); } }
然后是在activity里面的调用:
import android.app.Activity; import android.os.Bundle; import android.widget.Toast; public class ThreeActivity extends Activity { private AutoLinkStyleTextView autoLinkStyleTextView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_three); autoLinkStyleTextView = (AutoLinkStyleTextView) findViewById(R.id.tv); autoLinkStyleTextView.setOnClickCallBack(new AutoLinkStyleTextView.ClickCallBack() { @Override public void onClick(int position) { if (position == 0) { Toast.makeText(ThreeActivity.this, "购买须知", Toast.LENGTH_SHORT).show(); } else if (position == 1) { Toast.makeText(ThreeActivity.this, "用户条款", Toast.LENGTH_SHORT).show(); } } }); } }
第四种方式:
使用Html.fromHtml来加载富文本。
先看效果,第一次录制gif文件,效果有点差哈,不要介意。
下面看见代码:
布局文件就两个TextView。
import android.app.Activity; import android.os.Bundle; import android.text.Html; import android.text.Spannable; import android.text.SpannableString; import android.text.Spanned; import android.text.method.LinkMovementMethod; import android.text.style.ClickableSpan; import android.text.style.ForegroundColorSpan; import android.view.View; import android.widget.TextView; import android.widget.Toast; public class FourActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_four); TextView tips = (TextView) findViewById(R.id.text); // https://ss0.bdstatic.com/5aV1bjqh_Q23odCf/static/superman/img/logo/logo_white_fe6da1ec.png String str = "单击打开 <a href='http://www.baidu.com/'>百度首页</a>"; tips.setText(Html.fromHtml(str)); // Html.fromHtml( // "<b>text3:</b> Text with a " + // "<a href=\"http://www.google.com\">link</a> " + // "created in the Java source code using HTML.")) tips.setMovementMethod(LinkMovementMethod.getInstance()); TextView protocalTv = (TextView) findViewById(R.id.text1); String str1 = "https://ss0.bdstatic.com/5aV1bjqh_Q23odCf/static/superman/img/logo/logo_white_fe6da1ec.png"; SpannableString spannableString1 = new SpannableString(str1); spannableString1.setSpan(new ClickableSpan() { public void onClick(View widget) { Toast.makeText(getApplicationContext(), "点击了第一处", 0).show(); } }, str1.length() - 5, str1.length(), Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); spannableString1.setSpan(new ForegroundColorSpan(getResources() .getColor(android.R.color.holo_blue_bright)), str1.length() - 5, str1.length(), Spannable.SPAN_EXCLUSIVE_INCLUSIVE); spannableString1.setSpan(new ClickableSpan() { public void onClick(View widget) { Toast.makeText(getApplicationContext(), "点击了第二处", 0).show(); } }, 7, 14, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); spannableString1.setSpan(new ForegroundColorSpan(getResources() .getColor(android.R.color.holo_red_light)), 7, 14, Spannable.SPAN_EXCLUSIVE_INCLUSIVE); protocalTv.setText(spannableString1); protocalTv.setMovementMethod(LinkMovementMethod.getInstance()); } }
最后给出下载链接:
http://download.csdn.net/detail/u014727709/9710194
转载自: http://blog.csdn.net/u014727709/article/details/53610381
欢迎start,欢迎评论,欢迎指正
相关文章推荐
- Android实现加载富文本以及SpannableString、SpannableStringBuilder实现部分文字可以点击,更换颜色
- android Textview 实现展开收缩功能+部分文字点击 (SpannableString)
- android textview可以设置文字颜色 部分文字点击事件处理
- Android利用SpannableStringBuilder设置TextView中部分文字的颜色...
- Android中为TextView中的部分文字设置颜色和点击事件
- 让部分TextView里的文字可以点击并改变颜色
- Android View的点击事件导致文字颜色变化的实现原理
- Android - SpannableString或SpannableStringBuilder以及string.xml文件中的整型和string型代替
- 浅谈ClickableSpan , 实现TextView文本某一部分文字的点击响应
- android - SpannableString或SpannableStringBuilder以及string.xml文件中的整型和string型代替
- 浅谈ClickableSpan , 实现TextView文本某一部分文字的点击响应
- Android 点击按钮同时更换按钮背景颜色和按钮文本颜色
- Android TextView 嵌套图片及其点击,TextView 部分文字点击,文字多颜色
- Android基础:android - SpannableString或SpannableStringBuilder以及string.xml文件中的整型和string型代替(转载)
- android 实现textview部分文字点击效果,类似于微博的话题丶用户
- android - SpannableString或SpannableStringBuilder以及string.xml文件中的整型和string型代替
- 使用SpannableString设置部分文字大小、颜色、超链接、点击事件
- android - SpannableString或SpannableStringBuilder以及string.xml文件中的整型和string型代替
- Android 中TextView部分文字颜色、点击跳转设置
- Android 部分文字颜色大小点击事件的处理