Android使用webService(发送xml数据的方式,不使用jar包)
2016-12-07 09:04
337 查看
Android使用webService可以用ksoap2.jar包来使用。但是我觉得代码不好理解,而且记不住。
所以我查询了好多资料,以及自己的理解。可以用代码发送http请求(发送xml数据)来访问WebService的功能。
这里我主要是用到了http://www.webxml.com.cn上的webService。
打开网页,我们会看到这个网站里面有很多的web服务,比如说查询手机归属地,查询天气预报,等等。
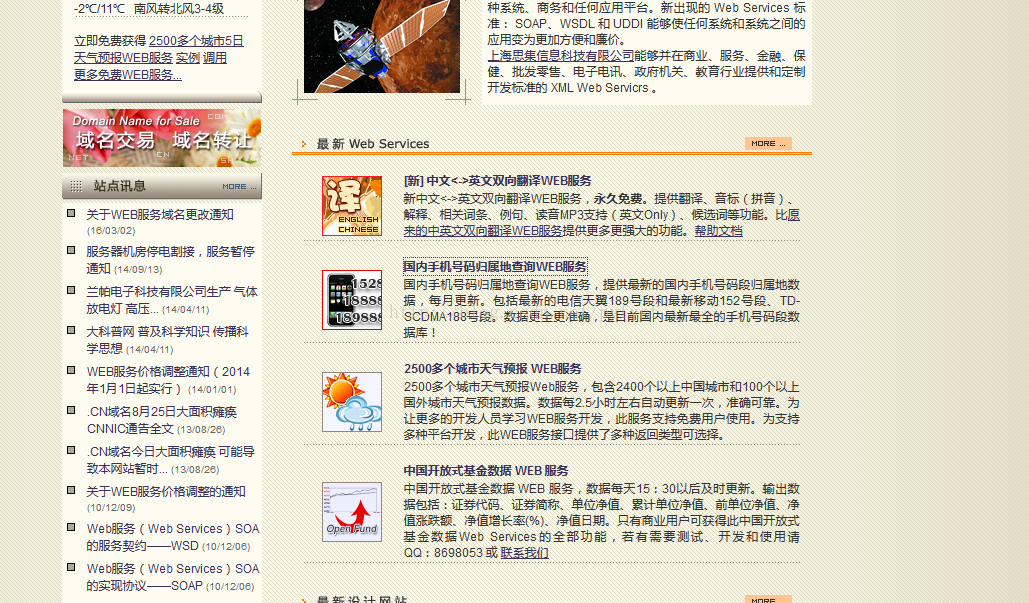
我们在这里以查询手机号的为Demo,总结一下如何使用WebService
布局文件的界面:
布局文件编写:
查询www.webxml.com.cn网址上服务的访问方式:
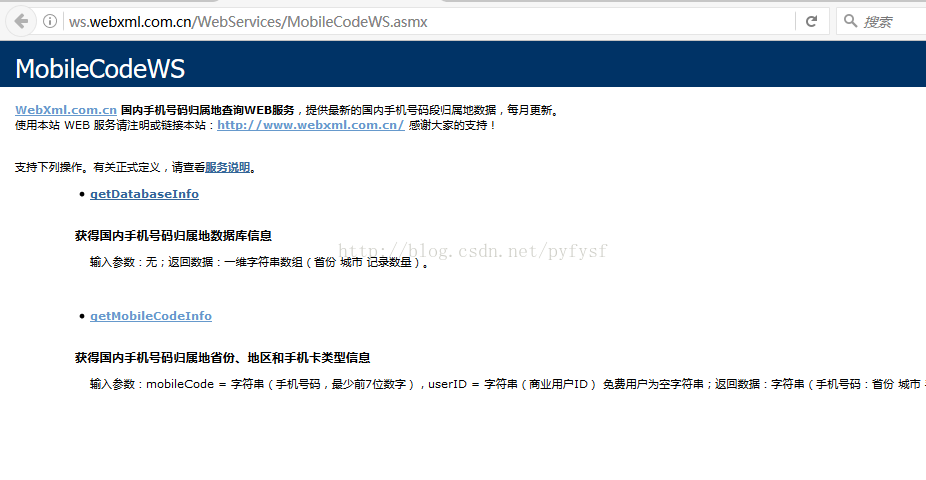
选择我们调用的方法:
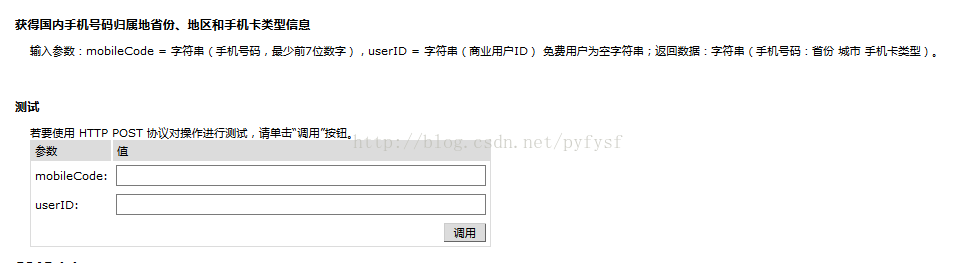
我们可以在网页上试试这个方法的调用,输入我们想要查询的手机号码,userID--为空,点击调用即可查询出来。
在下面会有soap1.1和soap1.2的请求响应实例。
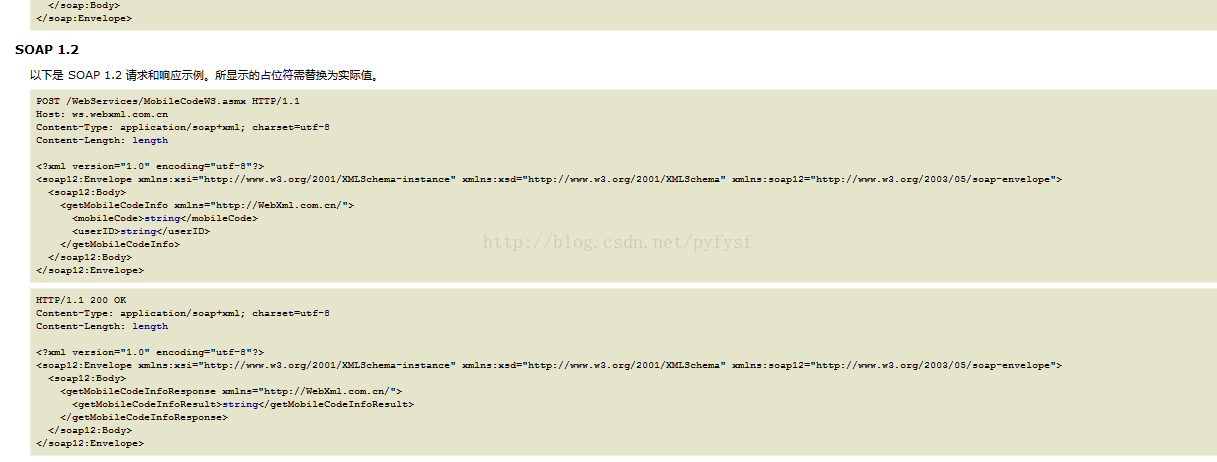
我们只需要把请求的xml文件发送既可返回一个响应的xml文件。
所以我们就可以通过发送http请求,解析http响应就可以获得我们想要的功能。
在src目录下创建一个xml文件,我这里命名为queryPhone.xml
文件内容就是上面图片中的内容
下面我们开始写查询手机号码归属地的业务逻辑类(QueryPhone.java)
package com.example.webservice.service;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import android.util.Log;
import com.example.webservice.Utils.WebServiceUtil;
/**
* 用于查询手机号码归属地的业务逻辑类
*
* @author info
*
*/
public class QueryPhone {
private static String address;
private static String tag = "QueryPhone";
public static String queryAddressByPhone(String phone) {
// 这是我们访问网络的url地址-就是webxml网站上提供的方法那个网页的地址的前半部分
String path = "http://ws.webxml.com.cn/WebServices/MobileCodeWS.asmx";
// 读取本地的xml文件queryPhone.xml
InputStream is = QueryPhone.class.getClassLoader().getResourceAsStream(
"queryPhone.xml");
// 调用工具类中的方法,把流转换为String
String soap = WebServiceUtil.stream2String(is);
// 替换我们调用方法的参数
soap = soap.replaceAll("phoneNumber", phone);
// 把需要传输的数据转换为字节数组
byte[] datas = soap.getBytes();
// Log.i(tag ,"本地文件"+soap);
try {
// 获取连接
HttpURLConnection conn = (HttpURLConnection) new URL(path)
.openConnection();
// 设置超时时间
conn.setConnectTimeout(4000);
// 设置请求方式
conn.setRequestMethod("POST");
// 允许发送数据
conn.setDoOutput(true);
// 设置请求头信息--不是固定的,有wenxml网站提供的
conn.setRequestProperty("Content-Type", "text/xml; charset=utf-8");
conn.setRequestProperty("Content-Length",
String.valueOf(datas.length));
// 发送数据
conn.getOutputStream().write(datas);
Log.i(tag, "==响应码======" + conn.getResponseCode());
// 获取响应码
if (conn.getResponseCode() == 200) {
// 访问网络成功--获取返回的流数据
InputStream resultIs = conn.getInputStream();
// 我们知道这个流数据里面就是一个xml文件,我们解析xml文档
address = WebServiceUtil.parseXml(resultIs);
Log.i(tag, "结果是:" + address);
}
} catch (Exception exception) {
exception.printStackTrace();
}
return address;
}
}
我们使用到的工具类
package com.example.webservice.Utils;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import org.xmlpull.v1.XmlPullParser;
import android.util.Xml;
public class WebServiceUtil {
/**
* 把流转换为String
*
* @param is
* 需要转换的流
* @return 返回转化后的数据 返回null则代表是有异常
*
*/
public static String stream2String(InputStream is) {
String result = null;
ByteArrayOutputStream baos = null;
try {
baos = new ByteArrayOutputStream();
// 转化流
byte[] buffer = new byte[1024];
int len = -1;
while ((len = is.read(buffer)) != -1) {
baos.write(buffer, 0, len);
}
// 转换为字符串
result = baos.toString();
} catch (Exception e) {
e.printStackTrace();
} finally {
// 关闭流
if (is != null) {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
} finally {
is = null;
}
}
if (baos != null) {
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
} finally {
baos = null;
}
}
}
return result;
}
/**
* 解析返回的xml文件
*
* @param resultIs
* 需要解析的流数据
* @return 返回解析后的结果
*/
public static String parseXml(InputStream resultIs) {
XmlPullParser parser = Xml.newPullParser();
try {
parser.setInput(resultIs, "utf-8");
int type = parser.getEventType();
while (type != XmlPullParser.END_DOCUMENT) {
/*
* <?xml version="1.0" encoding="utf-8"?> <soap12:Envelope
* xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
* xmlns:xsd="http://www.w3.org/2001/XMLSchema"
* xmlns:soap12="http://www.w3.org/2003/05/soap-envelope">
* <soap12:Body> <getMobileCodeInfoResponse
* xmlns="http://WebXml.com.cn/">
* <getMobileCodeInfoResult>string</getMobileCodeInfoResult>
* </getMobileCodeInfoResponse> </soap12:Body>
* </soap12:Envelope>
*/
// 我们根据返回回来的xml文件的格式,获取我们需要的节点内容
switch (type) {
case XmlPullParser.START_TAG:
if (parser.getName().equals("getMobileCodeInfoResult")) {
return parser.nextText();
}
break;
}
type = parser.next();
}
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
}
我们在MainActivity中进行查询即可:
package com.example.webservice;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import com.example.webservice.service.QueryPhone;
public class MainActivity extends Activity {
private EditText et_phone;
private Button btn_query;
private TextView tv_result;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 找到控件
et_phone = (EditText) findViewById(R.id.et_phone);
btn_query = (Button) findViewById(R.id.btn_query);
tv_result = (TextView) findViewById(R.id.tv_result);
// 设置按钮的点击事件
btn_query.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
// 获取手机号码
final String phone = et_phone.getText().toString().trim();
// 开启子线程查询手机归属地
new Thread() {
public void run() {
final String address = QueryPhone.queryAddressByPhone(phone);
// 不能在子线程中修改UI
runOnUiThread(new Runnable() {
public void run() {
tv_result.setText(address);
}
});
};
}.start();
}
});
}
}
不要忘记了增加联网的权限:
<uses-permission android:name="android.permission.INTERNET"/>
这样我们就可以查询手机号码的归属地了。
当然输入的手机号码必须大于7位数字。
完成后的效果图片:
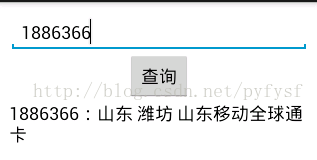
当然我们也可以用这样的方式访问其他的webService---天气预报,验证码等等。
写的第一篇博客,欢迎批评。
所以我查询了好多资料,以及自己的理解。可以用代码发送http请求(发送xml数据)来访问WebService的功能。
这里我主要是用到了http://www.webxml.com.cn上的webService。
打开网页,我们会看到这个网站里面有很多的web服务,比如说查询手机归属地,查询天气预报,等等。
我们在这里以查询手机号的为Demo,总结一下如何使用WebService
布局文件的界面:
布局文件编写:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:padding="10dp" tools:context=".MainActivity" > <EditText android:id="@+id/et_phone" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="输入手机号码" /> <Button android:id="@+id/btn_query" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:text="查询" /> <TextView android:id="@+id/tv_result" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="查询结果为:" android:textColor="#000" android:textSize="18sp" /> </LinearLayout>
查询www.webxml.com.cn网址上服务的访问方式:
选择我们调用的方法:
我们可以在网页上试试这个方法的调用,输入我们想要查询的手机号码,userID--为空,点击调用即可查询出来。
在下面会有soap1.1和soap1.2的请求响应实例。
我们只需要把请求的xml文件发送既可返回一个响应的xml文件。
所以我们就可以通过发送http请求,解析http响应就可以获得我们想要的功能。
在src目录下创建一个xml文件,我这里命名为queryPhone.xml
文件内容就是上面图片中的内容
<?xml version="1.0" encoding="utf-8"?> <soap12:Envelope xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:soap12="http://www.w3.org/2003/05/soap-envelope"> <soap12:Body> <getMobileCodeInfo xmlns="http://WebXml.com.cn/"> <mobileCode>phoneNumber</mobileCode><!--特别注意这里,这里是这个方法需要的参数,我们先用一个值占位,我们会在代码中动态的修改这个canshu--> <userID></userID><!--免费用户为空--> </getMobileCodeInfo> </soap12:Body> </soap12:Envelope>
下面我们开始写查询手机号码归属地的业务逻辑类(QueryPhone.java)
package com.example.webservice.service;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import android.util.Log;
import com.example.webservice.Utils.WebServiceUtil;
/**
* 用于查询手机号码归属地的业务逻辑类
*
* @author info
*
*/
public class QueryPhone {
private static String address;
private static String tag = "QueryPhone";
public static String queryAddressByPhone(String phone) {
// 这是我们访问网络的url地址-就是webxml网站上提供的方法那个网页的地址的前半部分
String path = "http://ws.webxml.com.cn/WebServices/MobileCodeWS.asmx";
// 读取本地的xml文件queryPhone.xml
InputStream is = QueryPhone.class.getClassLoader().getResourceAsStream(
"queryPhone.xml");
// 调用工具类中的方法,把流转换为String
String soap = WebServiceUtil.stream2String(is);
// 替换我们调用方法的参数
soap = soap.replaceAll("phoneNumber", phone);
// 把需要传输的数据转换为字节数组
byte[] datas = soap.getBytes();
// Log.i(tag ,"本地文件"+soap);
try {
// 获取连接
HttpURLConnection conn = (HttpURLConnection) new URL(path)
.openConnection();
// 设置超时时间
conn.setConnectTimeout(4000);
// 设置请求方式
conn.setRequestMethod("POST");
// 允许发送数据
conn.setDoOutput(true);
// 设置请求头信息--不是固定的,有wenxml网站提供的
conn.setRequestProperty("Content-Type", "text/xml; charset=utf-8");
conn.setRequestProperty("Content-Length",
String.valueOf(datas.length));
// 发送数据
conn.getOutputStream().write(datas);
Log.i(tag, "==响应码======" + conn.getResponseCode());
// 获取响应码
if (conn.getResponseCode() == 200) {
// 访问网络成功--获取返回的流数据
InputStream resultIs = conn.getInputStream();
// 我们知道这个流数据里面就是一个xml文件,我们解析xml文档
address = WebServiceUtil.parseXml(resultIs);
Log.i(tag, "结果是:" + address);
}
} catch (Exception exception) {
exception.printStackTrace();
}
return address;
}
}
我们使用到的工具类
package com.example.webservice.Utils;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import org.xmlpull.v1.XmlPullParser;
import android.util.Xml;
public class WebServiceUtil {
/**
* 把流转换为String
*
* @param is
* 需要转换的流
* @return 返回转化后的数据 返回null则代表是有异常
*
*/
public static String stream2String(InputStream is) {
String result = null;
ByteArrayOutputStream baos = null;
try {
baos = new ByteArrayOutputStream();
// 转化流
byte[] buffer = new byte[1024];
int len = -1;
while ((len = is.read(buffer)) != -1) {
baos.write(buffer, 0, len);
}
// 转换为字符串
result = baos.toString();
} catch (Exception e) {
e.printStackTrace();
} finally {
// 关闭流
if (is != null) {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
} finally {
is = null;
}
}
if (baos != null) {
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
} finally {
baos = null;
}
}
}
return result;
}
/**
* 解析返回的xml文件
*
* @param resultIs
* 需要解析的流数据
* @return 返回解析后的结果
*/
public static String parseXml(InputStream resultIs) {
XmlPullParser parser = Xml.newPullParser();
try {
parser.setInput(resultIs, "utf-8");
int type = parser.getEventType();
while (type != XmlPullParser.END_DOCUMENT) {
/*
* <?xml version="1.0" encoding="utf-8"?> <soap12:Envelope
* xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
* xmlns:xsd="http://www.w3.org/2001/XMLSchema"
* xmlns:soap12="http://www.w3.org/2003/05/soap-envelope">
* <soap12:Body> <getMobileCodeInfoResponse
* xmlns="http://WebXml.com.cn/">
* <getMobileCodeInfoResult>string</getMobileCodeInfoResult>
* </getMobileCodeInfoResponse> </soap12:Body>
* </soap12:Envelope>
*/
// 我们根据返回回来的xml文件的格式,获取我们需要的节点内容
switch (type) {
case XmlPullParser.START_TAG:
if (parser.getName().equals("getMobileCodeInfoResult")) {
return parser.nextText();
}
break;
}
type = parser.next();
}
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
}
我们在MainActivity中进行查询即可:
package com.example.webservice;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import com.example.webservice.service.QueryPhone;
public class MainActivity extends Activity {
private EditText et_phone;
private Button btn_query;
private TextView tv_result;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 找到控件
et_phone = (EditText) findViewById(R.id.et_phone);
btn_query = (Button) findViewById(R.id.btn_query);
tv_result = (TextView) findViewById(R.id.tv_result);
// 设置按钮的点击事件
btn_query.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
// 获取手机号码
final String phone = et_phone.getText().toString().trim();
// 开启子线程查询手机归属地
new Thread() {
public void run() {
final String address = QueryPhone.queryAddressByPhone(phone);
// 不能在子线程中修改UI
runOnUiThread(new Runnable() {
public void run() {
tv_result.setText(address);
}
});
};
}.start();
}
});
}
}
不要忘记了增加联网的权限:
<uses-permission android:name="android.permission.INTERNET"/>
这样我们就可以查询手机号码的归属地了。
当然输入的手机号码必须大于7位数字。
完成后的效果图片:
当然我们也可以用这样的方式访问其他的webService---天气预报,验证码等等。
写的第一篇博客,欢迎批评。
相关文章推荐
- Android使用HttpClient以Post、Get请求服务器发送数据的方式(普通和json)
- 使用.NET向webService传double、int、DateTime类型数据, 在发送包的XML中没有提交数据到 服务器
- Android使用HttpClient以Post、Get请求服务器发送数据的方式(普通和json)
- android 解析服务器数据使用json还是xml方式
- Android之通过向WebService服务器发送XML数据获取相关服务
- Android使用HttpClient以Post、Get请求服务器发送数据的方式(普通和json)
- Android--通过Http协议向web服务器发送XML数据和调用webService
- Android:网络:发送xml数据和调用webservice
- Android 向服务器发送XML数据及调用webservice
- android 解析服务器数据使用json还是xml方式
- Android开发使用GET方式向服务器请求和发送数据
- 使用HttpClient通过POST方式发送XML,使用TCP/IP Monitor观察数据
- Android--通过Http协议向web服务器发送XML数据和调用webService
- Android之通过向WebService服务器发送XML数据获取相关服务
- Android之通过向WebService服务器发送XML数据获取相关服务
- Android之通过向WebService服务器发送XML数据获取相关服务
- Android开发入门之发送XML数据和调用webservice
- Android开发使用POST方式向服务器请求和发送数据
- Android使用HttpClient以Post、Get请求服务器发送数据的方式(普通和json)
- Android 网络发送xml数据和调用webservice(传智播客)