【Android自定义View实战】之自定义项目通用的标题栏CustomTitleBar
2016-10-29 09:42
597 查看
转载请注明出处:http://blog.csdn.net/linglongxin24/article/details/52948152 【DylanAndroid的csdn博客】
在Android开发中,一般来说项目中都会用到一个通用风格的标题栏,比如说左边返回按钮,中间显示标题,最后边可能会有一个保存按钮。如下图

那么我们是不是每次在新建一个布局的时候都要去用一个线性布局去加载三个控件,特别麻烦。我们自定义之后,一个控件就好了,下面就来看一下如何来打造通用的自定义标题栏。
在Activity中的用法
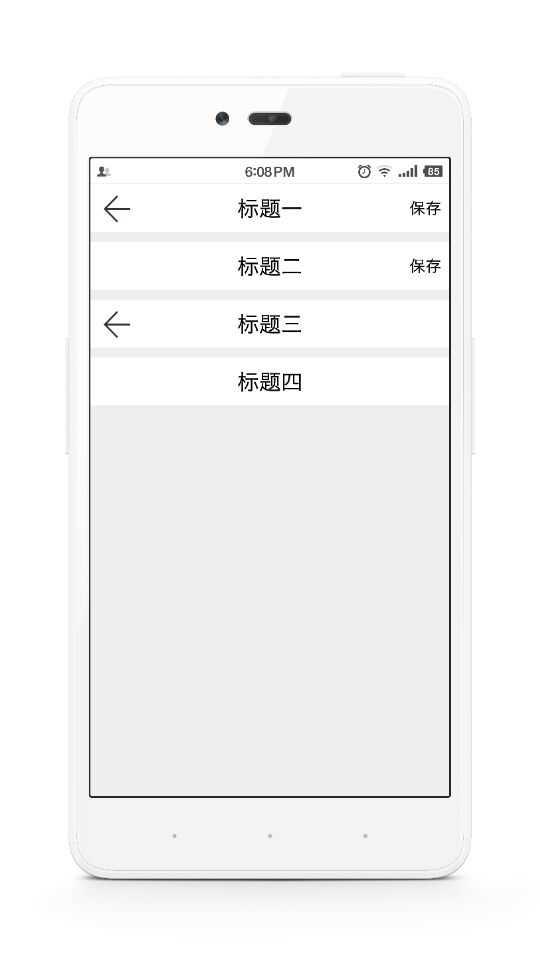
在Android开发中,一般来说项目中都会用到一个通用风格的标题栏,比如说左边返回按钮,中间显示标题,最后边可能会有一个保存按钮。如下图
那么我们是不是每次在新建一个布局的时候都要去用一个线性布局去加载三个控件,特别麻烦。我们自定义之后,一个控件就好了,下面就来看一下如何来打造通用的自定义标题栏。
1.首先在layout文件夹下定义一个pub_titlebar.xml文件
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/ll" android:layout_width="match_parent" android:layout_height="48dp" android:background="#654454" android:orientation="horizontal"> <TextView android:id="@+id/left_button" android:layout_width="48dp" android:layout_height="match_parent" android:textColor="#FFFFFF" android:background="@mipmap/arrow_left" /> <TextView android:id="@+id/title" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_weight="1" android:gravity="center" android:textColor="#000000" android:text="标题" android:textSize="20sp" /> <TextView android:id="@+id/right_button" android:layout_width="48dp" android:layout_height="match_parent" android:background="@null" android:gravity="center" android:textColor="#000000" android:text="保存" android:textSize="16sp" /> </LinearLayout>
2.在res的values文件夹下新建一个attrs.xml
这些可以设置的变量可以在布局文件中动态去设置,特别方便<?xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name="CustomTitleBar"> <attr name="title_background" format="color"/> <attr name="left_button_image" format="reference|integer"/> <attr name="left_button_text" format="string"/> <attr name="left_button_textColor" format="color"/> <attr name="left_button_textSize" format="dimension"/> <attr name="show_left_button" format="boolean"/> <attr name="title_text" format="string"/> <attr name="title_textColor" format="color"/> <attr name="title_textSize" format="dimension"/> <attr name="right_button_image" format="reference|integer"/> <attr name="right_button_text" format="string"/> <attr name="right_button_textColor" format="color"/> <attr name="right_button_textSize" format="dimension"/> <attr name="show_right_button" format="boolean"/> </declare-styleable> </resources>
3.自定义CustomTitleBar继承自LinearLayout
import android.content.Context; import android.content.res.TypedArray; import android.graphics.Color; import android.text.TextUtils; import android.util.AttributeSet; import android.view.LayoutInflater; import android.view.View; import android.widget.LinearLayout; import android.widget.TextView; /** * Android自定义标题栏 * Created by yuandl on 2016-10-27. */ public class CustomTitleBar extends LinearLayout { /** * 标题栏的根布局 */ private LinearLayout ll; /** * 标题栏的左边按返回按钮 */ private TextView left_button; /** * 标题栏的右边按保存按钮 */ private TextView right_button; /** * 标题栏的中间的文字 */ private TextView title; /** * 标题栏的背景颜色 */ private int title_background_color; /** * 标题栏的显示的标题文字 */ private String title_text; /** * 标题栏的显示的标题文字颜色 */ private int title_textColor; /** * 标题栏的显示的标题文字大小 */ private int title_textSize; /** * 返回按钮的资源图片 */ private int left_button_imageId; /** * 返回按钮上显示的文字 */ private String left_button_text; /** * 返回按钮上显示的文字颜色 */ private int left_button_textColor; /** * 返回按钮上显示的文字大小 */ private int left_button_textSize; /** * 是否显示返回按钮 */ private boolean show_left_button; /** * 右边保存按钮的资源图片 */ private int right_button_imageId; /** * 右边保存按钮的文字 */ private String right_button_text; /** * 右边保存按钮的文字颜色 */ private int right_button_textColor; /** * 右边保存按钮的文字大小 */ private int right_button_textSize; /** * 是否显示右边保存按钮 */ private boolean show_right_button; /** * 标题的点击事件 */ private TitleOnClickListener titleOnClickListener; public CustomTitleBar(Context context, AttributeSet attrs) { super(context, attrs); /**加载布局文件*/ LayoutInflater.from(context).inflate(R.layout.pub_titlebar, this, true); ll = (LinearLayout) findViewById(R.id.ll); left_button = (TextView) findViewById(R.id.left_button); right_button = (TextView) findViewById(R.id.right_button); title = (TextView) findViewById(R.id.title); /**获取属性值*/ TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.CustomTitleBar); /**标题相关*/ title_background_color = typedArray.getColor(R.styleable.CustomTitleBar_title_background, Color.WHITE); title_text = typedArray.getString(R.styleable.CustomTitleBar_title_text); title_textColor = typedArray.getColor(R.styleable.CustomTitleBar_title_textColor, Color.BLACK); title_textSize = typedArray.getColor(R.styleable.CustomTitleBar_title_textSize, 22); /**返回按钮相关*/ left_button_imageId = typedArray.getResourceId(R.styleable.CustomTitleBar_left_button_image, R.mipmap.arrow_left); left_button_text = typedArray.getString(R.styleable.CustomTitleBar_left_button_text); left_button_textColor = typedArray.getColor(R.styleable.CustomTitleBar_left_button_textColor, Color.WHITE); left_button_textSize = typedArray.getColor(R.styleable.CustomTitleBar_left_button_textSize, 20); show_left_button = typedArray.getBoolean(R.styleable.CustomTitleBar_show_left_button, true); /**右边保存按钮相关*/ right_button_imageId = typedArray.getResourceId(R.styleable.CustomTitleBar_right_button_image, 0); right_button_text = typedArray.getString(R.styleable.CustomTitleBar_right_button_text); right_button_textColor = typedArray.getColor(R.styleable.CustomTitleBar_right_button_textColor, Color.WHITE); right_button_textSize = typedArray.getColor(R.styleable.CustomTitleBar_right_button_textSize, 20); show_right_button = typedArray.getBoolean(R.styleable.CustomTitleBar_show_right_button, true); /**设置值*/ setTitle_background_color(title_background_color); setTitle_text(title_text); setTitle_textSize(title_textSize); setTitle_textColor(title_textColor); setShow_left_button(show_left_button); setShow_right_button(show_right_button); if (!TextUtils.isEmpty(left_button_text)) {//返回按钮显示为文字 setLeft_button_text(left_button_text); setLeft_button_textColor(left_button_textColor); setLeft_button_textSize(left_button_textSize); } else { setLeft_button_imageId(left_button_imageId); } if (!TextUtils.isEmpty(right_button_text)) {//返回按钮显示为文字 setRight_button_text(right_button_text); setRight_button_textColor(right_button_textColor); setRight_button_textSize(right_button_textSize); } else { setRight_button_imageId(right_button_imageId); } left_button.setOnClickListener(new OnClickListener() { @Override public void onClick(View v) { if (titleOnClickListener != null) { titleOnClickListener.onLeftClick(); } } }); right_button.setOnClickListener(new OnClickListener() { @Override public void onClick(View v) { if (titleOnClickListener != null) { titleOnClickListener.onRightClick(); } } }); } /** * 获取左边的返回按钮 * * @return Button */ public TextView getLeft_button() { return left_button; } /** * 获取标题栏的跟布局 * * @return LinearLayout */ public LinearLayout getLl() { return ll; } /** * 获取标题栏标题TextView * * @return TextView */ public TextView getTitle() { return title; } /** * 获取右边的保存按钮 * * @return Button */ public TextView getRight_button() { return right_button; } /** * 设置返回按钮的资源图片id * * @param left_button_imageId 资源图片id */ public void setLeft_button_imageId(int left_button_imageId) { left_button.setBackgroundResource(left_button_imageId); } /** * 设置返回按钮的文字 * * @param left_button_text */ public void setLeft_button_text(String left_button_text) { left_button.setText(left_button_text); } /** * 设置返回按钮的文字颜色 * * @param left_button_textColor */ public void setLeft_button_textColor(int left_button_textColor) { left_button.setTextColor(left_button_textColor); } /** * 设置返回按钮的文字大小 * * @param left_button_textSize */ public void setLeft_button_textSize(int left_button_textSize) { left_button.setTextSize(left_button_textSize); } /** * 设置是否显示返回按钮 * * @param show_left_button */ public void setShow_left_button(boolean show_left_button) { left_button.setVisibility(show_left_button ? VISIBLE : INVISIBLE); } /** * 设置右边保存按钮的资源图片 * * @param right_button_imageId */ public void setRight_button_imageId(int right_button_imageId) { right_button.setBackgroundResource(right_button_imageId); } /** * 设置右边的保存按钮的文字 * * @param right_button_text */ public void setRight_button_text(String right_button_text) { right_button.setText(right_button_text); } /** * 设置右边保存按钮的文字颜色 * * @param right_button_textColor */ public void setRight_button_textColor(int right_button_textColor) { right_button.setTextColor(right_button_textColor); } /** * 设置右边保存按钮的文字大小 * * @param right_button_textSize */ public void setRight_button_textSize(int right_button_textSize) { right_button.setTextSize(right_button_textSize); } /** * 设置是显示右边保存按钮 * * @param show_right_button */ public void setShow_right_button(boolean show_right_button) { right_button.setVisibility(show_right_button ? VISIBLE : INVISIBLE); } /** * 设置标题背景的颜色 * * @param title_background_color */ public void setTitle_background_color(int title_background_color) { ll.setBackgroundColor(title_background_color); } /** * 设置标题的文字 * * @param title_text */ public void setTitle_text(String title_text) { title.setText(title_text); } /** * 设置标题的文字颜色 * * @param title_textColor */ public void setTitle_textColor(int title_textColor) { title.setTextColor(title_textColor); } /** * 设置标题的文字大小 * * @param title_textSize */ public void setTitle_textSize(int title_textSize) { title.setTextSize(title_textSize); } /** * 设置标题的点击监听 * * @param titleOnClickListener */ public void setOnTitleClickListener(TitleOnClickListener titleOnClickListener) { this.titleOnClickListener = titleOnClickListener; } /** * 监听标题点击接口 */ public interface TitleOnClickListener { /** * 返回按钮的点击事件 */ void onLeftClick(); /** * 保存按钮的点击事件 */ void onRightClick(); } }
4.用法
在布局文件中的引用<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context="cn.bluemobi.dylan.MainActivity"> <cn.bluemobi.dylan.CustomTitleBar android:id="@+id/ct" android:layout_width="match_parent" android:layout_height="wrap_content" app:title_text="标题一"></cn.bluemobi.dylan.CustomTitleBar> <cn.bluemobi.dylan.CustomTitleBar android:layout_marginTop="10dp" android:layout_width="match_parent" android:layout_height="wrap_content" app:show_left_button="false" app:title_text="标题二"></cn.bluemobi.dylan.CustomTitleBar> <cn.bluemobi.dylan.CustomTitleBar android:layout_marginTop="10dp" android:layout_width="match_parent" android:layout_height="wrap_content" app:show_right_button="false" app:title_text="标题三"></cn.bluemobi.dylan.CustomTitleBar> <cn.bluemobi.dylan.CustomTitleBar android:layout_marginTop="10dp" android:layout_width="match_parent" android:layout_height="wrap_content" app:show_left_button="false" app:show_right_button="false" app:title_text="标题四"></cn.bluemobi.dylan.CustomTitleBar> </LinearLayout>
在Activity中的用法
import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.widget.Toast; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); CustomTitleBar customTitleBar = (CustomTitleBar) findViewById(R.id.ct); customTitleBar.setOnTitleClickListener(new CustomTitleBar.TitleOnClickListener() { @Override public void onLeftClick() { Toast.makeText(MainActivity.this, "点击了左边的返回按钮", Toast.LENGTH_SHORT).show(); } @Override public void onRightClick() { Toast.makeText(MainActivity.this, "点击了右边的保存按钮", Toast.LENGTH_SHORT).show(); } }); } }
5.效果图
6 GitHub地址
相关文章推荐
- Android自定义View之TitleBar,通用标题栏
- android 自定义View开发实战(一) CustomTitleView
- 【Android自定义View实战】之自定义项目通用的加载等待对话框LoadingDialog
- Android自定义View-TitleBar(标题栏)详细说明
- [Android]自定义View标题栏TitleBar
- Android实战之 自定义GitHub开源项目ViewPagerIndicator
- 【Android Demo】自定义Activity的标题栏(Titlebar)
- android学习——自定义标题栏titlebar
- android 自定义View开发实战(二) CustomCircleView
- Android绘图机制(三)——自定义View的三种实现方式以及实战项目操作
- Android 自定义标题栏 TitleBar
- android 自定义titlebar标题栏冲突问题
- Android 自定义View、ViewGroup 实战训练之CustomFrameDialog
- Android 自定义标题栏Title Bar
- Android 自定义Activity的标题栏(Titlebar)
- android自定义标题栏时候you cannot combine custom titles with other title
- 自定义Android标题栏TitleBar
- Android 标题栏(TItle Bar)自定义 美化
- 转:自定义Android标题栏TitleBar
- 自定义android标题栏TitleBar