python核心编程第5章课后习题
2016-10-18 22:41
489 查看
5-5:
我一开始的做法:
结果:
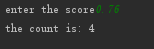
后来想了想这方法太蠢了,根本没必要判断money在哪个区间,不在该区间直接就变0了。
改成:
来源于:https://my.oschina.net/linglingqixianke/blog/671220
5.6
主要熟悉一下str.split()的用法:
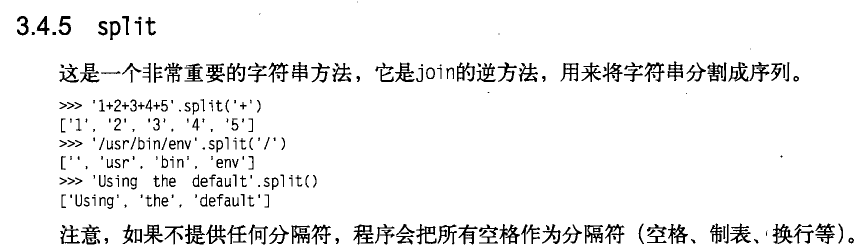

5.12
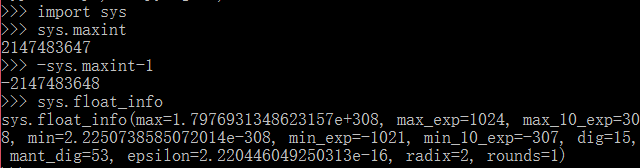
max int 是sys.maxint获得
min int 是-sys.maxint-1
float的信息通过sys.float_info获得
对于long,最小即为sys.maxint+1
最大取决于虚拟机内存大小(但是怎么获得目前还不知道)
对于虚数:
所以取决于float的大小
5-15
5-17
我一开始的做法:
# -*- coding: utf-8 -*- def count(money): money=money*100 #因为输入的是美金,所以要转换成美分 count=0 while money>0: if money>=25: count=count+money//25 money=money%25 elif 10<=money: count=count+money//10 money=money%10 elif 5<=money: count=count+money//5 money=money%5 elif 1<=money: count=count+money money=0 return count if __name__=='__main__': money=raw_input('enter the score') print 'the count is:',int( count(float(money)) )
结果:
后来想了想这方法太蠢了,根本没必要判断money在哪个区间,不在该区间直接就变0了。
改成:
# -*- coding: utf-8 -*- def count(money): money=money*100 #因为输入的是美金,所以要转换成美分 count=0 meiFen=[25,10,5,1] for i in meiFen: (shang,yuShu)=divmod(money,i) count=count+shang money=yuShu return count if __name__=='__main__': money=raw_input('enter the score') print 'the count is:',int( count(float(money)) )
来源于:https://my.oschina.net/linglingqixianke/blog/671220
5.6
主要熟悉一下str.split()的用法:
# -*- coding: utf-8 -*- def caculate(inputStr): (num1,sign,num2)=inputStr.split(' ')#中间是空格 num1=float(num1) num2=float(num2) if sign=='+': return num1+num2 elif sign=='-': return num1-num2 elif sign=='*': return num1-num2 elif sign=='/': return num1/num2 if __name__=='__main__': inputStr=raw_input('enter the inputString:') print 'the result is:',caculate(inputStr)
5.12
max int 是sys.maxint获得
min int 是-sys.maxint-1
float的信息通过sys.float_info获得
对于long,最小即为sys.maxint+1
最大取决于虚拟机内存大小(但是怎么获得目前还不知道)
对于虚数:
>>> type(1j) <type 'complex'> >>> type(1j.real) <type 'float'> >>> type(1j.imag) <type 'float'
所以取决于float的大小
5-15
# -*- coding: utf-8 -*- '''最大公约数采用辗转相除法,最小公倍数 = 两数乘积/最大公约数 辗转相除法介绍:: 例如,求(319,377): ∵ 319÷377=0(余319) ∴(319,377)=(377,319); ∵ 377÷319=1(余58) ∴(377,319)=(319,58); ∵ 319÷58=5(余29), ∴ (319,58)=(58,29); ∵ 58÷29=2(余0), ∴ (58,29)= 29; ∴ (319,377)=29.''' def gcd(a,b):#最大公约数 if a>=b: (bigNum,smallNum)=(a,b) else: (smallNum,bigNum)=(a,b) while bigNum%smallNum!=0: temp=bigNum%smallNum bigNum=smallNum smallNum=temp return smallNum def lcm(a,b): return a*b/gcd(a,b) print gcd(319,377) print lcm(319,377)
5-17
# -*- coding: utf-8 -*- import random as rd randNumList=[] for i in range(100): randNumList.append( rd.randint(0,2**31-1) ) newList=[] N=int( raw_input('enter the len of List:') ) for i in range(N): newList.append( randNumList[ rd.randint(0,100-1)] ) print newList print sorted(newList)
相关文章推荐
- 《Python核心编程》第5章 习题
- Python核心编程(第三版)课后习题解答——第二章
- java基础学习(6)疯狂java讲义第5章课后习题解答源码
- 计算机网络(第3版)_第5章课后习题_三(个人)
- 《Python核心编程》第二版课后习题——第四章(记录自己做的习题,可能有误)
- python核心编程(第二版) 课后习题
- python核心编程课后习题-正则式2
- python核心编程(第二版) 课后习题
- python核心编程课后习题-正则式1
- Python核心编程-第四章课后习题
- 《Python核心编程》 第五章 数字 - 课后习题
- 《Python核心编程》第二版课后习题——第二章 (记录自己做的习题,可能有误)
- Python核心编程(第三版)课后习题解答——第四章
- 《Python核心编程》 第六章 序列 - 课后习题
- 【廖雪峰python3.0】-课后习题:第5章:高级特性
- 《Python核心编程》第二版课后习题——第五章 (记录自己做的习题,可能有误)
- 计算机网络第4章及第5章课后习题答案
- Python核心编程-第二章课后习题
- python核心编程第三版课后习题一
- python核心编程第5章课后题答案