POJ1577 二叉树的创建及先序遍历(给定一些二叉树中的值,大于根的排在右边,小于根的排在左边)
2016-10-18 19:26
363 查看
Description
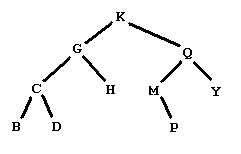
Figure 1
Figure 1 shows a graphical representation of a binary tree of letters. People familiar with binary trees can skip over the definitions of a binary tree of letters, leaves of a binary tree, and a binary search tree of letters, and go right to The problem.
A binary tree of letters may be one of two things:
It may be empty.
It may have a root node. A node has a letter as data and refers to a left and a right subtree. The left and right subtrees are also binary trees of letters.
In the graphical representation of a binary tree of letters:
Empty trees are omitted completely.
Each node is indicated by
Its letter data,
A line segment down to the left to the left subtree, if the left subtree is nonempty,
A line segment down to the right to the right subtree, if the right subtree is nonempty.
A leaf in a binary tree is a node whose subtrees are both empty. In the example in Figure 1, this would be the five nodes with data B, D, H, P, and Y.
The preorder traversal of a tree of letters satisfies the defining properties:
If the tree is empty, then the preorder traversal is empty.
If the tree is not empty, then the preorder traversal consists of the following, in order
The data from the root node,
The preorder traversal of the root's left subtree,
The preorder traversal of the root's right subtree.
The preorder traversal of the tree in Figure 1 is KGCBDHQMPY.
A tree like the one in Figure 1 is also a binary search tree of letters. A binary search tree of letters is a binary tree of letters in which each node satisfies:
The root's data comes later in the alphabet than all the data in the nodes in the left subtree.
The root's data comes earlier in the alphabet than all the data in the nodes in the right subtree.
The problem:
Consider the following sequence of operations on a binary search tree of letters
Remove the leaves and list the data removed
Repeat this procedure until the tree is empty
Starting from the tree below on the left, we produce the sequence of trees shown, and then the empty tree
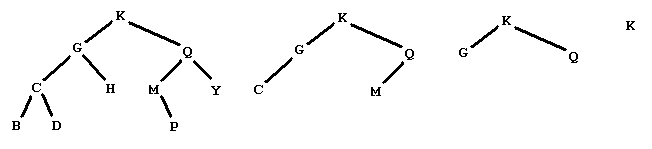
by removing the leaves with data
BDHPY
CM
GQ
K
Your problem is to start with such a sequence of lines of leaves from a binary search tree of letters and output the preorder traversal of the tree.
Input
The input will contain one or more data sets. Each data set is a sequence of one or more lines of capital letters.
The lines contain the leaves removed from a binary search tree in the stages described above. The letters on a line will be listed in increasing alphabetical order. Data sets are separated by a line containing only an asterisk ('*').
The last data set is followed by a line containing only a dollar sign ('$'). There are no blanks or empty lines in the input.
Output
For each input data set, there is a unique binary search tree that would produce the sequence of leaves. The output is a line containing only the preorder traversal of that tree, with no blanks.
Sample Input
#include<iostream>
#include<stdio.h>
using namespace std;
struct Node //定义一个结点
{
char ch; //结点的值
Node *left; //结点的左子树
Node *right; //结点右子树
};
Node node[50000]; //结点的数组对象
char str[100][1000]={0}; //用来存储字符串的数组
int t; //设定一个变量,用来进行比较时改变元素
void insert(Node *root) //对跟进行比较,以及新的左右子树的遍历
{
if (node[t].ch<root->ch)
{
if (root->left == NULL)
root->left = &node[t];
else
insert(root->left);
}
if (node[t].ch>root->ch)
{
if (root->right == NULL)
root->right = &node[t];
else
insert(root->right);
}
}
void tre(Node *root) //用来输出结点的值
{
if (root)
{
printf("%c", root->ch);
//cout << root->ch;
tre(root->left);
tre(root->right);
}
}
int main()
{
int i, j = 0, k, p;
bool flag;
while (1)
{
flag = false;
for (i = 0; i<100; i++)
{
scanf("%c", &str[i]);
cin >> str[i]; //输入字符
if (str[i][0] == '*' || str[i][0] == '$') //判断是否已经达到输出的标准了
{
if (str[i][0] == '$')
flag = true;
p = 1;
i--;
node[0].ch = str[i][0]; //对 每个结点进行初始化
node[0].left = node[0].right = NULL;
i--;
while (i >= 0) //判断该节点的位置是否存在
{
k = 0;
while (str[i][k]) //给某一结点的值
{
node[p].ch = str[i][k];
node[p].left = node[p].right = NULL;
t = p;
insert(&node[0]); //把结点插入二叉树中
k++;
p++; //用来比较时控制元素的变化
}
i--;
}
tre(&node[0]); //打印出结点
printf("\n");
}
//
if (flag)
//
return 0;
}
}
return 0;
}
---------------------------------------上面的方法提交时超时 ,下面的方法我还没看懂,可以注释的大神请留言或私信我/*
题意:定义一个由字母构成的二叉树。对于二叉树内任一个结点,左子树的所有字母的字母序均在该结点字母之前,右子树的所有字母的字母序均在该结点字母之后。
现从二叉树的各叶子开始,拆除二叉树内无左右子结点的结点,最终使得该二叉树为空。现对一字母二叉树进行拆除操作,给定每一步拆除字母,问原二叉树的先序遍历序列。
思路:可根据每步拆除的字母,反过来还原该二叉树。也就是,从后往前扫描每一步拆除的字母,重新构建字母二叉树。最后一步肯定是根。
而对于其他步,遂于每一个字母,对二叉树进行有序的插入操作。将树结构还原。
每一步的每一个字母,进行位置的查找,找出该字母对应的父节点。然后在作为叶子,加至父节点的左子或者右子。
*/
#include <iostream>
#include <string>
#include <fstream>
#include <deque>
using namespace std;
template<typename T>
struct Node
{
T value;
Node<T>* lChild;
Node<T>* rChild;
Node<T>* parent;
};
template<typename T>
class FallingLeaves
{
protected:
Node<T>* root;
int count;
void Distroy(Node<T>* r);
void Insert(Node<T>*& r, T value);
void Output(Node<T>* r) const;
public:
FallingLeaves();
~FallingLeaves();
void Insert(T value);
void Output() const;
};
template<typename T>
FallingLeaves<T>::FallingLeaves()
{
root = NULL;
count = 0;
}
template<typename T>
FallingLeaves<T>::~FallingLeaves()
{
Distroy(root);
}
template<typename T>
void FallingLeaves<T>::Distroy(Node<T>* r)
{
if (!r)
{
return;
}
Distroy(r->lChild);
Distroy(r->rChild);
delete r;
}
template<typename T>
void FallingLeaves<T>::Insert(Node<T>*& r, T value)
{
if (!r)
{
r = new Node<T>();
r->value = value;
r->parent = r;
r->lChild = NULL;
r->rChild = NULL;
count++;
return;
}
if (value < r->value)
{
Insert(r->lChild, value);
}
else
{
Insert(r->rChild, value);
}
}
template<typename T>
void FallingLeaves<T>::Insert(T value)
{
Insert(root, value);
}
template<typename T>
void FallingLeaves<T>::Output(Node<T>* r) const
{
if (!r)
{
return;
}
cout << r->value;
Output(r->lChild);
Output(r->rChild);
}
template<typename T>
void FallingLeaves<T>::Output() const
{
Output(root);
cout << "\n";
}
int main()
{
string s;
deque<string> dq; //双端队列
while (cin >> s)
{
if (s == "*" || s == "$")
{
FallingLeaves<char> obj;
for (unsigned int i = 0; i < dq.size(); i++)
{
for (string::reverse_iterator iter = dq[i].rbegin(); iter != dq[i].rend(); iter++)
{
obj.Insert(*iter);
}
}
obj.Output();
dq.clear();
if (s == "$")
{
break;
}
}
else
{
dq.push_front(s);
}
}
return 0;
}
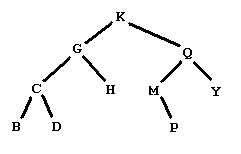
Figure 1
Figure 1 shows a graphical representation of a binary tree of letters. People familiar with binary trees can skip over the definitions of a binary tree of letters, leaves of a binary tree, and a binary search tree of letters, and go right to The problem.
A binary tree of letters may be one of two things:
It may be empty.
It may have a root node. A node has a letter as data and refers to a left and a right subtree. The left and right subtrees are also binary trees of letters.
In the graphical representation of a binary tree of letters:
Empty trees are omitted completely.
Each node is indicated by
Its letter data,
A line segment down to the left to the left subtree, if the left subtree is nonempty,
A line segment down to the right to the right subtree, if the right subtree is nonempty.
A leaf in a binary tree is a node whose subtrees are both empty. In the example in Figure 1, this would be the five nodes with data B, D, H, P, and Y.
The preorder traversal of a tree of letters satisfies the defining properties:
If the tree is empty, then the preorder traversal is empty.
If the tree is not empty, then the preorder traversal consists of the following, in order
The data from the root node,
The preorder traversal of the root's left subtree,
The preorder traversal of the root's right subtree.
The preorder traversal of the tree in Figure 1 is KGCBDHQMPY.
A tree like the one in Figure 1 is also a binary search tree of letters. A binary search tree of letters is a binary tree of letters in which each node satisfies:
The root's data comes later in the alphabet than all the data in the nodes in the left subtree.
The root's data comes earlier in the alphabet than all the data in the nodes in the right subtree.
The problem:
Consider the following sequence of operations on a binary search tree of letters
Remove the leaves and list the data removed
Repeat this procedure until the tree is empty
Starting from the tree below on the left, we produce the sequence of trees shown, and then the empty tree
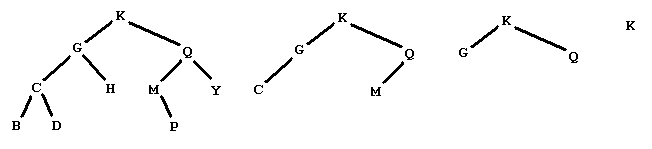
by removing the leaves with data
BDHPY
CM
GQ
K
Your problem is to start with such a sequence of lines of leaves from a binary search tree of letters and output the preorder traversal of the tree.
Input
The input will contain one or more data sets. Each data set is a sequence of one or more lines of capital letters.
The lines contain the leaves removed from a binary search tree in the stages described above. The letters on a line will be listed in increasing alphabetical order. Data sets are separated by a line containing only an asterisk ('*').
The last data set is followed by a line containing only a dollar sign ('$'). There are no blanks or empty lines in the input.
Output
For each input data set, there is a unique binary search tree that would produce the sequence of leaves. The output is a line containing only the preorder traversal of that tree, with no blanks.
Sample Input
BDHPY CM GQ K * AC B $
Sample Output
KGCBDHQMPY BAC
---------------------------------------
#include<iostream>
#include<stdio.h>
using namespace std;
struct Node //定义一个结点
{
char ch; //结点的值
Node *left; //结点的左子树
Node *right; //结点右子树
};
Node node[50000]; //结点的数组对象
char str[100][1000]={0}; //用来存储字符串的数组
int t; //设定一个变量,用来进行比较时改变元素
void insert(Node *root) //对跟进行比较,以及新的左右子树的遍历
{
if (node[t].ch<root->ch)
{
if (root->left == NULL)
root->left = &node[t];
else
insert(root->left);
}
if (node[t].ch>root->ch)
{
if (root->right == NULL)
root->right = &node[t];
else
insert(root->right);
}
}
void tre(Node *root) //用来输出结点的值
{
if (root)
{
printf("%c", root->ch);
//cout << root->ch;
tre(root->left);
tre(root->right);
}
}
int main()
{
int i, j = 0, k, p;
bool flag;
while (1)
{
flag = false;
for (i = 0; i<100; i++)
{
scanf("%c", &str[i]);
cin >> str[i]; //输入字符
if (str[i][0] == '*' || str[i][0] == '$') //判断是否已经达到输出的标准了
{
if (str[i][0] == '$')
flag = true;
p = 1;
i--;
node[0].ch = str[i][0]; //对 每个结点进行初始化
node[0].left = node[0].right = NULL;
i--;
while (i >= 0) //判断该节点的位置是否存在
{
k = 0;
while (str[i][k]) //给某一结点的值
{
node[p].ch = str[i][k];
node[p].left = node[p].right = NULL;
t = p;
insert(&node[0]); //把结点插入二叉树中
k++;
p++; //用来比较时控制元素的变化
}
i--;
}
tre(&node[0]); //打印出结点
printf("\n");
}
//
if (flag)
//
return 0;
}
}
return 0;
}
---------------------------------------上面的方法提交时超时 ,下面的方法我还没看懂,可以注释的大神请留言或私信我/*
题意:定义一个由字母构成的二叉树。对于二叉树内任一个结点,左子树的所有字母的字母序均在该结点字母之前,右子树的所有字母的字母序均在该结点字母之后。
现从二叉树的各叶子开始,拆除二叉树内无左右子结点的结点,最终使得该二叉树为空。现对一字母二叉树进行拆除操作,给定每一步拆除字母,问原二叉树的先序遍历序列。
思路:可根据每步拆除的字母,反过来还原该二叉树。也就是,从后往前扫描每一步拆除的字母,重新构建字母二叉树。最后一步肯定是根。
而对于其他步,遂于每一个字母,对二叉树进行有序的插入操作。将树结构还原。
每一步的每一个字母,进行位置的查找,找出该字母对应的父节点。然后在作为叶子,加至父节点的左子或者右子。
*/
#include <iostream>
#include <string>
#include <fstream>
#include <deque>
using namespace std;
template<typename T>
struct Node
{
T value;
Node<T>* lChild;
Node<T>* rChild;
Node<T>* parent;
};
template<typename T>
class FallingLeaves
{
protected:
Node<T>* root;
int count;
void Distroy(Node<T>* r);
void Insert(Node<T>*& r, T value);
void Output(Node<T>* r) const;
public:
FallingLeaves();
~FallingLeaves();
void Insert(T value);
void Output() const;
};
template<typename T>
FallingLeaves<T>::FallingLeaves()
{
root = NULL;
count = 0;
}
template<typename T>
FallingLeaves<T>::~FallingLeaves()
{
Distroy(root);
}
template<typename T>
void FallingLeaves<T>::Distroy(Node<T>* r)
{
if (!r)
{
return;
}
Distroy(r->lChild);
Distroy(r->rChild);
delete r;
}
template<typename T>
void FallingLeaves<T>::Insert(Node<T>*& r, T value)
{
if (!r)
{
r = new Node<T>();
r->value = value;
r->parent = r;
r->lChild = NULL;
r->rChild = NULL;
count++;
return;
}
if (value < r->value)
{
Insert(r->lChild, value);
}
else
{
Insert(r->rChild, value);
}
}
template<typename T>
void FallingLeaves<T>::Insert(T value)
{
Insert(root, value);
}
template<typename T>
void FallingLeaves<T>::Output(Node<T>* r) const
{
if (!r)
{
return;
}
cout << r->value;
Output(r->lChild);
Output(r->rChild);
}
template<typename T>
void FallingLeaves<T>::Output() const
{
Output(root);
cout << "\n";
}
int main()
{
string s;
deque<string> dq; //双端队列
while (cin >> s)
{
if (s == "*" || s == "$")
{
FallingLeaves<char> obj;
for (unsigned int i = 0; i < dq.size(); i++)
{
for (string::reverse_iterator iter = dq[i].rbegin(); iter != dq[i].rend(); iter++)
{
obj.Insert(*iter);
}
}
obj.Output();
dq.clear();
if (s == "$")
{
break;
}
}
else
{
dq.push_front(s);
}
}
return 0;
}
相关文章推荐
- 这是一个我面试某公司的算法题目:对一个字符数组进行排序,根据给定的字符,大于它的,放在数组的左边,小于它的,放在数组的右边,且数组中的元素之间的相对位置要保持不变。
- 找出数组中满足其左边的数都小于等于它,右边的数都大于等于它的数
- 81 第1 组百度面试题 数组 左边的数都小于等 于它,右边的数都大于等于它
- 一个int数组,里面数据无任何限制,要求求出所有这样的数a[i],其左边的数都小于等于它,右边的数都大于等于它
- int数组,找小于右边所有数,大于左边所有数的数
- 百度三面:找出数组中所有这样的数,大于等于左边的所有数,小于等于右边的所有的数
- 一个int数组,里面数据无任何限制,要求求出所有这样的数a[i],其左边的数都小于等于它,右边的数都大于等于它。
- 编写一个算法将顺序表A分成两部分,大于0 的在A的左边,小于0的在A的右边
- 面试--求数组,左边的数都小于等于它,右边的数都大于等于它
- 一个int数组,里面数据无任何限制,要求求出所有这样的数a[i],其左边的数都小于等于它,右边的数都大于等于它
- 给定先序:ABCDEFGHIJK 给定中序:CBEDGFAHJIK 首先分析上述给定的先,中序,首先得知先序遍历的肯定是二叉树的根节点:A,在看中序遍历,根据中序遍历的原理可知,在A左边的一定全部属于
- 将一个字符串中小于0的数字放到左边,大于0的数字放到右边
- 一个int 数组,里面数据无任何限制,要求求出所有这样的数a[i],其左边的数都小于等于它,右边的数都大于等于它。能否只用一个额外数组和少量其它空间实现。
- 一个int数组,里面数据无任何限制,要求求出所有这样的数a[i],其左边的数都小于等于它,右边的数都大于等于它
- 一个int 数组,里面数据无任何限制,要求求出所有这样的数a[i],其左边的数都小于等于它,右边的数都大于等于它。能否只用一个额外数组和少量其它空间实现。
- 一个int 数组,里面数据无任何限制,要求求出所有这样的数a[i],其左边的数都小于等于它,右边的数都大于等于它。
- 一个int数组, 比如 array[],里面数据无任何限制,要求求出 所有这样的数array[i],其左边的数都小于等于它,右边的数都大于等于它。能否只用一个额外数组和少量其它空间实现
- 在一个数组中,找出所有这样的数a[i],其左边的数都小于等于它,右边的数都大于等于它
- 据说是淘宝面试题“给定一个数组将大于0的放在最右边,等于0的放在中间,大于0的放在最左边”
- 左边的数都小于等于它,右边的数都大于等于它