JavaScript/Jsp 数据读取和request访问数据库
2016-10-17 20:54
706 查看
完成目标:利用MyEclipse和jQuery完成登录验证和获取所填数据功能。
1.注册页面请求信息获取
登录界面代码:
显示页面代码:
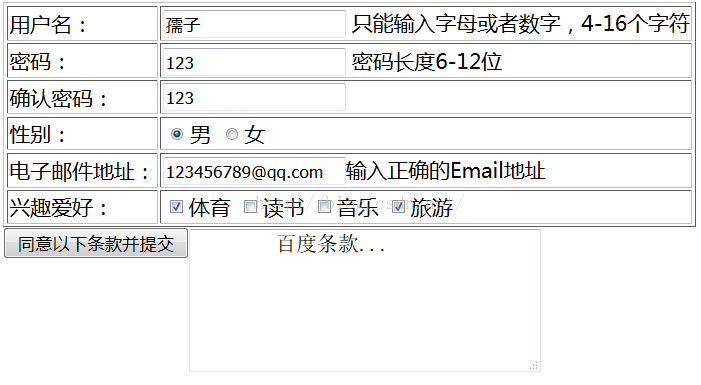
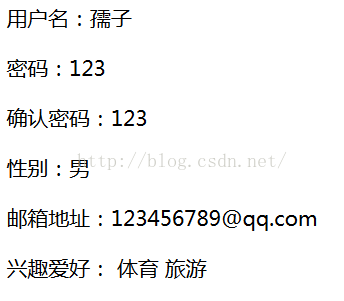
2.与数据库相连,并且验证登录功能。
一个简单的登录界面(主要为了测试功能):
数据库数据:
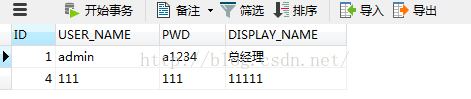
登录界面显示:
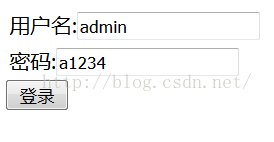
3.将数据动态添加到表格中,并且表格单双行颜色不通,鼠标移动时,点击时颜色发生变化。
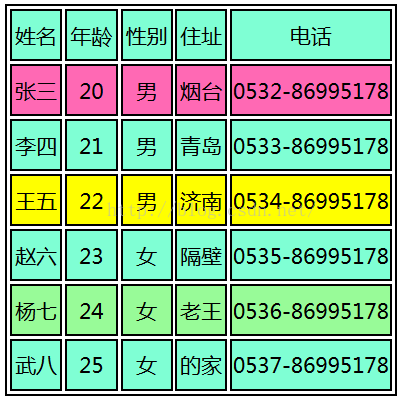
1.注册页面请求信息获取
登录界面代码:
<%@ page language="java" import="java.util.*" pageEncoding="utf-8" contentType="text/html;charset:utf-8"%> <% String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; %> <html> <head> <base href="<%=basePath%>"> <title>百度用户注册</title> <meta charset="utf-8"> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> <script type="text/javascript" src="js/jquery-1.4.2.min.js"></script> <script> function tijiao(){ alert("提交"); document.baseform.submit(); } </script> </head> <body> <form action="showbaidu.jsp" method="post" name="baseform"> <table border="1" > <tr> <td>用户名:</td> <td><input type="text" name="name"> 只能输入字母或者数字,4-16个字符</td> </tr> <tr> <td>密码:</td> <td><input type="text" name="pwd"> 密码长度6-12位</td> </tr> <tr> <td>确认密码:</td> <td><input type="text" name="pwd1"></td> </tr> <tr> <td>性别:</td> <td><input type="radio" name="sex" value="男" checked>男 <input type="radio" name="sex" value="女">女 </td> </tr> <tr> <td>电子邮件地址:</td> <td><input type="text" name="mail">输入正确的Email地址</td> </tr> <tr> <td>兴趣爱好:</td> <td> <input type="checkbox" name="sport" value="体育">体育 <input type="checkbox" name="sport" value="读书">读书 <input type="checkbox" name="sport" value="音乐">音乐 <input type="checkbox" name="sport" value="旅游">旅游 </td> </tr> </table> <input type="button" value="同意以下条款并提交" style="float: left" id="bt" onclick="tijiao()"> <textarea rows="5" cols="30"> 百度条款... </textarea> </form> </body> </html>
显示页面代码:
<%@ page language="java" import="java.util.*" pageEncoding="utf-8" contentType="text/html;charset:utf-8"%> <% request.setCharacterEncoding("utf-8"); String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; String name=request.getParameter("name"); String pwd=request.getParameter("pwd"); String pwd1=request.getParameter("pwd1"); String sex=request.getParameter("sex"); String mail=request.getParameter("mail"); String arr[]=request.getParameterValues("sport"); %> <html> <head> <base href="<%=basePath%>"> <title>百度用户注册信息</title> <meta charset="utf-8"> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> </head> <body> <p> 用户名:<%=name%> </p> <p> 密码:<%=pwd%> </p> <p> 确认密码:<%=pwd1%> </p> <p> 性别:<%=sex%> </p> <p> 邮箱地址:<%=mail%> </p> <p> 兴趣爱好: <% for(int i=0;i<arr.length;i++){ %> <%=arr[i]%> <% } %> </p> </body> </html>效果:
2.与数据库相连,并且验证登录功能。
一个简单的登录界面(主要为了测试功能):
<%@ page language="java" import="java.util.*" pageEncoding="utf-8" contentType="text/html;charset:utf-8"%> <% String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; %> <html> <head> <base href="<%=basePath%>"> <title>登录</title> <meta charset="utf-8"> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> <script> function tijiao(){ document.baseform.submit(); } </script> </head> <body> <form action="shujuku.jsp" method="post" name="baseform"> <table> <tr> <td>用户名:<input type="text" name="uname"></td> </tr> <tr> <td>密码:<input type="text" name="upwd"></td> </tr> </table> <input type="button" id="login" value="登录" onclick="tijiao()"> </form> </body> </html>所转到jsp文件代码(与数据库相连代码):
<%@ page language="java" contentType="text/html;charset=utf-8"%> <%@ page import="java.util.*"%> <%@ page import="java.text.*"%> <%@ page import="java.sql.*" %> <%@ page import="com.mysql.jdbc.Driver" %> <% String flag="0"; String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; //驱动程序名 String driverName = "com.mysql.jdbc.Driver"; //数据库用户名 String userName = "root"; //密码 String userPasswd = "ffffff"; //数据库名 String dbName = "shcoolapp"; //联结字符串 String url = "jdbc:mysql://localhost/" + dbName + "?user="+ userName + "&password=" + userPasswd + "&useUnicode=true&characterEncoding=gbk"; request.setCharacterEncoding("utf-8"); String uName=request.getParameter("uname"); String upwd=request.getParameter("upwd"); try{ Class.forName(driverName); Connection con=DriverManager.getConnection(url); Statement stmt=con.createStatement(); ResultSet rs=stmt.executeQuery("SELECT * FROM users where user_name='"+uName+"' and pwd='"+upwd+"'"); if(rs.next()){ String dName=rs.getString("DISPLAY_NAME"); response.sendRedirect("baidu.jsp"); } /* else{ response.setHeader("REFRESH","4;url=login.jsp"); } */ /* while(rs.next()){ String name=rs.getString("USER_NAME"); String pwd=rs.getString("PWD"); String dname=rs.getString("DISPLAY_NAME"); out.println("用户名:"+name); out.println("密码:"+pwd); out.println("名称:"+dname+"<br>"); */ con.close(); }catch(Exception e){ e.printStackTrace(); } %> <html> <head> <base href="<%=basePath%>"> <meta charset="utf-8"> <title>JSP访问数据库</title> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> <script type="text/javascript" src="js/jquery-1.4.2.min.js"></script> <!-- <link rel="stylesheet" type="text/css" href="styles.css"> --> <script type="text/javascript"> $(function(){ if($('#flag').val()=="0"){ setTimeout(function(){ window.location.href="login.jsp"; /* $("form").attr("action","login.jsp"); b952 $("form").submit(); */ // document.baseForm.action = "login.jsp"; // document.baseForm.submit(); },4000); } }); </script> </head> <body> <form action="" method="post" name="baseform"> <input type="hidden" value='<%=flag%>' id="flag"> 中间等待画面 </form> </body> </html>效果(登录成功,跳转到一个界面。登录失败3秒后跳回到登录界面)
数据库数据:
登录界面显示:
3.将数据动态添加到表格中,并且表格单双行颜色不通,鼠标移动时,点击时颜色发生变化。
<%@ page language="java" pageEncoding="utf-8" contentType="text/html;charset:utf-8"%> <%@ page import="java.util.*"%> <%@ page import="com.jredu.java.*"%> <%@ page import="com.jredu.web.service.*"%> <% String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; List<Student> list=new ArrayList(); StudentService studentservice=new StudentService(); list=studentservice.selectAll(); %> <html> <head> <base href="<%=basePath%>"> <title>学生</title> <meta charset="utf-8"> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <script type="text/javascript" src="js/jquery-1.4.2.min.js"></script> <link rel="stylesheet" href="css/student.css" type="text/css"></link> <script> $(function(){ $("tr:odd").css("background","#98FB98"); $("tr:even").css("background","#7FFFD4"); $("td").attr("align","center"); var trColor ; $("tr").click(function () { $("tr:odd").css("background","#98FB98"); $("tr:even").css("background","#7FFFD4"); $(this).css("background","#FF69B4"); $(this).attr("check","1"); }) $("tr").mouseover(function () { if($(this).attr("check")=="1"){ return; } trColor= $(this).css("background"); $(this).css("background","#FFFF00"); }).mouseout(function () { if($(this).attr("check")=='1'){ return; } $(this).css("background",trColor); }); }); </script> </head> <body> <table class="table"> <tr> <td>姓名</td> <td>年龄</td> <td>性别</td> <td>住址</td> <td>电话</td> </tr> <%for(int i=0;i<list.size();i++){ Student st =new Student(); st=list.get(i);%> <tr> <td><%=st.getName() %></td> <td><%=st.getAge() %></td> <td><%=st.getSexId() %></td> <td><%=st.getAddress() %></td> <td><%=st.getPhone() %></td> </tr> <%} %> </table> </body> </html>src下的java类代码:
package com.jredu.java; /** * @author Administrator * */ public class Student { private String name; private int age; private String sexId; private String address; private String phone; public Student(){}; public Student(String name, int age, String sexId, String address, String phone) { super(); this.name = name; this.age = age; this.sexId = sexId; this.address = address; this.phone = phone; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getSexId() { return sexId; } public void setSexId(String sexId) { this.sexId = sexId; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } public String getPhone() { return phone; } public void setPhone(String phone) { this.phone = phone; } }src下的java代码:
package com.jredu.web.service; import java.util.ArrayList; import java.util.List; import com.jredu.java.Student; public class StudentService { public List<Student> selectAll(){ List<Student> list=new ArrayList<Student>(); Student stu=new Student("张三",20,"男","烟台","0532-86995178"); list.add(stu); stu=new Student("李四",21,"男","青岛","0533-86995178"); list.add(stu); stu=new Student("王五",22,"男","济南","0534-86995178"); list.add(stu); stu=new Student("赵六",23,"女","隔壁","0535-86995178"); list.add(stu); stu=new Student("杨七",24,"女","老王","0536-86995178"); list.add(stu); stu=new Student("武八",25,"女","的家","0537-86995178"); list.add(stu); return list; } }效果:(表格本身颜色为浅绿和深绿两种颜色,点击时变紫色,鼠标移动到上面时变黄色)
相关文章推荐
- 访问网站时只是显示主页(index.jsp),没有请求连接数据库读取数据。
- kendo ui 访问数据库数据,绘制jsp页面
- 读取数据库的数据并整合成3D饼图在jsp中显示详解
- c#数据库访问读取数据速度测试
- Javascript+XMLHttpRequest+asp.net无刷新读取数据库数据
- jsp访问数据库(学生数据表)
- JSP自定义标签实例---从数据库读取数据放在下拉列表中
- Javascript+XMLHttpRequest+asp.net无刷新读取数据库数据
- 实现jsp页面二级下拉框联动,实时读取数据库数据
- 将数据放入到session域中,还是访问的时候一直使用request进行访问数据库
- jsp+java下拉框读取数据库数据
- jsp连接mssql数据库的类及应用其读取数据
- Javascript+XMLHttpRequest+asp.net无刷新读取数据库数据
- Javascript+XMLHttpRequest+asp.net无刷新读取数据库数据
- 请问如何在Web页面中点击一个button之后,用jsp从数据库中读取数据显示到表格里
- java jsp struts2标签 从数据库中读取含有html标签的数据显示问题
- struts2中从数据库中读取数据,并在JSP页面中遍历保存有JavaBean对象的List对象
- Javascript+XMLHttpRequest+asp.net无刷新读取数据库数据
- Jsp读取数据库返回json数据,Android客户端接收json
- jsp 分页(数据库读取数据)