iOS触摸手势——UITouch
2016-10-15 08:36
253 查看
Demo实例
UITouch,主要是重写四个方法(触摸开始、触摸移动、触摸结束、触摸退出)以实现触摸响应方法
1、触摸开始 - (void)touchesBegan:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event { }
2、触摸移动 - (void)touchesMoved:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event { }
3、触摸结束 - (void)touchesEnded:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event { }
4、触摸退出(注意:通常是受到设备其他方法的影响:如来电、电量不足关机等) - (void)touchesCancelled:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event { }
使用注意事项:
1、触摸开始方法中,一般实现的属性有:
1-1、获取触摸对象,如:
[objc] view
plain copy


UITouch *touch = touches.anyObject;
1-2、触摸次数,以便判断实现对应方法,如:
[objc] view
plain copy


NSInteger clickNumber = touch.tapCount;
NSLog(@"click number = %@", @(clickNumber));
1-3、触摸位置是否用户想要处理的子视图,如:
[objc] view
plain copy


CGPoint currentPoint = [touch locationInView:self.view];
if (CGRectContainsPoint(self.label.frame, currentPoint))
{
// 改变标题
self.label.text = @"触摸位置在当前label";
}
2、触摸移动方法中,一般实现的属性有:
2-1、获取触摸对象,如:
UITouch *touch = touches.anyObject;
2-2、获取当前点击位置,以及上一个点击位置,如:
[objc] view
plain copy


CGPoint currentPoint = [touch locationInView:self.view];
CGPoint previousPoint = [touch previousLocationInView:self.view];
注:可通过触摸移动改变子视图的位置,如:
[objc] view
plain copy


float moveX = currentPoint.x - previousPoint.x;
float moveY = currentPoint.y - previousPoint.y;
CGFloat centerX = self.label.center.x + moveX;
CGFloat centerY = self.label.center.y + moveY;
self.label.center = CGPointMake(centerX, centerY);
[objc] view
plain copy


// 触摸开始
- (void)touchesBegan:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event
{
// self.view.backgroundColor = [UIColor colorWithRed:(arc4random() % 255 / 255.0) green:(arc4random() % 255 / 255.0) blue:(arc4random() % 255 / 255.0) alpha:1.0];
NSLog(@"触摸手势 开始");
// 获取触摸对象
UITouch *touch = touches.anyObject;
// 获取触摸次数
NSInteger clickNumber = touch.tapCount;
NSLog(@"click number = %@", @(clickNumber));
if (2 == clickNumber)
{
self.label.backgroundColor = [UIColor redColor];
}
else if (3 == clickNumber)
{
self.label.backgroundColor = [UIColor greenColor];
}
// 获取触摸状态
UITouchPhase state = touch.phase;
NSLog(@"state = %ld", state);
// 获取响应视图对象
UIView *view = touch.view;
NSLog(@"view = %@", view);
// 获取当前触摸位置
CGPoint currentPoint = [touch locationInView:self.view];
NSLog(@"touch.locationInView = {%2.3f, %2.3f}", currentPoint.x, currentPoint.y);
// 获取当前触摸的前一个位置
CGPoint previousPoint = [touch previousLocationInView:self.view];
NSLog(@"touch.previousLocationInView = {%2.3f, %2.3f}", previousPoint.x, previousPoint.y);
// 获取触摸区域的子视图
// 方法1
// for (UIView *oneView in self.view.subviews)
// {
// if ([oneView isKindOfClass:[UILabel class]])
// {
// // 是否选中图标(当前坐标是否包含所选图标)
// if (CGRectContainsPoint(oneView.frame, currentPoint))
// {
// // 获取当前被选择图标
// UILabel *selectedView = (UILabel *)oneView;
// // 改变标题
// selectedView.text = @"触摸位置在当前label";
// }
// }
// }
// 方法2
if (CGRectContainsPoint(self.label.frame, currentPoint))
{
// 改变标题
self.label.text = @"触摸位置在当前label";
}
}
[objc] view
plain copy


// 触摸移动
- (void)touchesMoved:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event
{
// self.view.backgroundColor = [UIColor colorWithRed:(arc4random() % 255 / 255.0) green:(arc4random() % 255 / 255.0) blue:(arc4random() % 255 / 255.0) alpha:1.0];
NSLog(@"触摸手势 移动");
// 移动UI视图控件
// 方法1 获得触摸点的集合,可以判断多点触摸事件
for (UITouch *touch in event.allTouches)
{
// 获取当前触摸坐标
CGPoint currentPoint = [touch locationInView:self.view];
NSLog(@"touch.locationInView = {%2.3f, %2.3f}", currentPoint.x, currentPoint.y);
// 获取当前触摸前一个坐标
CGPoint previousPoint = [touch previousLocationInView:self.view];
NSLog(@"touch.previousLocationInView = {%2.3f, %2.3f}", previousPoint.x, previousPoint.y);
// 坐标偏移量 (效果异常??)
float moveX = currentPoint.x - previousPoint.x;
float moveY = currentPoint.y - previousPoint.y;
CGFloat centerX = self.label.center.x + moveX;
CGFloat centerY = self.label.center.y + moveY;
self.label.center = CGPointMake(centerX, centerY);
}
// 方法2
// UITouch *touch = touches.anyObject;
// // 获取当前触摸坐标
// CGPoint currentPoint = [touch locationInView:self.view];
// NSLog(@"touch.locationInView = {%2.3f, %2.3f}", currentPoint.x, currentPoint.y);
// // 获取当前触摸前一个坐标
// CGPoint previousPoint = [touch previousLocationInView:self.view];
// NSLog(@"touch.previousLocationInView = {%2.3f, %2.3f}", previousPoint.x, previousPoint.y);
// // 坐标偏移量
// float moveX = currentPoint.x - previousPoint.x;
// float moveY = currentPoint.y - previousPoint.y;
// CGFloat centerX = self.label.center.x + moveX;
// CGFloat centerY = self.label.center.y + moveY;
// self.label.center = CGPointMake(centerX, centerY);
}
[objc] view
plain copy


// 触摸结束
- (void)touchesEnded:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event
{
// self.view.backgroundColor = [UIColor whiteColor];
NSLog(@"触摸手势 结束");
self.label.text = @"touch触摸手势";
}
[objc] view
plain copy


// 触摸退出(注意:通常是受到设备其他方法的影响:如来电、电量不足关机等)
- (void)touchesCancelled:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event
{
NSLog(@"触摸手势 退出");
}
效果图
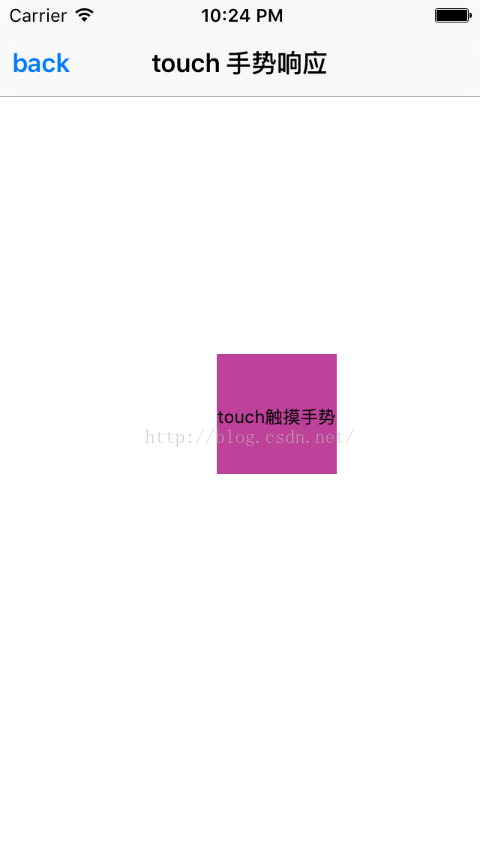
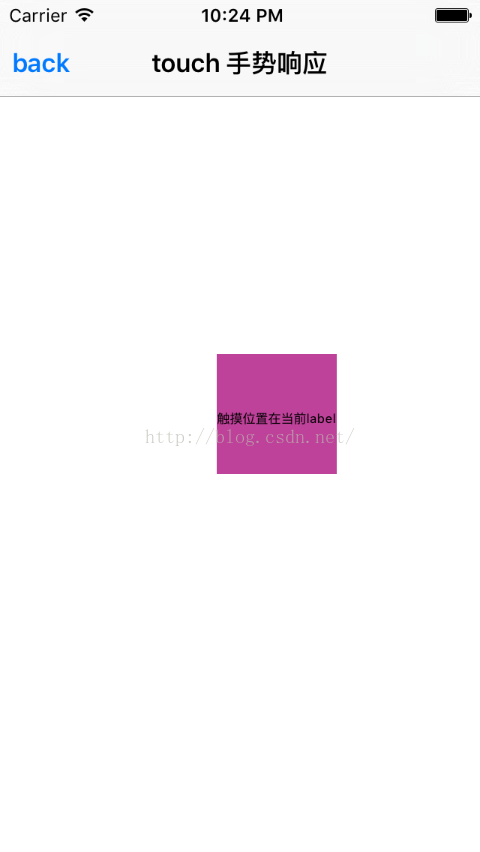
UITouch,主要是重写四个方法(触摸开始、触摸移动、触摸结束、触摸退出)以实现触摸响应方法
1、触摸开始 - (void)touchesBegan:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event { }
2、触摸移动 - (void)touchesMoved:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event { }
3、触摸结束 - (void)touchesEnded:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event { }
4、触摸退出(注意:通常是受到设备其他方法的影响:如来电、电量不足关机等) - (void)touchesCancelled:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event { }
使用注意事项:
1、触摸开始方法中,一般实现的属性有:
1-1、获取触摸对象,如:
[objc] view
plain copy

UITouch *touch = touches.anyObject;
1-2、触摸次数,以便判断实现对应方法,如:
[objc] view
plain copy

NSInteger clickNumber = touch.tapCount;
NSLog(@"click number = %@", @(clickNumber));
1-3、触摸位置是否用户想要处理的子视图,如:
[objc] view
plain copy

CGPoint currentPoint = [touch locationInView:self.view];
if (CGRectContainsPoint(self.label.frame, currentPoint))
{
// 改变标题
self.label.text = @"触摸位置在当前label";
}
2、触摸移动方法中,一般实现的属性有:
2-1、获取触摸对象,如:
UITouch *touch = touches.anyObject;
2-2、获取当前点击位置,以及上一个点击位置,如:
[objc] view
plain copy

CGPoint currentPoint = [touch locationInView:self.view];
CGPoint previousPoint = [touch previousLocationInView:self.view];
注:可通过触摸移动改变子视图的位置,如:
[objc] view
plain copy

float moveX = currentPoint.x - previousPoint.x;
float moveY = currentPoint.y - previousPoint.y;
CGFloat centerX = self.label.center.x + moveX;
CGFloat centerY = self.label.center.y + moveY;
self.label.center = CGPointMake(centerX, centerY);
[objc] view
plain copy

// 触摸开始
- (void)touchesBegan:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event
{
// self.view.backgroundColor = [UIColor colorWithRed:(arc4random() % 255 / 255.0) green:(arc4random() % 255 / 255.0) blue:(arc4random() % 255 / 255.0) alpha:1.0];
NSLog(@"触摸手势 开始");
// 获取触摸对象
UITouch *touch = touches.anyObject;
// 获取触摸次数
NSInteger clickNumber = touch.tapCount;
NSLog(@"click number = %@", @(clickNumber));
if (2 == clickNumber)
{
self.label.backgroundColor = [UIColor redColor];
}
else if (3 == clickNumber)
{
self.label.backgroundColor = [UIColor greenColor];
}
// 获取触摸状态
UITouchPhase state = touch.phase;
NSLog(@"state = %ld", state);
// 获取响应视图对象
UIView *view = touch.view;
NSLog(@"view = %@", view);
// 获取当前触摸位置
CGPoint currentPoint = [touch locationInView:self.view];
NSLog(@"touch.locationInView = {%2.3f, %2.3f}", currentPoint.x, currentPoint.y);
// 获取当前触摸的前一个位置
CGPoint previousPoint = [touch previousLocationInView:self.view];
NSLog(@"touch.previousLocationInView = {%2.3f, %2.3f}", previousPoint.x, previousPoint.y);
// 获取触摸区域的子视图
// 方法1
// for (UIView *oneView in self.view.subviews)
// {
// if ([oneView isKindOfClass:[UILabel class]])
// {
// // 是否选中图标(当前坐标是否包含所选图标)
// if (CGRectContainsPoint(oneView.frame, currentPoint))
// {
// // 获取当前被选择图标
// UILabel *selectedView = (UILabel *)oneView;
// // 改变标题
// selectedView.text = @"触摸位置在当前label";
// }
// }
// }
// 方法2
if (CGRectContainsPoint(self.label.frame, currentPoint))
{
// 改变标题
self.label.text = @"触摸位置在当前label";
}
}
[objc] view
plain copy

// 触摸移动
- (void)touchesMoved:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event
{
// self.view.backgroundColor = [UIColor colorWithRed:(arc4random() % 255 / 255.0) green:(arc4random() % 255 / 255.0) blue:(arc4random() % 255 / 255.0) alpha:1.0];
NSLog(@"触摸手势 移动");
// 移动UI视图控件
// 方法1 获得触摸点的集合,可以判断多点触摸事件
for (UITouch *touch in event.allTouches)
{
// 获取当前触摸坐标
CGPoint currentPoint = [touch locationInView:self.view];
NSLog(@"touch.locationInView = {%2.3f, %2.3f}", currentPoint.x, currentPoint.y);
// 获取当前触摸前一个坐标
CGPoint previousPoint = [touch previousLocationInView:self.view];
NSLog(@"touch.previousLocationInView = {%2.3f, %2.3f}", previousPoint.x, previousPoint.y);
// 坐标偏移量 (效果异常??)
float moveX = currentPoint.x - previousPoint.x;
float moveY = currentPoint.y - previousPoint.y;
CGFloat centerX = self.label.center.x + moveX;
CGFloat centerY = self.label.center.y + moveY;
self.label.center = CGPointMake(centerX, centerY);
}
// 方法2
// UITouch *touch = touches.anyObject;
// // 获取当前触摸坐标
// CGPoint currentPoint = [touch locationInView:self.view];
// NSLog(@"touch.locationInView = {%2.3f, %2.3f}", currentPoint.x, currentPoint.y);
// // 获取当前触摸前一个坐标
// CGPoint previousPoint = [touch previousLocationInView:self.view];
// NSLog(@"touch.previousLocationInView = {%2.3f, %2.3f}", previousPoint.x, previousPoint.y);
// // 坐标偏移量
// float moveX = currentPoint.x - previousPoint.x;
// float moveY = currentPoint.y - previousPoint.y;
// CGFloat centerX = self.label.center.x + moveX;
// CGFloat centerY = self.label.center.y + moveY;
// self.label.center = CGPointMake(centerX, centerY);
}
[objc] view
plain copy

// 触摸结束
- (void)touchesEnded:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event
{
// self.view.backgroundColor = [UIColor whiteColor];
NSLog(@"触摸手势 结束");
self.label.text = @"touch触摸手势";
}
[objc] view
plain copy

// 触摸退出(注意:通常是受到设备其他方法的影响:如来电、电量不足关机等)
- (void)touchesCancelled:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event
{
NSLog(@"触摸手势 退出");
}
效果图
相关文章推荐
- IOS开发—事件处理,触摸事件,UITouch,UIEvent,响应者链条,手势识别
- iOS开发——UI进阶篇(十二)事件处理,触摸事件,UITouch,UIEvent,响应者链条,手势识别
- IOS学习 触摸和手势UITouch 捏合
- IOS学习 触摸和手势UITouch 单击双击、移动视图
- iOS触摸手势——UITouch
- iOS触摸手势知识介绍(UITouch & UIGestureRecognizer)
- ios 触摸与手势 UItouch
- iOS触摸手势知识介绍(UITouch & UIGestureRecognizer)
- ios UITouch 触摸事件的使用方法
- [IOS]触摸事件和手势
- [IOS]触摸事件和手势
- iOS&nbsp;触摸和手势总结
- iOS基础 - 触摸事件与手势识别
- iOS基础 - 触摸事件&手势识别
- IOS之触摸事件和手势
- iOS开发学习之触摸事件和手势识别
- IOS_触摸UITouch+手势识别GestureRecognizer+摇一摇+转换点坐标
- iOS-触摸与手势识别
- IOS之触摸事件和手势
- IOS开发之---触摸和手势