获取短信验证码时的倒计时按钮实现
2016-10-11 18:55
495 查看
在Android中实现倒计时有多种方式,如使用传统的Java方式Timer+TimerTask等,这里我们使用系统自带的类CountDownTimer。CountDownTimer内部使用的是Handler来实现倒计时功能的。
先来看效果图。
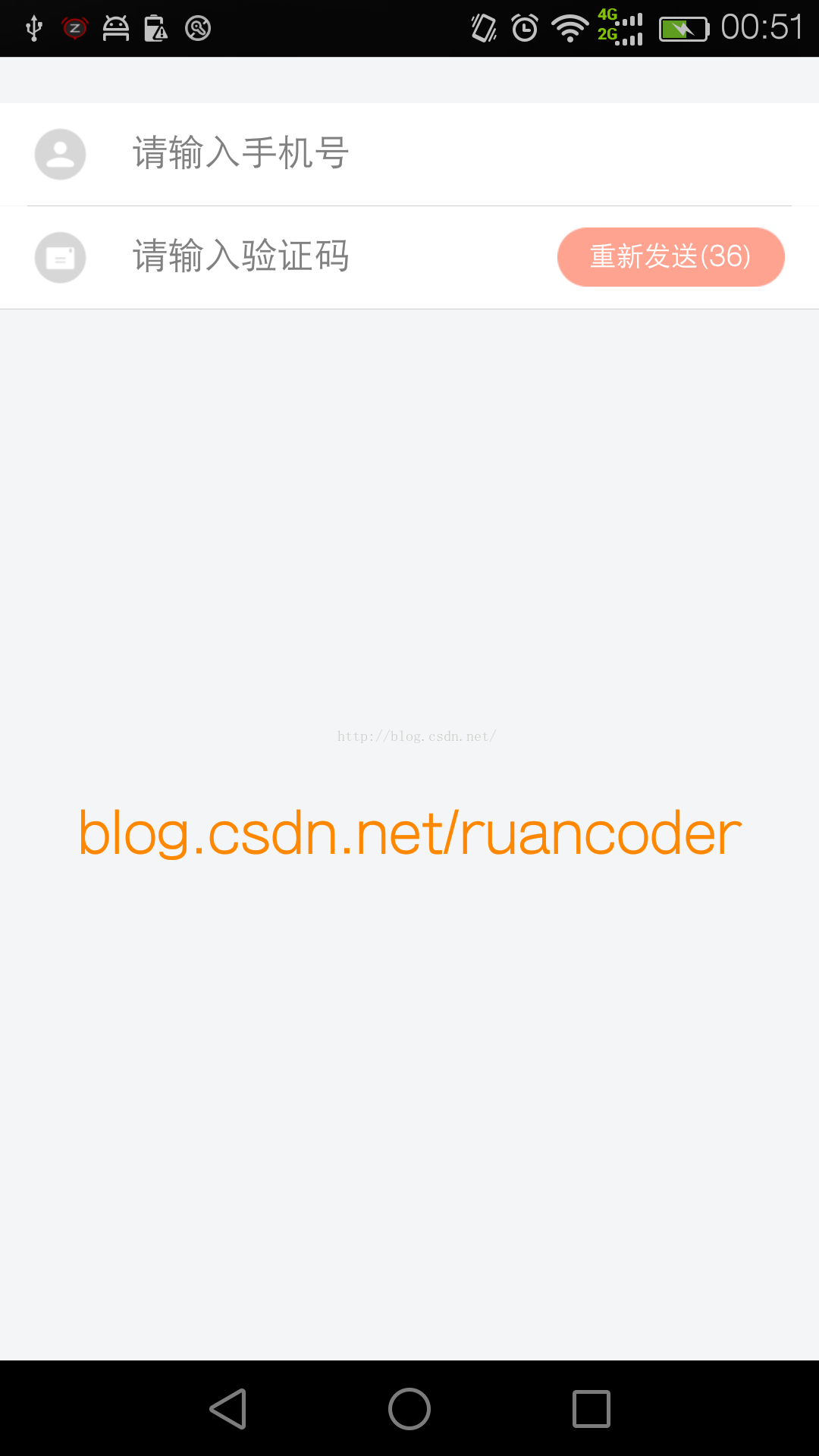
为了方便在多个页面中使用该功能(如注册、找回密码、重置密码),这里将该功能封装到CountDownTimerButton类中。
在使用CountDownTimer类时,需要重写两个方法。
public abstract void onTick(long millisUntilFinished);
倒计时进行中,在这里刷新按钮的数字。millisUntilFinished表示距离倒计时结束的剩余时间,单位毫秒。
public abstract void onFinish();
倒计时完成。
直接上CountDownTimerButton类代码,含详细注释:
[java]
view plain
copy
package net.csdn.blog.ruancoder;
import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.drawable.Drawable;
import android.os.CountDownTimer;
import android.util.AttributeSet;
import android.widget.Button;
/**
* 带倒计时的按钮
*/
public class CountDownTimerButton extends Button {
private Drawable mNormalBackground;
private Drawable mDisableBackground;
// 总倒计时时间
private static final long MILLIS_IN_FUTURE = 60 * 1000;
// 每次减去1秒
private static final long COUNT_DOWN_INTERVAL = 1000;
public CountDownTimerButton(Context context) {
this(context, null);
}
public CountDownTimerButton(Context context, AttributeSet attrs) {
this(context, attrs, 0);
}
public CountDownTimerButton(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init(context, attrs);
}
// 初始化
private void init(Context context, AttributeSet attrs) {
TypedArray a = context.obtainStyledAttributes(attrs, R.styleable.CountDownTimerButton);
mNormalBackground = a.getDrawable(R.styleable.CountDownTimerButton_normalBackground);// 默认背景
mDisableBackground = a.getDrawable(R.styleable.CountDownTimerButton_disableBackground);// 不可点击时的背景
setBackgroundDrawable(mNormalBackground);
}
// 启动倒计时
public void startCountDown() {
// 设置按钮为不可点击,并修改显示背景
setEnabled(false);
setBackgroundDrawable(mDisableBackground);
// 开始倒计时
new CountDownTimer(MILLIS_IN_FUTURE, COUNT_DOWN_INTERVAL) {
@Override
public void onTick(long millisUntilFinished) {
// 刷新文字
setText(getContext().getString(R.string.reget_sms_code_countdown, millisUntilFinished / COUNT_DOWN_INTERVAL));
}
@Override
public void onFinish() {
// 重置文字,并恢复按钮为可点击
setText(R.string.reget_sms_code);
setEnabled(true);
setBackgroundDrawable(mNormalBackground);
}
}.start();
}
}
上述代码中需要用到的attrs.xml:
[html]
view plain
copy
<?xml version="1.0" encoding="utf-8"?>
<resources>
<declare-styleable name="CountDownTimerButton">
<attr name="normalBackground" format="reference|color" />
<attr name="disableBackground" format="reference|color" />
</declare-styleable>
</resources>
以及strings.xml:
[html]
view plain
copy
<resources>
<string name="get_sms_code">获取验证码</string>
<string name="reget_sms_code">重新发送</string>
<string name="reget_sms_code_countdown">重新发送(%s)</string>
</resources>
最后附上完整工程下载链接:
http://download.csdn.net/detail/ruancoder/9571311
先来看效果图。
为了方便在多个页面中使用该功能(如注册、找回密码、重置密码),这里将该功能封装到CountDownTimerButton类中。
在使用CountDownTimer类时,需要重写两个方法。
public abstract void onTick(long millisUntilFinished);
倒计时进行中,在这里刷新按钮的数字。millisUntilFinished表示距离倒计时结束的剩余时间,单位毫秒。
public abstract void onFinish();
倒计时完成。
直接上CountDownTimerButton类代码,含详细注释:
[java]
view plain
copy
package net.csdn.blog.ruancoder;
import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.drawable.Drawable;
import android.os.CountDownTimer;
import android.util.AttributeSet;
import android.widget.Button;
/**
* 带倒计时的按钮
*/
public class CountDownTimerButton extends Button {
private Drawable mNormalBackground;
private Drawable mDisableBackground;
// 总倒计时时间
private static final long MILLIS_IN_FUTURE = 60 * 1000;
// 每次减去1秒
private static final long COUNT_DOWN_INTERVAL = 1000;
public CountDownTimerButton(Context context) {
this(context, null);
}
public CountDownTimerButton(Context context, AttributeSet attrs) {
this(context, attrs, 0);
}
public CountDownTimerButton(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init(context, attrs);
}
// 初始化
private void init(Context context, AttributeSet attrs) {
TypedArray a = context.obtainStyledAttributes(attrs, R.styleable.CountDownTimerButton);
mNormalBackground = a.getDrawable(R.styleable.CountDownTimerButton_normalBackground);// 默认背景
mDisableBackground = a.getDrawable(R.styleable.CountDownTimerButton_disableBackground);// 不可点击时的背景
setBackgroundDrawable(mNormalBackground);
}
// 启动倒计时
public void startCountDown() {
// 设置按钮为不可点击,并修改显示背景
setEnabled(false);
setBackgroundDrawable(mDisableBackground);
// 开始倒计时
new CountDownTimer(MILLIS_IN_FUTURE, COUNT_DOWN_INTERVAL) {
@Override
public void onTick(long millisUntilFinished) {
// 刷新文字
setText(getContext().getString(R.string.reget_sms_code_countdown, millisUntilFinished / COUNT_DOWN_INTERVAL));
}
@Override
public void onFinish() {
// 重置文字,并恢复按钮为可点击
setText(R.string.reget_sms_code);
setEnabled(true);
setBackgroundDrawable(mNormalBackground);
}
}.start();
}
}
上述代码中需要用到的attrs.xml:
[html]
view plain
copy
<?xml version="1.0" encoding="utf-8"?>
<resources>
<declare-styleable name="CountDownTimerButton">
<attr name="normalBackground" format="reference|color" />
<attr name="disableBackground" format="reference|color" />
</declare-styleable>
</resources>
以及strings.xml:
[html]
view plain
copy
<resources>
<string name="get_sms_code">获取验证码</string>
<string name="reget_sms_code">重新发送</string>
<string name="reget_sms_code_countdown">重新发送(%s)</string>
</resources>
最后附上完整工程下载链接:
http://download.csdn.net/detail/ruancoder/9571311
相关文章推荐
- 获取短信验证码时的倒计时按钮实现
- iOS开发中获取短信验证码倒计时按钮的实现
- 获取短信验证码 按钮 实现倒计时
- 获取短信验证码时的倒计时按钮实现
- iOS开发中获取短信验证码倒计时按钮的实现
- 实现短信验证码获取倒计时
- JS+HTML5实现获取手机验证码倒计时按钮
- JS实现用户注册时获取短信验证码和倒计时功能
- JS实现用户注册时获取短信验证码和倒计时功能
- 获取验证码按钮,点击后倒计时功能的实现
- 获取短信验证码之后按钮背景变化并且出现倒计时
- ionic+AngularJs实现获取验证码倒计时按钮
- JS获取短信验证码倒计时的实现代码
- js获取短信验证码按钮倒计时代码。
- iOS获取短信验证码倒计时的两种实现方法
- Android获取短信验证码按钮倒计时按钮
- js获取短信验证码倒计时重新发送的实现方法
- Angular.js实现获取验证码倒计时60秒按钮的简单方法
- 递归实现获取短信验证码按钮时间倒计时显示功能
- js获取时间查并实现倒计时读条