简单工厂模式Gof23+++小型mybatis+spring的整合总结
2016-09-11 21:37
344 查看
[b]一.代码无错就是优----简单工厂模式[/b]
[b]1.[/b]1)命名的规范性。
2)判断语句的合理写法。
3)考虑不肯能成立的条件。
[b]2[/b].活字印刷,面向对象
1)可维护
2)可扩展
3)可复用
4)灵活性好
[b]3.[/b]面向对象的好处
复用和复制,业务的封装
[b]4.[/b] 业务逻辑和界面逻辑分开
[b]5.[/b]紧耦合和松耦合
[b]6.[/b]简单工厂模式就是如何去实例化对象的问题
[b]注:UML的知识点补充[/b]
空三角形+实线:继承关系
Interface+棒棒糖:接口
空三角形+虚线:接口的实现
实线箭头:关联关系
空菱形+实线:聚合关系
实菱形+实线:拥有关系
虚箭头:依赖关系
开发j2ee的一些小总结:
1.建立如图所示的java工程
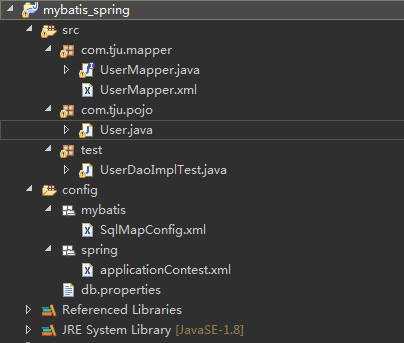
其中com.tju.mapper中存放的是mapper文件,必须有相同的名字,而且UserMapper.xml中的命名空间是UserMapper.java的包路径。
其中UserMapper.java
其中test包作为测试,这是成个程序的主入口,UserDaoImplTest.java的代码如下,是程序运行的开始
mybatis下的SqlMapConfig.xml:
程序运行的过程是:启动tomcat服务器,先到web.xml中,此时配置了spring中的applicationContext-*.xml,并且还有springmvc.xml配置。然后在applicationContext-dao中链接数据库配置中,配置了mybatis的SqlMapConfig.xml。
[b]一.代码无错就是优----简单工厂模式[/b]
[b]1.[/b]1)命名的规范性。
2)判断语句的合理写法。
3)考虑不肯能成立的条件。
[b]2[/b].活字印刷,面向对象
1)可维护
2)可扩展
3)可复用
4)灵活性好
[b]3.[/b]面向对象的好处
复用和复制,业务的封装
[b]4.[/b] 业务逻辑和界面逻辑分开
[b]5.[/b]紧耦合和松耦合
[b]6.[/b]简单工厂模式就是如何去实例化对象的问题
[b]注:UML的知识点补充[/b]
空三角形+实线:继承关系
Interface+棒棒糖:接口
空三角形+虚线:接口的实现
实线箭头:关联关系
空菱形+实线:聚合关系
实菱形+实线:拥有关系
虚箭头:依赖关系
开发j2ee的一些小总结:
1.建立如图所示的java工程
其中com.tju.mapper中存放的是mapper文件,必须有相同的名字,而且UserMapper.xml中的命名空间是UserMapper.java的包路径。
其中UserMapper.java
package com.tju.mapper; import java.util.List; import com.tju.pojo.User; public interface UserMapper { //查询用户通过id public User findUserById(int id) throws Exception; }其中UserMapper.xml
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <!-- namespace命名空间 ,对sql进行分类化的管理--> <mapper namespace="com.tju.mapper.UserMapper"> <select id="findUserById" parameterType="int" resultType="com.tju.pojo.User"> SELECT * FROM USER WHERE id=#{id} </select> </mapper>文件com.tju.pojo中存放的是简单User.java类
package com.tju.pojo; import java.io.Serializable; import java.util.Date; import java.util.List; public class User implements Serializable{ private int id; private String username; private Date birthday; private String sex; private String address; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } public Date getBirthday() { return birthday; } public void setBirthday(Date birthday) { this.birthday = birthday; } public String getSex() { return sex; } public void setSex(String sex) { this.sex = sex; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } public String toString() { return "User [id=" + id + ", username=" + username + ", birthday=" + birthday + ", sex=" + sex + ", address=" + address + "]"; } }
其中test包作为测试,这是成个程序的主入口,UserDaoImplTest.java的代码如下,是程序运行的开始
package test; import static org.junit.Assert.*; import org.junit.Before; import org.junit.Test; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import com.tju.mapper.UserMapper; import com.tju.pojo.User; public class UserDaoImplTest { private ApplicationContext applicationContext; @Before public void setUp() throws Exception { applicationContext = new ClassPathXmlApplicationContext("classpath:spring/applicationContest.xml"); } @Test public void testMapper() throws Exception { UserMapper userMapper = (UserMapper) applicationContext.getBean("userMapper"); User user = userMapper.findUserById(1); System.out.println(user); } }下面就是整个程序的配置文件config,分别包含mybatis,spring,db.properties的配置
mybatis下的SqlMapConfig.xml:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <typeAliases> <package name="com.tju.pojo"/> </typeAliases> </configuration>spring下的applicationContext.xml,其中配置mapperscanner能够自动扫描mapper中的接口函数,自动生成首字母小写的对象:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd"> <context:property-placeholder location="classpath:db.properties" /> <bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource" > <property name="driverClass" value="${jdbc.driver}" /> <property name="jdbcUrl" value="${jdbc.url}" /> <property name="user" value="${jdbc.username}" /> <property name="password" value="${jdbc.password}" /> <property name="maxPoolSize" value="30" /> <property name="minPoolSize" value="5" /> </bean> <bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean"> <!-- 加载mybatis配置文件 --> <property name="configLocation" value="mybatis/sqlMapConfig.xml"></property> <!-- 数据源 --> <property name="dataSource" ref="dataSource"></property> </bean> <!-- <bean id="userMapper" class="org.mybatis.spring.mapper.MapperFactoryBean"> <property name="mapperInterface" value="com.tju.mapper"></property> <property name="sqlSessionFactory" ref="sqlSessionFactory"></property> </bean> --> <bean class="org.mybatis.spring.mapper.MapperScannerConfigurer"> <property name="basePackage" value="com.tju.mapper"></property> </bean> </beans>其中db.properties:
jdbc.driver=com.mysql.jdbc.Driver jdbc.url=jdbc:mysql://localhost:3306/mybatis jdbc.username=root jdbc.password=
程序运行的过程是:启动tomcat服务器,先到web.xml中,此时配置了spring中的applicationContext-*.xml,并且还有springmvc.xml配置。然后在applicationContext-dao中链接数据库配置中,配置了mybatis的SqlMapConfig.xml。
相关文章推荐
- Spring + mybatis整合方案总结 结合实例应用
- MyBatis学习总结(八)——Mybatis3.x与Spring4.x整合
- Spring和Mybatis的整合总结
- MyBatis学习总结(八)——Mybatis3.x与Spring4.x整合
- MyBatis学习总结(八)——Mybatis3.x与Spring4.x整合
- Mybatis_spring 整合总结——1
- MyBatis学习总结(8)——Mybatis3.x与Spring4.x整合
- MyBatis学习总结——Mybatis3.x与Spring4.x整合
- MyBatis学习总结(八)——Mybatis3.x与Spring4.x整合
- MyBatis学习总结(八)——Mybatis3.x与Spring4.x整合
- mybatis-spring整合总结02_SqlSessionFactoryBean
- MyBatis学习总结(八)——Mybatis3.x与Spring4.x整合
- Java知识总结---整合SpringMVC+Mybatis+Spring(二)
- Spring学习总结——Spring整合MyBatis(Maven+MySQL)一
- Spring + mybatis整合方案总结 结合实例应用
- MyBatis学习总结(八)——Mybatis3.x与Spring4.x整合
- MyBatis学习总结(八)——Mybatis3.x与Spring4.x整合
- MyBatis学习总结(八)——Mybatis3.x与Spring4.x整合
- Spring整合mybatis总结:
- Spring学习总结——Spring整合MyBatis(Maven+MySQL)二