Servlet文件上传和下载---个人相册
2016-09-08 00:50
330 查看
这个小程序有以下功能:
上传照片、下载图片、浏览图片、删除图片(需要权限)
这个程序为了省事,dao层也没有分成接口、实现类和工厂类三个部分,就直接在dao的类中实现了想要的功能了
网页就相当于是表现层,值对象也写,servlet其实就相当于业务逻辑层了
此程序需要使用到几个jar包,导入到WEB-INF下的lib目录下:
用于文件传输:commons-fileupload-1.2.2.jar和commons-io-2.1.jar
don4j对xml文件操作:dom4j-1.6.1.jar和jaxen-1.1-beta-6.jar
在项目src下要建立一个xml文件存放上传的相片的信息,这个文件里面写上一对<photos></photos>标签,每上传一张照片就增加一对<photo>标签作为<photos>的子节点,其中photo中要添加照片的属性 id , 原文件名realName , 扩展名ext , 路径dir , 上传时间dateTime , ip地址;然后每个photo增加一个子节点<description>,其中设置text存放照片描述信息。
表现层:
主页index.jsp
值对象:
PhotoModel.java
业务逻辑层:
上传照片UploadServlet.java
DAO层
PhotoDAO.java
工具类
MyUtils.java
效果图:
主页:
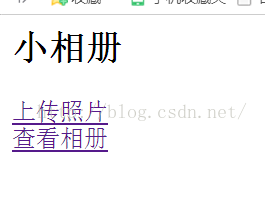
上传照片:
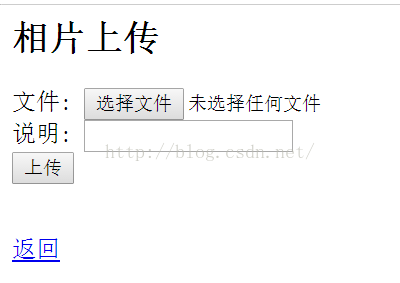
浏览相册:
上传照片、下载图片、浏览图片、删除图片(需要权限)
这个程序为了省事,dao层也没有分成接口、实现类和工厂类三个部分,就直接在dao的类中实现了想要的功能了
网页就相当于是表现层,值对象也写,servlet其实就相当于业务逻辑层了
此程序需要使用到几个jar包,导入到WEB-INF下的lib目录下:
用于文件传输:commons-fileupload-1.2.2.jar和commons-io-2.1.jar
don4j对xml文件操作:dom4j-1.6.1.jar和jaxen-1.1-beta-6.jar
在项目src下要建立一个xml文件存放上传的相片的信息,这个文件里面写上一对<photos></photos>标签,每上传一张照片就增加一对<photo>标签作为<photos>的子节点,其中photo中要添加照片的属性 id , 原文件名realName , 扩展名ext , 路径dir , 上传时间dateTime , ip地址;然后每个photo增加一个子节点<description>,其中设置text存放照片描述信息。
表现层:
主页index.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> </head> <body> <h2>小相册</h2> <a href="jsps/upload.jsp">上传照片</a><br/> <a href="showServlet">查看相册</a> </body> </html>上传照片upload.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> </head> <body> <h2>相片上传</h2> <form action="<%=request.getContextPath() %>/uploadServlet" method="post" enctype="multipart/form-data"> 文件: <input type="file" name="file" /><br/> 说明: <input type="text" name="description" /><br/> <input type="submit" value="上传" /> </form> <br/> <a href='javascript:history.go(-1)'>返回</a> </body> </html>
值对象:
PhotoModel.java
package cn.hncu.vo; public class PhotoModel { private String uuid; private String realName; private String ext; private String dir; private String dateTime; private String ip; private String description; public String getUuid() { return uuid; } public void setUuid(String uuid) { this.uuid = uuid; } public String getRealName() { return realName; } public void setRealName(String realName) { this.realName = realName; } public String getExt() { return ext; } public void setExt(String ext) { this.ext = ext; } public String getDir() { return dir; } public void setDir(String dir) { this.dir = dir; } public String getDateTime() { return dateTime; } public void setDateTime(String dateTime) { this.dateTime = dateTime; } public String getIp() { return ip; } public void setIp(String ip) { this.ip = ip; } public String getDescription() { return description; } public void setDescription(String description) { this.description = description; } @Override public String toString() { return "PhotoModel [uuid=" + uuid + ", realName=" + realName + ", ext=" + ext + ", dir=" + dir + ", dateTime=" + dateTime + ", ip=" + ip + ", description=" + description + "]"; } }
业务逻辑层:
上传照片UploadServlet.java
package cn.hncu.servlets; import java.io.File; import java.io.IOException; import java.io.InputStream; import java.io.PrintWriter; import java.util.List; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.apache.commons.fileupload.FileItem; import org.apache.commons.fileupload.FileUploadException; import org.apache.commons.fileupload.disk.DiskFileItemFactory; import org.apache.commons.fileupload.servlet.ServletFileUpload; import org.apache.commons.io.FileUtils; import cn.hncu.dao.PhotoDAO; import cn.hncu.utils.MyUtils; import cn.hncu.vo.PhotoModel; public class UploadServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html;charset=utf-8"); PrintWriter out = response.getWriter(); out.println("<!DOCTYPE HTML PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\">"); out.println("<HTML>"); out.println(" <HEAD><TITLE>A Servlet</TITLE></HEAD>"); out.println(" <BODY>"); out.print("不支持get方式提交"); out.println(" </BODY>"); out.println("</HTML>"); out.flush(); out.close(); } public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html;charset=utf-8"); PrintWriter out = response.getWriter(); out.println("<!DOCTYPE HTML PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\">"); out.println("<HTML>"); out.println(" <HEAD><TITLE>A Servlet</TITLE></HEAD>"); out.println(" <BODY>"); //处理上传文件 DiskFileItemFactory factory=new DiskFileItemFactory(); factory.setRepository(new File("D:/a/b")); ServletFileUpload upload=new ServletFileUpload(factory); upload.setSizeMax(1024*1024*100); request.setCharacterEncoding("utf-8"); FileItem fi=null; try { List<FileItem> list=upload.parseRequest(request); InputStream in=null; boolean boo=false; PhotoModel photo=new PhotoModel(); //for循环是把photo的信息封装完整,,,之后(for之后)才能把该photo对象保存到数据库 for (FileItem fii:list){ fi=fii; if (fi.isFormField()){ String description=fi.getString("utf-8"); photo.setDescription(description); } else { in=fi.getInputStream(); String fileName=fi.getName(); if (fileName==null||fileName.trim().equals("")){ out.print("没有选择文件!<br/><a href='javascript:history.go(-1)'>返回</a>"); return; } else { fileName=fileName.substring(fileName.lastIndexOf("/")+1); photo.setRealName(fileName);//文件名 String ext=fileName.substring(fileName.lastIndexOf(".")); photo.setExt(ext);//后缀名 photo.setDateTime(MyUtils.getCurrentTime());//时间 photo.setUuid(MyUtils.getUuid());//id photo.setDir(MyUtils.getDir(photo.getUuid()));//目录 photo.setIp(request.getRemoteAddr());//ip地址 } } } //把相片信息存储到数据库 PhotoDAO dao=new PhotoDAO(); boo=dao.save(photo); //如果上面的相片信息保存成功,那么才开始接收图片文件,把它保存到服务器硬盘 if (boo){ String path=getServletContext().getRealPath("/photos"); path=path+"/"+photo.getDir(); File file=new File(path); if (!file.exists()){ file.mkdirs(); } String fileName2=path+"/"+photo.getUuid()+photo.getExt(); FileUtils.copyInputStreamToFile(in, new File(fileName2)); out.print("上传成功!<br/><a href='javascript:history.go(-1)'>返回</a>"); } else { out.print("上传失败!<br/><a href='javascript:history.go(-1)'>返回</a>"); } } catch (FileUploadException e) { throw new RuntimeException("上传失败", e); } finally { if (fi!=null){ fi.delete(); } } out.println(" </BODY>"); out.println("</HTML>"); out.flush(); out.close(); } }浏览相册ShowServlet.java
package cn.hncu.servlets; import java.io.IOException; import java.io.PrintWriter; import java.util.List; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import cn.hncu.dao.PhotoDAO; import cn.hncu.vo.PhotoModel; public class ShowServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doPost(request, response); } public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html;charset=utf-8"); PrintWriter out = response.getWriter(); out.println("<!DOCTYPE HTML PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\">"); out.println("<HTML>"); out.println(" <HEAD><TITLE>A Servlet</TITLE></HEAD>"); out.println(" <BODY>"); String table="<table border=1px width=600px >"+ "<tr><th>文件名</th> <th>上传时间</th> <th>图片</th> <th>说明</th> <th>操作</th></tr>"; out.print(table); //显示所有的图片 PhotoDAO dao=new PhotoDAO(); List<PhotoModel> list=dao.getAll(); for (PhotoModel model:list){ out.print("<tr>"); out.print("<td width=100>"+model.getRealName()+"</td>"); out.print("<td width=100>"+model.getDateTime()+"</td>"); String path=request.getContextPath()+"/photos/"+model.getDir()+"/"+model.getUuid()+model.getExt(); out.print("<td><a href='"+path+"'><img height=200 width=200 src='"+path+"'/></a></td>"); out.print("<td width=100>"+model.getDescription()+"</td>"); String strDelete="<a href='"+request.getContextPath()+"/deleteServlet?id="+model.getUuid()+"'>删除</a>"; String strDownload="<a href='"+request.getContextPath()+"/downloadServlet?id="+model.getUuid()+"'>下载</a>"; String str=strDownload+" "+strDelete; out.print("<td width=100>"+str+"</td>"); out.print("</tr>"); } out.print("</table>"); out.println(" </BODY>"); out.println("</HTML>"); out.flush(); out.close(); } }下载文件DownloadServlet.java
package cn.hncu.servlets; import java.io.File; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; import java.io.PrintWriter; import java.net.URLEncoder; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import cn.hncu.dao.PhotoDAO; import cn.hncu.vo.PhotoModel; public class DownloadServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doPost(request, response); } public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { PhotoDAO dao=new PhotoDAO(); String id=request.getParameter("id"); PhotoModel photo=dao.getSingleById(id); if (photo!=null){ response.setContentType("application/force-download"); String realName=photo.getRealName(); realName=URLEncoder.encode(realName, "utf-8"); //设置响应头,以让客户端软件下载时能够显示该文件名 response.setHeader("content-disposition", "attachment;filename='"+realName+"'"); String fileName="photos/"+photo.getDir()+"/"+photo.getUuid()+photo.getExt(); String filePathName=getServletContext().getRealPath(fileName); InputStream in=new FileInputStream(new File(filePathName)); OutputStream out=response.getOutputStream(); int len=0; byte[] buf=new byte[1024]; while ((len=in.read(buf))!=-1){ out.write(buf, 0, len); } out.close(); } else { response.setContentType("text/html;charset=utf-8"); PrintWriter out=response.getWriter(); out.print("该文件已被删除"); out.print("<br/><a href='javascript:history.go(-1)'>返回</a>"); } } }删除照片DeleteServlet.java
package cn.hncu.servlets; import java.io.File; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import cn.hncu.dao.PhotoDAO; import cn.hncu.vo.PhotoModel; public class DeleteServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doPost(request, response); } public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html;charset=utf-8"); PrintWriter out = response.getWriter(); String id=request.getParameter("id"); String ip=request.getRemoteAddr(); PhotoDAO dao=new PhotoDAO(); PhotoModel photo=dao.getSingleById(id); if (photo!=null){ if (!ip.equals(photo.getIp())){ out.print("你无权删除!<br/><a href='javascript:history.go(-1)'>返回</a>"); return; } //1删除数据库中的信息 boolean boo=dao.delete(photo.getUuid()); //2把服务器硬盘中的文件删除 if (boo){ String fileName="photos/"+photo.getDir()+"/"+photo.getUuid()+photo.getExt(); String filePathName=getServletContext().getRealPath(fileName); File file=new File(filePathName); if (file.exists()){ file.delete(); } String showPath=getServletContext().getContextPath()+"/showServlet"; out.print("删除成功!<br/><a href='"+showPath+"'>浏览相册</a>"); } else { out.print("相片删除失败<br/><a href='javascript:history.go(-1)'>返回</a>"); } } else { out.print("该文件不存在<br/><a href='javascript:history.go(-1)'>返回</a>"); } } }
DAO层
PhotoDAO.java
package cn.hncu.dao; import java.util.ArrayList; import java.util.Iterator; import java.util.List; import org.dom4j.Document; import org.dom4j.Element; import cn.hncu.utils.Dom4jFactory; import cn.hncu.vo.PhotoModel; public class PhotoDAO { public boolean save(PhotoModel model){ Document dom=Dom4jFactory.getDom(); Element root=dom.getRootElement(); Element photoElement=root.addElement("photo"); photoElement.addAttribute("id", model.getUuid()); photoElement.addAttribute("ip", model.getIp()); photoElement.addAttribute("realName", model.getRealName()); photoElement.addAttribute("dir", model.getDir()); photoElement.addAttribute("ext", model.getExt()); photoElement.addAttribute("dateTime", model.getDateTime()); photoElement.addAttribute("description", model.getDescription()); boolean boo=Dom4jFactory.save(); return boo; } public List<PhotoModel> getAll(){ List<PhotoModel> list=new ArrayList<PhotoModel>(); Element root=Dom4jFactory.getDom().getRootElement(); Iterator<Element> it=root.elementIterator("photo"); while (it.hasNext()){ PhotoModel model=new PhotoModel(); Element e=it.next(); model.setDateTime(e.attributeValue("dateTime")); model.setDir(e.attributeValue("dir")); model.setExt(e.attributeValue("ext")); model.setIp(e.attributeValue("ip")); model.setRealName(e.attributeValue("realName")); model.setUuid(e.attributeValue("id")); model.setDescription(e.elementText("description")); list.add(model); } return list; } public PhotoModel getSingleById(String uuid){ List<PhotoModel> list=getAll(); for (PhotoModel p:list){ if (p.getUuid().equals(uuid)){ return p; } } return null; } public boolean delete(String id){ Document dom=Dom4jFactory.getDom(); Element e=(Element) dom.selectSingleNode("//photo[@id='"+id+"']"); return e.getParent().remove(e); } }
工具类
MyUtils.java
package cn.hncu.utils; import java.text.SimpleDateFormat; import java.util.Date; import java.util.UUID; public class MyUtils { public static String getUuid(){ return UUID.randomUUID().toString().replace("-", ""); } public static String getDir(String uuid){ String dir1=Integer.toHexString(uuid.hashCode() & 0xf); String dir2=Integer.toHexString((uuid.hashCode() & 0xf0)>>4); String dir=dir1+"/"+dir2; return dir; } private static SimpleDateFormat format=new SimpleDateFormat("yyyy-MM-dd hh:mm:ss"); public static String getCurrentTime(){ return format.format(new Date()); } }Dom4jFactory.java
package cn.hncu.utils; import java.text.SimpleDateFormat; import java.util.Date; import java.util.UUID; public class MyUtils { public static String getUuid(){ return UUID.randomUUID().toString().replace("-", ""); } public static String getDir(String uuid){ String dir1=Integer.toHexString(uuid.hashCode() & 0xf); String dir2=Integer.toHexString((uuid.hashCode() & 0xf0)>>4); String dir=dir1+"/"+dir2; return dir; } private static SimpleDateFormat format=new SimpleDateFormat("yyyy-MM-dd hh:mm:ss"); public static String getCurrentTime(){ return format.format(new Date()); } }
效果图:
主页:
上传照片:
浏览相册:
相关文章推荐
- 关于JSP/Servlet文件上传与下载
- 文件上传和下载-ServletFileUpload
- 【ZT】Jsp/Servlet:实现文件上传与下载【二】
- Servlet 实现文件的上传与下载
- Servlet中的上传下载文件
- 用SmartUpload实现文件的上传下载(在servlet中实现)
- Jsp/Servlet:实现文件上传与下载 推荐
- 【ZT】Jsp/Servlet:实现文件上传与下载【三】
- Servlet文件的上传、下载
- servlet文件上传下载
- servlet 实现 文件的上传与下载
- Jsp/Servlet:实现文件上传与下载
- Jsp/Servlet:实现文件上传与下载
- JSP/servlet实现文件上传下载和删除
- SERVLET与JSP_文件上传下载(4)-java
- JSP/Servlet文件上传下载
- 文件上传(servlet 中含下载和删除操作)
- Servlet实现文件的上传下载
- 文件上传和下载-ServletFileUpload and DiskFileItemFactory
- Servlet 实现文件的上传与下载