文件操作,输入输出流(stream, writer)
2016-08-07 03:06
363 查看
一, 文件常用API
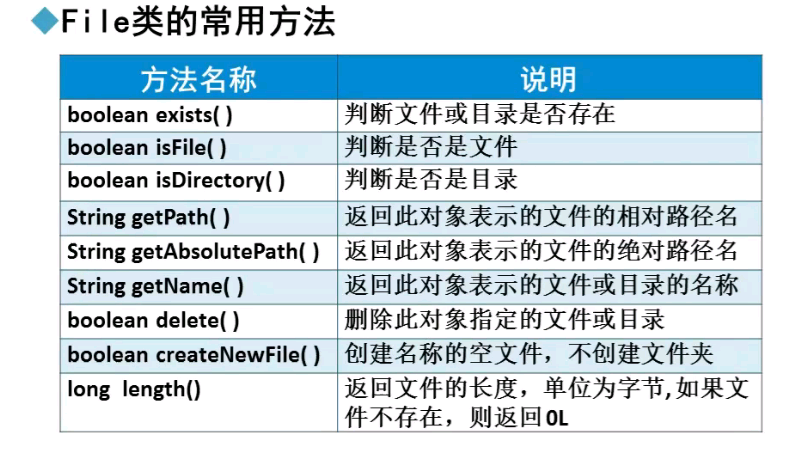
二,输入流
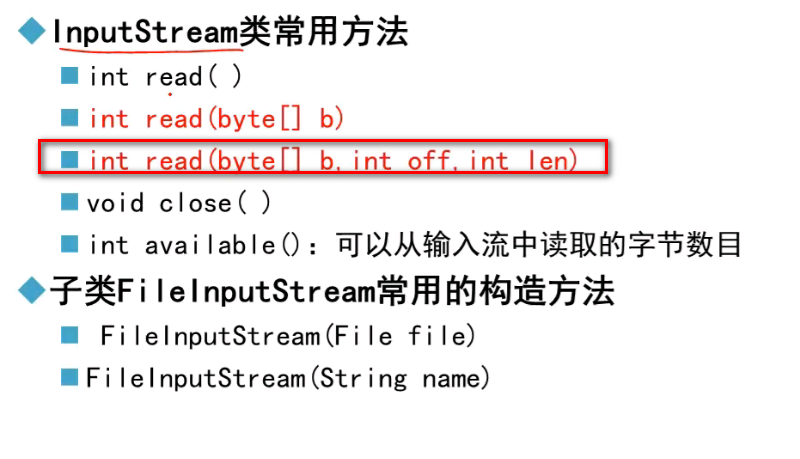
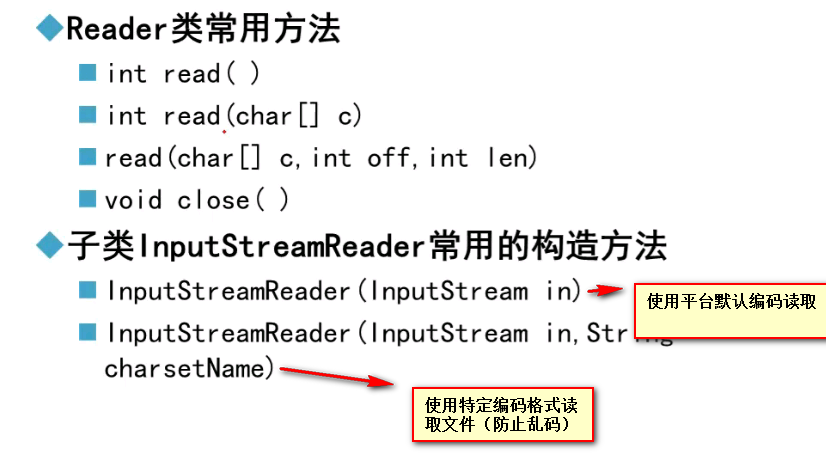
三,输出流
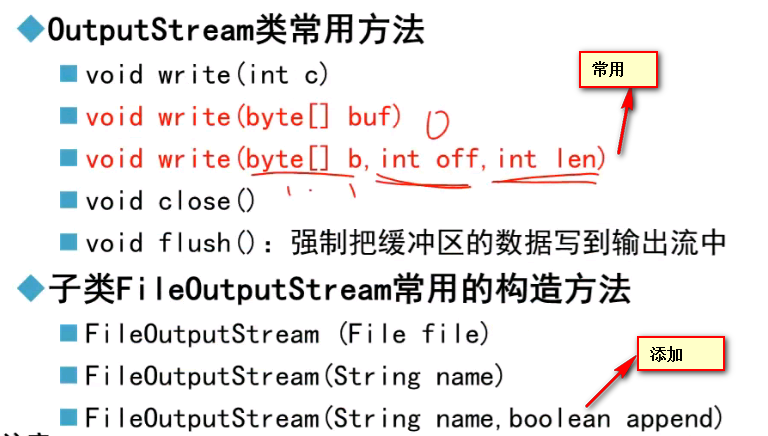
这里有一个文件内容复制的实例:
四,对象序列化实例
注意:
判断是否读取完的方式:
1,如果实 BufferedReader 对象的方法:判断是否为 null;
2,fouze否则判断是否为 -1;
字节流不会产生乱码,可以读取任何文件, 字符流可能会产生乱码
输出流操作不要忘记 flush() 操作;
二,输入流
三,输出流
这里有一个文件内容复制的实例:
package stream; import java.io.*; /** * Created by Administrator on 2016/8/7. * 文件输入输出流标准示范 */ public class Test { public static void main(String[] args) { File source = new File("f:/log/source.txt"); File target = new File("f:/log/target.txt"); FileInputStream fis = null; FileOutputStream fos = null; try { fis = new FileInputStream(source); fos = new FileOutputStream(target, true); //true 表示向文件添加数据,不覆盖 int len; byte[] bytes = new byte[1024]; while ((len = fis.read(bytes)) != -1) { //读取资源文件 /* 这里使用len ,而不是字节的长度*/ fos.write(bytes, 0, len); //写到目标文件之中 } fos.flush(); //刷新输出流 } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e1) { e1.printStackTrace(); } finally { //关闭流 try { fis.close(); fos.close(); } catch (IOException e) { e.printStackTrace(); } } } }
package stream; import java.io.*; /** * Created by Administrator on 2016/8/7. * 缓冲字符流实例 */ public class CharStream { public static void main(String[] args) { Reader fr = null; BufferedReader br = null; Writer wr = null; BufferedWriter bw = null; try { fr = new InputStreamReader(new FileInputStream("f:log/source.txt"), "utf-8"); //使用特定编码方式读取文件,防止文件乱码 br = new BufferedReader(fr); wr = new OutputStreamWriter(new FileOutputStream("f:log/target.txt")); //true:文件追加 bw = new BufferedWriter(wr); String line; while ((line = br.readLine()) != null) { bw.write(line); bw.newLine(); } bw.flush(); //刷新输出流 } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e1) { e1.printStackTrace(); } finally { try { bw.close(); br.close(); br.close(); fr.close(); } catch (IOException e) { e.printStackTrace(); } } } }
四,对象序列化实例
package stream; import threadTest.socket.Person; import java.io.*; /** * Created by Administrator on 2016/8/7. * 序列化对象 */ public class SeriaStream { public static void main(String[] args) { ByteArrayOutputStream baos = null; ObjectOutputStream ops = null; ByteArrayInputStream bais = null; ObjectInputStream ois = null; byte[] bytes = null; Person person = new Person(); //被实例化的类 person.setName("name"); try { /*序列化*/ baos = new ByteArrayOutputStream(); //存储对象序列化后的字节数组 ops = new ObjectOutputStream(baos); ops.writeObject(person); bytes = baos.toByteArray(); /*反序列化*/ bais = new ByteArrayInputStream(bytes); ois = new ObjectInputStream(bais); Person per = (Person)ois.readObject(); System.out.println(per.getName()); } catch (IOException e) { e.printStackTrace(); } catch(ClassNotFoundException e1){ e1.printStackTrace(); } finally { try { ois.close(); bais.close(); ops.close(); baos.close(); } catch (IOException e) { e.printStackTrace(); } } } }
注意:
判断是否读取完的方式:
1,如果实 BufferedReader 对象的方法:判断是否为 null;
2,fouze否则判断是否为 -1;
字节流不会产生乱码,可以读取任何文件, 字符流可能会产生乱码
输出流操作不要忘记 flush() 操作;
相关文章推荐
- 基于缓冲字节输入输出流进行复制文件的操作
- C++输入输出流(文件的操作)
- ASP.NET中StreamReader、FileStream、StreamWriter操作文件编码问题
- C++的输入输出流、文件操作
- 输入输出流(IO)—文件字节流(FileInputStream & FileOutputStream)的基本操作及运用
- 输入输出流(主要是文件操作)
- C++的输入输出流、文件操作
- C++标准程序库的输入输出流(I/O Stream)复制文件(4种方法)
- C++的输入输出流、文件操作
- 输入/输出流---打开,读写文件操作
- java输入输出流及文件操作
- c# 文件IO操作 StreamReader StreamWriter Split 使用
- java输入输出流及文件操作
- 输入输出流(IO)—文件字符流(FileReader & FileWriter)的基本操作及应用
- ASP.NET中StreamReader、FileStream、StreamWriter操作文件编码问题
- ASP.NET中StreamReader、FileStream、StreamWriter操作文件编码问题
- 12-21C#电脑蓝屏效果(可以恶搞整人哦)、输入输出流(StreamReader/streamWriter)
- 黑马程序员-----输入输出流及文件操作
- 2013级C++第15周(春)项目——输入输出流及文件文件操作
- 输入/输出流和文件操作