Android 自定义数字加减器
2016-07-17 15:14
423 查看
该自定义View主要是实现一款效果不错的数字加减器的功能的,但是也可以自定义选择器的外观颜色等。
1、自定义View的布局(add_sub_view.xml)
2、定义该View的一些属性(attrs.xml)
3、定义该View的Java类(AddAndSubView.java)
4、在显示视图中添加该自定义View
效果如下图:
1、自定义View的布局(add_sub_view.xml)
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@android:color/white" android:orientation="horizontal"> <Button android:id="@+id/bt01" android:layout_width="32dp" android:layout_height="32dp" android:scaleType="fitCenter" android:textSize="18sp"/> <TextView android:id="@+id/et01" android:layout_width="32dp" android:layout_height="32dp" android:enabled="false" android:layout_gravity="center" android:gravity="center" android:textColor="#000000" android:textSize="16sp"> </TextView> <Button android:id="@+id/bt02" android:layout_width="32dp" android:layout_height="32dp" android:scaleType="fitCenter" android:textSize="18sp"/> </LinearLayout>
2、定义该View的一些属性(attrs.xml)
<?xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name="AddAndSubView"> <attr name="textColor" format="color"/> <attr name="initValue" format="integer"/> <attr name="maxValue" format="integer"/> <attr name="minValue" format="integer"/> <attr name="textSize" format="dimension"/> <attr name="textFrameBackground" format="reference|color"/> <attr name="addBackground" format="reference|color"/> <attr name="subBackground" format="reference|color"/> <attr name="textFrameWidth" format="dimension"/> <attr name="addWidth" format="dimension"/> <attr name="subWidth" format="dimension"/> <attr name="addText" format="string"/> <attr name="subText" format="string"/> </declare-styleable> </resources>
3、定义该View的Java类(AddAndSubView.java)
package com.ileevey.addsub; import android.content.Context; import android.content.res.Resources; import android.content.res.TypedArray; import android.graphics.drawable.ColorDrawable; import android.graphics.drawable.Drawable; import android.util.AttributeSet; import android.view.LayoutInflater; import android.view.View; import android.widget.Button; import android.widget.LinearLayout; import android.widget.TextView; public class AddAndSubView extends LinearLayout{ /** 显示文本 */ private TextView mTextView; /** 增加按钮 */ private Button btAdd; /** 减少按钮 */ private Button btReduce; /** 显示文本的长宽 */ private int textFrameWidth; /** 显示文本及button中文字的颜色 */ private int textColor; /** 初始值 */ private int initValue; /** 最大值 */ private int maxValue; /** 最小值 */ private int minValue; /** 显示文本及button中文字的大小 */ private int textSize; /** 显示文本的背景 */ private Drawable textFrameBackground; /** 增加按钮的背景 */ private Drawable addBackground; /** 减少按钮的背景 */ private Drawable subBackground; /** 增加按钮的大小 */ private int addWidth; /** 减少按钮的大小 */ private int subWidth; /** 增加按钮中的文本 */ private String addText; /** 减少按钮中的文本 */ private String subText; public AddAndSubView(Context context, AttributeSet attrs) { super(context, attrs); initWidget(context); TypedArray a = context.obtainStyledAttributes(attrs, R.styleable.AddAndSubView); textColor = a.getColor(R.styleable.AddAndSubView_textColor, getResources().getColor(android.R.color.black)); textSize = a.getDimensionPixelSize(R.styleable.AddAndSubView_textSize, 16); textFrameBackground = a.getDrawable(R.styleable.AddAndSubView_textFrameBackground); textFrameWidth = a.getDimensionPixelSize(R.styleable.AddAndSubView_textFrameWidth, 48); addBackground = a.getDrawable(R.styleable.AddAndSubView_addBackground); subBackground = a.getDrawable(R.styleable.AddAndSubView_subBackground); initValue = a.getInt(R.styleable.AddAndSubView_initValue, 0); maxValue = a.getInt(R.styleable.AddAndSubView_maxValue, 1000000000); minValue = a.getInt(R.styleable.AddAndSubView_minValue, -1000000000); addWidth = a.getDimensionPixelSize(R.styleable.AddAndSubView_addWidth, 48); subWidth = a.getDimensionPixelSize(R.styleable.AddAndSubView_subWidth, 48); addText = a.getString(R.styleable.AddAndSubView_addText); subText = a.getString(R.styleable.AddAndSubView_subText); setAddBackground(addBackground); setAddText(addText); setAddWidth(addWidth); setInitValue(initValue); setMaxValue(maxValue); setMinValue(minValue); setSubBackground(subBackground); setSubText(subText); setSubWidth(subWidth); setTextColor(textColor); setTextFrameBackground(textFrameBackground); setTextFrameWidth(textFrameWidth); setTextSize(textSize); a.recycle(); } protected void onFinishInflate() { super.onFinishInflate(); addListener(); } public void initWidget(Context context){ LayoutInflater.from(context).inflate(R.layout.add_sub_view, this); mTextView = (TextView)findViewById(R.id.et01); btAdd = (Button)findViewById(R.id.bt01); btReduce = (Button)findViewById(R.id.bt02); } public void addListener(){ btAdd.setOnClickListener(new OnClickListener() { public void onClick(View v) { int num = Integer.valueOf(mTextView.getText().toString()); num++; if (num >= maxValue+1) return; mTextView.setText(Integer.toString(num)); } }); btReduce.setOnClickListener(new OnClickListener() { @Override public void onClick(View v) { int num = Integer.valueOf(mTextView.getText().toString()); num--; if (num <= minValue-1) return; mTextView.setText(Integer.toString(num)); } }); } public int getTextFrameWidth() { return textFrameWidth; } public void setTextFrameWidth(int textFrameWidth) { this.textFrameWidth = textFrameWidth; mTextView.setWidth(textFrameWidth); mTextView.setHeight(textFrameWidth); } public int getTextColor() { return textColor; } public void setTextColor(int textColor) { this.textColor = textColor; mTextView.setTextColor(textColor); btAdd.setTextColor(textColor); btReduce.setTextColor(textColor); } public int getInitValue() { return initValue; } public void setInitValue(int initValue) { this.initValue = initValue; mTextView.setText(String.valueOf(initValue)); } public int getMaxValue() { return maxValue; } public void setMaxValue(int maxValue) { this.maxValue = maxValue; } public int getMinValue() { return minValue; } public void setMinValue(int minValue) { this.minValue = minValue; } public int getTextSize() { return textSize; } public void setTextSize(int textSize) { this.textSize = textSize; mTextView.setTextSize(textSize); } public Drawable getTextFrameBackground() { return textFrameBackground; } public void setTextFrameBackground(Drawable textFrameBackground) { this.textFrameBackground = textFrameBackground; mTextView.setBackgroundDrawable(textFrameBackground); } public Drawable getAddBackground() { return addBackground; } public void setAddBackground(Drawable addBackground) { this.addBackground = addBackground; Resources res = getResources(); int color = res.getColor(android.R.color.darker_gray); Drawable drawable = new ColorDrawable(color); btAdd.setBackgroundDrawable(addBackground==null?drawable:addBackground); } public Drawable getSubBackground() { return subBackground; } public void setSubBackground(Drawable subBackground) { this.subBackground = subBackground; Resources res = getResources(); int color = res.getColor(android.R.color.darker_gray); Drawable drawable = new ColorDrawable(color); btReduce.setBackgroundDrawable(subBackground==null?drawable:subBackground); } public int getAddWidth() { return addWidth; } public void setAddWidth(int addWidth) { this.addWidth = addWidth; btAdd.setWidth(addWidth); btAdd.setHeight(addWidth); } public int getSubWidth() { return subWidth; } public void setSubWidth(int subWidth) { this.subWidth = subWidth; btReduce.setWidth(subWidth); btReduce.setHeight(subWidth); } public String getAddText() { return addText; } public void setAddText(String addText) { this.addText = addText; btAdd.setText(addText); } public String getSubText() { return subText; } public void setSubText(String subText) { this.subText = subText; btReduce.setText(subText); } }
4、在显示视图中添加该自定义View
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:asv="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@android:color/white" android:orientation="vertical"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_margin="16dp" android:text="数字通过按键可以加减: " android:textSize="20sp"> </TextView> <com.ileevey.addsub.AddAndSubView android:id="@+id/meter" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" asv:addBackground="@drawable/selector_add" asv:initValue="0" asv:maxValue="10" asv:minValue="-10" asv:subBackground="@drawable/selector_sub"/> </LinearLayout>
效果如下图:
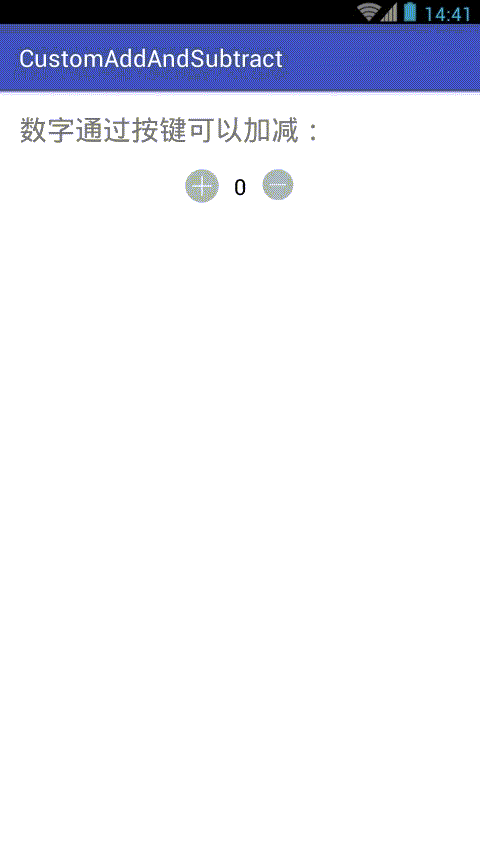
相关文章推荐
- [Android Pro] Android 性能分析工具dumpsys的使用
- (OK) [android-x86-6.0-rc1] grub - set timeout=5
- Android启动模式与onNewIntent
- android fragment重影问题
- Android 隐式意图和显示意图的使用场景
- Android 自定义ViewGroup 实战篇 -> 实现FlowLayout
- android软件盘弹出一系列问题
- android 线程小笔记2
- Windows环境下Android Studio进行NDK开发
- Android MVP开发模式实例
- Android下拉刷新之ZrcListView
- 【转】Android 服务器之SFTP服务器上传下载功能
- android ListView详解
- Android安全小知识
- [Android Pro] Android libdvm.so 与 libart.so
- Android主题换肤 无缝切换
- android-----关于通过AIDL注册监听之后无法解除监听的探索
- [Android Pro] Android 进程级别 和 oom_adj对应关系
- 使用Gradle管理你的Android Studio工程
- Android学习过程中遇到的一些名词