Android之动画3D旋转
2016-07-16 13:30
381 查看
本文主要记录一个坑
关于3D的旋转,三维空间有xyz轴,手机上x轴:手机的宽;y轴:手机的高;z轴:手机到人眼那个垂直于手机面的方向,3D旋转就是围绕y轴旋转。本文主要内容来自Google的官方demo。
不知道怎么下载google sample请看步骤(Android studio)
File --> New --> Import samlpe...(如果你被墙了,戳这里,只能帮你到这里了)
先说说这个坑是啥:在onCreate()里无法得到布局控件的宽和高,那就去onResume()里,那时视图都加载完了,不好意思,依旧获取不到,只能在onClick(listener是观察者模式)里使用时再得到值
看看3D效果
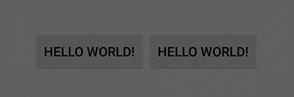
看看3D旋转的Util
怎么使用的,我就两个button布局
mainActivity里,基于上面的坑,只能使用时再获取控件宽和高了
关于3D的旋转,三维空间有xyz轴,手机上x轴:手机的宽;y轴:手机的高;z轴:手机到人眼那个垂直于手机面的方向,3D旋转就是围绕y轴旋转。本文主要内容来自Google的官方demo。
不知道怎么下载google sample请看步骤(Android studio)
File --> New --> Import samlpe...(如果你被墙了,戳这里,只能帮你到这里了)
先说说这个坑是啥:在onCreate()里无法得到布局控件的宽和高,那就去onResume()里,那时视图都加载完了,不好意思,依旧获取不到,只能在onClick(listener是观察者模式)里使用时再得到值
看看3D效果
看看3D旋转的Util
import android.graphics.Camera; import android.graphics.Matrix; import android.view.animation.Animation; import android.view.animation.Transformation; /** * <p>Description: 使用Camera来实现,Rotate3dAnimation使用的是绕Y轴旋转 </p> * Created by slack on 2016/7/13 18:35 . * * from : http://blog.163.com/benben_long/blog/static/19945824320141117443156/ */ public class Rotate3dAnimation extends Animation { private final float mFromDegrees; private final float mToDegrees; private final float mCenterX; private final float mCenterY; private final float mDepthZ; private final boolean mReverse; private Camera mCamera; /** * Creates a new 3D rotation on the Y axis. The rotation is defined by its * start angle and its end angle. Both angles are in degrees. The rotation * is performed around a center point on the 2D space, definied by a pair * of X and Y coordinates, called centerX and centerY. When the animation * starts, a translation on the Z axis (depth) is performed. The length * of the translation can be specified, as well as whether the translation * should be reversed in time. * * @param fromDegrees the start angle of the 3D rotation * @param toDegrees the end angle of the 3D rotation * @param centerX the X center of the 3D rotation * @param centerY the Y center of the 3D rotation * @param reverse true if the translation should be reversed, false otherwise */ public Rotate3dAnimation(float fromDegrees, float toDegrees, float centerX, float centerY, float depthZ, boolean reverse) { mFromDegrees = fromDegrees; mToDegrees = toDegrees; mCenterX = centerX; mCenterY = centerY; mDepthZ = depthZ; mReverse = reverse; } @Override public void initialize(int width, int height, int parentWidth, int parentHeight) { super.initialize(width, height, parentWidth, parentHeight); mCamera = new Camera(); } @Override protected void applyTransformation(float interpolatedTime, Transformation t) { final float fromDegrees = mFromDegrees; float degrees = fromDegrees + ((mToDegrees - fromDegrees) * interpolatedTime); final float centerX = mCenterX; final float centerY = mCenterY; final Camera camera = mCamera; final Matrix matrix = t.getMatrix(); camera.save(); if (mReverse) { camera.translate(0.0f, 0.0f, mDepthZ * interpolatedTime); } else { camera.translate(0.0f, 0.0f, mDepthZ * (1.0f - interpolatedTime)); } camera.rotateY(degrees); camera.getMatrix(matrix); camera.restore(); matrix.preTranslate(-centerX, -centerY); matrix.postTranslate(centerX, centerY); } }
怎么使用的,我就两个button布局
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:gravity="center" android:id="@+id/layout" tools:context="com.example.rotate3d.MainActivity"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:onClick="rotate" android:text="Hello World!" /> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:onClick="buttonRotate" android:text="Hello World!" /> </LinearLayout>
mainActivity里,基于上面的坑,只能使用时再获取控件宽和高了
import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.support.v7.widget.LinearLayoutCompat; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.LinearLayout; public class MainActivity extends AppCompatActivity { LinearLayout layout; Button button; boolean isback = false; Rotate3dAnimation animation; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); layout = (LinearLayout) findViewById(R.id.layout); button = (Button)findViewById(R.id.button); } @Override protected void onResume() { super.onResume(); Log.i("slack",layout.getWidth()+","+layout.getHeight()); } public void rotate(View view) { float centerX = layout.getWidth() / 2f; float centerY = layout.getHeight() / 2f; Log.i("slack",layout.getWidth()+","+layout.getHeight()); Log.i("slack",centerX+","+centerY); if(isback){ animation = new Rotate3dAnimation(180, 360, centerX, centerY , 100.0f, false); }else{ animation = new Rotate3dAnimation(0, 180, centerX, centerY , 100.0f, false); } animation.setDuration(1000);//动画持续时间设为500毫秒 animation.setFillAfter(true);//动画完成后保持完成的状态 layout.startAnimation(animation); isback = !isback; } public void buttonRotate(View view) { /** * 重点是两个参数 float centerX, float centerY * 动画都是建立在某一个视图View上的,所以这个 坐标参数是 * 以要动画的view 为坐标系里的坐标 * 比如下面这个只是单个的view以中轴为中心旋转,关键是x轴 * 这里的x轴是这个button的坐标系里的中心 button.getWidth()/2f * y轴无所谓,因为是绕着Y轴旋转的 ,如下图 * - - - - - - - - - * \ (x,y) \ * \ * \ * \ \ * - - - - - - - - - - */ Rotate3dAnimation animation = new Rotate3dAnimation(0, 360, ( button.getWidth()/2f), ( button.getHeight()/2f) , 100.0f, false); animation.setDuration(1000);//动画持续时间设为500毫秒 animation.setFillAfter(true);//动画完成后保持完成的状态 button.startAnimation(animation); } }
相关文章推荐
- 3D设计 Adobe Acrobat 3D 8.1.0 英文版 下载
- jquery带翻页动画的电子杂志代码分享
- jquery 3D 标签云示例代码
- jQuery+CSS3实现3D立方体旋转效果
- jquery实现叠层3D文字特效代码分享
- Android编程实现3D滑动旋转效果的方法
- 基于Android实现3D翻页效果
- Android编程实现3D旋转效果实例
- Android利用Camera实现中轴3D卡牌翻转效果
- 一个很有趣3D球状标签云兼容IE8
- javascript实现3D变换的立体圆圈实例
- 基于css3新属性transform及原生js实现鼠标拖动3d立方体旋转
- 原生javascript+css3编写的3D魔方动画旋扭特效
- JS实现3D图片旋转展示效果代码
- js实现3D图片逐张轮播幻灯片特效代码分享
- Ubuntu 3D 桌面
- CSS3中3D综合应用及分析
- Unity3D上路_01-2D太空射击游戏
- Unity3D上路_02-第一视角射击游戏
- Unity3D上路_03-塔防游戏