IDEA下创建Maven,Servlet,JSP,MYSQL项目
2016-06-28 23:01
671 查看
引用jar包
Maven: javax.servlet:javax.servlet-api:3.1.0(Servlet)
Maven: javax.servlet.jsp:jsp-api:2.2(JSP)
Maven: org.slf4j:slf4j-log4j12:1.7.7(日志包)
Maven: commons-dbutils:commons-dbutils:1.6(JDBC封装包)
Maven: org.apache.commons:commons-dbcp2:2.0.1(数据库连接池封装包)
Maven: junit:junit:4.12(单元测试包)
Maven: javax.servlet:jstl:1.2(JSTL)
Maven: mysql:mysql-connector-java:5.1.33(mysql连接包)
Maven: org.apache.commons:commons-collections4:4.0(常用工具类)
Maven: org.apache.commons:commons-lang3:3.3.2(常用工具类)
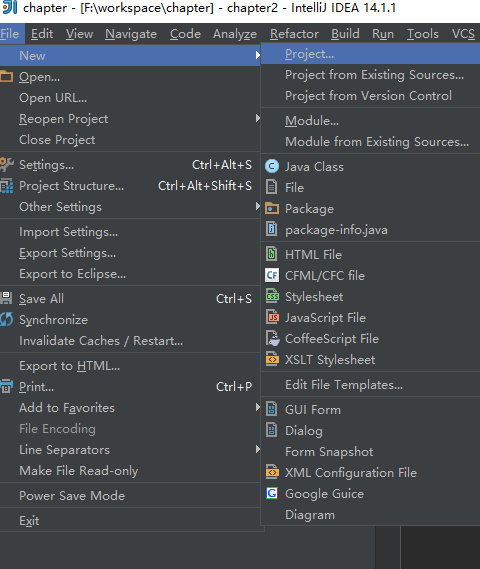
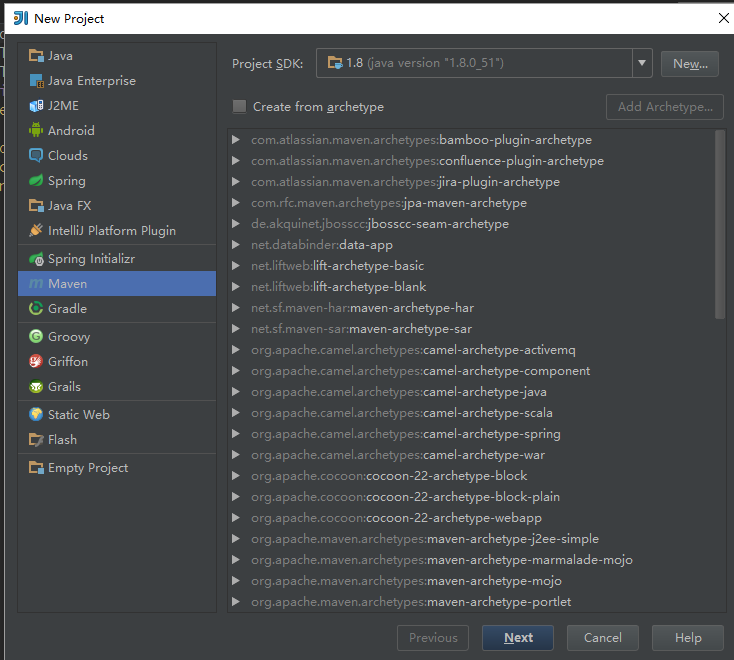
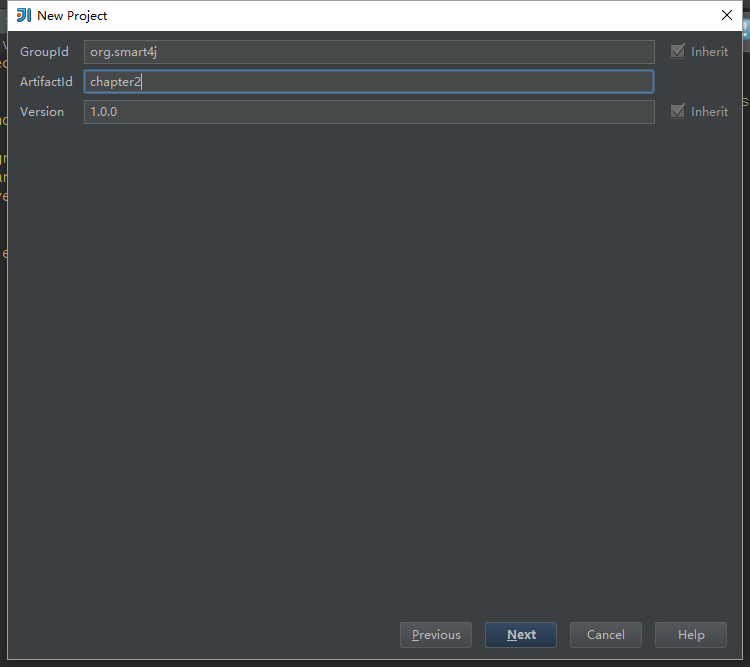
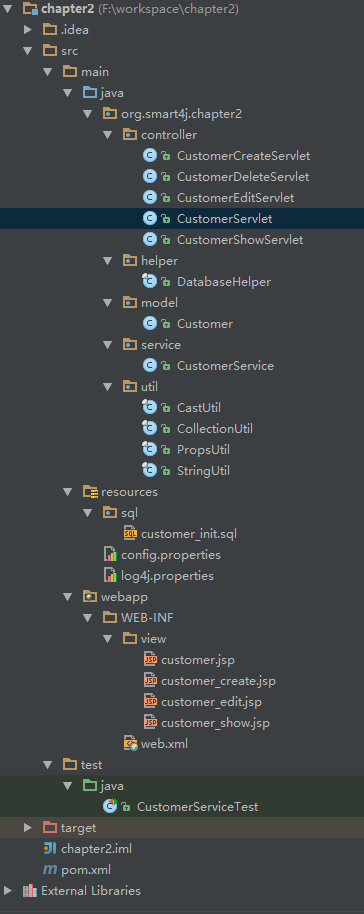
pom.xml代码
Mysql配置
customer.jsp
CustomerServlet.java
CustomerService.java
DatabaseHelper.java
CustomerServiceTest.java
customer_init.sql
在junit测试的时候,每次都会初始化表数据,只要mysql有demo_test这个数据库。
只贴出关键代码。要看全部代码,点击下载代码即可。
推荐一个影视公众号,感兴趣的童鞋可以顺便关注下~
Maven: javax.servlet:javax.servlet-api:3.1.0(Servlet)
Maven: javax.servlet.jsp:jsp-api:2.2(JSP)
Maven: org.slf4j:slf4j-log4j12:1.7.7(日志包)
Maven: commons-dbutils:commons-dbutils:1.6(JDBC封装包)
Maven: org.apache.commons:commons-dbcp2:2.0.1(数据库连接池封装包)
Maven: junit:junit:4.12(单元测试包)
Maven: javax.servlet:jstl:1.2(JSTL)
Maven: mysql:mysql-connector-java:5.1.33(mysql连接包)
Maven: org.apache.commons:commons-collections4:4.0(常用工具类)
Maven: org.apache.commons:commons-lang3:3.3.2(常用工具类)
新建项目
选择maven
命名
创建目录结构为下图所示
pom.xml代码
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.smart4j</groupId> <artifactId>chapter2</artifactId> <version>1.0.0</version> <packaging>war</packaging> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <build> <plugins> <!-- Compile--> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.3</version> <configuration> <source>1.8</source> <target>1.8</target> </configuration> </plugin> <!-- Test --> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <version>2.18.1</version> <configuration> <skipTests>false</skipTests> </configuration> </plugin> <!-- Tomcat --> <plugin> <groupId>org.apache.tomcat.maven</groupId> <artifactId>tomcat7-maven-plugin</artifactId> <version>2.2</version> <configuration> <path>/${project.artifactId}</path> </configuration> </plugin> </plugins> </build> <dependencies> <!-- Servlet --> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.1.0</version> <scope>provided</scope> </dependency> <!-- jsp --> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>jsp-api</artifactId> <version>2.2</version> <scope>provided</scope> </dependency> <!-- JSTL --> <dependency> <groupId>javax.servlet</groupId> <artifactId>jstl</artifactId> <version>1.2</version> <scope>runtime</scope> </dependency> <!-- JUnit --> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> <scope>test</scope> </dependency> <!--JSTL4J--> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-log4j12</artifactId> <version>1.7.7</version> </dependency> <!-- MySQL --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.33</version> <scope>runtime</scope> </dependency> <!-- Apache Commons Lang--> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-lang3</artifactId> <version>3.3.2</version> </dependency> <!-- Apache Commons Collections--> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-collections4</artifactId> <version>4.0</version> </dependency> <!-- Apache Commons Dbutils--> <dependency> <groupId>commons-dbutils</groupId> <artifactId>commons-dbutils</artifactId> <version>1.6</version> </dependency> <!-- Apache Commons DBCP2 --> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-dbcp2</artifactId> <version>2.0.1</version> </dependency> </dependencies> </project>
Mysql配置
jdbc.driver=com.mysql.jdbc.Driver jdbc.url=jdbc:mysql://localhost:3306/demo_test jdbc.username=root jdbc.password=root
customer.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <c:set var="BASE" value="${pageContext.request.contextPath}"/> <html> <head> <title>customer manager</title> </head> <body> <h1>CustomerList</h1> <table> <tr> <th>name</th> <th>contact</th> <th>telephone</th> <th>email</th> <th>edit</th> </tr> <c:forEach var="customer" items="${customerList}"> <tr> <th>${customer.name}</th> <th>${customer.contact}</th> <th>${customer.telephone}</th> <th>${customer.email}</th> <th> <a href="${BASE}/customer_edit?id=${customer.id}">edit</a> <a href="${BASE}/customer_delete?id=${customer.id}">delete</a> </th> </tr> </c:forEach> </table> </body> </html>
CustomerServlet.java
@WebServlet("/customer") public class CustomerServlet extends HttpServlet{ private CustomerService customerService; @Override public void init() throws ServletException { customerService = new CustomerService(); } @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { List<Customer> customerList = customerService.getCustomerList(); req.setAttribute("customerList",customerList); req.getRequestDispatcher("WEB-INF/view/customer.jsp").forward(req,resp); } }
CustomerService.java
public class CustomerService { private static final Logger LOGGER = LoggerFactory.getLogger(CustomerService.class); /** * 获取客户列表 */ public List<Customer> getCustomerList() { String sql = "select * from customer"; return DatabaseHelper.queryEntityList(Customer.class, sql); } public Customer getCustomer(Long id) { String sql = "select * from customer where id = ? "; return DatabaseHelper.queryEntity(Customer.class,sql, id); } /** * 創建客戶 */ public boolean cre 10540 ateCustomer(Map<String, Object> fieldMap) { return DatabaseHelper.insertEntity(Customer.class,fieldMap); } /** * 更新客戶 */ public boolean updateCustomer(Long id, Map<String, Object> fieldMap) { return DatabaseHelper.updateEntity(Customer.class,id,fieldMap); } /** * 刪除客戶 */ public boolean deleteCustomer(Long id) { return DatabaseHelper.deleteEntity(Customer.class,id); } }
DatabaseHelper.java
public final class DatabaseHelper { private static final Logger LOGGER = LoggerFactory.getLogger(DatabaseHelper.class); private static final QueryRunner QUERY_RUNNER ; private static final ThreadLocal<Connection> CONNECTION_HOLDER; private static final BasicDataSource DATA_SOURCE; static { CONNECTION_HOLDER = new ThreadLocal<Connection>(); QUERY_RUNNER = new QueryRunner(); Properties conf = PropsUtil.loadProps("config.properties"); String driver = conf.getProperty("jdbc.driver"); String url = conf.getProperty("jdbc.url"); String username = conf.getProperty("jdbc.username"); String password = conf.getProperty("jdbc.password"); DATA_SOURCE=new BasicDataSource(); DATA_SOURCE.setDriverClassName(driver); DATA_SOURCE.setUrl(url); DATA_SOURCE.setUsername(username); DATA_SOURCE.setPassword(password); } /** * 连接数据库 */ public static Connection getConnection() { Connection conn = CONNECTION_HOLDER.get(); if(conn==null){ try { conn = DATA_SOURCE.getConnection(); } catch (SQLException e) { LOGGER.error("get connection failure", e); throw new RuntimeException(e); } finally { CONNECTION_HOLDER.set(conn); } } return conn; } /** * 查询实体列表 */ public static <T> List<T> queryEntityList(Class<T> entityClass, String sql,Object... params) { List<T> entityList; try { Connection conn=getConnection(); entityList = QUERY_RUNNER.query(conn, sql, new BeanListHandler<T>(entityClass),params); } catch (SQLException e) { LOGGER.error("query entity list failure", e); throw new RuntimeException(e); } return entityList; } /** * 查询实体 */ public static <T>T queryEntity(Class<T> entityClass,String sql,Object...params){ T entity; try{ Connection conn=getConnection(); entity=QUERY_RUNNER.query(conn,sql,new BeanHandler<T>(entityClass),params); }catch (SQLException e){ LOGGER.error("query entity failure",e); throw new RuntimeException(e); } return entity; } /** * 执行查询语句 */ public static List<Map<String,Object>> executeQuery(String sql,Object...params){ List<Map<String,Object>> list; try{ Connection conn=getConnection(); list = QUERY_RUNNER.query(conn, sql, new MapListHandler(), params); }catch (SQLException e){ LOGGER.error("execute query failure",e); throw new RuntimeException(e); } return list; } /** * 执行更新语句 */ public static int executeUpdate(String sql,Object...params){ int result=0; try{ Connection conn =getConnection(); result = QUERY_RUNNER.update(conn, sql, params); }catch (SQLException e){ LOGGER.error("execute update failure",e); throw new RuntimeException(e); } return result; } /** * 插入实体 */ public static <T> boolean insertEntity(Class<T> entityClass,Map<String,Object> fieldMap){ if(CollectionUtil.isEmpty(fieldMap)){ LOGGER.error("can not insert entity:fieldMap is empty"); return false; } String sql="insert into " +getTableName(entityClass); StringBuilder columns=new StringBuilder("("); StringBuilder values=new StringBuilder("("); for(String fieldName:fieldMap.keySet()){ columns.append(fieldName).append(", "); values.append("?, "); } columns.replace(columns.lastIndexOf(", "),columns.length(),")"); values.replace(values.lastIndexOf(", "),values.length(),")"); sql += columns +"values" +values; Object[] params=fieldMap.values().toArray(); return executeUpdate(sql, params) == 1; } /** * 更新实体 */ public static <T> boolean updateEntity(Class<T> entityClass,Long id,Map<String,Object> fieldMap){ if(CollectionUtil.isEmpty(fieldMap)){ LOGGER.error("can not update entity:fieldMap is empty"); return false; } String sql="update "+getTableName(entityClass) +" set "; StringBuilder columns =new StringBuilder(); for(String fieldName:fieldMap.keySet()){ columns.append(fieldName).append("=?, "); } sql +=columns.substring(0,columns.lastIndexOf(", "))+" where id=?"; List<Object> paramList =new ArrayList<Object>(); paramList.addAll(fieldMap.values()); paramList.add(id); Object[] params=paramList.toArray(); return executeUpdate(sql,params)==1; } /** * 删除实体 */ public static <T>boolean deleteEntity(Class<T> enetityClass,long id){ String sql = "delete from "+getTableName(enetityClass) +" where id= ?"; return executeUpdate(sql,id)==1; } private static String getTableName(Class<?> entityClass) { return entityClass.getSimpleName(); } /** * 執行sql語句 */ public static void executeSqlFile(String filePath){ InputStream is = Thread.currentThread().getContextClassLoader().getResourceAsStream(filePath); BufferedReader reader = new BufferedReader(new InputStreamReader(is)); try { String sql; while ((sql=reader.readLine()) !=null){ executeUpdate(sql); } } catch (IOException e) { LOGGER.error("execute sql file failure",e); throw new RuntimeException(e); } } }
CustomerServiceTest.java
public class CustomerServiceTest { private final CustomerService customerService; public CustomerServiceTest(){ customerService = new CustomerService(); } @Before public void init() throws Exception{ DatabaseHelper.executeSqlFile("sql/customer_init.sql"); } @Test public void getCustomerListTest() throws Exception{ List<Customer> customerList = customerService.getCustomerList(); Assert.assertEquals(2, customerList.size()); } @Test public void getCustomerTest() throws Exception{ long id = 1; Customer customer = customerService.getCustomer(id); Assert.assertNotNull(customer); } @Test public void createCustomerTest() throws Exception{ Map<String,Object> map = new HashMap<String,Object>(); map.put("name","customer100"); map.put("contact","Jonn"); map.put("telephone","123456454542"); boolean result = customerService.createCustomer(map); Assert.assertTrue(result); } @Test public void updateCustomerTest() throws Exception{ long id = 1; Map<String,Object> map = new HashMap<String,Object>(); map.put("contact", "Eric"); boolean result = customerService.updateCustomer(id, map); Assert.assertTrue(result); } @Test public void deleteCustomerTest() throws Exception{ long id = 2; boolean result = customerService.deleteCustomer(id); Assert.assertTrue(result); } }
customer_init.sql
在junit测试的时候,每次都会初始化表数据,只要mysql有demo_test这个数据库。
TRUNCATE customer; INSERT INTO customer (name,contact,telephone,email,remark) VALUES ('customer1','jack','18817578888','123@qq.com',NULL); INSERT INTO customer (name,contact,telephone,email,remark) VALUES ('customer2','Rose','15261111111','321@11.com',NULL);
只贴出关键代码。要看全部代码,点击下载代码即可。
推荐一个影视公众号,感兴趣的童鞋可以顺便关注下~