D3D中的光照(3)
2016-06-20 00:00
239 查看
平行光示例:
The GetAsyncKeyState function determines whether a key is up or down at the time the function is called, and whether the key was pressed after a previous call to GetAsyncKeyState.
Syntax
Parameters
vKey
[in] Specifies one of 256 possible virtual-key codes. For more information, see Virtual-Key Codes.
Return Value
If the function succeeds, the return value specifies whether the key was pressed since the last call to GetAsyncKeyState, and whether the key is currently up or down. If the most significant bit is set, the key is down, and if the least significant bit is set, the key was pressed after the previous call to GetAsyncKeyState. However, you should not rely on this last behavior; for more information, see the Remarks.
截图:
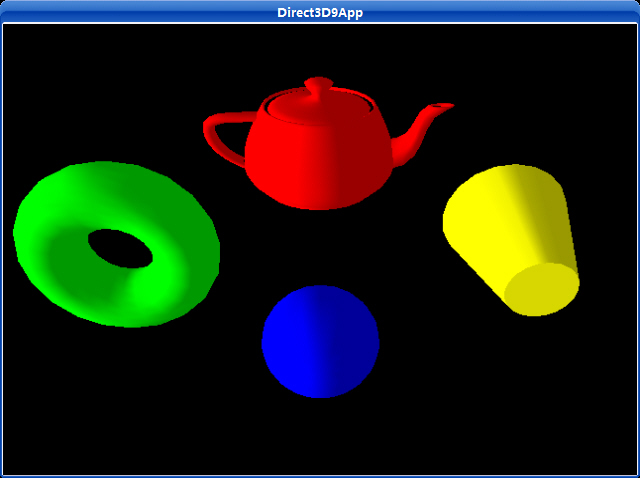
源代码:
/**************************************************************************************
Demonstrates using a directional light with D3DX objects.
You can orbit the scene using the left and right arrow keys.
In addition you can elevate the camera with the up and down arrow keys.
**************************************************************************************/
#include "d3dUtility.h"
#pragma warning(disable : 4100)
#define MESH_TEAPOT 0
#define MESH_SPHERE 1
#define MESH_TORUS 2
#define MESH_CYLINDER 3
#define NUM_MESH 4
const int WIDTH = 640;
const int HEIGHT = 480;
IDirect3DDevice9* g_d3d_device = NULL;
ID3DXMesh* g_object_meshes[NUM_MESH];
D3DXMATRIX g_world_matrices[NUM_MESH];
D3DMATERIAL9 g_materials[NUM_MESH];
////////////////////////////////////////////////////////////////////////////////////////////////////
bool setup()
{
// create objects
D3DXCreateTeapot(g_d3d_device, &g_object_meshes[MESH_TEAPOT], NULL);
D3DXCreateSphere(g_d3d_device, 1.0f, 20, 20, &g_object_meshes[MESH_SPHERE], NULL);
D3DXCreateTorus(g_d3d_device, 0.5f, 1.0f, 20, 20, &g_object_meshes[MESH_TORUS], NULL);
D3DXCreateCylinder(g_d3d_device, 0.5f, 1.0f, 2.0f, 20, 20, &g_object_meshes[MESH_CYLINDER], NULL);
// build world matrices - position the objects in world space
D3DXMatrixTranslation(&g_world_matrices[MESH_TEAPOT], 0.0f, 2.0f, 0.0f);
D3DXMatrixTranslation(&g_world_matrices[MESH_SPHERE], 0.0f, -2.0f, 0.0f);
D3DXMatrixTranslation(&g_world_matrices[MESH_TORUS], -3.0f, 0.0f, 0.0f);
D3DXMatrixTranslation(&g_world_matrices[MESH_CYLINDER], 3.0f, 0.0f, 0.0f);
// setup the object's materials
g_materials[MESH_TEAPOT] = RED_MATERIAL;
g_materials[MESH_SPHERE] = BLUE_MATERIAL;
g_materials[MESH_TORUS] = GREEN_MATERIAL;
g_materials[MESH_CYLINDER] = YELLOW_MATERIAL;
// setup a directional light
D3DXVECTOR3 light_direction(1.0f, -0.0f, 0.25f);
D3DXCOLOR color = WHITE;
D3DLIGHT9 dir_light = init_directional_light(&light_direction, &color);
// set and enable the light
g_d3d_device->SetLight(0, &dir_light);
g_d3d_device->LightEnable(0, TRUE);
// turn off specular lighting and instruct Direct3D to renormalize normals
g_d3d_device->SetRenderState(D3DRS_NORMALIZENORMALS, TRUE);
g_d3d_device->SetRenderState(D3DRS_SPECULARENABLE, FALSE);
// set the projection matrix
D3DXMATRIX proj;
D3DXMatrixPerspectiveFovLH(&proj, D3DX_PI * 0.25f, (float)WIDTH/HEIGHT, 1.0f, 1000.0f);
g_d3d_device->SetTransform(D3DTS_PROJECTION, &proj);
return true;
}
void cleanup()
{
for(int i = 0; i < NUM_MESH; i++)
safe_release<ID3DXMesh*>(g_object_meshes[i]);
}
bool display(float time_delta)
{
// update the scene: update camera position
static float angle = (3.0f * D3DX_PI) / 2.0f;
static float height = 5.0f;
if(GetAsyncKeyState(VK_LEFT) & 0x8000f)
angle -= 0.5f * time_delta;
if(GetAsyncKeyState(VK_RIGHT) & 0x8000f)
angle += 0.5f * time_delta;
if(GetAsyncKeyState(VK_UP) & 0x8000f)
height += 5.0f * time_delta;
if(GetAsyncKeyState(VK_DOWN) & 0x8000f)
height -= 5.0f * time_delta;
D3DXVECTOR3 position(cosf(angle) * 7.0f, height, sinf(angle) * 7.0f);
D3DXVECTOR3 target(0.0f, 0.0f, 0.0f);
D3DXVECTOR3 up(0.0f, 1.0f, 0.0f);
D3DXMATRIX view_matrix;
D3DXMatrixLookAtLH(&view_matrix, &position, &target, &up);
g_d3d_device->SetTransform(D3DTS_VIEW, &view_matrix);
// draw the scene
g_d3d_device->Clear(0, NULL, D3DCLEAR_TARGET | D3DCLEAR_ZBUFFER, 0x00000000, 1.0f, 0);
g_d3d_device->BeginScene();
for(int i = 0; i < NUM_MESH; i++)
{
// set material and world matrix for ith object, then render the ith object.
g_d3d_device->SetMaterial(&g_materials[i]);
g_d3d_device->SetTransform(D3DTS_WORLD, &g_world_matrices[i]);
g_object_meshes[i]->DrawSubset(0);
}
g_d3d_device->EndScene();
g_d3d_device->Present(NULL, NULL, NULL, NULL);
return true;
}
LRESULT CALLBACK wnd_proc(HWND hwnd, UINT msg, WPARAM word_param, LPARAM long_param)
{
switch(msg)
{
case WM_DESTROY:
PostQuitMessage(0);
break;
case WM_KEYDOWN:
if(word_param == VK_ESCAPE)
DestroyWindow(hwnd);
break;
}
return DefWindowProc(hwnd, msg, word_param, long_param);
}
int WINAPI WinMain(HINSTANCE inst, HINSTANCE, PSTR cmd_line, int cmd_show)
{
if(! init_d3d(inst, WIDTH, HEIGHT, true, D3DDEVTYPE_HAL, &g_d3d_device))
{
MessageBox(NULL, "init_d3d() - failed.", 0, MB_OK);
return 0;
}
if(! setup())
{
MessageBox(NULL, "Steup() - failed.", 0, MB_OK);
return 0;
}
enter_msg_loop(display);
cleanup();
g_d3d_device->Release();
return 0;
}
下载源程序
The GetAsyncKeyState function determines whether a key is up or down at the time the function is called, and whether the key was pressed after a previous call to GetAsyncKeyState.
Syntax
SHORT GetAsyncKeyState( int vKey );
Parameters
vKey
[in] Specifies one of 256 possible virtual-key codes. For more information, see Virtual-Key Codes.
Return Value
If the function succeeds, the return value specifies whether the key was pressed since the last call to GetAsyncKeyState, and whether the key is currently up or down. If the most significant bit is set, the key is down, and if the least significant bit is set, the key was pressed after the previous call to GetAsyncKeyState. However, you should not rely on this last behavior; for more information, see the Remarks.
截图:
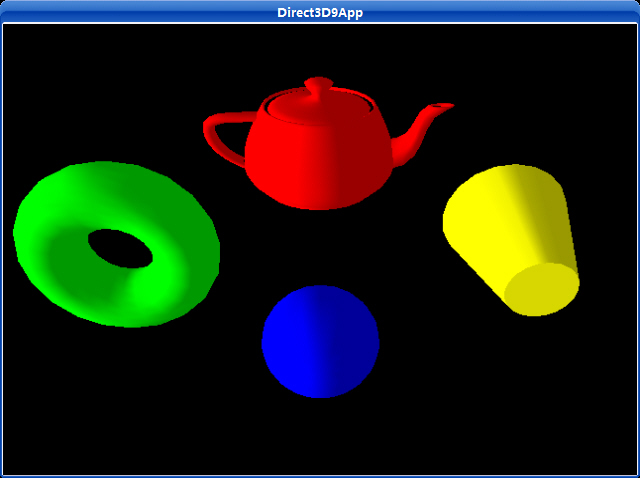
源代码:
/**************************************************************************************
Demonstrates using a directional light with D3DX objects.
You can orbit the scene using the left and right arrow keys.
In addition you can elevate the camera with the up and down arrow keys.
**************************************************************************************/
#include "d3dUtility.h"
#pragma warning(disable : 4100)
#define MESH_TEAPOT 0
#define MESH_SPHERE 1
#define MESH_TORUS 2
#define MESH_CYLINDER 3
#define NUM_MESH 4
const int WIDTH = 640;
const int HEIGHT = 480;
IDirect3DDevice9* g_d3d_device = NULL;
ID3DXMesh* g_object_meshes[NUM_MESH];
D3DXMATRIX g_world_matrices[NUM_MESH];
D3DMATERIAL9 g_materials[NUM_MESH];
////////////////////////////////////////////////////////////////////////////////////////////////////
bool setup()
{
// create objects
D3DXCreateTeapot(g_d3d_device, &g_object_meshes[MESH_TEAPOT], NULL);
D3DXCreateSphere(g_d3d_device, 1.0f, 20, 20, &g_object_meshes[MESH_SPHERE], NULL);
D3DXCreateTorus(g_d3d_device, 0.5f, 1.0f, 20, 20, &g_object_meshes[MESH_TORUS], NULL);
D3DXCreateCylinder(g_d3d_device, 0.5f, 1.0f, 2.0f, 20, 20, &g_object_meshes[MESH_CYLINDER], NULL);
// build world matrices - position the objects in world space
D3DXMatrixTranslation(&g_world_matrices[MESH_TEAPOT], 0.0f, 2.0f, 0.0f);
D3DXMatrixTranslation(&g_world_matrices[MESH_SPHERE], 0.0f, -2.0f, 0.0f);
D3DXMatrixTranslation(&g_world_matrices[MESH_TORUS], -3.0f, 0.0f, 0.0f);
D3DXMatrixTranslation(&g_world_matrices[MESH_CYLINDER], 3.0f, 0.0f, 0.0f);
// setup the object's materials
g_materials[MESH_TEAPOT] = RED_MATERIAL;
g_materials[MESH_SPHERE] = BLUE_MATERIAL;
g_materials[MESH_TORUS] = GREEN_MATERIAL;
g_materials[MESH_CYLINDER] = YELLOW_MATERIAL;
// setup a directional light
D3DXVECTOR3 light_direction(1.0f, -0.0f, 0.25f);
D3DXCOLOR color = WHITE;
D3DLIGHT9 dir_light = init_directional_light(&light_direction, &color);
// set and enable the light
g_d3d_device->SetLight(0, &dir_light);
g_d3d_device->LightEnable(0, TRUE);
// turn off specular lighting and instruct Direct3D to renormalize normals
g_d3d_device->SetRenderState(D3DRS_NORMALIZENORMALS, TRUE);
g_d3d_device->SetRenderState(D3DRS_SPECULARENABLE, FALSE);
// set the projection matrix
D3DXMATRIX proj;
D3DXMatrixPerspectiveFovLH(&proj, D3DX_PI * 0.25f, (float)WIDTH/HEIGHT, 1.0f, 1000.0f);
g_d3d_device->SetTransform(D3DTS_PROJECTION, &proj);
return true;
}
void cleanup()
{
for(int i = 0; i < NUM_MESH; i++)
safe_release<ID3DXMesh*>(g_object_meshes[i]);
}
bool display(float time_delta)
{
// update the scene: update camera position
static float angle = (3.0f * D3DX_PI) / 2.0f;
static float height = 5.0f;
if(GetAsyncKeyState(VK_LEFT) & 0x8000f)
angle -= 0.5f * time_delta;
if(GetAsyncKeyState(VK_RIGHT) & 0x8000f)
angle += 0.5f * time_delta;
if(GetAsyncKeyState(VK_UP) & 0x8000f)
height += 5.0f * time_delta;
if(GetAsyncKeyState(VK_DOWN) & 0x8000f)
height -= 5.0f * time_delta;
D3DXVECTOR3 position(cosf(angle) * 7.0f, height, sinf(angle) * 7.0f);
D3DXVECTOR3 target(0.0f, 0.0f, 0.0f);
D3DXVECTOR3 up(0.0f, 1.0f, 0.0f);
D3DXMATRIX view_matrix;
D3DXMatrixLookAtLH(&view_matrix, &position, &target, &up);
g_d3d_device->SetTransform(D3DTS_VIEW, &view_matrix);
// draw the scene
g_d3d_device->Clear(0, NULL, D3DCLEAR_TARGET | D3DCLEAR_ZBUFFER, 0x00000000, 1.0f, 0);
g_d3d_device->BeginScene();
for(int i = 0; i < NUM_MESH; i++)
{
// set material and world matrix for ith object, then render the ith object.
g_d3d_device->SetMaterial(&g_materials[i]);
g_d3d_device->SetTransform(D3DTS_WORLD, &g_world_matrices[i]);
g_object_meshes[i]->DrawSubset(0);
}
g_d3d_device->EndScene();
g_d3d_device->Present(NULL, NULL, NULL, NULL);
return true;
}
LRESULT CALLBACK wnd_proc(HWND hwnd, UINT msg, WPARAM word_param, LPARAM long_param)
{
switch(msg)
{
case WM_DESTROY:
PostQuitMessage(0);
break;
case WM_KEYDOWN:
if(word_param == VK_ESCAPE)
DestroyWindow(hwnd);
break;
}
return DefWindowProc(hwnd, msg, word_param, long_param);
}
int WINAPI WinMain(HINSTANCE inst, HINSTANCE, PSTR cmd_line, int cmd_show)
{
if(! init_d3d(inst, WIDTH, HEIGHT, true, D3DDEVTYPE_HAL, &g_d3d_device))
{
MessageBox(NULL, "init_d3d() - failed.", 0, MB_OK);
return 0;
}
if(! setup())
{
MessageBox(NULL, "Steup() - failed.", 0, MB_OK);
return 0;
}
enter_msg_loop(display);
cleanup();
g_d3d_device->Release();
return 0;
}
下载源程序
相关文章推荐
- hypervisor与VMware共存方法
- 终于弄到个百度Hi的邀请
- Lua脚本语法说明(修订)
- 多线程 C#解决方案小结
- 这样软件开发人才级别的划分你同意吗(ZT)
- WQL Analyzer V1.1(WMI查询分析器)
- 如何调用未公开的API函数[转]
- 何编写网络监视器
- 25种成为百万富翁的方法
- 易中天与单田芳的区别在哪儿
- 用DirectX Audio和DirectShow播放声音和音乐(4)
- 不要让捐款变了味
- 【ZT】中西医的区别
- Nebula3学习笔记(7): 脚本系统
- IntelliJ IDEA 使用总结[zz]
- 立法禁食猫狗肉属本末倒置
- OGame银河系说明
- AVP主动防御之隐藏进程
- 不会“思维”只会“批判”,谨防网络舆论“怨妇化”
- DirectX 9高层着色语言介绍3——语言基础(2)