Android入门--返回数据到前一个Activity--startActivityForResult 方法
2016-06-13 10:42
597 查看
activity_forward.xml页面代码如下:<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.hanji.forward.Forward" >
<TextView
android:id="@+id/showText"
android:layout_width="wrap_content"
android:layout_height="26px"
android:text="计算你的标准体重!"
android:textSize="20px" >
</TextView>
<TextView
android:id="@+id/text_Height"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/showText"
android:layout_marginTop="20dp"
android:text="身高:" >
</TextView>
<EditText
android:id="@+id/height_Edit"
android:layout_width="123px"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/text_Height"
android:layout_alignBottom="@+id/text_Height"
android:layout_marginLeft="20dp"
android:layout_toRightOf="@+id/text_Height"
android:textSize="18sp" >
</EditText>
<TextView
android:id="@+id/text_Sex"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/text_Height"
android:layout_marginTop="20dp"
android:text="性别:" >
</TextView>
<RadioGroup
android:id="@+id/radioGroup"
android:layout_width="wrap_content"
android:layout_height="37px"
android:layout_alignBaseline="@+id/text_Sex"
android:layout_alignBottom="@+id/text_Sex"
android:layout_marginLeft="20dp"
android:layout_toRightOf="@+id/text_Sex"
android:orientation="horizontal" >
<RadioButton
android:id="@+id/Sex_Man"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="男" >
</RadioButton>
<RadioButton
android:id="@+id/Sex_Woman"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="女" >
</RadioButton>
</RadioGroup>
<Button
android:id="@+id/button_OK"
android:layout_width="160px"
android:layout_height="wrap_content"
android:layout_below="@+id/text_Sex"
android:layout_marginTop="20dp"
android:text="计算" />
</RelativeLayout>
Forward.java页面代码如下:package com.hanji.forward;
import android.support.v7.app.ActionBarActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.RadioButton;
import android.widget.Toast;
public class Forward extends ActionBarActivity {
protected int my_requestCode = 1550;
private EditText edit_height;
private RadioButton radiobutton_Man, radiobutton_Woman;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
/* 载入main.xml Layout */
setContentView(R.layout.activity_forward);
/* 以findViewById()取得Button 对象,并添加onClickListener */
Button ok = (Button) findViewById(R.id.button_OK);
edit_height = (EditText) findViewById(R.id.height_Edit);
radiobutton_Man = (RadioButton) findViewById(R.id.Sex_Man);
radiobutton_Woman = (RadioButton) findViewById(R.id.Sex_Woman);
ok.setOnClickListener(new Button.OnClickListener() {
public void onClick(View v) {
try {
/* 取得输入的身高 */
EditText et = (EditText) findViewById(R.id.height_Edit);
double height = Double.parseDouble(et.getText().toString());
/* 取得选择的性别 */
String sex = "";
RadioButton rb1 = (RadioButton) findViewById(R.id.Sex_Man);
if (rb1.isChecked()) {
sex = "M";
} else {
sex = "F";
} /*
* new 一 个 Intent 对 象 , 并 指 定 class
*/
Intent intent = new Intent();
intent.setClass(Forward.this, ForwardTarget.class);
/* new 一个Bundle对象,并将要传递的数据传入 */
Bundle bundle = new Bundle();
bundle.putDouble("height", height);
bundle.putString("sex", sex);
/* 将Bundle 对象assign 给Intent */
intent.putExtras(bundle);
/* 调用Activity ForwardTarget */
startActivityForResult(intent, my_requestCode);
} catch (Exception e) {
// TODO: handle exception
Toast.makeText(Forward.this, R.string.errorString,
Toast.LENGTH_LONG).show();
}
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
// TODO Auto-generated method stub
super.onActivityResult(requestCode, resultCode, data);
switch (resultCode) {
case RESULT_OK:
/* 取得来自Activity2 的数据,并显示于画面上 */
Bundle bunde = data.getExtras();
String sex = bunde.getString("sex");
double height = bunde.getDouble("height");
edit_height.setText("" + height);
if (sex.equals("M")) {
radiobutton_Man.setChecked(true);
} else {
radiobutton_Woman.setChecked(true);
}
break;
default:
break;
}
}
}
activity_forward_target.xml页面代码如下:<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.hanji.forward.ForwardTarget" >
<TextView
android:id="@+id/text1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="20sp">
</TextView>
<Button
android:id="@+id/button_OK"
android:layout_width="160px"
android:layout_height="wrap_content"
android:layout_below="@+id/text1"
android:layout_marginTop="20dp"
android:text="返回" />
</RelativeLayout>
ForwardTarget.java页面代码如下:package com.hanji.forward;
import java.text.DecimalFormat;
import java.text.NumberFormat;
import android.support.v7.app.ActionBarActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class ForwardTarget extends ActionBarActivity {
private Intent intent;
private Bundle bunde;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
/* 加载main.xml Layout */
setContentView(R.layout.activity_forward_target);
/* 取得Intent 中的Bundle 对象 */
intent = this.getIntent();
bunde = this.getIntent().getExtras();
/* 取得Bundle 对象中的数据 */
String sex = bunde.getString("sex");
double height = bunde.getDouble("height");
/* 判断性别 */
String sexText = "";
if (sex.equals("M")) {
sexText = "男性";
} else {
sexText = "女性";
}
/* 取得标准体重 */
String weight = this.getWeight(sex, height);
/* 设置输出文字 */
TextView tv1 = (TextView) findViewById(R.id.text1);
tv1.setText("你是一位" + sexText + "\n你的身高是" + height + "厘米\n你的标准体重是"
+ weight + "公斤");
/* 返回事件 */
Button ok = (Button) findViewById(R.id.button_OK);
ok.setOnClickListener(new Button.OnClickListener() {
public void onClick(View v) {
setResult(RESULT_OK, intent);
finish();
}
});
}
/* 四舍五入的method */
private String format(double num) {
NumberFormat formatter = new DecimalFormat("0.00");
String s = formatter.format(num);
return s;
}
/* 计算标准体重 */
private String getWeight(String sex, double height) {
String weight = "";
if (sex.equals("M")) {
weight = format((height - 80) * 0.7);
} else {
weight = format((height - 70) * 0.6);
}
return weight;
}
}
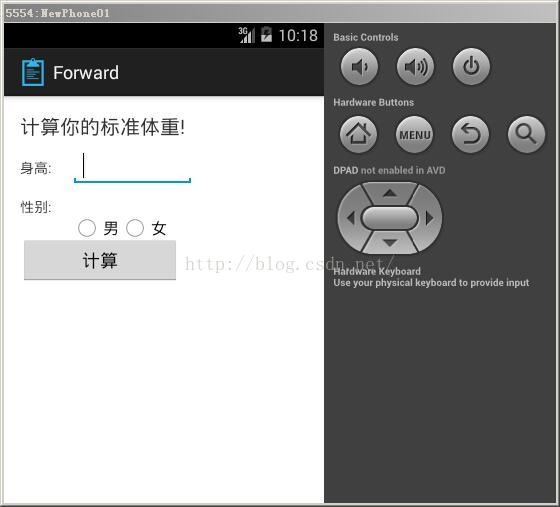
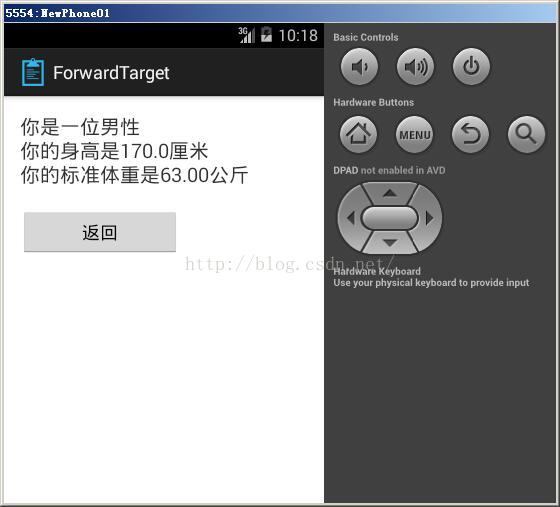
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.hanji.forward.Forward" >
<TextView
android:id="@+id/showText"
android:layout_width="wrap_content"
android:layout_height="26px"
android:text="计算你的标准体重!"
android:textSize="20px" >
</TextView>
<TextView
android:id="@+id/text_Height"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/showText"
android:layout_marginTop="20dp"
android:text="身高:" >
</TextView>
<EditText
android:id="@+id/height_Edit"
android:layout_width="123px"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/text_Height"
android:layout_alignBottom="@+id/text_Height"
android:layout_marginLeft="20dp"
android:layout_toRightOf="@+id/text_Height"
android:textSize="18sp" >
</EditText>
<TextView
android:id="@+id/text_Sex"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/text_Height"
android:layout_marginTop="20dp"
android:text="性别:" >
</TextView>
<RadioGroup
android:id="@+id/radioGroup"
android:layout_width="wrap_content"
android:layout_height="37px"
android:layout_alignBaseline="@+id/text_Sex"
android:layout_alignBottom="@+id/text_Sex"
android:layout_marginLeft="20dp"
android:layout_toRightOf="@+id/text_Sex"
android:orientation="horizontal" >
<RadioButton
android:id="@+id/Sex_Man"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="男" >
</RadioButton>
<RadioButton
android:id="@+id/Sex_Woman"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="女" >
</RadioButton>
</RadioGroup>
<Button
android:id="@+id/button_OK"
android:layout_width="160px"
android:layout_height="wrap_content"
android:layout_below="@+id/text_Sex"
android:layout_marginTop="20dp"
android:text="计算" />
</RelativeLayout>
Forward.java页面代码如下:package com.hanji.forward;
import android.support.v7.app.ActionBarActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.RadioButton;
import android.widget.Toast;
public class Forward extends ActionBarActivity {
protected int my_requestCode = 1550;
private EditText edit_height;
private RadioButton radiobutton_Man, radiobutton_Woman;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
/* 载入main.xml Layout */
setContentView(R.layout.activity_forward);
/* 以findViewById()取得Button 对象,并添加onClickListener */
Button ok = (Button) findViewById(R.id.button_OK);
edit_height = (EditText) findViewById(R.id.height_Edit);
radiobutton_Man = (RadioButton) findViewById(R.id.Sex_Man);
radiobutton_Woman = (RadioButton) findViewById(R.id.Sex_Woman);
ok.setOnClickListener(new Button.OnClickListener() {
public void onClick(View v) {
try {
/* 取得输入的身高 */
EditText et = (EditText) findViewById(R.id.height_Edit);
double height = Double.parseDouble(et.getText().toString());
/* 取得选择的性别 */
String sex = "";
RadioButton rb1 = (RadioButton) findViewById(R.id.Sex_Man);
if (rb1.isChecked()) {
sex = "M";
} else {
sex = "F";
} /*
* new 一 个 Intent 对 象 , 并 指 定 class
*/
Intent intent = new Intent();
intent.setClass(Forward.this, ForwardTarget.class);
/* new 一个Bundle对象,并将要传递的数据传入 */
Bundle bundle = new Bundle();
bundle.putDouble("height", height);
bundle.putString("sex", sex);
/* 将Bundle 对象assign 给Intent */
intent.putExtras(bundle);
/* 调用Activity ForwardTarget */
startActivityForResult(intent, my_requestCode);
} catch (Exception e) {
// TODO: handle exception
Toast.makeText(Forward.this, R.string.errorString,
Toast.LENGTH_LONG).show();
}
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
// TODO Auto-generated method stub
super.onActivityResult(requestCode, resultCode, data);
switch (resultCode) {
case RESULT_OK:
/* 取得来自Activity2 的数据,并显示于画面上 */
Bundle bunde = data.getExtras();
String sex = bunde.getString("sex");
double height = bunde.getDouble("height");
edit_height.setText("" + height);
if (sex.equals("M")) {
radiobutton_Man.setChecked(true);
} else {
radiobutton_Woman.setChecked(true);
}
break;
default:
break;
}
}
}
activity_forward_target.xml页面代码如下:<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.hanji.forward.ForwardTarget" >
<TextView
android:id="@+id/text1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="20sp">
</TextView>
<Button
android:id="@+id/button_OK"
android:layout_width="160px"
android:layout_height="wrap_content"
android:layout_below="@+id/text1"
android:layout_marginTop="20dp"
android:text="返回" />
</RelativeLayout>
ForwardTarget.java页面代码如下:package com.hanji.forward;
import java.text.DecimalFormat;
import java.text.NumberFormat;
import android.support.v7.app.ActionBarActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class ForwardTarget extends ActionBarActivity {
private Intent intent;
private Bundle bunde;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
/* 加载main.xml Layout */
setContentView(R.layout.activity_forward_target);
/* 取得Intent 中的Bundle 对象 */
intent = this.getIntent();
bunde = this.getIntent().getExtras();
/* 取得Bundle 对象中的数据 */
String sex = bunde.getString("sex");
double height = bunde.getDouble("height");
/* 判断性别 */
String sexText = "";
if (sex.equals("M")) {
sexText = "男性";
} else {
sexText = "女性";
}
/* 取得标准体重 */
String weight = this.getWeight(sex, height);
/* 设置输出文字 */
TextView tv1 = (TextView) findViewById(R.id.text1);
tv1.setText("你是一位" + sexText + "\n你的身高是" + height + "厘米\n你的标准体重是"
+ weight + "公斤");
/* 返回事件 */
Button ok = (Button) findViewById(R.id.button_OK);
ok.setOnClickListener(new Button.OnClickListener() {
public void onClick(View v) {
setResult(RESULT_OK, intent);
finish();
}
});
}
/* 四舍五入的method */
private String format(double num) {
NumberFormat formatter = new DecimalFormat("0.00");
String s = formatter.format(num);
return s;
}
/* 计算标准体重 */
private String getWeight(String sex, double height) {
String weight = "";
if (sex.equals("M")) {
weight = format((height - 80) * 0.7);
} else {
weight = format((height - 70) * 0.6);
}
return weight;
}
}
相关文章推荐
- 使用C++实现JNI接口需要注意的事项
- Android IPC进程间通讯机制
- Android Manifest 用法
- [转载]Activity中ConfigChanges属性的用法
- Android之获取手机上的图片和视频缩略图thumbnails
- Android之使用Http协议实现文件上传功能
- Android学习笔记(二九):嵌入浏览器
- android string.xml文件中的整型和string型代替
- i-jetty环境搭配与编译
- android之定时器AlarmManager
- android wifi 无线调试
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- android 代码实现控件之间的间距
- android FragmentPagerAdapter的“标准”配置
- Android"解决"onTouch和onClick的冲突问题
- android:installLocation简析
- android searchView的关闭事件
- SourceProvider.getJniDirectories