iOS CGAffineTransform 动画
2016-06-12 18:48
549 查看
CoreGraphics框架中的CGAffineTransform类可用于设定UIView的transform属性,控制视图的缩放、旋转和平移操作:
另称放射变换矩阵,可参照线性代数的矩阵实现方式
这里附上的CGAffineTransform官方文档:
https://developer.apple.com/library/ios/documentation/GraphicsImaging/Reference/CGAffineTransform/index.html
Transform 是一种状态,并且只有一种状态
(1)CGAffineTransformMakeTranslation(<#CGFloat tx#>, <#CGFloat ty#>):只能变化一次,因为这种方式的变化始终是以最原始的状态值进行变化的,所以只能变化一次
(2)CGAffineTransformTranslate(CGAffineTransform t, <#CGFloat tx#>, <#CGFloat ty#>):能够多次变化,每次变化都是以上一次的状态(CGAffineTransform t)进行的变化,所以可以多次变化
(3) CGAffineTransformIdentity:清空所有的设置的transform(一般和动画配合使用,只能使用于transfofrm设置的画面)
(4)CGAffineTransformMakeScale( CGFloat sx, CGFloat sy) (缩放:设置缩放比例)仅通过设置缩放比例就可实现视图扑面而来和缩进频幕的效果。
(5) CGAffineTransformMakeRotation( CGFloat angle) (旋转:设置旋转角度)
关键代码如下:
项目结构如下
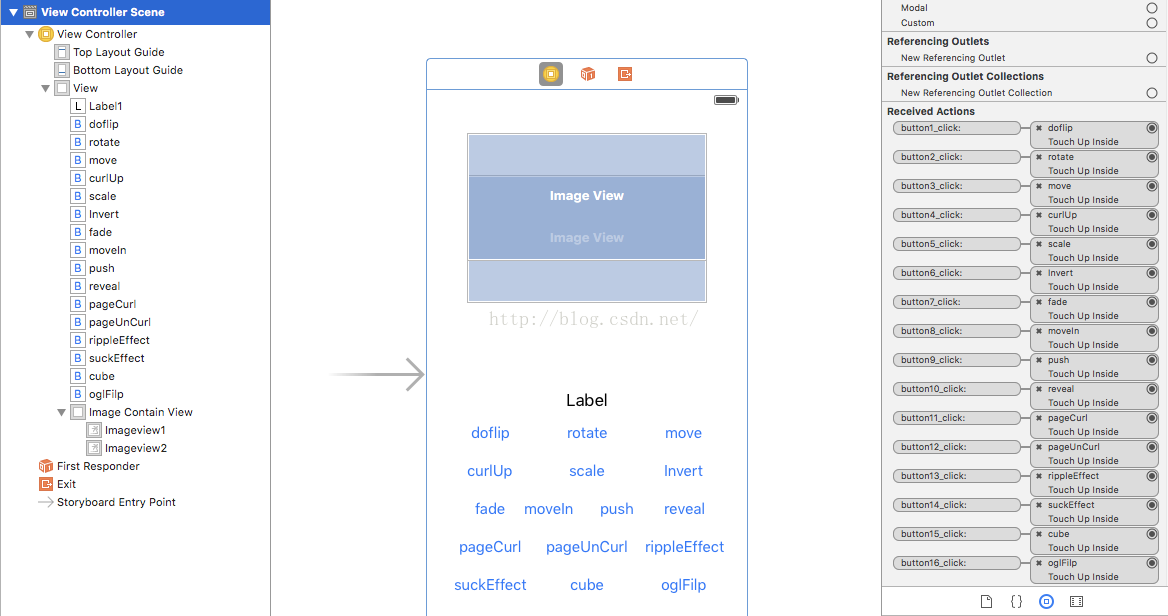
运行截图
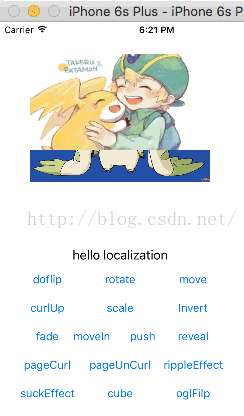
可以看到,fade和oglFilp是无效了,另外cameraIrisHollowOpen和cameraIrisHollowClose也是无效的。
还有当view旋转一定的角度之后,翻页动画也是有问题的。
另称放射变换矩阵,可参照线性代数的矩阵实现方式
这里附上的CGAffineTransform官方文档:
https://developer.apple.com/library/ios/documentation/GraphicsImaging/Reference/CGAffineTransform/index.html
Transform 是一种状态,并且只有一种状态
(1)CGAffineTransformMakeTranslation(<#CGFloat tx#>, <#CGFloat ty#>):只能变化一次,因为这种方式的变化始终是以最原始的状态值进行变化的,所以只能变化一次
(2)CGAffineTransformTranslate(CGAffineTransform t, <#CGFloat tx#>, <#CGFloat ty#>):能够多次变化,每次变化都是以上一次的状态(CGAffineTransform t)进行的变化,所以可以多次变化
(3) CGAffineTransformIdentity:清空所有的设置的transform(一般和动画配合使用,只能使用于transfofrm设置的画面)
(4)CGAffineTransformMakeScale( CGFloat sx, CGFloat sy) (缩放:设置缩放比例)仅通过设置缩放比例就可实现视图扑面而来和缩进频幕的效果。
(5) CGAffineTransformMakeRotation( CGFloat angle) (旋转:设置旋转角度)
关键代码如下:
@implementation ViewController @synthesize imageview1,imageview2, label1, imageContainView; UIImage *image1, *image2; UIView *view1, *view2; - (IBAction)button1_click:(id)sender { //翻转动画,旋转360度后复原(doflip) // additional context info passed to will start/did stop selectors. begin/commit can be nested //注意:动画效果是setAnimationTransition控制的,beginAnimations只是标识了这一组动画的名称 [UIView beginAnimations:@"doflip" context:nil]; [UIView setAnimationDuration:2]; /* 动画速度 typedef NS_ENUM(NSInteger, UIViewAnimationCurve) { UIViewAnimationCurveEaseInOut, // slow at beginning and end UIViewAnimationCurveEaseIn, // slow at beginning UIViewAnimationCurveEaseOut, // slow at end UIViewAnimationCurveLinear }; */ [UIView setAnimationCurve:UIViewAnimationCurveLinear]; [UIView setAnimationDelegate:self]; //设置翻转动画的起始方向 //UIViewAnimationTransitionFlipFromLeft(从左到右) //UIViewAnimationTransitionFlipFromLeft(从右到左) [UIView setAnimationTransition:UIViewAnimationTransitionFlipFromLeft forView:imageContainView cache:YES]; // starts up any animations when the top level animation is commited [UIView commitAnimations]; } - (IBAction)button2_click:(id)sender { //旋转动画,保持旋转后的状态(rotate) CGAffineTransform transform = CGAffineTransformRotate(imageContainView.transform, M_PI/6.0); [UIView beginAnimations:@"rotate" context:nil]; [UIView setAnimationDuration:2]; [UIView setAnimationDelegate:self]; [imageContainView setTransform:transform]; [UIView commitAnimations]; } - (IBAction)button3_click:(id)sender { //偏移动画(move) [UIView beginAnimations:@"move" context:nil]; [UIView setAnimationDuration:2]; [UIView setAnimationDelegate:self]; imageContainView.frame = CGRectMake(80, 80, 200, 200); [label1 setBackgroundColor:[UIColor yellowColor]]; [label1 setTextColor:[UIColor redColor]]; [UIView commitAnimations]; } - (IBAction)button4_click:(id)sender { //翻页动画(curlUp 和 curlDown) //curlUp表示将这一页翻上去 //curlDown表示将上一页翻下来 //注意:翻页动画针对的是与屏幕平行的矩形,如果被旋转相应角度,翻页不正常 [UIView beginAnimations:@"xxx" context:nil]; [UIView setAnimationDuration:2]; [UIView setAnimationDelegate:self]; [UIView setAnimationTransition:UIViewAnimationTransitionCurlUp forView:imageContainView cache:YES]; NSInteger imageview1Index = [[imageContainView subviews] indexOfObject:imageview1]; NSInteger imageview2Index = [[imageContainView subviews] indexOfObject:imageview2]; NSLog(@"subview index %ld %ld", imageview1Index, imageview2Index); //交换2视图的位置 [imageContainView exchangeSubviewAtIndex:imageview2Index withSubviewAtIndex:imageview1Index]; [UIView commitAnimations]; } - (IBAction)button5_click:(id)sender { //缩放动画(scale) //Scale `t' by `(sx, sy)',缩放默认是以视图的中心点为轴的 CGAffineTransform transform = CGAffineTransformScale(imageContainView.transform, 1.2, 1.2); [UIView beginAnimations:@"scale" context:nil]; [UIView setAnimationDuration:2]; [UIView setAnimationDelegate:self]; [imageContainView setTransform:transform]; [UIView commitAnimations]; } - (IBAction)button6_click:(id)sender { //根据当前的动画取反(Invert) //注意:只针对setTransform的视图,并且是取反而不是还原,其它动画无法取反 CGAffineTransform transform = CGAffineTransformInvert(imageContainView.transform); [UIView beginAnimations:@"Invert" context:nil]; [UIView setAnimationDuration:2]; [UIView setAnimationDelegate:self]; [imageContainView setTransform:transform]; [UIView commitAnimations]; } - (void)startAnimation:(NSString*) transitionType { CATransition *transition = [CATransition animation]; transition.delegate = self; transition.duration = 2; //时间函数(控制动画速度) transition.timingFunction = [CAMediaTimingFunction functionWithName:kCAMediaTimingFunctionEaseIn]; /* The name of the transition. Current legal transition types include * `fade', `moveIn', `push' and `reveal'. Defaults to `fade'. 另外还有一些私有动画 “pageCurl”(向上翻页) “pageUnCurl”(向下翻页)、"rippleEffect"(水滴) "suckEffect"(收缩抽走) "cube"(立方体) "oglFilp"(上下翻转) "cameraIrisHollowOpen"(镜头开) "cameraIrisHollowClose"(镜头关)*/ transition.type = transitionType; /* An optional subtype for the transition. E.g. used to specify the * transition direction for motion-based transitions, in which case * the legal values are `fromLeft', `fromRight', `fromTop' and * `fromBottom'. */ transition.subtype = kCATransitionFromTop; [imageContainView.layer addAnimation:transition forKey:@"transition"]; } - (IBAction)button7_click:(id)sender { [self startAnimation:kCATransitionFade]; } - (IBAction)button8_click:(id)sender { [self startAnimation:kCATransitionMoveIn]; } - (IBAction)button9_click:(id)sender { [self startAnimation:kCATransitionPush]; } - (IBAction)button10_click:(id)sender { [self startAnimation:kCATransitionReveal]; } - (IBAction)button11_click:(id)sender { [self startAnimation:@"pageCurl"]; } - (IBAction)button12_click:(id)sender { [self startAnimation:@"pageUnCurl"]; } - (IBAction)button13_click:(id)sender { [self startAnimation:@"rippleEffect"]; } - (IBAction)button14_click:(id)sender { [self startAnimation:@"suckEffect"]; } - (IBAction)button15_click:(id)sender { [self startAnimation:@"cube"]; } - (IBAction)button16_click:(id)sender { [self startAnimation:@"olgFilp"]; } - (void)animationWillStart:(CAAnimation *)anim { NSLog(@"animationWillStart"); } -(void)animationDidStop:(CAAnimation *)anim finished:(BOOL)flag { NSLog(@"animationDidStop"); }
项目结构如下
运行截图
可以看到,fade和oglFilp是无效了,另外cameraIrisHollowOpen和cameraIrisHollowClose也是无效的。
还有当view旋转一定的角度之后,翻页动画也是有问题的。
相关文章推荐
- iOS 使用NSTimer写出Button获取验证码倒计时的效果
- Multithreading annd Grand Central Dispatch on ios for Beginners Tutorial-多线程和GCD的入门教程
- iOS 检查更新
- 【Xamarin挖墙脚系列:Xamarin正式发布了IOS的模拟器在Windows下】
- (?)企业部分之nagios(未完)
- iOS组件化实践方案-LDBusMediator炼就
- iOS 组件化方案探索
- IOS Dev Intro - scutil command usage
- Charles 从入门到精通
- iOS runtime的理解和应用
- 浅析Runtime
- 关于iOS通话记录的问题
- iOS -关于Xcode中的单元测试unitTest的使用
- 导航栏、状态栏字体颜色大小和背景颜色
- iOS AFNetWorking 3.0
- iOS快速打企业包ipa
- iOS9的新特性以及适配方案
- iOS根据键盘弹出计算键盘高度
- 需求 - 22 - 多任务管理器效果
- 解决 iOS NSDictionary 输出中文字符”乱码”(Unicode编码)问题