android中实现透明悬浮的PopupWindow效果
2016-06-08 09:36
543 查看
最近做的android项目中经常用到PopupWindow,今天就来总结一下如何实现透明悬浮的PopupWindow效果,废话不多说,先上图和代码。
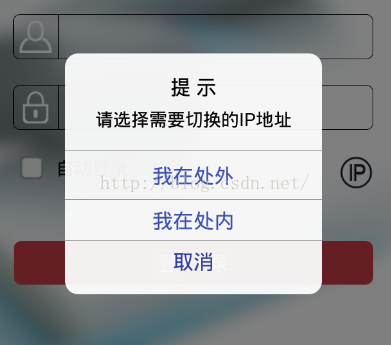
下面是PopupWindow的布局文件XML:
下面是圆角背景的XML文件:
这里的color值就设置了透明效果,EE就是透明度,透明度值为00-ff(00,11,22,...aa,bb,...,ee,ff),00为完全透明,ff为不透明。我这里是在背景资源文件中就设置了透明度值,当然在代码中也可以通过“.getBackground().setAlpha()”来设置透明度值,但这不一定有用。
下面就自定义我们需要的PopupWindow了:
接下来就是调用我们自定义的PopupWindow代码:
设置背景透明的方法:
这样就能实现透明悬浮的PopupWindow效果啦!
下面是PopupWindow的布局文件XML:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:gravity="center_horizontal" android:background="@drawable/bg_change_ip"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/login_name2" android:padding="5dp" android:textSize="16sp" android:layout_marginTop="10dp" android:textColor="@color/bg_line"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/login_name3" android:textColor="@color/bg_line"/> <View android:layout_width="match_parent" android:layout_height="0.5dp" android:background="@color/bg_line1" android:layout_marginTop="15dp"/> <TextView android:id="@+id/tv_change_ip_nei" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/login_name4" android:padding="8dp" android:textSize="16sp" android:textColor="@color/colorPrimary"/> <View android:layout_width="match_parent" android:layout_height="0.5dp" android:background="@color/bg_line1" /> <TextView android:id="@+id/tv_change_ip_wai" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/login_name5" android:padding="5dp" android:textSize="16sp" android:textColor="@color/colorPrimary"/> <View android:layout_width="match_parent" android:layout_height="0.5dp" android:background="@color/bg_line1" /> <TextView android:id="@+id/tv_change_ip_cancel" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/login_name6" android:paddingTop="5dp" android:textSize="16sp" android:textColor="@color/colorPrimaryDark" android:paddingBottom="15dp"/> </LinearLayout>
下面是圆角背景的XML文件:
<shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle"> <corners android:radius="10dp"/>//设置圆角角度 <solid android:color="#EEFFFFFF"/>//设置背景为白色带透明 </shape>
这里的color值就设置了透明效果,EE就是透明度,透明度值为00-ff(00,11,22,...aa,bb,...,ee,ff),00为完全透明,ff为不透明。我这里是在背景资源文件中就设置了透明度值,当然在代码中也可以通过“.getBackground().setAlpha()”来设置透明度值,但这不一定有用。
下面就自定义我们需要的PopupWindow了:
public class ChangeIPPopupWindow extends PopupWindow{ private Context context; //弹出的视图对象 private View popView; private TextView tvNei; private TextView tvWai; private TextView tvCancel; public ChangeIPPopupWindow(Context context){ this.context = context; LayoutInflater inflater = (LayoutInflater) context .getSystemService(Context.LAYOUT_INFLATER_SERVICE); popView = inflater.inflate(R.layout.popup_change_ip,null); //设置参数 initSetting(); initView(); } private void initSetting() { //加载视图 this.setContentView(popView); //设置宽高 this.setWidth(400); this.setHeight(ActionBar.LayoutParams.WRAP_CONTENT); //设置点击消失 this.setFocusable(true); this.setOutsideTouchable(true); //设置背景 ColorDrawable cd = new ColorDrawable(); this.setBackgroundDrawable(cd); //this.getBackground().setAlpha(50); //this.setBackgroundDrawable(new ColorDrawable(android.graphics.Color.parseColor("#FFFFFF"))); } private void initView(){ tvNei = (TextView) popView.findViewById(R.id.tv_change_ip_nei); tvWai = (TextView) popView.findViewById(R.id.tv_change_ip_wai); tvCancel = (TextView) popView.findViewById(R.id.tv_change_ip_cancel); tvNei.setOnClickListener(new IpOnClickListener()); tvWai.setOnClickListener(new IpOnClickListener()); tvCancel.setOnClickListener(new IpOnClickListener()); } class IpOnClickListener implements View.OnClickListener { @Override public void onClick(View v) { if(v == tvNei){//修改处内ip }else if(v == tvWai){//修改处外ip }else if(v == tvCancel){//取消 dismiss(); } } } }
接下来就是调用我们自定义的PopupWindow代码:
private void showChangeIPView(){ //创建自定义的PopupWindow ChangeIPPopupWindow ipView = new ChangeIPPopupWindow(this); //背景变透明 MainUtils.setBackgroundAlpha(this,0.5f); //居中显示 ipView.showAtLocation(etName, Gravity.CENTER,0,0); //设置PopupWindow消失监听,消失时恢复背景透明 ipView.setOnDismissListener(new PopupWindow.OnDismissListener() { @Override public void onDismiss() { MainUtils.setBackgroundAlpha(LoginActivity.this,1.0f); } }); }
设置背景透明的方法:
/** * 改变当前页面的背景透明度 * @param activity * @param alpha 透明度值,0-1 */ public static void setBackgroundAlpha(Activity activity,float alpha){ WindowManager.LayoutParams lp = activity.getWindow().getAttributes(); lp.alpha = alpha; activity.getWindow().setAttributes(lp); }
这样就能实现透明悬浮的PopupWindow效果啦!
相关文章推荐
- Android之Adapter的封装与抽象(二)
- Android JNI开发高级篇
- Android 时间为隔天的九点、并且跳过周末
- android studio \65279错误
- This tag and its children can be replaced by one <TextView/> and a compound drawable
- Android中的 Handler
- Android RecyclerView详解及实现瀑布流式布局 推荐
- Android开发书籍推荐 & 学习路线图 & 资料汇整
- android 三种定时器的写法
- Android中的颜色设置
- 今日推荐(三)AndroidResideMenu类似QQ侧滑效果
- 玩转Android之Drawable的使用
- AndroidStudio项目导入第三方library
- Android基础知识之frame动画效果
- AndroidStudio配置SVN以及使用代码管理
- Android基础知识之tween动画效果
- Android判断是否是黑屏
- android windowmanager各个属性
- Android studio - Unable to move Android SDK
- Android 4个常用的工具类