android控件ImageView加载sdcard卡,网络及res中的图片
2016-05-29 23:14
597 查看
提前声明
加载网络资源需要网络访问权限:
<uses-permission android:name="android.permission.INTERNET" />
加载sd卡中的资源则需要权限:
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
不然, 不然 , 代码正确,但一直会抛异常哟 因为没权限呀 没权限
效果图:
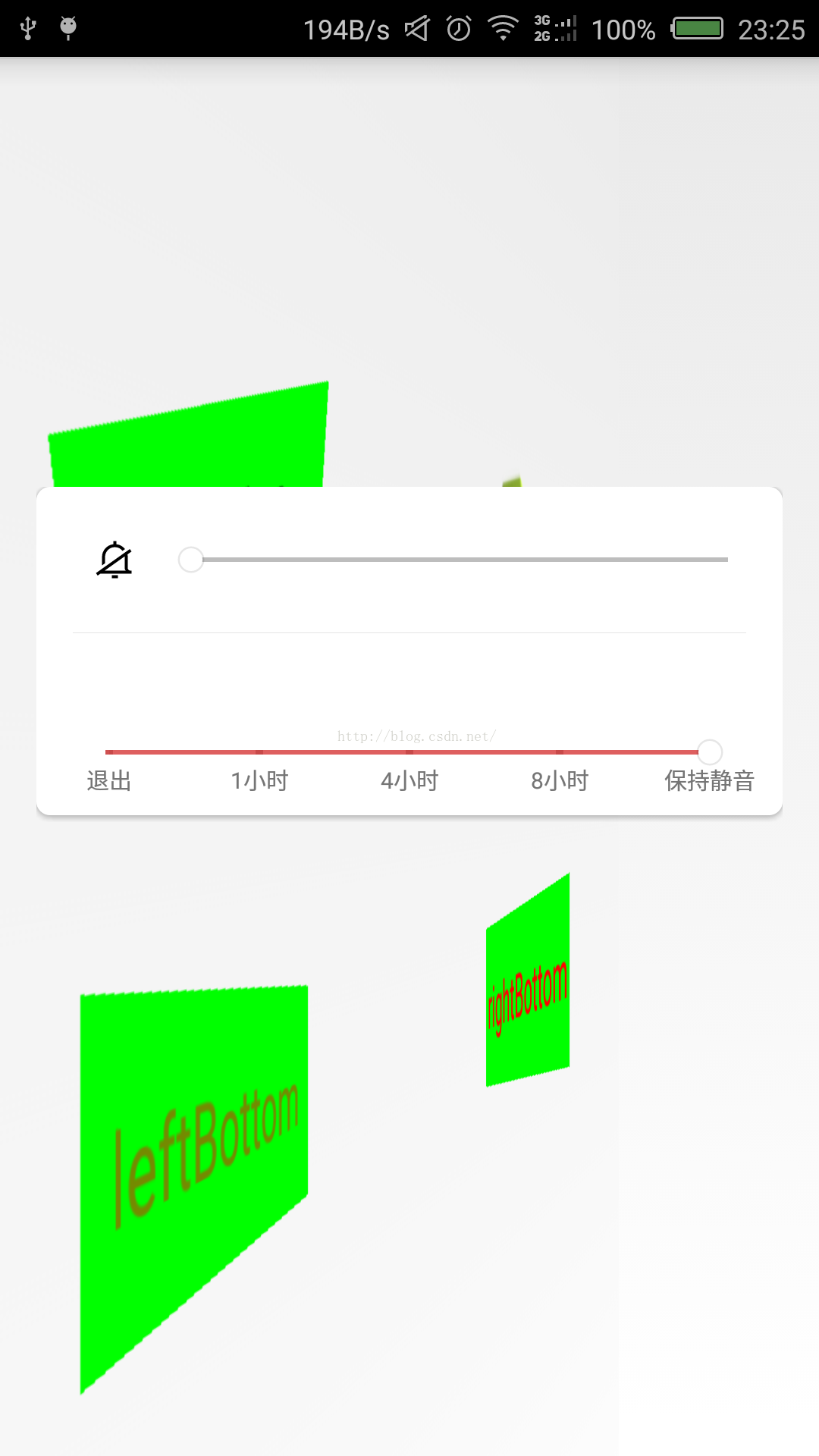
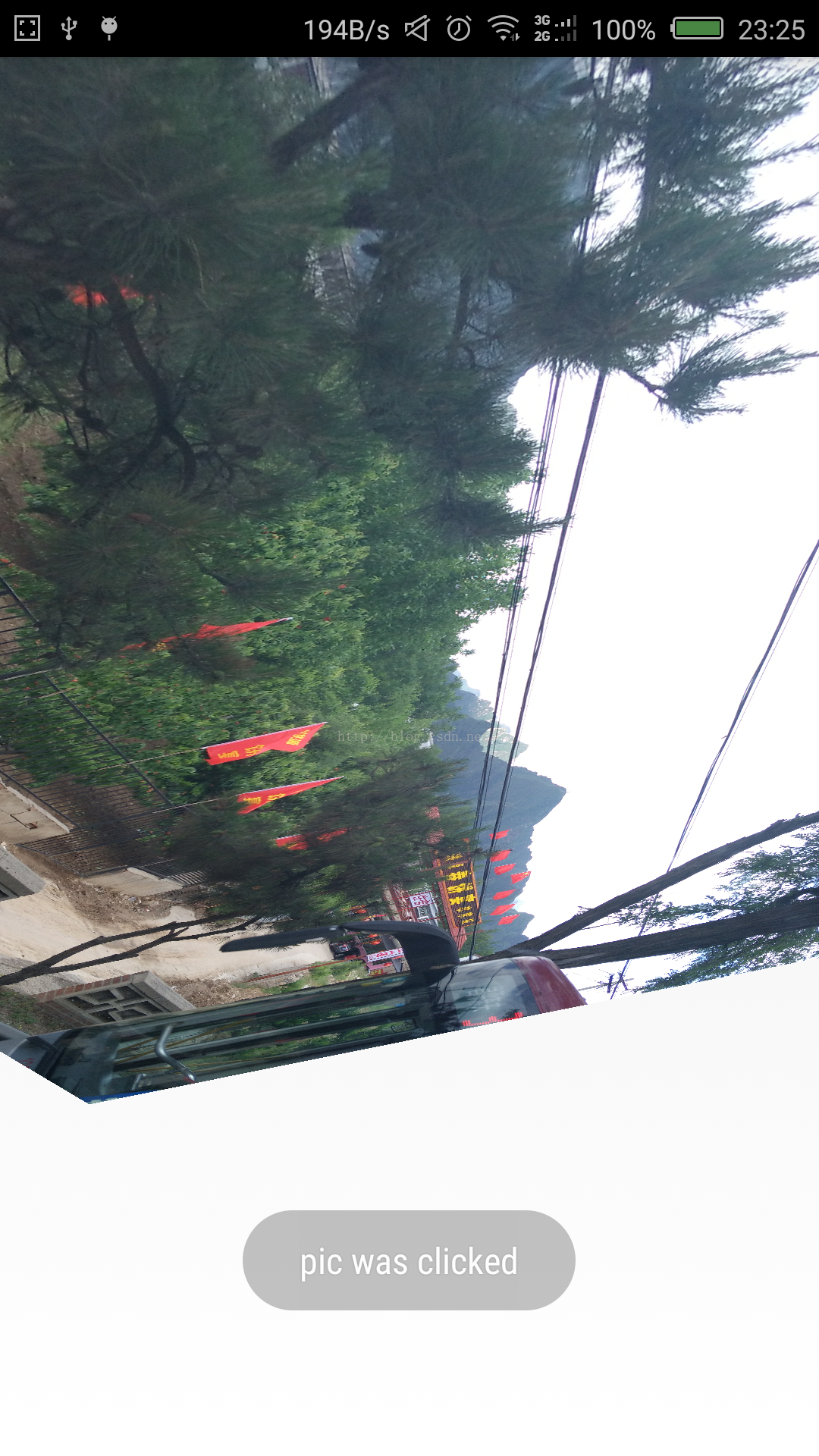
一:
先说访问res/drawable/下的图片的访问方法中,因为这种最简单,getResources()是Context的方法。
二:
访问本地SD卡中的图片
三:
访问网络上的图片资源
这种情况下,要注意一下,android不允许在 在跟界面操作所在的线程中加入会导致程序反应迟钝的操作,譬如上网加载网上的数据,这些操作,要实现就一定得放到其他线程中去执行。这里,为了简便起见,咱们就直接用网上的async-http开源框架好了,内部实现原理就是开线程专门加载资源,加载完再反馈界面主线程。
操作起来还算简单,记得下载android-async-http-1.4.6.jar包,我用的1.4.6 后面的版本会出点问题。
源码:
activity_main.xml
MainActivity.java
AndroidManifest.xml
加载网络资源需要网络访问权限:
<uses-permission android:name="android.permission.INTERNET" />
加载sd卡中的资源则需要权限:
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
不然, 不然 , 代码正确,但一直会抛异常哟 因为没权限呀 没权限
效果图:
一:
先说访问res/drawable/下的图片的访问方法中,因为这种最简单,getResources()是Context的方法。
myPic.setImageDrawable(getResources().getDrawable(R.drawable.ic_launcher));
二:
访问本地SD卡中的图片
String path=Environment.getExternalStorageDirectory().toString()+"/aa.jpg"; Bitmap bitmap = getLoacalBitmap(path); //从本地取图片
三:
访问网络上的图片资源
这种情况下,要注意一下,android不允许在 在跟界面操作所在的线程中加入会导致程序反应迟钝的操作,譬如上网加载网上的数据,这些操作,要实现就一定得放到其他线程中去执行。这里,为了简便起见,咱们就直接用网上的async-http开源框架好了,内部实现原理就是开线程专门加载资源,加载完再反馈界面主线程。
操作起来还算简单,记得下载android-async-http-1.4.6.jar包,我用的1.4.6 后面的版本会出点问题。
String url="http://img.club.pchome.net/upload/club/other/2015/6/24/pics_kdsxxoo_1435102809.jpg"; AsyncHttpClient client=new AsyncHttpClient(); client.get(url, new AsyncHttpResponseHandler(){ @Override public void onSuccess(int statusCode, Header[] headers, byte[] responseBody) { if(statusCode==200){ BitmapFactory factory=new BitmapFactory(); Bitmap bitmap=factory.decodeByteArray(responseBody, 0, responseBody.length); myPic.setImageBitmap(bitmap); } } @Override public void onFailure(int statusCode, Header[] headers, byte[] responseBody, Throwable error) { error.printStackTrace(); } });
源码:
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.liang.test1.MainActivity" > <ImageView android:id="@+id/mypic" android:layout_width="400dp" android:layout_height="400dp" android:layout_centerInParent="true" android:scaleY="3" android:scaleX="1.5" android:rotationY="35" android:src="@drawable/aaa"/> </RelativeLayout>
MainActivity.java
package com.liang.test1; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException; import java.io.InputStream; import java.net.HttpURLConnection; import java.net.MalformedURLException; import java.net.URL; import org.apache.http.Header; import com.loopj.android.http.AsyncHttpClient; import com.loopj.android.http.AsyncHttpResponseHandler; import android.app.Activity; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.os.Bundle; import android.os.Environment; import android.util.Log; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.view.View.OnClickListener; import android.view.Window; import android.view.animation.AlphaAnimation; import android.view.animation.Animation; import android.view.animation.RotateAnimation; import android.widget.ImageView; import android.widget.Toast; public class MainActivity extends Activity implements OnClickListener{ private static final String TAG = "MAIN_ACTIVITY"; // private Button leftBottomBut; // private Button rightBottomBut; // private Button leftTopBut; // private Button rightTopBut; private ImageView myPic; private AlphaAnimation myAnimation_Alpha; private RotateAnimation myAnimation_Rotate; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); this.getWindow().requestFeature(Window.FEATURE_NO_TITLE); setContentView(R.layout.activity_main); myPic=(ImageView)findViewById(R.id.mypic); myPic.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View arg0) { // TODO Auto-generated method stub String url="http://img.club.pchome.net/upload/club/other/2015/6/24/pics_kdsxxoo_1435102809.jpg"; AsyncHttpClient client=new AsyncHttpClient(); client.get(url, new AsyncHttpResponseHandler(){ @Override public void onSuccess(int statusCode, Header[] headers, byte[] responseBody) { if(statusCode==200){ BitmapFactory factory=new BitmapFactory(); Bitmap bitmap=factory.decodeByteArray(responseBody, 0, responseBody.length); myPic.setImageBitmap(bitmap); } } @Override public void onFailure(int statusCode, Header[] headers, byte[] responseBody, Throwable error) { error.printStackTrace(); } }); Toast.makeText(MainActivity.this, "pic was clicked", Toast.LENGTH_SHORT).show(); // Bitmap bitmap =getHttpBitmap("http://blog.3gstdy.com/wp-content/themes/twentyten/images/headers/path.jpg"); String path=Environment.getExternalStorageDirectory().toString()+"/aa.jpg"; Bitmap bitmap = getLoacalBitmap(path); //从本地取图片 // myPic.setImageDrawable(getResources().getDrawable(R.drawable.ic_launcher)); myPic.setImageBitmap(bitmap); // AlphaAnimation(float fromAlpha, float toAlpha) //说明:0.0表示完全透明,1.0表示完全不透明 myAnimation_Rotate=new RotateAnimation(0.0f, +350.0f,Animation.RELATIVE_TO_SELF,0.5f,Animation.RELATIVE_TO_SELF, 0.5f); myAnimation_Alpha=new AlphaAnimation(0.1f, 1.0f); myAnimation_Rotate.setDuration(5000); myPic.setAnimation(myAnimation_Rotate); myAnimation_Rotate.start(); } }); // leftBottomBut=(Button)findViewById(R.id.left_bottom_but); // leftBottomBut.setOnClickListener(this); // rightBottomBut=(Button)findViewById(R.id.right_bottom_but); // rightBottomBut.setOnClickListener(this); // leftTopBut=(Button)findViewById(R.id.left_top_but); // leftTopBut.setOnClickListener(new View.OnClickListener() { // // @Override // public void onClick(View arg0) { // // TODO Auto-generated method stub // Toast.makeText(MainActivity.this, "left_top_but clicked", Toast.LENGTH_SHORT).show(); // } // }); // rightTopBut=(Button)findViewById(R.id.right_top_but); // rightTopBut.setOnClickListener(this); } /** * 加载本地图片 * http://bbs.3gstdy.com * @param url * @return */ public static Bitmap getLoacalBitmap(String url) { try { FileInputStream fis = new FileInputStream(url); return BitmapFactory.decodeStream(fis); } catch (FileNotFoundException e) { e.printStackTrace(); return null; } } public static Bitmap getHttpBitmap(String url) { URL myFileUrl = null; Bitmap bitmap = null; try { Log.d(TAG, url); myFileUrl = new URL(url); } catch (MalformedURLException e) { e.printStackTrace(); } try { HttpURLConnection conn = (HttpURLConnection) myFileUrl.openConnection(); conn.setConnectTimeout(0); conn.setDoInput(true); conn.connect(); InputStream is = conn.getInputStream(); bitmap = BitmapFactory.decodeStream(is); is.close(); } catch (IOException e) { e.printStackTrace(); } return bitmap; } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); } @Override public void onClick(View v) { // TODO Auto-generated method stub // switch(v.getId()) // { // case R.id.left_bottom_but: // Toast.makeText(this, "left_bottom_but clicked", Toast.LENGTH_SHORT).show(); // break; // case R.id.right_bottom_but: // Toast.makeText(this, "right_bottom_but clicked", Toast.LENGTH_SHORT).show(); // break; // default: // break; // } } public void butclicked(View v) { // switch(v.getId()) // { // case R.id.right_top_but: // Toast.makeText(this, "right_top_but clicked", Toast.LENGTH_SHORT).show(); // break; // default: // break; // } } }
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.liang.test1" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="15" android:targetSdkVersion="15" /> <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name=".MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
相关文章推荐
- 初识XMLHttpRequeset
- 初识XMLHttpRequeset
- HTTP协议 keep-alive连接 与 BS(firefox-thttpd)实验
- 网络编程
- HttpClient连接管理
- 使用Agera,gson,okhttp获取豆瓣读书数据
- Chp6 域名系统DNS
- Tinyhttp源码分析
- 20159217《网络攻防实践》第十三周学习总结
- TCP延迟确认
- 图片的HTTP请求
- HttpClient get请求在HttpResponse中无法获得Location的问题
- Socket实现两台手机通信,定向转发数据
- SOCKET API和TCP STATE的对应关系__三次握手(listen,accept,connect)__四次挥手close及TCP延迟确认(调用一次setsockopt函数,设
- TCP/IP模型 ,Socket编程总结
- 或许是 Nginx 上配置 HTTP2 最实在的教程了
- Ubuntu l连接网络
- Go学习笔记二: 函数,文件操作及网络通信
- Tinyhttp服务器编译运行
- BSOJ: 1708 【USACO 2008 January Gold】Cell Phone Network手机网络