AsyncTask - 基本原理 后台线程和UI线程的交互
2016-05-24 13:30
465 查看
前面一个文章大概描述了一下任务是怎么被执行的?http://blog.csdn.net/zj510/article/details/51485120
其实也就是AsyncTask里面的doInBackground怎么通过FutureTask和WorkerRunnable在ThreadPoolExecutor的某个线程里面执行的。
那么执行过程中和执行完毕了总要通知主调线程(主线程)吧?
AsyncTask的事件
基本上,AsyncTask有如下一些事件通知
onPreExecute()
onPostExecute()
onProgressUpdate()
onCancelled()
下面的代码来自AsyncTask。
那么我们就来看看每个事件回调是怎么被调用的?
onPreExecute()
AsyncTask的调用者需要调用execute来启动一个AsyncTask,如:
onPostExecute
onPostExecute这个就有点讲究了,之前也讲到了,AsyncTask的构造函数里面会创建一个WorkerRunnable的子类,重写了call函数。而call函数将在ThreadPoolExecutor的一个线程里面被调用。
MESSAGE_POST_RESULT是AsyncTask定义的一个消息ID,如下:
InternalHandler定义如下:
在case MESSAGE_POST_RESULT里面看到result.mTask.finish(result.mData[0]);
result是由postResult发过来的,它是一个AsyncTaskResult对象,它的定义如下:
finish函数在handler的处理函数里面被调用,而实际上handlerMessage实在handler所在线程被调用,那么finish也将在Asynctask的创建线程里面被调用。
看实现:
画了个示意图,不是很准确,但大致就这么个意思。
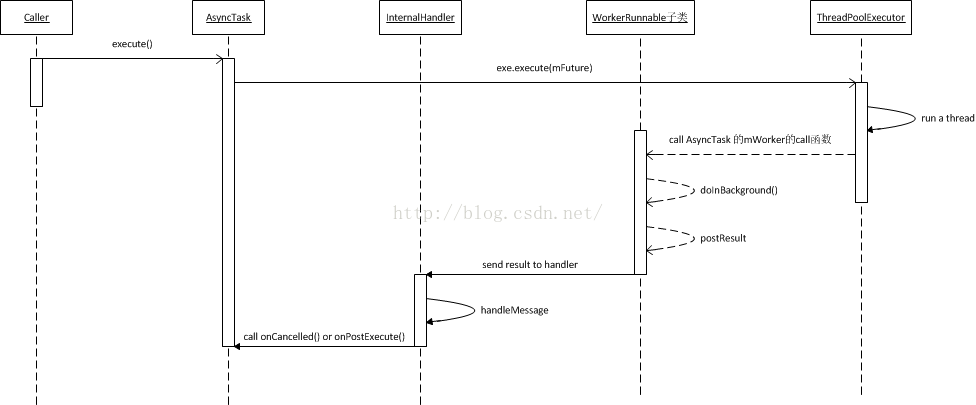
对于onCancelled和onPostExecute,这两个消息响应函数都是通过发送MESSAGE_POST_RESULT给handler,在handler的handleMessage里面被处理的。
onProgressUpdate
对于onProgressUpdate,就得先看看publishProgress了。
比如:
publishProgress其实就是通过handler发送消息,这里发送的是MESSAGE_POST_PROGRESS。然后InternalExecutor会处理这个消息。
private static class InternalHandler extends Handler {
@SuppressWarnings({"unchecked", "RawUseOfParameterizedType"})
@Override
public void handleMessage(Message msg) {
AsyncTaskResult result = (AsyncTaskResult) msg.obj;
switch (msg.what) {
case MESSAGE_POST_RESULT:
// There is only one result
result.mTask.finish(result.mData[0]);
break;
case MESSAGE_POST_PROGRESS:
result.mTask.onProgressUpdate(result.mData);
break;
}
}
}直接调用AsyncTask的onProgressUpdate,因为调用者重写了AsyncTask的onProgressUpdate函数,所以派生类的这个函数就被调用了(在主线程)。然后我们就可以更新一些UI什么的。
本质上onProgressUpdate和onPostExecute,onCancelled一样,都是通过handler从后台线程发送到主调线程的,用了不同的MESSAGE而已。
其实也就是AsyncTask里面的doInBackground怎么通过FutureTask和WorkerRunnable在ThreadPoolExecutor的某个线程里面执行的。
那么执行过程中和执行完毕了总要通知主调线程(主线程)吧?
AsyncTask的事件
基本上,AsyncTask有如下一些事件通知
onPreExecute()
onPostExecute()
onProgressUpdate()
onCancelled()
下面的代码来自AsyncTask。
protected abstract Result doInBackground(Params... params); /** * Runs on the UI thread before {@link #doInBackground}. * * @see #onPostExecute * @see #doInBackground */ protected void onPreExecute() { } /** * <p>Runs on the UI thread after {@link #doInBackground}. The * specified result is the value returned by {@link #doInBackground}.</p> * * <p>This method won't be invoked if the task was cancelled.</p> * * @param result The result of the operation computed by {@link #doInBackground}. * * @see #onPreExecute * @see #doInBackground * @see #onCancelled(Object) */ @SuppressWarnings({"UnusedDeclaration"}) protected void onPostExecute(Result result) { } /** * Runs on the UI thread after {@link #publishProgress} is invoked. * The specified values are the values passed to {@link #publishProgress}. * * @param values The values indicating progress. * * @see #publishProgress * @see #doInBackground */ @SuppressWarnings({"UnusedDeclaration"}) protected void onProgressUpdate(Progress... values) { } /** * <p>Runs on the UI thread after {@link #cancel(boolean)} is invoked and * {@link #doInBackground(Object[])} has finished.</p> * * <p>The default implementation simply invokes {@link #onCancelled()} and * ignores the result. If you write your own implementation, do not call * <code>super.onCancelled(result)</code>.</p> * * @param result The result, if any, computed in * {@link #doInBackground(Object[])}, can be null * * @see #cancel(boolean) * @see #isCancelled() */ @SuppressWarnings({"UnusedParameters"}) protected void onCancelled(Result result) { onCancelled(); } /** * <p>Applications should preferably override {@link #onCancelled(Object)}. * This method is invoked by the default implementation of * {@link #onCancelled(Object)}.</p> * * <p>Runs on the UI thread after {@link #cancel(boolean)} is invoked and * {@link #doInBackground(Object[])} has finished.</p> * * @see #onCancelled(Object) * @see #cancel(boolean) * @see #isCancelled() */ protected void onCancelled() { }
那么我们就来看看每个事件回调是怎么被调用的?
onPreExecute()
AsyncTask的调用者需要调用execute来启动一个AsyncTask,如:
AsyncTask<Void, Void, String> asyncTask = new AsyncTask<Void, Void, String>(){ @Override protected String doInBackground(Void... param) { Log.v("AsyncTask", "doInBackground"); return "hello asyncTask"; } @Override public void onPostExecute(String response) { // callback.onSendRequestFinished(JsonUtil.jsonToBean(response, mBeanType)); Toast.makeText(MainActivity.this, "result: " + response, Toast.LENGTH_LONG).show(); } }; asyncTask.execute();那么execute里面做了什么呢?看:
public final AsyncTask<Params, Progress, Result> execute(Params... params) { return executeOnExecutor(sDefaultExecutor, params); } /** * Executes the task with the specified parameters. The task returns * itself (this) so that the caller can keep a reference to it. * * <p>This method is typically used with {@link #THREAD_POOL_EXECUTOR} to * allow multiple tasks to run in parallel on a pool of threads managed by * AsyncTask, however you can also use your own {@link Executor} for custom * behavior. * * <p><em>Warning:</em> Allowing multiple tasks to run in parallel from * a thread pool is generally <em>not</em> what one wants, because the order * of their operation is not defined. For example, if these tasks are used * to modify any state in common (such as writing a file due to a button click), * there are no guarantees on the order of the modifications. * Without careful work it is possible in rare cases for the newer version * of the data to be over-written by an older one, leading to obscure data * loss and stability issues. Such changes are best * executed in serial; to guarantee such work is serialized regardless of * platform version you can use this function with {@link #SERIAL_EXECUTOR}. * * <p>This method must be invoked on the UI thread. * * @param exec The executor to use. {@link #THREAD_POOL_EXECUTOR} is available as a * convenient process-wide thread pool for tasks that are loosely coupled. * @param params The parameters of the task. * * @return This instance of AsyncTask. * * @throws IllegalStateException If {@link #getStatus()} returns either * {@link AsyncTask.Status#RUNNING} or {@link AsyncTask.Status#FINISHED}. * * @see #execute(Object[]) */ public final AsyncTask<Params, Progress, Result> executeOnExecutor(Executor exec, Params... params) { if (mStatus != Status.PENDING) { switch (mStatus) { case RUNNING: throw new IllegalStateException("Cannot execute task:" + " the task is already running."); case FINISHED: throw new IllegalStateException("Cannot execute task:" + " the task has already been executed " + "(a task can be executed only once)"); } } mStatus = Status.RUNNING; onPreExecute(); mWorker.mParams = params; exec.execute(mFuture); return this; }很明显,onPreExecute()直接在调用线程里面被调用了,然后才调用Executor的execute来执行任务(mFuture)。onPreExecute很简单,没啥好说的。
onPostExecute
onPostExecute这个就有点讲究了,之前也讲到了,AsyncTask的构造函数里面会创建一个WorkerRunnable的子类,重写了call函数。而call函数将在ThreadPoolExecutor的一个线程里面被调用。
public AsyncTask() { mWorker = new WorkerRunnable<Params, Result>() { public Result call() throws Exception { mTaskInvoked.set(true); Process.setThreadPriority(Process.THREAD_PRIORITY_BACKGROUND); //noinspection unchecked Result result = doInBackground(mParams); Binder.flushPendingCommands(); return postResult(result); } }; mFuture = new FutureTask<Result>(mWorker) { @Override protected void done() { try { postResultIfNotInvoked(get()); } catch (InterruptedException e) { android.util.Log.w(LOG_TAG, e); } catch (ExecutionException e) { throw new RuntimeException("An error occurred while executing doInBackground()", e.getCause()); } catch (CancellationException e) { postResultIfNotInvoked(null); } } }; }从上面的代码可以看到doInBackground是在ThreadPoolExecutor的一个线程里面被调用的。当doInBackground调用结束,注意postResult(result)这行。先看看代码:
private Result postResult(Result result) { @SuppressWarnings("unchecked") Message message = sHandler.obtainMessage(MESSAGE_POST_RESULT, new AsyncTaskResult<Result>(this, result)); message.sendToTarget(); return result; }很清楚,通过一个handler,把MESSAGE_POST_RESULT消息发给了handler的拥有者,同时通过AsyncTaskResult对象把doInBackground返回值一并给handler的拥有者。
MESSAGE_POST_RESULT是AsyncTask定义的一个消息ID,如下:
private static final int MESSAGE_POST_RESULT = 0x1; private static final int MESSAGE_POST_PROGRESS = 0x2;那么现在来看看sHandler,
private static final InternalHandler sHandler = new InternalHandler();sHandler是AsyncTask的一个静态数据成员。
InternalHandler定义如下:
private static class InternalHandler extends Handler { @SuppressWarnings({"unchecked", "RawUseOfParameterizedType"}) @Override public void handleMessage(Message msg) { AsyncTaskResult result = (AsyncTaskResult) msg.obj; switch (msg.what) { case MESSAGE_POST_RESULT: // There is only one result result.mTask.finish(result.mData[0]); break; case MESSAGE_POST_PROGRESS: result.mTask.onProgressUpdate(result.mData); break; } } }超简单的一个类,就是继承自Handler,然后重写handleMessage,处理了MESSAGE_POST_RESULT和MESSAGE_POST_PROGRESS两个消息。
在case MESSAGE_POST_RESULT里面看到result.mTask.finish(result.mData[0]);
result是由postResult发过来的,它是一个AsyncTaskResult对象,它的定义如下:
private static class AsyncTaskResult<Data> { final AsyncTask mTask; final Data[] mData; AsyncTaskResult(AsyncTask task, Data... data) { mTask = task; mData = data; } }其实第一个数据成员其实就是当前AsyncTask对象,可以看postResult里面把this当作了AsyncTaskResult构造函数的第一个参数。所以我们只需要看AsyncTask的finish函数。
finish函数在handler的处理函数里面被调用,而实际上handlerMessage实在handler所在线程被调用,那么finish也将在Asynctask的创建线程里面被调用。
看实现:
private void finish(Result result) { if (isCancelled()) { onCancelled(result); } else { onPostExecute(result); } mStatus = Status.FINISHED; }very simple,如果调用者调用了AsyncTask的cancel,那么就调用onCancel(result),否则就调用onPostExecute(result);
画了个示意图,不是很准确,但大致就这么个意思。
对于onCancelled和onPostExecute,这两个消息响应函数都是通过发送MESSAGE_POST_RESULT给handler,在handler的handleMessage里面被处理的。
onProgressUpdate
对于onProgressUpdate,就得先看看publishProgress了。
protected final void publishProgress(Progress... values) { if (!isCancelled()) { sHandler.obtainMessage(MESSAGE_POST_PROGRESS, new AsyncTaskResult<Progress>(this, values)).sendToTarget(); } }这个一般是用来显示后台线程处理进度了。我们使用AsyncTask的时候,都是会派生一个子类,然后重写doInBackground来处理事情。比如在doInBackground里面需要进行网络访问,那么doInBackground就会在后台线程里面被执行。当我们想知道进度的时候,就需要自己在doInBackground里面调用publishProgress了。
比如:
AsyncTask<Void, Void, String> asyncTask = new AsyncTask<Void, Void, String>(){ @Override protected String doInBackground(Void... param) { publishProgress(10%); Log.v("AsyncTask", "doInBackground"); publishProgress(50%); do something; publishProgress(100%); return "hello asyncTask"; } @Override protected onProgressUpdate() { update UI progress bar; } @Override public void onPostExecute(String response) { // callback.onSendRequestFinished(JsonUtil.jsonToBean(response, mBeanType)); Toast.makeText(MainActivity.this, "result: " + response, Toast.LENGTH_LONG).show(); } }; asyncTask.execute();
publishProgress其实就是通过handler发送消息,这里发送的是MESSAGE_POST_PROGRESS。然后InternalExecutor会处理这个消息。
private static class InternalHandler extends Handler {
@SuppressWarnings({"unchecked", "RawUseOfParameterizedType"})
@Override
public void handleMessage(Message msg) {
AsyncTaskResult result = (AsyncTaskResult) msg.obj;
switch (msg.what) {
case MESSAGE_POST_RESULT:
// There is only one result
result.mTask.finish(result.mData[0]);
break;
case MESSAGE_POST_PROGRESS:
result.mTask.onProgressUpdate(result.mData);
break;
}
}
}直接调用AsyncTask的onProgressUpdate,因为调用者重写了AsyncTask的onProgressUpdate函数,所以派生类的这个函数就被调用了(在主线程)。然后我们就可以更新一些UI什么的。
本质上onProgressUpdate和onPostExecute,onCancelled一样,都是通过handler从后台线程发送到主调线程的,用了不同的MESSAGE而已。
相关文章推荐
- 使用C++实现JNI接口需要注意的事项
- Android IPC进程间通讯机制
- Android Manifest 用法
- [转载]Activity中ConfigChanges属性的用法
- Android之获取手机上的图片和视频缩略图thumbnails
- Android之使用Http协议实现文件上传功能
- Android学习笔记(二九):嵌入浏览器
- android string.xml文件中的整型和string型代替
- i-jetty环境搭配与编译
- android之定时器AlarmManager
- android wifi 无线调试
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- android 代码实现控件之间的间距
- android FragmentPagerAdapter的“标准”配置
- Android"解决"onTouch和onClick的冲突问题
- android:installLocation简析
- android searchView的关闭事件
- SourceProvider.getJniDirectories