Linux 进程间通信
2016-05-12 14:20
471 查看
linux下进程间通信的几种主要手段简介:
1 管道(Pipe)及有名管道(named pipe):管道可用于具有亲缘关系进程间的通信,有名管道克服了管道没有名字的限制,因此,除具有管道所具有的功能外,它还允许无亲缘关系进程间的通信;
2 信号(Signal):信号是比较复杂的通信方式,用于通知接受进程有某种事件发生,除了用于进程间通信外,进程还可以发送信号给进程本身;linux除了支持Unix早期信号语义函数sigal外,还支持语义符合Posix.1标准的信号函数sigaction(实际上,该函数是基于BSD的,BSD为了实现可靠信号机制,又能够统一对外接口,用sigaction函数重新实现了signal函数);
3 报文(Message)队列(消息队列):消息队列是消息的链接表,包括Posix消息队列system V消息队列。有足够权限的进程可以向队列中添加消息,被赋予读权限的进程则可以读走队列中的消息。消息队列克服了信号承载信息量少,管道只能承载无格式字节流以及缓冲区大小受限等缺点。
4 共享内存:使得多个进程可以访问同一块内存空间,是最快的可用IPC形式。是针对其他通信机制运行效率较低而设计的。往往与其它通信机制,如信号量结合使用,来达到进程间的同步及互斥。
5 信号量(semaphore):主要作为进程间以及同一进程不同线程之间的同步手段。
6 套接口(Socket):更为一般的进程间通信机制,可用于不同机器之间的进程间通信。起初是由Unix系统的BSD分支开发出来的,但现在一般可以移植到其它类Unix系统上:Linux和System V的变种都支持套接字。
pipe(fd[2])
fd[1]写,fd[0]读
运行结果:
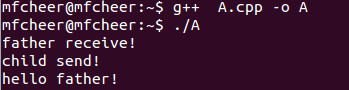
运行结果:

shmat() 函数将共享内存添加到进程地址中
shmdt() 进程退出共享内存
shmctl() 对内存区域的操作
运行结果:
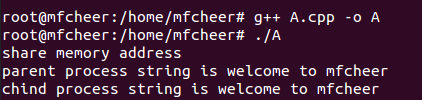
semop() 信号量操作
semctl() 信号量的控制
1 管道(Pipe)及有名管道(named pipe):管道可用于具有亲缘关系进程间的通信,有名管道克服了管道没有名字的限制,因此,除具有管道所具有的功能外,它还允许无亲缘关系进程间的通信;
2 信号(Signal):信号是比较复杂的通信方式,用于通知接受进程有某种事件发生,除了用于进程间通信外,进程还可以发送信号给进程本身;linux除了支持Unix早期信号语义函数sigal外,还支持语义符合Posix.1标准的信号函数sigaction(实际上,该函数是基于BSD的,BSD为了实现可靠信号机制,又能够统一对外接口,用sigaction函数重新实现了signal函数);
3 报文(Message)队列(消息队列):消息队列是消息的链接表,包括Posix消息队列system V消息队列。有足够权限的进程可以向队列中添加消息,被赋予读权限的进程则可以读走队列中的消息。消息队列克服了信号承载信息量少,管道只能承载无格式字节流以及缓冲区大小受限等缺点。
4 共享内存:使得多个进程可以访问同一块内存空间,是最快的可用IPC形式。是针对其他通信机制运行效率较低而设计的。往往与其它通信机制,如信号量结合使用,来达到进程间的同步及互斥。
5 信号量(semaphore):主要作为进程间以及同一进程不同线程之间的同步手段。
6 套接口(Socket):更为一般的进程间通信机制,可用于不同机器之间的进程间通信。起初是由Unix系统的BSD分支开发出来的,但现在一般可以移植到其它类Unix系统上:Linux和System V的变种都支持套接字。
1 pipe管道
子进程写,父进程读。pipe(fd[2])
fd[1]写,fd[0]读
#include <unistd.h> #include <stdio.h> #include <string.h> #define MAXSIZE 100 int main() { int fd[2],pid,line; char message[100]; if (pipe(fd) == -1) { printf("create failed!\n"); return 1; } else if ((pid =fork()) < 0) { printf("child process failed\n!"); return 1; } else if (pid == 0) { close(fd[0]); printf("child send!\n"); write(fd[1],"hello father!",14); } else { close(fd[1]); printf("father receive!\n"); line = read(fd[0],message,MAXSIZE); write(STDOUT_FILENO,message,line); printf("\n"); _exit(0); } return 0; }
运行结果:
2 命名管道:
mkfifo(“路径名”,文件权限)#include <unistd.h> #include <stdio.h> #include <string.h> #include <sys/types.h> #include <sys/stat.h> #include <fcntl.h> #define FIFO "/home/fifo" int main() { int fd,pid,line; char r_msg[BUFSIZ]; if ((pid = mkfifo(FIFO,0777)) == -1) { printf("fifo failed\n!"); return 1; } else printf("create success!"); fd = open(FIFO,O_RDWR); if(fd == -1) { printf("fifo failed!\n"); return 1; } if(write(fd,"hello world",12) == -1) { perror("write error"); return 1; } else printf("write success!\n"); if(read(fd,r_msg,BUFSIZ) == -1) { perror("read error"); return 1; } else printf("receive data id %s !\n",r_msg); close(fd); return 0; }
运行结果:
3 共享内存
shmget() 函数创建共享内存shmat() 函数将共享内存添加到进程地址中
shmdt() 进程退出共享内存
shmctl() 对内存区域的操作
#include <unistd.h> #include <stdio.h> #include <string.h> #include <sys/types.h> #include <sys/stat.h> #include <fcntl.h> #include <sys/ipc.h> #include <sys/shm.h> int main() { int shmid; int proj_id; key_t key; int size; char *addr; pid_t pid; key = IPC_PRIVATE; shmid = shmget(key,1024,IPC_CREAT|0660); if (shmid == -1) { perror("create share memory failed"); return 1; } addr = (char *)shmat(shmid,NULL,0); if (addr == (char *)(-1)) { perror("error!!!"); return 1; } printf("share memory address %s\n",addr); strcpy(addr,"welcome to mfcheer"); pid = fork(); if (pid == -1) { printf("error!\n"); return 1; } else if (pid == 0) { printf("chind process string is %s\n",addr); _exit(0); } else { printf("parent process string is %s\n",addr); if(shmdt (addr) == -1) { printf("release failed!\n"); return 1; } if (shmctl(shmid,IPC_RMID,NULL) == -1) { printf("erroe!\n"); return 1; } } return 0; }
运行结果:
4 信号量
semget() 创建信号量semop() 信号量操作
semctl() 信号量的控制
5 消息队列
相关文章推荐
- mysql5.6 centos7下安装之rpm安装
- 学习老男孩教育linux班级的计划书
- 每天进步一点点——Linux中的文件描述符与打开文件之间的关系
- Lsi卡和IB卡在CentOS中升级
- (OK) firefox - Adobe Flash Player 11.2 on Fedora 23/22, CentOS/RHEL 7.2/6.7/5.11
- 在CentOS中安装思维导图软件XMind
- Linux学习
- MV命令 及如何在linux系统中设置环境变量
- linux开机启动服务和chkconfig使用方法
- Linux流程解析二
- 树莓派LINUX 截屏
- yum安装于卸载软件常见命令
- linux下安装protobuf教程+示例(详细)
- Linux 学习
- linux的mysql权限错误导致看不到mysql数据库
- 守护进程
- Linux系统下修改环境变量PATH路径
- Linux用户管理与权限
- Linux常用命令汇总--tree
- Linux进程ID号--Linux进程的管理与调度(三)