android-使用AsyncTask做下载进度条
2016-05-04 22:54
435 查看
效果:
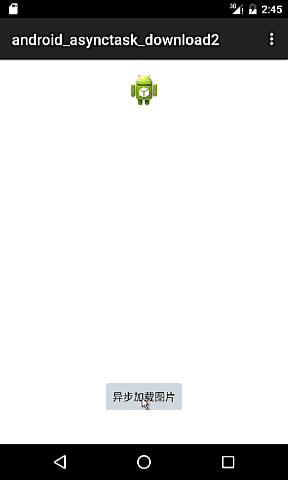
代码:
package com.example.android_asynctask_download2;
import android.support.v7.app.ActionBarActivity;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import android.app.ProgressDialog;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.AsyncTask;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
public class MainActivity extends ActionBarActivity {
private Button button;
private ImageView imageView;
private ProgressDialog dialog;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
button = (Button) this.findViewById(R.id.button1);
imageView = (ImageView) this.findViewById(R.id.imageView1);
dialog = new ProgressDialog(MainActivity.this);
dialog.setCancelable(false);// 让dialog不能失去焦点,一直在最上层显示
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 执行异步任务的操作
new MyAsynTask().execute(
"http://c.hiphotos.baidu.com/zhidao/pic/item/730e0cf3d7ca7bcb48f80cb9bc096b63f724a8a1.jpg");
}
});
}
/**
* 使用异步任务的规则: 1、生命一个集成AsyncTask标注三个参数的类型 2、第一个参数标识要执行的任务通常是网络的路径
* 3、第二个参数表示进度的刻度 4、第三个参数表示任务执行的结果
*/
public class MyAsynTask extends AsyncTask<String, Integer, Bitmap> {
// 表示任务执行之前的操作
@Override
protected void onPreExecute() {
super.onPreExecute();
dialog.setTitle("进度条");
dialog.setMessage("正在加载图片,请稍后...");
dialog.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL);
dialog.show();
}
// 主要完成耗时操作
@Override
protected Bitmap doInBackground(String... params) {
Bitmap bitmap = null;
InputStream inputStream = null;
try {
URL url = new URL(params[0]);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setConnectTimeout(3000);
connection.setRequestMethod("GET");
connection.setDoInput(true);
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
if (connection.getResponseCode() == 200) {
int file_length = connection.getContentLength();
inputStream = connection.getInputStream();
int len = 0;
byte[] data = new byte[1024];
int total_length = 0;
while ((len = inputStream.read(data)) != -1) {
total_length += len;
outputStream.write(data, 0, len);
int value = (int) ((total_length / (float) file_length) * 100);
publishProgress(value);// 通过publishProgress将进度传递到onProgressUpdate中对进度更新
}
bitmap = BitmapFactory.decodeByteArray(outputStream.toByteArray(), 0, outputStream.size());
}
} catch (Exception e) {
e.printStackTrace();
}finally
{
if(inputStream!=null)
try {
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return bitmap;
}
// 更新进度条操作
@Override
protected void onProgressUpdate(Integer... values) {
super.onProgressUpdate(values);
dialog.setProgress(values[0]);
}
// 主要更新UI操作
@Override
protected void onPostExecute(Bitmap result) {
super.onPostExecute(result);
imageView.setImageBitmap(result);
dialog.dismiss();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
代码:
package com.example.android_asynctask_download2;
import android.support.v7.app.ActionBarActivity;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import android.app.ProgressDialog;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.AsyncTask;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
public class MainActivity extends ActionBarActivity {
private Button button;
private ImageView imageView;
private ProgressDialog dialog;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
button = (Button) this.findViewById(R.id.button1);
imageView = (ImageView) this.findViewById(R.id.imageView1);
dialog = new ProgressDialog(MainActivity.this);
dialog.setCancelable(false);// 让dialog不能失去焦点,一直在最上层显示
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 执行异步任务的操作
new MyAsynTask().execute(
"http://c.hiphotos.baidu.com/zhidao/pic/item/730e0cf3d7ca7bcb48f80cb9bc096b63f724a8a1.jpg");
}
});
}
/**
* 使用异步任务的规则: 1、生命一个集成AsyncTask标注三个参数的类型 2、第一个参数标识要执行的任务通常是网络的路径
* 3、第二个参数表示进度的刻度 4、第三个参数表示任务执行的结果
*/
public class MyAsynTask extends AsyncTask<String, Integer, Bitmap> {
// 表示任务执行之前的操作
@Override
protected void onPreExecute() {
super.onPreExecute();
dialog.setTitle("进度条");
dialog.setMessage("正在加载图片,请稍后...");
dialog.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL);
dialog.show();
}
// 主要完成耗时操作
@Override
protected Bitmap doInBackground(String... params) {
Bitmap bitmap = null;
InputStream inputStream = null;
try {
URL url = new URL(params[0]);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setConnectTimeout(3000);
connection.setRequestMethod("GET");
connection.setDoInput(true);
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
if (connection.getResponseCode() == 200) {
int file_length = connection.getContentLength();
inputStream = connection.getInputStream();
int len = 0;
byte[] data = new byte[1024];
int total_length = 0;
while ((len = inputStream.read(data)) != -1) {
total_length += len;
outputStream.write(data, 0, len);
int value = (int) ((total_length / (float) file_length) * 100);
publishProgress(value);// 通过publishProgress将进度传递到onProgressUpdate中对进度更新
}
bitmap = BitmapFactory.decodeByteArray(outputStream.toByteArray(), 0, outputStream.size());
}
} catch (Exception e) {
e.printStackTrace();
}finally
{
if(inputStream!=null)
try {
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return bitmap;
}
// 更新进度条操作
@Override
protected void onProgressUpdate(Integer... values) {
super.onProgressUpdate(values);
dialog.setProgress(values[0]);
}
// 主要更新UI操作
@Override
protected void onPostExecute(Bitmap result) {
super.onPostExecute(result);
imageView.setImageBitmap(result);
dialog.dismiss();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
相关文章推荐
- 使用C++实现JNI接口需要注意的事项
- Android IPC进程间通讯机制
- Android Manifest 用法
- [转载]Activity中ConfigChanges属性的用法
- Android之获取手机上的图片和视频缩略图thumbnails
- Android之使用Http协议实现文件上传功能
- Android学习笔记(二九):嵌入浏览器
- android string.xml文件中的整型和string型代替
- i-jetty环境搭配与编译
- android之定时器AlarmManager
- android wifi 无线调试
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- android 代码实现控件之间的间距
- android FragmentPagerAdapter的“标准”配置
- Android"解决"onTouch和onClick的冲突问题
- android:installLocation简析
- android searchView的关闭事件
- SourceProvider.getJniDirectories