【Android动画九章】-RotateAnimation(旋转动画)和ScaleAnimation(尺寸动画)
2016-04-29 16:30
706 查看
public abstract class
Animation
extends Object
implements Cloneable
java.lang.Object
↳ android.view.animation.Animation
Known Direct Subclasses
AlphaAnimation, AnimationSet, RotateAnimation, ScaleAnimation, TranslateAnimation
同AlphaAnimation和TranslateAnimation一样,RotateAnimation和ScaleAnimation动画也都是Animation类的子类。这里一起进行介绍。
RotateAnimation(float fromDegrees, float toDegrees, int pivotXType, float pivotXValue, int pivotYType, float pivotYValue)
fromDegrees:起始角度值
toDegrees: 结束角度值
pivotXType: 转动点X轴的转动标准,共三种,RELATIVE_TO_SELF 以自己为标准,RELATIVE_TO_PARENT以父组件为标准,ABSOLUTE表示绝对位置。
pivotXValue: 针对上面标准的值,取值0-1之间。
pivotYType: 转动点Y轴的转动标准,也是三种,RELATIVE_TO_SELF 以自己为标准,RELATIVE_TO_PARENT以父组件为标准,ABSOLUTE表示绝对位置。
pivotYValue: 针对上面标准的值,取值0-1之间。
下面通过一个实例进行演示:
2.MainActivity.java:
RotateAnimation构造方法传入6个参数,运行项目实例如下:
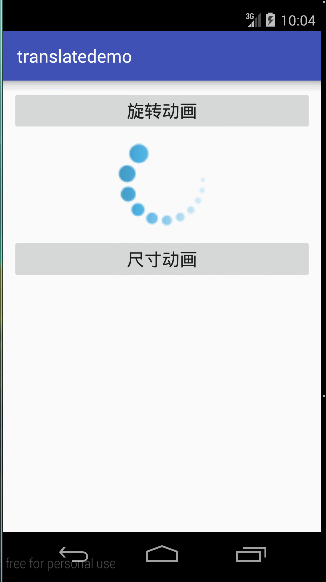
MainActivity代码如下:
运行项目实例:
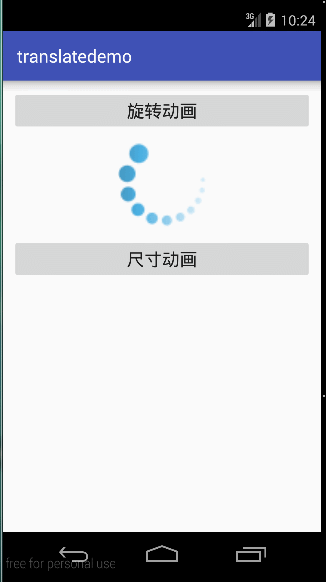
public ScaleAnimation (float fromX, float toX, float fromY, float toY, int pivotXType, float pivotXValue, int pivotYType, float pivotYValue)
fromX:表示起始时X轴方向上的大小,取值0-1。
toX :表示结束是X轴方向上的大小,其值0-1。
fromY:表示起始时Y轴方向上的大小,取值0-1。
toY :表示结束时Y轴方向上的大小,取值0-1。
pivotXType: 缩放点X轴的缩放标准,共三种,RELATIVE_TO_SELF 以自己为标准,RELATIVE_TO_PARENT以父组件为标准,ABSOLUTE表示绝对位置。
pivotXValue: 针对上面标准的值,取值0-1之间。
pivotYType: 缩放点Y轴的缩放标准,也是三种,RELATIVE_TO_SELF 以自己为标准,RELATIVE_TO_PARENT以父组件为标准,ABSOLUTE表示绝对位置。
pivotYValue: 针对上面标准的值,取值0-1之间。
下面通过一个实例进行演示:
构造方法需要传入8个参数,每个参数的含义在实例之前已经进行了介绍。
运行实例如下:
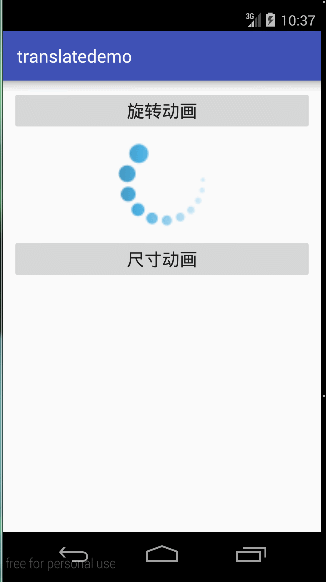
MainActivity.java代码修改如下:
运行项目如下:
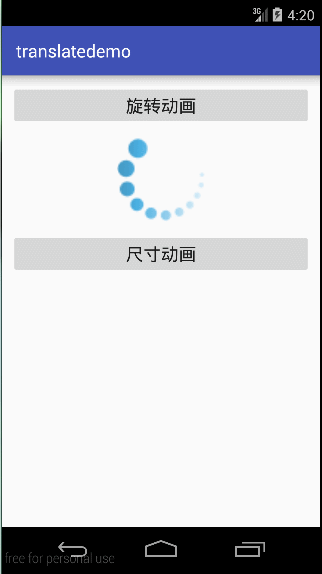
**喜欢的朋友请关注我,另欢迎阅读我的电子书
百度阅读:
http://yuedu.baidu.com/ebook/284b41a1e518964bce847c90?pn=1&click_type=10010002&rf=http%3A%2F%2Fblog.csdn.net%2Fyayun0516%2Farticle%2Fdetails%2F51277821
亚马逊:
http://www.amazon.cn/Android-%E7%99%BE%E6%88%98%E7%BB%8F%E5%85%B8-%E5%8D%B7I-%E5%BC%A0%E4%BA%9A%E8%BF%90/dp/B01ER5R9U2?ie=UTF8&keywords=Android%E7%BB%8F%E5%85%B8&qid=1461806976&ref_=sr_1_6&s=digital-text&sr=1-6**
Animation
extends Object
implements Cloneable
java.lang.Object
↳ android.view.animation.Animation
Known Direct Subclasses
AlphaAnimation, AnimationSet, RotateAnimation, ScaleAnimation, TranslateAnimation
同AlphaAnimation和TranslateAnimation一样,RotateAnimation和ScaleAnimation动画也都是Animation类的子类。这里一起进行介绍。
RotateAnimation
常用构造方法:RotateAnimation(float fromDegrees, float toDegrees, int pivotXType, float pivotXValue, int pivotYType, float pivotYValue)
fromDegrees:起始角度值
toDegrees: 结束角度值
pivotXType: 转动点X轴的转动标准,共三种,RELATIVE_TO_SELF 以自己为标准,RELATIVE_TO_PARENT以父组件为标准,ABSOLUTE表示绝对位置。
pivotXValue: 针对上面标准的值,取值0-1之间。
pivotYType: 转动点Y轴的转动标准,也是三种,RELATIVE_TO_SELF 以自己为标准,RELATIVE_TO_PARENT以父组件为标准,ABSOLUTE表示绝对位置。
pivotYValue: 针对上面标准的值,取值0-1之间。
下面通过一个实例进行演示:
代码方式实现
1.主布局文件:<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context="demo.androidwar.com.translatedemo.MainActivity"> <Button android:id="@+id/btn_rotate" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="10dp" android:gravity="center" android:onClick="rotate" android:text="旋转动画" android:textSize="20sp" /> <ImageView android:layout_gravity="center" android:id="@+id/imgview" android:layout_width="100dp" android:layout_height="100dp" android:src="@mipmap/load" /> </LinearLayout>
2.MainActivity.java:
package demo.androidwar.com.translatedemo; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.view.animation.Animation; import android.view.animation.RotateAnimation; import android.widget.ImageView; public class MainActivity extends AppCompatActivity { private ImageView imageView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); imageView = (ImageView) findViewById(R.id.imgview); } public void rotate(View view) { RotateAnimation rotateAnimation = new RotateAnimation(0, 360, Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f); imageView.setAnimation(rotateAnimation); rotateAnimation.setDuration(2000); imageView.startAnimation(rotateAnimation); } }
RotateAnimation构造方法传入6个参数,运行项目实例如下:
引入xml文件方式实现
xml代码如下:<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:interpolator/accelerate_decelerate"> <rotate android:duration="2000" android:fromDegrees="0" android:pivotX="50%" android:pivotY="50%" android:repeatCount="2" android:toDegrees="360" /> </set>
MainActivity代码如下:
package demo.androidwar.com.translatedemo; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.view.animation.Animation; import android.view.animation.AnimationUtils; import android.widget.ImageView; public class MainActivity extends AppCompatActivity { private ImageView imageView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); imageView = (ImageView) findViewById(R.id.imgview); } public void rotate(View view) { Animation rotateAnimation= AnimationUtils.loadAnimation(MainActivity.this,R.anim.rotate); imageView.setAnimation(rotateAnimation); imageView.startAnimation(rotateAnimation); } }
运行项目实例:
ScaleAnimation
尺寸动画,顾名思义,改变添加动画的对象的尺寸大小,其常用构造方法:public ScaleAnimation (float fromX, float toX, float fromY, float toY, int pivotXType, float pivotXValue, int pivotYType, float pivotYValue)
fromX:表示起始时X轴方向上的大小,取值0-1。
toX :表示结束是X轴方向上的大小,其值0-1。
fromY:表示起始时Y轴方向上的大小,取值0-1。
toY :表示结束时Y轴方向上的大小,取值0-1。
pivotXType: 缩放点X轴的缩放标准,共三种,RELATIVE_TO_SELF 以自己为标准,RELATIVE_TO_PARENT以父组件为标准,ABSOLUTE表示绝对位置。
pivotXValue: 针对上面标准的值,取值0-1之间。
pivotYType: 缩放点Y轴的缩放标准,也是三种,RELATIVE_TO_SELF 以自己为标准,RELATIVE_TO_PARENT以父组件为标准,ABSOLUTE表示绝对位置。
pivotYValue: 针对上面标准的值,取值0-1之间。
下面通过一个实例进行演示:
代码方式实现
MainActivity.java:package demo.androidwar.com.translatedemo; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.view.animation.Animation; import android.view.animation.AnimationUtils; import android.view.animation.ScaleAnimation; import android.widget.ImageView; public class MainActivity extends AppCompatActivity { private ImageView imageView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); imageView = (ImageView) findViewById(R.id.imgview); } public void rotate(View view) { Animation rotateAnimation= AnimationUtils.loadAnimation(MainActivity.this,R.anim.rotate); imageView.setAnimation(rotateAnimation); imageView.startAnimation(rotateAnimation); } public void scale(View view) { ScaleAnimation scaleAnimation=new ScaleAnimation(1f,0f,1f,0f,Animation.RELATIVE_TO_SELF,0.5f,Animation.RELATIVE_TO_SELF,0.5f); scaleAnimation.setDuration(2000); imageView.setAnimation(scaleAnimation); imageView.startAnimation(scaleAnimation); } }
构造方法需要传入8个参数,每个参数的含义在实例之前已经进行了介绍。
运行实例如下:
引入xml方式实现
xml代码如下:<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:interpolator/accelerate_decelerate"> <scale android:duration="2000" android:fromXScale="1" android:fromYScale="1" android:pivotX="50%" android:pivotY="50%" android:toXScale="0.1" android:toYScale="0.1" /> </set>
MainActivity.java代码修改如下:
package demo.androidwar.com.translatedemo; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.view.animation.Animation; import android.view.animation.AnimationUtils; import android.widget.ImageView; public class MainActivity extends AppCompatActivity { private ImageView imageView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); imageView = (ImageView) findViewById(R.id.imgview); } public void rotate(View view) { Animation rotateAnimation = AnimationUtils.loadAnimation(MainActivity.this, R.anim.rotate); imageView.setAnimation(rotateAnimation); imageView.startAnimation(rotateAnimation); } public void scale(View view) { Animation scaleAnimation = AnimationUtils.loadAnimation(MainActivity.this, R.anim.scale); imageView.setAnimation(scaleAnimation); scaleAnimation.setFillAfter(true); imageView.startAnimation(scaleAnimation); } }
运行项目如下:
**喜欢的朋友请关注我,另欢迎阅读我的电子书
百度阅读:
http://yuedu.baidu.com/ebook/284b41a1e518964bce847c90?pn=1&click_type=10010002&rf=http%3A%2F%2Fblog.csdn.net%2Fyayun0516%2Farticle%2Fdetails%2F51277821
亚马逊:
http://www.amazon.cn/Android-%E7%99%BE%E6%88%98%E7%BB%8F%E5%85%B8-%E5%8D%B7I-%E5%BC%A0%E4%BA%9A%E8%BF%90/dp/B01ER5R9U2?ie=UTF8&keywords=Android%E7%BB%8F%E5%85%B8&qid=1461806976&ref_=sr_1_6&s=digital-text&sr=1-6**
相关文章推荐
- 使用C++实现JNI接口需要注意的事项
- Android IPC进程间通讯机制
- Android Manifest 用法
- [转载]Activity中ConfigChanges属性的用法
- Android之获取手机上的图片和视频缩略图thumbnails
- Android之使用Http协议实现文件上传功能
- Android学习笔记(二九):嵌入浏览器
- android string.xml文件中的整型和string型代替
- i-jetty环境搭配与编译
- android之定时器AlarmManager
- android wifi 无线调试
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- android 代码实现控件之间的间距
- android FragmentPagerAdapter的“标准”配置
- Android"解决"onTouch和onClick的冲突问题
- android:installLocation简析
- android searchView的关闭事件
- SourceProvider.getJniDirectories