Android彩色二维码带logo
2016-04-12 09:49
453 查看
最近看到彩色二维码挺有意思,感觉我们常见的黑色二维码有些单调。

看了一下大牛们的博客,这里在大牛的基础上更改了一下。做了一个有颜色渐变的二维码。
在这里记录一下自己的学习成果。

我这里用的是zxing-4.7.5.jar。
效果图如下:
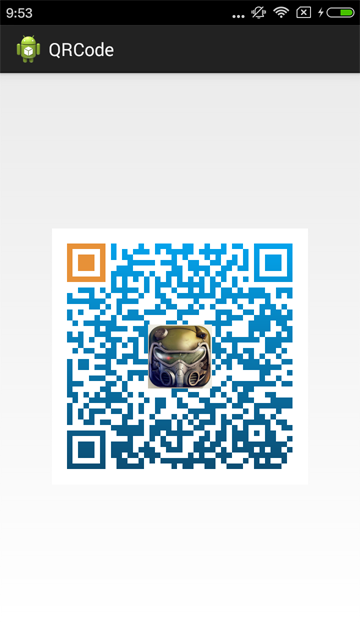
直接上代码了
相关文章:
http://www.open-open.com/lib/view/open1433399323182.html
http://blog.csdn.net/cq1982/article/details/45620741
http://blog.csdn.net/gao36951/article/details/41149049
http://wenku.baidu.com/link?url=kYJw8F8uQmMlOuo3zmg4Sx886RiCiglOAis55Kf5OjqtxSgnN55Ce5OLzYjuWsaZrOBKMyDUFAcwbXXjveXotZ9Q_bHEBPlQ7LuldY0LUYi

看了一下大牛们的博客,这里在大牛的基础上更改了一下。做了一个有颜色渐变的二维码。
在这里记录一下自己的学习成果。

我这里用的是zxing-4.7.5.jar。
效果图如下:
直接上代码了
package com.yaojian.qrcode; import java.util.Hashtable; import android.graphics.Bitmap; import android.graphics.Canvas; import android.graphics.Color; import android.graphics.Paint; import android.graphics.PaintFlagsDrawFilter; import com.google.zxing.BarcodeFormat; import com.google.zxing.EncodeHintType; import com.google.zxing.WriterException; import com.google.zxing.common.BitMatrix; import com.google.zxing.qrcode.QRCodeWriter; import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel; /** * @ClassName: QRCodeUtils * @Description: zxing生成二维码工具,可带logo,可调颜色。 * @author yaojian */ public class QRCodeUtils { /** * 根据指定内容生成自定义宽高的二维码图片 * * param content 需要生成二维码的内容 * param width 二维码宽度 * param height 二维码高度 */ public static Bitmap makeQRImage(String content, int QR_WIDTH, int QR_HEIGHT) { return makeQRImage(content, QR_WIDTH, QR_HEIGHT, null); } /** * 根据指定内容生成自定义宽高的二维码图片 * * param logoBm logo图标 * param content 需要生成二维码的内容 * param width 二维码宽度 * param height 二维码高度 */ public static Bitmap makeQRImage(String content, int QR_WIDTH, int QR_HEIGHT, Bitmap logoBmp) { try { // 图像数据转换,使用了矩阵转换 Hashtable hints = new Hashtable(); hints.put(EncodeHintType.CHARACTER_SET, "utf-8"); hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H);// 容错级别 // 设置空白边距的宽度 hints.put(EncodeHintType.MARGIN, 2); // default is 4 hints.put(EncodeHintType.MAX_SIZE, 350); hints.put(EncodeHintType.MIN_SIZE, 100); BitMatrix bitMatrix = new QRCodeWriter().encode(content, BarcodeFormat.QR_CODE, QR_WIDTH, QR_HEIGHT, hints); int[] pixels = new int[QR_WIDTH * QR_HEIGHT]; // 下面这里按照二维码的算法,逐个生成二维码的图片 // 两个for循环是图片横列扫描的结果 // 设置二维码颜色渐变 int[] rgb = setGradientColor("#03a9f4"); for (int y = 0; y < QR_HEIGHT; y++) { for (int x = 0; x < QR_WIDTH; x++) { if (bitMatrix.get(x, y)) { // 二维码颜色设置 if (x > 0 && x < 110 && y > 0 && y < 110) { Color color = new Color(); int colorInt = color.parseColor("#e79138"); pixels[y * QR_WIDTH + x] = bitMatrix.get(x, y) ? colorInt : 16777215; } else { // 设置二维码颜色渐变 int r = (int) (rgb[0] - (rgb[0] - 13.0) / bitMatrix.getHeight() * (y + 1)); int g = (int) (rgb[1] - (rgb[1] - 72.0) / bitMatrix.getHeight() * (y + 1)); int b = (int) (rgb[2] - (rgb[2] - 107.0) / bitMatrix.getHeight() * (y + 1)); Color color = new Color(); int colorInt = color.argb(255, r, g, b); pixels[y * QR_WIDTH + x] = bitMatrix.get(x, y) ? colorInt : 16777215; } } else { pixels[y * QR_WIDTH + x] = 0xffffffff;// 白色 } } } // ------------------添加logo部分------------------// Bitmap bitmap = Bitmap.createBitmap(QR_WIDTH, QR_HEIGHT, Bitmap.Config.ARGB_8888); // 设置像素点 bitmap.setPixels(pixels, 0, QR_WIDTH, 0, 0, QR_WIDTH, QR_HEIGHT); if (logoBmp == null) { return bitmap; } // 获取图片宽高 int logoWidth = logoBmp.getWidth(); int logoHeight = logoBmp.getHeight(); if (QR_WIDTH == 0 || QR_HEIGHT == 0) { return null; } if (logoWidth == 0 || logoHeight == 0) { return bitmap; } // 图片绘制在二维码中央,合成二维码图片 // logo大小为二维码整体大小的1/4 float scaleFactor = QR_WIDTH * 1.0f / 4 / logoWidth; try { Canvas canvas = new Canvas(bitmap); canvas.setDrawFilter(new PaintFlagsDrawFilter(0, Paint.ANTI_ALIAS_FLAG | Paint.FILTER_BITMAP_FLAG)); canvas.drawBitmap(bitmap, 0, 0, null); canvas.scale(scaleFactor, scaleFactor, QR_WIDTH / 2, QR_HEIGHT / 2); canvas.drawBitmap(logoBmp, (QR_WIDTH - logoWidth) / 2, (QR_HEIGHT - logoHeight) / 2, null); canvas.save(Canvas.ALL_SAVE_FLAG); canvas.restore(); return bitmap; } catch (Exception e) { bitmap = null; e.getStackTrace(); } } catch (WriterException e) { e.printStackTrace(); } return null; } private static int[] setGradientColor(String colorStr) { int[] rgbs = new int[3]; rgbs[0] = Integer.parseInt(colorStr.substring(1, 3), 16); rgbs[1] = Integer.parseInt(colorStr.substring(3, 5), 16); rgbs[2] = Integer.parseInt(colorStr.substring(5, 7), 16); return rgbs; } }
相关文章:
http://www.open-open.com/lib/view/open1433399323182.html
http://blog.csdn.net/cq1982/article/details/45620741
http://blog.csdn.net/gao36951/article/details/41149049
http://wenku.baidu.com/link?url=kYJw8F8uQmMlOuo3zmg4Sx886RiCiglOAis55Kf5OjqtxSgnN55Ce5OLzYjuWsaZrOBKMyDUFAcwbXXjveXotZ9Q_bHEBPlQ7LuldY0LUYi
相关文章推荐
- 使用C++实现JNI接口需要注意的事项
- Android IPC进程间通讯机制
- Android Manifest 用法
- [转载]Activity中ConfigChanges属性的用法
- Android之获取手机上的图片和视频缩略图thumbnails
- Android之使用Http协议实现文件上传功能
- Android学习笔记(二九):嵌入浏览器
- android string.xml文件中的整型和string型代替
- i-jetty环境搭配与编译
- android之定时器AlarmManager
- android wifi 无线调试
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- android 代码实现控件之间的间距
- android FragmentPagerAdapter的“标准”配置
- Android"解决"onTouch和onClick的冲突问题
- android:installLocation简析
- android searchView的关闭事件
- SourceProvider.getJniDirectories