获取输入法的高度/让输入框正好在输入法之上
2016-03-29 22:08
513 查看
此功能类似于QQ或者微信的输入框,当输入框获取焦点时,输入法弹出,输入框自动上移并且正好保持在输入法的上面
最终效果图如下
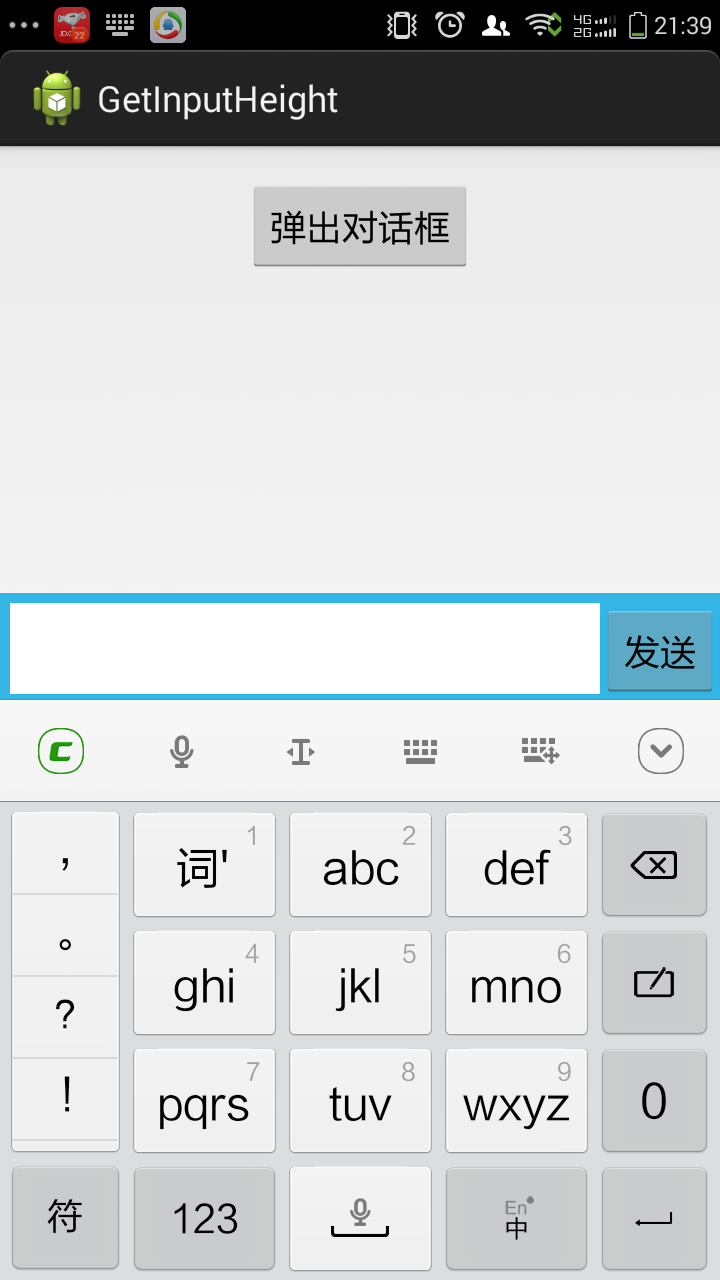
整体思路就是重写一个布局文件,然后再布局布局发生变化时(即输入法弹起)onmeasure()方法中获取现在的布局尺寸,得到输入法的高度
思路不难,下面看看代码 注意一些细节
主activity布局文件
输入框布局用了Popupwindows,界面很简单代码如下
清单配置文件也贴一下 主要是输入面板的弹出模式要注意一下,另外,application不要忘记注册
自定义布局文件代码
Application:
主Activity代码
PopupWindows代码
以上就是主要代码,又需要的同学可以凑合看下
http://download.csdn.net/detail/lantiankongmo/9476028
最终效果图如下
整体思路就是重写一个布局文件,然后再布局布局发生变化时(即输入法弹起)onmeasure()方法中获取现在的布局尺寸,得到输入法的高度
思路不难,下面看看代码 注意一些细节
主activity布局文件
<com.example.getinputheight.KeyboardDetectorRelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" xmlns:custom="http://schemas.android.com/apk/res/com.example.getinputheight" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:id="@+id/root_layout" tools:context=".MainActivity" > <Button android:id="@+id/btn_h" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" android:text="弹出对话框" /> </com.example.getinputheight.KeyboardDetectorRelativeLayout>
输入框布局用了Popupwindows,界面很简单代码如下
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="40dp" android:background="@android:color/holo_blue_light" android:orientation="horizontal" > <RelativeLayout android:id="@+id/select_layout" android:layout_width="match_parent" android:layout_height="wrap_content" > <EditText android:id="@+id/input_et" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="5dp" android:layout_marginRight="60dp" android:layout_marginTop="5dp" android:background="@android:color/white" android:focusable="true" android:layout_marginLeft="5dp" android:focusableInTouchMode="true" android:lines="2" > <requestFocus /> </EditText> <Button android:id="@+id/send_btn" android:layout_width="60dp" android:layout_height="wrap_content" android:layout_alignParentRight="true" android:layout_alignTop="@+id/input_et" android:text="发送" /> </RelativeLayout> </RelativeLayout>
清单配置文件也贴一下 主要是输入面板的弹出模式要注意一下,另外,application不要忘记注册
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.getinputheight" android:versionCode="1" android:versionName="1.0"> <uses-sdk android:minSdkVersion="14" android:targetSdkVersion="17" /> <application android:name="com.example.getinputheight.MyApplication" android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme"> <activity android:name="com.example.getinputheight.MainActivity" /> //注意这里 android:windowSoftInputMode="adjustResize|stateHidden" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
自定义布局文件代码
package com.example.getinputheight; import android.app.Activity; import android.content.Context; import android.content.SharedPreferences; import android.util.AttributeSet; import android.util.Log; import android.widget.RelativeLayout; public class KeyboardDetectorRelativeLayout extends RelativeLayout { private OnSoftKeyboardListener listener; public KeyboardDetectorRelativeLayout(Context context) { this(context, null); // TODO Auto-generated constructor stub } public KeyboardDetectorRelativeLayout(Context context, AttributeSet attrs) { this(context, attrs, 0); } public KeyboardDetectorRelativeLayout(Context context, AttributeSet attrs, int defStyle) { super(context, attrs, defStyle); } //获取输入法的高度主要在此方法中执行,当界面布局发生变化时,会执行此方法,从而获取到输入法的高度 @Override protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) { // TODO Auto-generated method stub final int proposedHeight = MeasureSpec.getSize(heightMeasureSpec); final int actualHeight = getHeight(); int diff = actualHeight - proposedHeight; Log.i("TAG", "proposedHeight=" + proposedHeight); Log.i("TAG", "actualHeight=" + actualHeight); Log.i("TAG", "diff=" + diff); if (diff > 100) { Log.i("TAG", MyApplication.context.toString()); SharedPreferences sh = MyApplication.context.getSharedPreferences("in_put", Context.MODE_PRIVATE); SharedPreferences.Editor editor = sh.edit(); editor.putInt("size", diff); Log.i("TAG", "size=" + diff); editor.commit(); if (null != listener) { listener.onShown(diff); } } else if (diff < -100) { if (null != listener) { listener.onHidden(); } } super.onMeasure(widthMeasureSpec, heightMeasureSpec); if (null != listener) { listener.onMeasureFinished(); } } public void setOnSoftKeyboardListener(OnSoftKeyboardListener listener) { this.listener = listener; } //输入法面板弹出。隐藏以及布局测量完毕执行的方法 interface OnSoftKeyboardListener { void onShown(int keyboardHeight); void onHidden(); void onMeasureFinished(); } }
Application:
package com.example.getinputheight; import android.app.Application; import android.content.Context; import android.util.Log; public class MyApplication extends Application { static Context context; @Override public void onCreate() { // TODO Auto-generated method stub context = getApplicationContext(); super.onCreate(); } }
主Activity代码
package com.example.getinputheight; import com.example.getinputheight.KeyboardDetectorRelativeLayout.OnSoftKeyboardListener; import android.os.Bundle; import android.app.Activity; import android.content.Context; import android.content.SharedPreferences; import android.util.Log; import android.view.Gravity; import android.view.Menu; import android.view.MotionEvent; import android.view.View; import android.view.View.OnClickListener; import android.view.inputmethod.InputMethodManager; import android.widget.Button; import android.widget.EditText; import android.widget.RelativeLayout; import android.widget.Toast; public class MainActivity extends Activity { KeyboardDetectorRelativeLayout root_layout; EditText et; Button btn; InputContentPopupWindon popu; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); btn = (Button) findViewById(R.id.btn_h); btn.setOnClickListener(new OnClickListener() { @Override public void onClick(View v) { // TODO Auto-generated method stub InputMethodManager manager = (InputMethodManager) getSystemService(INPUT_METHOD_SERVICE); manager.showSoftInput(v, InputMethodManager.RESULT_SHOWN); manager.toggleSoftInput(InputMethodManager.SHOW_FORCED, InputMethodManager.HIDE_IMPLICIT_ONLY); } }); root_layout = (KeyboardDetectorRelativeLayout) findViewById(R.id.root_layout); root_layout.setOnSoftKeyboardListener(new OnSoftKeyboardListener() { @Override public void onShown(int keyboardHeight) { // TODO Auto-generated method stub showPopupwin(); } @Override public void onMeasureFinished() { // TODO Auto-generated method stub } @Override public void onHidden() { // TODO Auto-generated method stub if (popu != null) { popu.dismiss(); } } }); } public void showPopupwin() { popu = new InputContentPopupWindon(this, listener); popu.setOutsideTouchable(false);//点击屏幕意外位置消失 popu.setFocusable(true); SharedPreferences pref = this.getSharedPreferences("in_put", Context.MODE_PRIVATE); int size = pref.getInt("size", 0); Log.i("TAG", "输入框的高度为==" + size); popu.showAtLocation(this.findViewById(R.id.root_layout), Gravity.BOTTOM | Gravity.CENTER_HORIZONTAL, 0, size); } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.main, menu); return true; } OnClickListener listener = new OnClickListener() { @Override public void onClick(View v) { switch (v.getId()) { case R.id.send_btn: Toast.makeText(MainActivity.this, ((EditText) (popu.getContentView().findViewById(R.id.input_et))).getText().toString(), 0).show(); //点击发送 强制隐藏输入法面板 v.requestFocus(); InputMethodManager manager = (InputMethodManager) getSystemService(INPUT_METHOD_SERVICE); manager.hideSoftInputFromWindow(v.getWindowToken(), 0); //隐藏 break; default: break; } } }; //切回桌面时隐藏输入面板 protected void onPause() { super.onPause(); InputMethodManager manager = (InputMethodManager) getSystemService(INPUT_METHOD_SERVICE); //强制隐藏 manager.hideSoftInputFromWindow(popu.getContentView().findViewById(R.id.input_et).getWindowToken(), 0); } ; //界面重新可见时如果popupwindows还可见,手动dismiss @Override protected void onResume() { // TODO Auto-generated method stub super.onResume(); if (popu != null) { popu.dismiss(); } } }
PopupWindows代码
package com.example.getinputheight; import android.content.Context; import android.content.IntentSender.SendIntentException; import android.graphics.drawable.ColorDrawable; import android.support.v4.view.ViewPager.LayoutParams; import android.view.LayoutInflater; import android.view.MotionEvent; import android.view.View; import android.view.View.OnClickListener; import android.view.View.OnTouchListener; import android.widget.Button; import android.widget.PopupWindow; public class InputContentPopupWindon extends PopupWindow { Context context; OnClickListener listener; View layout; Button btn; public InputContentPopupWindon(Context context, OnClickListener listener) { this.context = context; this.listener = listener; LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE); layout = inflater.inflate(R.layout.input_layout, null); btn = (Button) layout.findViewById(R.id.send_btn); btn.setOnClickListener(listener); this.setContentView(layout); this.setWidth(LayoutParams.MATCH_PARENT);//设置宽度 this.setHeight(LayoutParams.WRAP_CONTENT);//设置高度 ColorDrawable dw = new ColorDrawable(0xb0000000); // this.setBackgroundDrawable(dw);//设置背景 // layout.setOnTouchListener(new OnTouchListener() { // // @Override // public boolean onTouch(View v, MotionEvent event) { // // TODO Auto-generated method stub // int height = layout.findViewById(R.id.select_layout).getTop(); // int y = (int) event.getY(); // if(event.getAction()==MotionEvent.ACTION_DOWN){ // if(y>height){ // dismiss(); // } // } // return false; // } // }); } }
以上就是主要代码,又需要的同学可以凑合看下
http://download.csdn.net/detail/lantiankongmo/9476028
相关文章推荐
- Android布局的小窍门?
- Web布局连载——两栏固定布局(五)
- 样式表CSS布局经验
- 在winform下实现左右布局多窗口界面的方法之续篇
- QQ输入法自动删除其它输入法的解决方法
- Access中字段上自动打开的输入法的解决方法
- C#中Winfrom默认输入法的设置方法
- css网页布局中注意的几个问题小结
- DL.DT.DD实现左右的布局简单例子第1/2页
- 使用CSS框架布局的缺点和优点小结
- div+CSS网页布局的意义与副作用原因小结第1/2页
- 在winform下实现左右布局多窗口界面的方法
- Android编程之代码创建布局实例分析
- CSS顶级技巧大放送,div+css布局必知
- 用div实现像table一样的布局方法
- 精彩的Bootstrap案例分享 重点在注释!(选项卡、栅格布局)
- jQuery EasyUi实战教程之布局篇
- jQuery EasyUI 布局之动态添加tabs标签页
- jQuery Easyui实现左右布局