Servlet和Jsp实现原生的上传与下载文件
2016-03-16 00:00
896 查看
摘要: 有兴趣的朋友可以试试,以后做小型项目可以直接拿来用了。
1.01.jsp 前台代码
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP '01.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
<form action="uploadServlet.do" method="post" enctype="multipart/form-data">//
<!--这里注意下 表单中enctype="multipart/form-data"的意思,是设置表单的MIME编码。默认情况,这个编码格式是application/x-www-form-urlencoded,不能用于文件上传;只有使用了multipart/form-data,才能完整的传递文件数据,进行下面的操作.
enctype="multipart/form-data"是上传二进制数据; form里面的input的值以2进制的方式传过去 -->
请选择文件:<input id="myfile" name="myfile" type="file" onchange="showPreview(this)"/>
<input type="submit" value="提交" />${result}
</form>
下载:<a href="downloadServlet.do?filename=xxx.txt">xxx.txt</a> ${errorResult}//下载文件这里名字我写死了,但是用JS方法应该能实现动态下载文件功能
<div id="large"></div>
</body>
</html>
2.后台代码 UploadServlet.java
package com.imooc.servlet;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.RandomAccessFile;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class UploadServlet extends HttpServlet {
public void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
doPost(req,resp);
}
public void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
//req.setCharacterEncoding("text/html;charset=UTF-8");
//从request当中获取流信息
InputStream fileSource = req.getInputStream();..
String tempFileName = "E:/tempFile";
//tempFile指向临时文件
File tempFile = new File(tempFileName);
//outputStram文件输出流指向这个临时文件
FileOutputStream outputStream = new FileOutputStream(tempFile);
byte b[] = new byte[1024];
int n;
while(( n = fileSource.read(b)) != -1){
outputStream.write(b, 0, n);
}
//关闭输出流、输入流
outputStream.close();
fileSource.close();
//获取上传文件的名称
RandomAccessFile randomFile = new RandomAccessFile(tempFile,"r");//RandomAccessFile是用来访问那些保存数据记录的文件的,第二个参数“r”表示只读。
randomFile.readLine();//读取第一行数据
String str = randomFile.readLine();//读取第二行数据,为什么读取了两次,看临时文件的保存的内容便知,第一行,和最后一行都是无用的内容数据,所以直接从第二行读取。
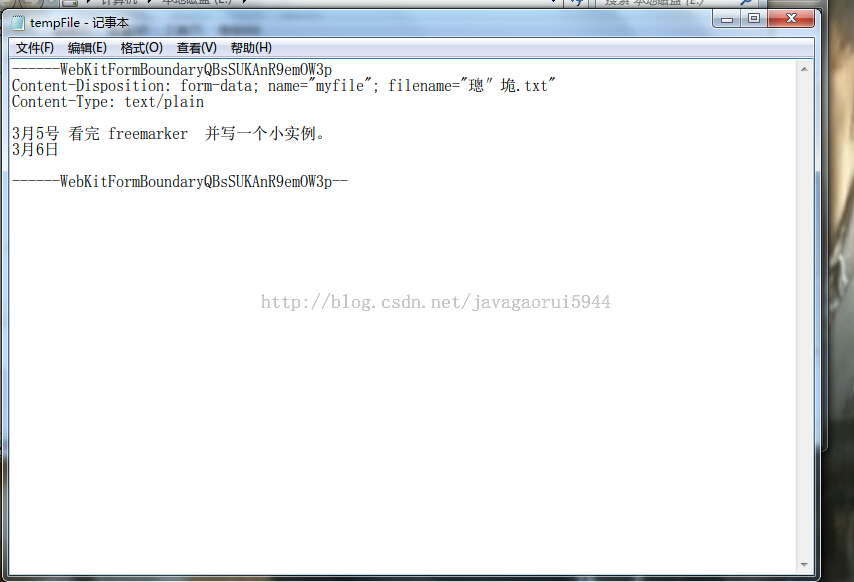
String str1 = str.substring(0,str.lastIndexOf("\""));//根据最后一个引号来定位
int endIndex = str.lastIndexOf("\"");
int beginIndex = str1.lastIndexOf("\"")+1 ;//beginIndex,endIndex,得到文件name 的初始位置。
String filename = str.substring(beginIndex, endIndex);
filename=new String(filename.getBytes("ISO-8859-1"),"utf-8");//因为从记事本中读取出来的是ISO-8859-1,所以需要转码,防止乱码
System.out.println("filename:" + filename);
//重新定位文件指针到文件头
randomFile.seek(0);// seek方法可以在文件中移动。
long startPosition = 0;
int i = 1;
//获取文件内容 开始位置
while(( n = randomFile.readByte()) != -1 && i <=4){
if(n == '\n'){
startPosition = randomFile.getFilePointer()//.getFilePointer()用于定位
i ++;
}
}
startPosition = randomFile.getFilePointer() -1;
//获取文件内容 结束位置
randomFile.seek(randomFile.length());
long endPosition = randomFile.getFilePointer();
int j = 1;
while(endPosition >=0 && j<=2){
endPosition--;
randomFile.seek(endPosition);
if(randomFile.readByte() == '\n'){
j++;
}
}
endPosition = endPosition -1;
//设置保存上传文件的路径
String realPath = getServletContext().getRealPath("/") + "images";//为文件保存的绝对路径
File fileupload = new File(realPath);
if(!fileupload.exists()){
fileupload.mkdir();
}
File saveFile = new File(realPath,filename);
RandomAccessFile randomAccessFile = new RandomAccessFile(saveFile,"rw");
//从临时文件当中读取文件内容(根据起止位置获取)
randomFile.seek(startPosition);
while(startPosition < endPosition){
randomAccessFile.write(randomFile.readByte());
startPosition = randomFile.getFilePointer();
}
//关闭输入输出流、删除临时文件
randomAccessFile.close();
randomFile.close();
/* tempFile.delete();*/ //我觉得不必关闭,
req.setAttribute("result", "上传成功!");
RequestDispatcher dispatcher = req.getRequestDispatcher("jsp/01.jsp");
dispatcher.forward(req, resp);
}
}
3.DownloadServlet.java 下载文件
package com.imooc.servlet;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class DownloadServlet extends HttpServlet {
public void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
//获取文件下载路径
String path = getServletContext().getRealPath("/") + "images/";
String filename = req.getParameter("filename");
filename=new String(filename.getBytes("ISO-8859-1"),"utf-8");
System.out.println(filename);
System.out.println("filename:"+filename);
File file = new File(path + filename);
if(file.exists()){
//设置相应类型application/octet-stream
resp.setContentType("application/x-msdownload");
//设置头信息
filename = new String(filename.getBytes("utf-8"),"ISO-8859-1");//将数据写入到记事本,需要转码,这和上传文件刚好那个步骤刚好相反
resp.setHeader("Content-Disposition", "attachment;filename=\"" + filename + "\"");
InputStream inputStream = new FileInputStream(file);
ServletOutputStream ouputStream = resp.getOutputStream();
byte b[] = new byte[1024];
int n ;
while((n = inputStream.read(b)) != -1){
ouputStream.write(b,0,n);
}
//关闭流、释放资源
ouputStream.close();
inputStream.close();
}else{
req.setAttribute("errorResult", "文件不存在下载失败!");
RequestDispatcher dispatcher = req.getRequestDispatcher("jsp/01.jsp");
dispatcher.forward(req, resp);
}
}
public void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
doGet(req,resp);
}
}
项目目录
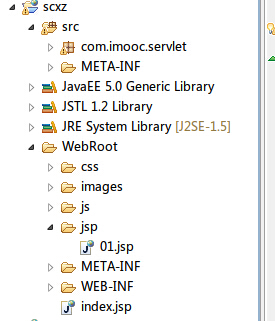
配置文件
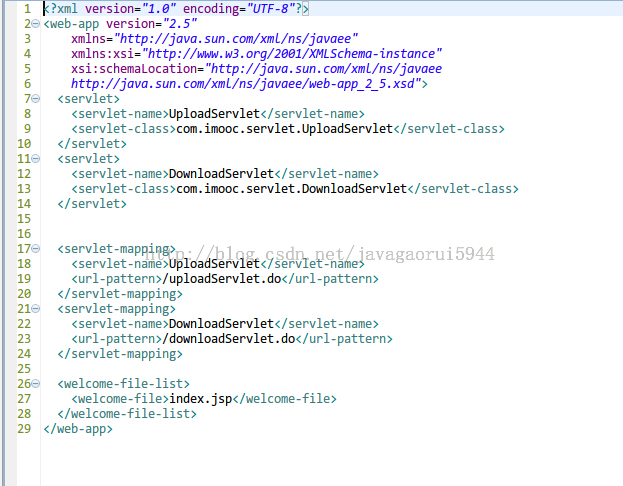
有兴趣的朋友可以试试,以后做小型项目可以直接拿来用了。
1.01.jsp 前台代码
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP '01.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
<form action="uploadServlet.do" method="post" enctype="multipart/form-data">//
<!--这里注意下 表单中enctype="multipart/form-data"的意思,是设置表单的MIME编码。默认情况,这个编码格式是application/x-www-form-urlencoded,不能用于文件上传;只有使用了multipart/form-data,才能完整的传递文件数据,进行下面的操作.
enctype="multipart/form-data"是上传二进制数据; form里面的input的值以2进制的方式传过去 -->
请选择文件:<input id="myfile" name="myfile" type="file" onchange="showPreview(this)"/>
<input type="submit" value="提交" />${result}
</form>
下载:<a href="downloadServlet.do?filename=xxx.txt">xxx.txt</a> ${errorResult}//下载文件这里名字我写死了,但是用JS方法应该能实现动态下载文件功能
<div id="large"></div>
</body>
</html>
2.后台代码 UploadServlet.java
package com.imooc.servlet;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.RandomAccessFile;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class UploadServlet extends HttpServlet {
public void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
doPost(req,resp);
}
public void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
//req.setCharacterEncoding("text/html;charset=UTF-8");
//从request当中获取流信息
InputStream fileSource = req.getInputStream();..
String tempFileName = "E:/tempFile";
//tempFile指向临时文件
File tempFile = new File(tempFileName);
//outputStram文件输出流指向这个临时文件
FileOutputStream outputStream = new FileOutputStream(tempFile);
byte b[] = new byte[1024];
int n;
while(( n = fileSource.read(b)) != -1){
outputStream.write(b, 0, n);
}
//关闭输出流、输入流
outputStream.close();
fileSource.close();
//获取上传文件的名称
RandomAccessFile randomFile = new RandomAccessFile(tempFile,"r");//RandomAccessFile是用来访问那些保存数据记录的文件的,第二个参数“r”表示只读。
randomFile.readLine();//读取第一行数据
String str = randomFile.readLine();//读取第二行数据,为什么读取了两次,看临时文件的保存的内容便知,第一行,和最后一行都是无用的内容数据,所以直接从第二行读取。
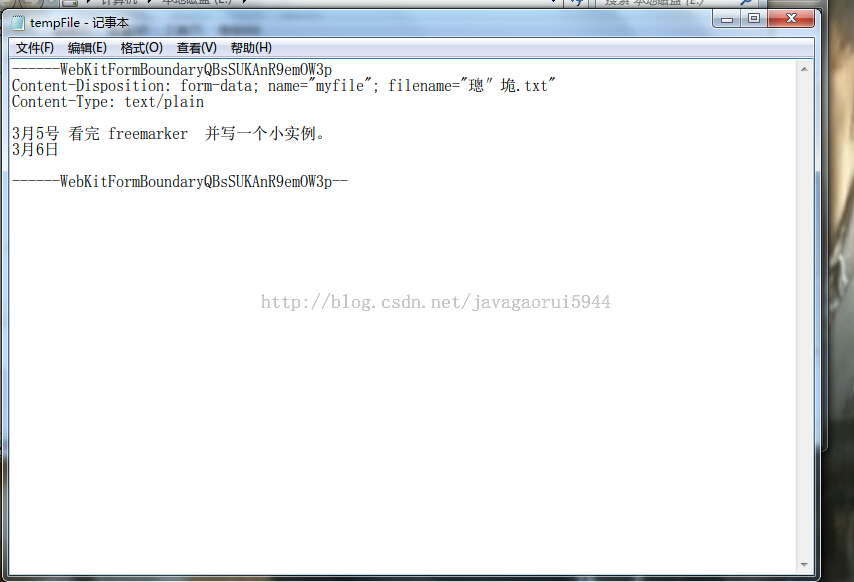
String str1 = str.substring(0,str.lastIndexOf("\""));//根据最后一个引号来定位
int endIndex = str.lastIndexOf("\"");
int beginIndex = str1.lastIndexOf("\"")+1 ;//beginIndex,endIndex,得到文件name 的初始位置。
String filename = str.substring(beginIndex, endIndex);
filename=new String(filename.getBytes("ISO-8859-1"),"utf-8");//因为从记事本中读取出来的是ISO-8859-1,所以需要转码,防止乱码
System.out.println("filename:" + filename);
//重新定位文件指针到文件头
randomFile.seek(0);// seek方法可以在文件中移动。
long startPosition = 0;
int i = 1;
//获取文件内容 开始位置
while(( n = randomFile.readByte()) != -1 && i <=4){
if(n == '\n'){
startPosition = randomFile.getFilePointer()//.getFilePointer()用于定位
i ++;
}
}
startPosition = randomFile.getFilePointer() -1;
//获取文件内容 结束位置
randomFile.seek(randomFile.length());
long endPosition = randomFile.getFilePointer();
int j = 1;
while(endPosition >=0 && j<=2){
endPosition--;
randomFile.seek(endPosition);
if(randomFile.readByte() == '\n'){
j++;
}
}
endPosition = endPosition -1;
//设置保存上传文件的路径
String realPath = getServletContext().getRealPath("/") + "images";//为文件保存的绝对路径
File fileupload = new File(realPath);
if(!fileupload.exists()){
fileupload.mkdir();
}
File saveFile = new File(realPath,filename);
RandomAccessFile randomAccessFile = new RandomAccessFile(saveFile,"rw");
//从临时文件当中读取文件内容(根据起止位置获取)
randomFile.seek(startPosition);
while(startPosition < endPosition){
randomAccessFile.write(randomFile.readByte());
startPosition = randomFile.getFilePointer();
}
//关闭输入输出流、删除临时文件
randomAccessFile.close();
randomFile.close();
/* tempFile.delete();*/ //我觉得不必关闭,
req.setAttribute("result", "上传成功!");
RequestDispatcher dispatcher = req.getRequestDispatcher("jsp/01.jsp");
dispatcher.forward(req, resp);
}
}
3.DownloadServlet.java 下载文件
package com.imooc.servlet;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class DownloadServlet extends HttpServlet {
public void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
//获取文件下载路径
String path = getServletContext().getRealPath("/") + "images/";
String filename = req.getParameter("filename");
filename=new String(filename.getBytes("ISO-8859-1"),"utf-8");
System.out.println(filename);
System.out.println("filename:"+filename);
File file = new File(path + filename);
if(file.exists()){
//设置相应类型application/octet-stream
resp.setContentType("application/x-msdownload");
//设置头信息
filename = new String(filename.getBytes("utf-8"),"ISO-8859-1");//将数据写入到记事本,需要转码,这和上传文件刚好那个步骤刚好相反
resp.setHeader("Content-Disposition", "attachment;filename=\"" + filename + "\"");
InputStream inputStream = new FileInputStream(file);
ServletOutputStream ouputStream = resp.getOutputStream();
byte b[] = new byte[1024];
int n ;
while((n = inputStream.read(b)) != -1){
ouputStream.write(b,0,n);
}
//关闭流、释放资源
ouputStream.close();
inputStream.close();
}else{
req.setAttribute("errorResult", "文件不存在下载失败!");
RequestDispatcher dispatcher = req.getRequestDispatcher("jsp/01.jsp");
dispatcher.forward(req, resp);
}
}
public void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
doGet(req,resp);
}
}
项目目录
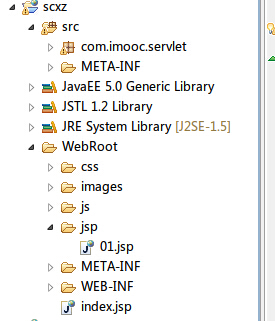
配置文件
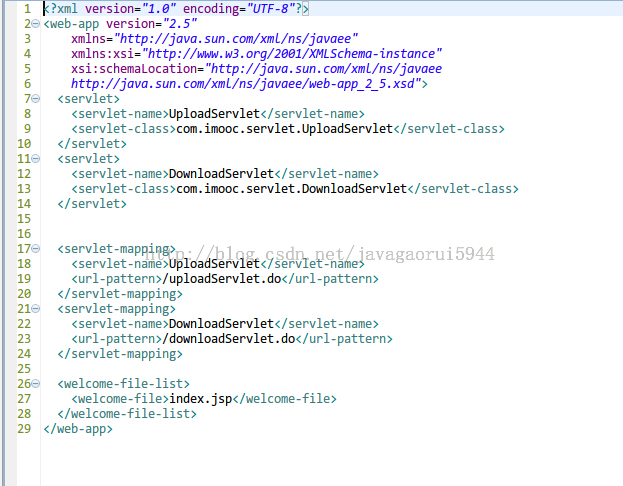
有兴趣的朋友可以试试,以后做小型项目可以直接拿来用了。
相关文章推荐
- JSP/PHP基于Ajax的分页功能实现
- 开发阶段Jetty运行Jsp报错且响应空白
- jsp简单实现页面之间共享信息的方法
- Apache Web让JSP“动”起来
- JSP学习经验小结分享
- JSP 多条SQL语句同时执行的方法
- 在jsp页面中响应速度提高的7种方法分享
- (jsp/html)网页上嵌入播放器(常用播放器代码整理)
- JSP上传excel及excel插入至数据库的方法
- JSP中文乱码常见3个例子及其解决方法
- jsp实现将动态网页转换成静态页面的方法
- JSP避免Form重复提交的三种方案
- 纯jsp实现的倒计时动态显示效果完整代码
- JSP自定义标签入门学习
- JSP实现添加功能和分页显示实例分析
- JSP中param标签用法实例分析
- JSP实现从不同服务器上下载文件的方法