Matlab R2014+visual studio 2013 混合编程(2)
2016-03-14 09:37
1021 查看
看了一篇配置 Visual studio 的博客 写的简单明了,前面已经讲了如何安装Matlab R2014a,visual studio 2013 这里不再说明
先贴上博客的代码
代码一:
mychckxy.m
function [x,y,sizey,endslopes] = mychckxy(x,y)
%CHCKXY check and adjust input for SPLINE and PCHIP
% [X,Y,SIZEY] = CHCKXY(X,Y) checks the data sites X and corresponding data
% values Y, making certain that there are exactly as many sites as values,
% that no two data sites are the same, removing any data points that involve
% NaNs, reordering the sites if necessary to ensure that X is a strictly
% increasing row vector and reordering the data values correspondingly,
% and reshaping Y if necessary to make sure that it is a matrix, with Y(:,j)
% the data value corresponding to the data site X(j), and with SIZEY the
% actual dimensions of the given values.
% This call to CHCKXY is suitable for PCHIP.
%
% [X,Y,SIZEY,ENDSLOPES] = CHCKXY(X,Y) also considers the possibility that
% there are two more data values than there are data sites.
% If there are, then the first and the last data value are removed from Y
% and returned separately as ENDSLOPES. Otherwise, an empty ENDSLOPES is
% returned. This call to CHCKXY is suitable for SPLINE.
%
% See also PCHIP, SPLINE.
% Copyright 1984-2011 The MathWorks, Inc.
% make sure X is a vector:
if length(find(size(x)>1))>1
error(message('MATLAB:chckxy:XNotVector'))
end
% ensure X is real
if any(~isreal(x))
error(message('MATLAB:chckxy:XComplex'))
end
% deal with NaN's among the sites:
nanx = find(isnan(x));
if ~isempty(nanx)
x(nanx) = [];
warning(message('MATLAB:chckxy:nan'))
end
n=length(x);
if n<2
error(message('MATLAB:chckxy:NotEnoughPts'))
end
% re-sort, if needed, to ensure strictly increasing site sequence:
x=x(:).';
dx = diff(x);
if any(dx<0), [x,ind] = sort(x); dx = diff(x); else ind=1:n; end
if ~all(dx), error(message('MATLAB:chckxy:RepeatedSites')), end
% if Y is ND, reshape it to a matrix by combining all dimensions but the last:
sizey = size(y);
while length(sizey)>2&&sizey(end)==1, sizey(end) = []; end
yn = sizey(end);
sizey(end)=[];
yd = prod(sizey);
if length(sizey)>1
y = reshape(y,yd,yn);
else
% if Y happens to be a column matrix, change it to the expected row matrix.
if yn==1
yn = yd;
y = reshape(y,1,yn);
yd = 1;
sizey = yd;
end
end
% determine whether not-a-knot or clamped end conditions are to be used:
nstart = n+length(nanx);
if yn==nstart
endslopes = [];
elseif nargout==4&&yn==nstart+2
endslopes = y(:,[1 n+2]); y(:,[1 n+2])=[];
if any(isnan(endslopes))
error(message('MATLAB:chckxy:EndslopeNaN'))
end
if any(isinf(endslopes))
error(message('MATLAB:chckxy:EndslopeInf'))
end
else
error(message('MATLAB:chckxy:NumSitesMismatchValues',nstart, yn))
end
% deal with NaN's among the values:
if ~isempty(nanx)
y(:,nanx) = [];
end
y=y(:,ind);
nany = find(sum(isnan(y),1));
if ~isempty(nany)
y(:,nany) = []; x(nany) = [];
warning(message('MATLAB:chckxy:IgnoreNaN'))
n = length(x);
if n<2
error(message('MATLAB:chckxy:NotEnoughPts'))
end
end
代码二:
function output = spline(x,y,xx)
%SPLINE Cubic spline data interpolation.
% PP = SPLINE(X,Y) provides the piecewise polynomial form of the
% cubic spline interpolant to the data values Y at the data sites X,
% for use with the evaluator PPVAL and the spline utility UNMKPP.
% X must be a vector.
% If Y is a vector, then Y(j) is taken as the value to be matched at X(j),
% hence Y must be of the same length as X -- see below for an exception
% to this.
% If Y is a matrix or ND array, then Y(:,...,:,j) is taken as the value to
% be matched at X(j), hence the last dimension of Y must equal length(X) --
% see below for an exception to this.
%
% YY = SPLINE(X,Y,XX) is the same as YY = PPVAL(SPLINE(X,Y),XX), thus
% providing, in YY, the values of the interpolant at XX. For information
% regarding the size of YY see PPVAL.
%
% Ordinarily, the not-a-knot end conditions are used. However, if Y contains
% two more values than X has entries, then the first and last value in Y are
% used as the endslopes for the cubic spline. If Y is a vector, this
% means:
% f(X) = Y(2:end-1), Df(min(X))=Y(1), Df(max(X))=Y(end).
% If Y is a matrix or N-D array with SIZE(Y,N) equal to LENGTH(X)+2, then
% f(X(j)) matches the value Y(:,...,:,j+1) for j=1:LENGTH(X), then
% Df(min(X)) matches Y(:,:,...:,1) and Df(max(X)) matches Y(:,:,...:,end).
%
% Example:
% This generates a sine-like spline curve and samples it over a finer mesh:
% x = 0:10; y = sin(x);
% xx = 0:.25:10;
% yy = spline(x,y,xx);
% plot(x,y,'o',xx,yy)
%
% Example:
% This illustrates the use of clamped or complete spline interpolation where
% end slopes are prescribed. In this example, zero slopes at the ends of an
% interpolant to the values of a certain distribution are enforced:
% x = -4:4; y = [0 .15 1.12 2.36 2.36 1.46 .49 .06 0];
% cs = spline(x,[0 y 0]);
% xx = linspace(-4,4,101);
% plot(x,y,'o',xx,ppval(cs,xx),'-');
%
% Class support for inputs x, y, xx:
% float: double, single
%
% See also INTERP1, PCHIP, PPVAL, MKPP, UNMKPP.
% Carl de Boor 7-2-86
% Copyright 1984-2010 The MathWorks, Inc.
% $Revision: 5.18.4.6 $ $Date: 2010/09/02 13:36:29 $
% Check that data are acceptable and, if not, try to adjust them appropriately
[x,y,sizey,endslopes] = mychckxy(x,y);
n = length(x); yd = prod(sizey);
% Generate the cubic spline interpolant in ppform
dd = ones(yd,1); dx = diff(x); divdif = diff(y,[],2)./dx(dd,:);
if n==2
if isempty(endslopes) % the interpolant is a straight line
pp=mkpp(x,[divdif y(:,1)],sizey);
else % the interpolant is the cubic Hermite polynomial
pp = pwch(x,y,endslopes,dx,divdif); pp.dim = sizey;
end
elseif n==3&&isempty(endslopes) % the interpolant is a parabola
y(:,2:3)=divdif;
y(:,3)=diff(divdif')'/(x(3)-x(1));
y(:,2)=y(:,2)-y(:,3)*dx(1);
pp = mkpp(x([1,3]),y(:,[3 2 1]),sizey);
else % set up the sparse, tridiagonal, linear system b = ?*c for the slopes
b=zeros(yd,n);
b(:,2:n-1)=3*(dx(dd,2:n-1).*divdif(:,1:n-2)+dx(dd,1:n-2).*divdif(:,2:n-1));
if isempty(endslopes)
x31=x(3)-x(1);xn=x(n)-x(n-2);
b(:,1)=((dx(1)+2*x31)*dx(2)*divdif(:,1)+dx(1)^2*divdif(:,2))/x31;
b(:,n)=...
(dx(n-1)^2*divdif(:,n-2)+(2*xn+dx(n-1))*dx(n-2)*divdif(:,n-1))/xn;
else
x31 = 0; xn = 0; b(:,[1 n]) = dx(dd,[2 n-2]).*endslopes;
end
dxt = dx(:);
c = spdiags([ [x31;dxt(1:n-2);0] ...
[dxt(2);2*(dxt(2:n-1)+dxt(1:n-2));dxt(n-2)] ...
[0;dxt(2:n-1);xn] ],[-1 0 1],n,n);
% sparse linear equation solution for the slopes
mmdflag = spparms('autommd');
spparms('autommd',0);
s=b/c;
spparms('autommd',mmdflag);
% construct piecewise cubic Hermite interpolant
% to values and computed slopes
pp = pwch(x,y,s,dx,divdif); pp.dim = sizey;
end
if nargin==2, output = pp; else output = ppval(pp,xx); end
先测试下调用是否正确 spline.m 调用 mychckxy.m
测试代码:
输出结果:
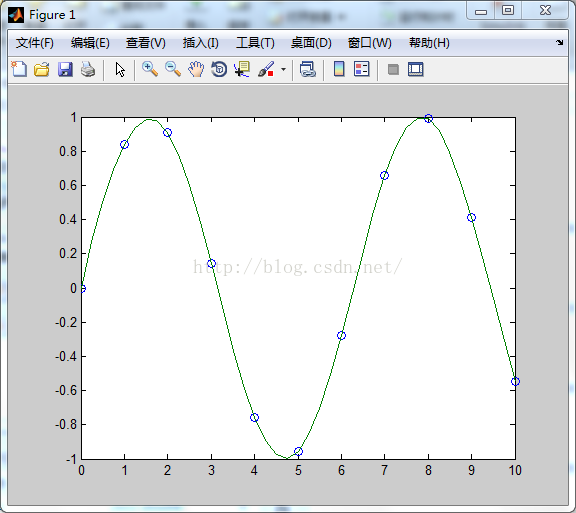
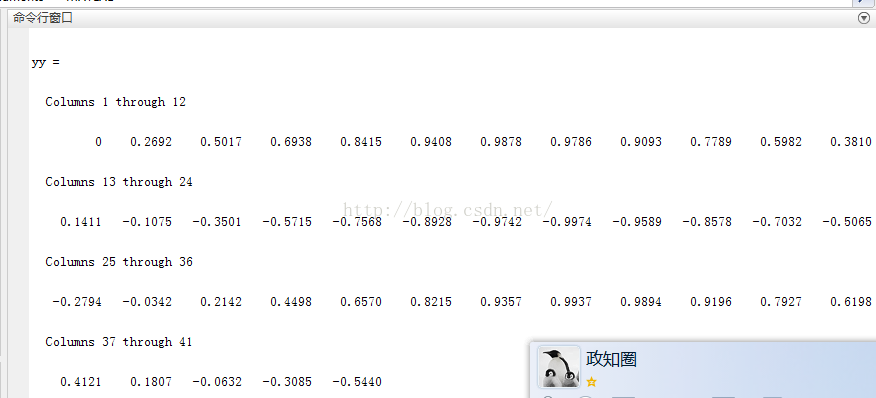
出现如上结果说明调用没有问题
下面生成我们想要的 .dll; .lib; .h;文件在matlab中输入命令:
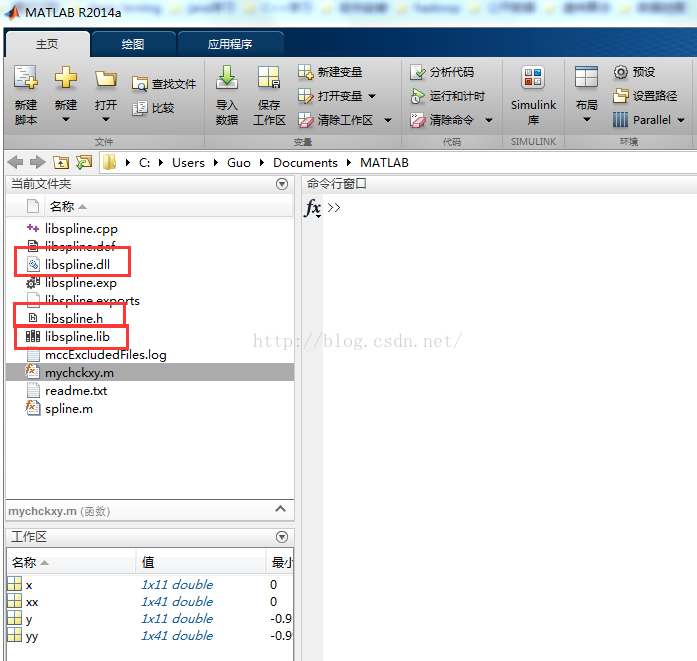
转到Visual studio中 创建 win32控制台应用程序
把上面的三个文件拷到创建的项目的根目录中
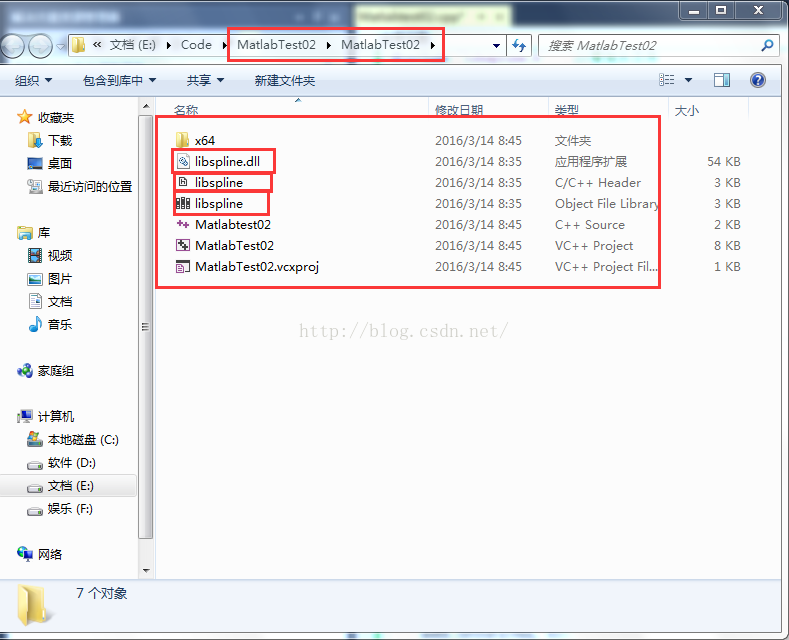
配置项目
再声明下:
我的所有安装程序都是64位的(VS,Matlab)
所以我们选择的是X64平台
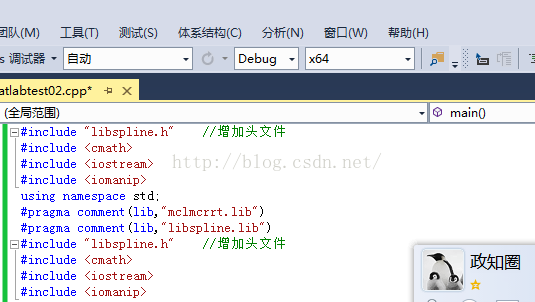
配置VC++目录
包含目录:
库目录:
第二种方案
在源文件中加入:
libspline.lib是我们上面生成的动态库。
贴上程序测试代码:
输出结果:
先贴上博客的代码
代码一:
mychckxy.m
function [x,y,sizey,endslopes] = mychckxy(x,y)
%CHCKXY check and adjust input for SPLINE and PCHIP
% [X,Y,SIZEY] = CHCKXY(X,Y) checks the data sites X and corresponding data
% values Y, making certain that there are exactly as many sites as values,
% that no two data sites are the same, removing any data points that involve
% NaNs, reordering the sites if necessary to ensure that X is a strictly
% increasing row vector and reordering the data values correspondingly,
% and reshaping Y if necessary to make sure that it is a matrix, with Y(:,j)
% the data value corresponding to the data site X(j), and with SIZEY the
% actual dimensions of the given values.
% This call to CHCKXY is suitable for PCHIP.
%
% [X,Y,SIZEY,ENDSLOPES] = CHCKXY(X,Y) also considers the possibility that
% there are two more data values than there are data sites.
% If there are, then the first and the last data value are removed from Y
% and returned separately as ENDSLOPES. Otherwise, an empty ENDSLOPES is
% returned. This call to CHCKXY is suitable for SPLINE.
%
% See also PCHIP, SPLINE.
% Copyright 1984-2011 The MathWorks, Inc.
% make sure X is a vector:
if length(find(size(x)>1))>1
error(message('MATLAB:chckxy:XNotVector'))
end
% ensure X is real
if any(~isreal(x))
error(message('MATLAB:chckxy:XComplex'))
end
% deal with NaN's among the sites:
nanx = find(isnan(x));
if ~isempty(nanx)
x(nanx) = [];
warning(message('MATLAB:chckxy:nan'))
end
n=length(x);
if n<2
error(message('MATLAB:chckxy:NotEnoughPts'))
end
% re-sort, if needed, to ensure strictly increasing site sequence:
x=x(:).';
dx = diff(x);
if any(dx<0), [x,ind] = sort(x); dx = diff(x); else ind=1:n; end
if ~all(dx), error(message('MATLAB:chckxy:RepeatedSites')), end
% if Y is ND, reshape it to a matrix by combining all dimensions but the last:
sizey = size(y);
while length(sizey)>2&&sizey(end)==1, sizey(end) = []; end
yn = sizey(end);
sizey(end)=[];
yd = prod(sizey);
if length(sizey)>1
y = reshape(y,yd,yn);
else
% if Y happens to be a column matrix, change it to the expected row matrix.
if yn==1
yn = yd;
y = reshape(y,1,yn);
yd = 1;
sizey = yd;
end
end
% determine whether not-a-knot or clamped end conditions are to be used:
nstart = n+length(nanx);
if yn==nstart
endslopes = [];
elseif nargout==4&&yn==nstart+2
endslopes = y(:,[1 n+2]); y(:,[1 n+2])=[];
if any(isnan(endslopes))
error(message('MATLAB:chckxy:EndslopeNaN'))
end
if any(isinf(endslopes))
error(message('MATLAB:chckxy:EndslopeInf'))
end
else
error(message('MATLAB:chckxy:NumSitesMismatchValues',nstart, yn))
end
% deal with NaN's among the values:
if ~isempty(nanx)
y(:,nanx) = [];
end
y=y(:,ind);
nany = find(sum(isnan(y),1));
if ~isempty(nany)
y(:,nany) = []; x(nany) = [];
warning(message('MATLAB:chckxy:IgnoreNaN'))
n = length(x);
if n<2
error(message('MATLAB:chckxy:NotEnoughPts'))
end
end
代码二:
function output = spline(x,y,xx)
%SPLINE Cubic spline data interpolation.
% PP = SPLINE(X,Y) provides the piecewise polynomial form of the
% cubic spline interpolant to the data values Y at the data sites X,
% for use with the evaluator PPVAL and the spline utility UNMKPP.
% X must be a vector.
% If Y is a vector, then Y(j) is taken as the value to be matched at X(j),
% hence Y must be of the same length as X -- see below for an exception
% to this.
% If Y is a matrix or ND array, then Y(:,...,:,j) is taken as the value to
% be matched at X(j), hence the last dimension of Y must equal length(X) --
% see below for an exception to this.
%
% YY = SPLINE(X,Y,XX) is the same as YY = PPVAL(SPLINE(X,Y),XX), thus
% providing, in YY, the values of the interpolant at XX. For information
% regarding the size of YY see PPVAL.
%
% Ordinarily, the not-a-knot end conditions are used. However, if Y contains
% two more values than X has entries, then the first and last value in Y are
% used as the endslopes for the cubic spline. If Y is a vector, this
% means:
% f(X) = Y(2:end-1), Df(min(X))=Y(1), Df(max(X))=Y(end).
% If Y is a matrix or N-D array with SIZE(Y,N) equal to LENGTH(X)+2, then
% f(X(j)) matches the value Y(:,...,:,j+1) for j=1:LENGTH(X), then
% Df(min(X)) matches Y(:,:,...:,1) and Df(max(X)) matches Y(:,:,...:,end).
%
% Example:
% This generates a sine-like spline curve and samples it over a finer mesh:
% x = 0:10; y = sin(x);
% xx = 0:.25:10;
% yy = spline(x,y,xx);
% plot(x,y,'o',xx,yy)
%
% Example:
% This illustrates the use of clamped or complete spline interpolation where
% end slopes are prescribed. In this example, zero slopes at the ends of an
% interpolant to the values of a certain distribution are enforced:
% x = -4:4; y = [0 .15 1.12 2.36 2.36 1.46 .49 .06 0];
% cs = spline(x,[0 y 0]);
% xx = linspace(-4,4,101);
% plot(x,y,'o',xx,ppval(cs,xx),'-');
%
% Class support for inputs x, y, xx:
% float: double, single
%
% See also INTERP1, PCHIP, PPVAL, MKPP, UNMKPP.
% Carl de Boor 7-2-86
% Copyright 1984-2010 The MathWorks, Inc.
% $Revision: 5.18.4.6 $ $Date: 2010/09/02 13:36:29 $
% Check that data are acceptable and, if not, try to adjust them appropriately
[x,y,sizey,endslopes] = mychckxy(x,y);
n = length(x); yd = prod(sizey);
% Generate the cubic spline interpolant in ppform
dd = ones(yd,1); dx = diff(x); divdif = diff(y,[],2)./dx(dd,:);
if n==2
if isempty(endslopes) % the interpolant is a straight line
pp=mkpp(x,[divdif y(:,1)],sizey);
else % the interpolant is the cubic Hermite polynomial
pp = pwch(x,y,endslopes,dx,divdif); pp.dim = sizey;
end
elseif n==3&&isempty(endslopes) % the interpolant is a parabola
y(:,2:3)=divdif;
y(:,3)=diff(divdif')'/(x(3)-x(1));
y(:,2)=y(:,2)-y(:,3)*dx(1);
pp = mkpp(x([1,3]),y(:,[3 2 1]),sizey);
else % set up the sparse, tridiagonal, linear system b = ?*c for the slopes
b=zeros(yd,n);
b(:,2:n-1)=3*(dx(dd,2:n-1).*divdif(:,1:n-2)+dx(dd,1:n-2).*divdif(:,2:n-1));
if isempty(endslopes)
x31=x(3)-x(1);xn=x(n)-x(n-2);
b(:,1)=((dx(1)+2*x31)*dx(2)*divdif(:,1)+dx(1)^2*divdif(:,2))/x31;
b(:,n)=...
(dx(n-1)^2*divdif(:,n-2)+(2*xn+dx(n-1))*dx(n-2)*divdif(:,n-1))/xn;
else
x31 = 0; xn = 0; b(:,[1 n]) = dx(dd,[2 n-2]).*endslopes;
end
dxt = dx(:);
c = spdiags([ [x31;dxt(1:n-2);0] ...
[dxt(2);2*(dxt(2:n-1)+dxt(1:n-2));dxt(n-2)] ...
[0;dxt(2:n-1);xn] ],[-1 0 1],n,n);
% sparse linear equation solution for the slopes
mmdflag = spparms('autommd');
spparms('autommd',0);
s=b/c;
spparms('autommd',mmdflag);
% construct piecewise cubic Hermite interpolant
% to values and computed slopes
pp = pwch(x,y,s,dx,divdif); pp.dim = sizey;
end
if nargin==2, output = pp; else output = ppval(pp,xx); end
先测试下调用是否正确 spline.m 调用 mychckxy.m
测试代码:
clc; clear all; close all; x = 0:10; y = sin(x); xx = 0:.25:10; yy = spline(x,y,xx) plot(x,y,'o',xx,yy);
输出结果:
出现如上结果说明调用没有问题
下面生成我们想要的 .dll; .lib; .h;文件在matlab中输入命令:
mcc -W cpplib:libspline -T link:lib spline.m
转到Visual studio中 创建 win32控制台应用程序
把上面的三个文件拷到创建的项目的根目录中
配置项目
再声明下:
我的所有安装程序都是64位的(VS,Matlab)
所以我们选择的是X64平台
配置VC++目录
包含目录:
D:\Program Files\MATLAB\R2014a\extern\include
库目录:
D:\Program Files\MATLAB\R2014a\extern\lib\win64\microsoft 至于需要链接的库的配置,这里有两种解决方案,第一种在vs中配置。
项目 ----> 属性 ----> 连接器 ----> 输入 ---->附加依赖项
第二种方案
在源文件中加入:
#pragma comment(lib,"mclmcrrt.lib") #pragma comment(lib,"libspline.lib")
libspline.lib是我们上面生成的动态库。
贴上程序测试代码:
#include "libspline.h" //增加头文件
#include <cmath>
#include <iostream>
#include <iomanip>
using namespace std;
#pragma comment(lib,"mclmcrrt.lib") #pragma comment(lib,"libspline.lib")
#include "libspline.h" //增加头文件
#include <cmath>
#include <iostream>
#include <iomanip>
using namespace std;
#pragma comment(lib,"mclmcrrt.lib") #pragma comment(lib,"libspline.lib")
int main()
{
//初始化lib(必须)
if (!libsplineInitialize())
return -1;
int i, j;
double x[1][11], y[1][11];
for (i = 0; i<11; i++)
{
x[0][i] = i;
y[0][i] = sin(x[0][i]);
}
double xx[1][41];
for (i = 0; i<41; i++)
xx[0][i] = i*0.25;
double yy[1][41];
mwArray mwX(1, 11, mxDOUBLE_CLASS);
mwArray mwY(1, 11, mxDOUBLE_CLASS);
mwArray mwXX(1, 41, mxDOUBLE_CLASS);
mwArray mwYY(1, 41, mxDOUBLE_CLASS);
mwX.SetData(*x, 11);
mwY.SetData(*y, 11);
mwXX.SetData(*xx, 41);
mwYY.SetData(*yy, 41);
spline(1, mwYY, mwX, mwY, mwXX); //调用spline
cout << "yy = " << endl;
i = 0;
for (j = 0; j < 41; j++)
{
//Get第一个参数表示用1个下标访问元素,j+1是列号(MATLAB下标从1开始,而C++从0开始,故做+1操作)
yy[0][j] = mwYY.Get(1, j + 1);
cout << setprecision(4) << right << setw(10) << yy[0][j];
i++;
if (i % 7 == 0) cout << endl; //换行
}
cout << endl;
//终止调用
libsplineTerminate();
return 0;
}
输出结果:
相关文章推荐
- 使用C++实现JNI接口需要注意的事项
- 关于指针的一些事情
- c++ primer 第五版 笔记前言
- share_ptr的几个注意点
- Lua中调用C++函数示例
- Lua教程(一):在C++中嵌入Lua脚本
- Lua教程(二):C++和Lua相互传递数据示例
- C++联合体转换成C#结构的实现方法
- C++高级程序员成长之路
- C++编写简单的打靶游戏
- C++ 自定义控件的移植问题
- C++变位词问题分析
- C/C++数据对齐详细解析
- C++基于栈实现铁轨问题
- C++中引用的使用总结
- 使用Lua来扩展C++程序的方法
- C++中调用Lua函数实例
- Lua和C++的通信流程代码实例
- C与C++之间相互调用实例方法讲解
- 解析C++中派生的概念以及派生类成员的访问属性