通知Notification
2016-01-30 00:13
232 查看
简单Notification
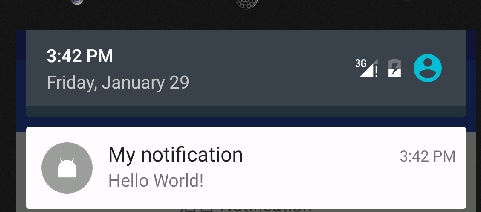
创建Builder
NotificationCompat.Builder mBuilder = new NotificationCompat.Builder(this) .setSmallIcon(R.mipmap.ic_launcher) .setContentTitle("My notification") .setContentText("Hello World!");
添加Active
// 2. 创建点击回调动作 Intent resultIntent = new Intent(this, ResultActivity.class); // Because clicking the notification opens a new ("special") activity, there's // no need to create an artificial back stack. PendingIntent resultPendingIntent = PendingIntent.getActivity( this, 0, resultIntent, PendingIntent.FLAG_UPDATE_CURRENT ); mBuilder.setContentIntent(resultPendingIntent);
通知
// Builds the notification and issues it. mNotifyMgr.notify(101, mBuilder.build());
后台调用
// Creates an Intent for the Activity Intent resultIntent = new Intent(this, ResultActivity.class); // Sets the Activity to start in a new, empty task resultIntent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_CLEAR_TASK); // Creates the PendingIntent notifyIntent = PendingIntent.getActivity( this, 0, resultIntent, PendingIntent.FLAG_UPDATE_CURRENT );
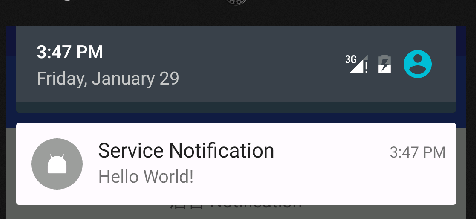
移除Notification
调用后自动清除builder.setAutoCancel(true);
指定的 notification ID调用了cancel()
指定的 notification ID调用了cancelAll()
接下来的几个样式就不说了,简单,先贴图再看代码
BigView之Text
调用bigTextNotification
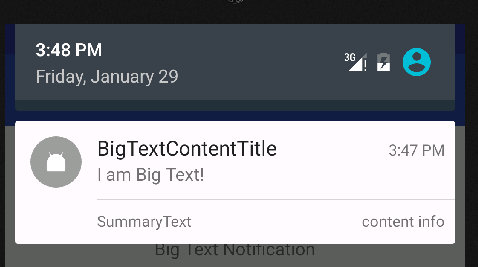
BigView之Picture
调用bigPictureNotification
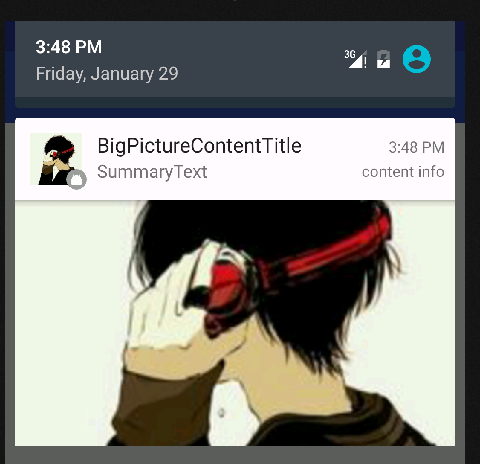
BigView之Inbox
调用bigInboxNotification
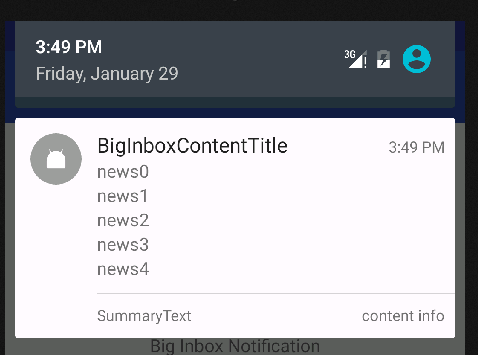
BigView之Custom
调用customNotification
大图
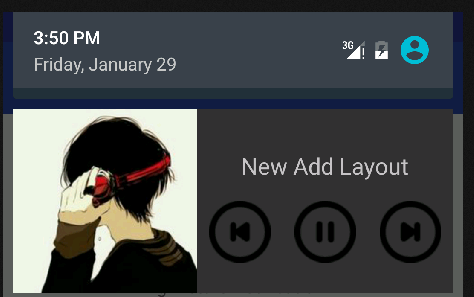
小图
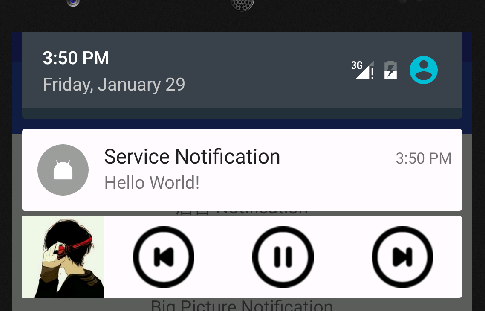
Code
代码部分package com.xuie.notificationdemo;
import android.app.Notification;
import android.app.NotificationManager;
import android.app.PendingIntent;
import android.app.Service;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.content.IntentFilter;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Binder;
import android.os.IBinder;
import android.support.v4.app.NotificationCompat;
import android.widget.RemoteViews;
public class MyService extends Service {
PendingIntent notifyIntent;
NotificationManager mNotificationManager;
@Override
public void onCreate() {
super.onCreate();
// Creates an Intent for the Activity Intent resultIntent = new Intent(this, ResultActivity.class); // Sets the Activity to start in a new, empty task resultIntent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_CLEAR_TASK); // Creates the PendingIntent notifyIntent = PendingIntent.getActivity( this, 0, resultIntent, PendingIntent.FLAG_UPDATE_CURRENT );
// Notifications are issued by sending them to the
// NotificationManager system service.
mNotificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
// Builds an anonymous Notification object from the builder, and
// passes it to the NotificationManager
IntentFilter filter = new IntentFilter();
filter.addAction(REMOTE_PLAY);
filter.addAction(REMOTE_NEXT);
filter.addAction(REMOTE_PREVIOUS);
registerReceiver(receiver, filter);
}
public void startNotification() {
// Instantiate a Builder object.
NotificationCompat.Builder builder = new NotificationCompat.Builder(this);
// Puts the PendingIntent into the notification builder
builder.setContentIntent(notifyIntent);
builder.setSmallIcon(R.mipmap.ic_launcher)
.setContentTitle("Service Notification")
.setContentText("Hello World!");
// 设置自动清除Notification。其它方法:指定的 notification ID调用了cancel(),或cancelAll()
builder.setAutoCancel(true);
mNotificationManager.notify(101, builder.build());
}
public void bigTextNotification() {
NotificationCompat.BigTextStyle textStyle = new NotificationCompat.BigTextStyle()
.setBigContentTitle("BigTextContentTitle")
.setSummaryText("SummaryText")
.bigText("I am Big Text!");
Notification notification = new NotificationCompat.Builder(this)
.setSmallIcon(R.mipmap.ic_launcher)
.setTicker("show big view text") // 第一次提示消息的时候显示在通知栏上
.setContentInfo("content info")
.setContentTitle("ContentTitle").setContentText("ContentText")
.setStyle(textStyle)
.setAutoCancel(true).setDefaults(Notification.DEFAULT_ALL)
.setContentIntent(notifyIntent)
.setAutoCancel(true)
.build();
mNotificationManager.notify(101, notification);
}
public void bigPictureNotification() {
NotificationCompat.BigPictureStyle pictureStyle = new NotificationCompat.BigPictureStyle()
.setBigContentTitle("BigPictureContentTitle")
.setSummaryText("SummaryText")
.bigPicture(resource2Bitmap(this, R.mipmap.ic_big_view));
Notification notification = new NotificationCompat.Builder(this)
.setLargeIcon(resource2Bitmap(this, R.mipmap.ic_big_view))// 这个与setSmallIcon相冲? - 测试手机Nexus5
.setSmallIcon(R.mipmap.ic_launcher)
.setTicker("show big view picture")
.setContentInfo("content info")
.setContentTitle("ContentTitle")
.setContentText("ContentText")
.setStyle(pictureStyle)
.setAutoCancel(true)
.setDefaults(Notification.DEFAULT_ALL)
.build();
mNotificationManager.notify(101, notification);
}
public void bigInboxNotification() {
NotificationCompat.InboxStyle inboxStyle = new NotificationCompat.InboxStyle();
inboxStyle.setBigContentTitle("BigInboxContentTitle").setSummaryText("SummaryText");
for (int i = 0; i < 5; i++) {
inboxStyle.addLine("news" + i);
}
Notification notification = new NotificationCompat.Builder(this)
.setSmallIcon(R.mipmap.ic_launcher)
.setTicker("show big inbox picture")
.setContentInfo("content info")
.setContentTitle("ContentTitle")
.setContentText("ContentText")
.setStyle(inboxStyle)
.setAutoCancel(true)
.setDefaults(Notification.DEFAULT_ALL)
.build();
mNotificationManager.notify(101, notification);
}
public void customNotification() {
PendingIntent playIntent = PendingIntent.getBroadcast(this, 0, new Intent(REMOTE_PLAY), PendingIntent.FLAG_UPDATE_CURRENT);
PendingIntent nextIntent = PendingIntent.getBroadcast(this, 0, new Intent(REMOTE_NEXT), PendingIntent.FLAG_UPDATE_CURRENT);
PendingIntent previousIntent = PendingIntent.getBroadcast(this, 0, new Intent(REMOTE_PREVIOUS), PendingIntent.FLAG_UPDATE_CURRENT);
RemoteViews remoteViews = new RemoteViews(getPackageName(), R.layout.custom_notification);
remoteViews.setOnClickPendingIntent(R.id.play_pause, playIntent);
remoteViews.setImageViewResource(R.id.play_pause, R.mipmap.pause);
remoteViews.setOnClickPendingIntent(R.id.next, nextIntent);
remoteViews.setOnClickPendingIntent(R.id.previous, previousIntent);
RemoteViews remoteViews_expand = new RemoteViews(getPackageName(), R.layout.custom_expanded_notification);
remoteViews_expand.setOnClickPendingIntent(R.id.play_pause, playIntent);
remoteViews_expand.setOnClickPendingIntent(R.id.next, nextIntent);
remoteViews_expand.setOnClickPendingIntent(R.id.previous, previousIntent);
remoteViews_expand.setTextViewText(R.id.new_text, "New Add Layout");
NotificationCompat.Builder builder = new NotificationCompat.Builder(this)
.setWhen(System.currentTimeMillis())
.setSmallIcon(R.mipmap.ic_launcher)
// .setContentIntent(notifyIntent)
// .setOngoing(true)
.setAutoCancel(true)
.setTicker("music is playing");
Notification notification = builder.build();
notification.contentView = remoteViews;
notification.bigContentView = remoteViews_expand;
mNotificationManager.notify(102, notification);
}
public static final String REMOTE_PLAY = "remote play";
public static final String REMOTE_PREVIOUS = "remote previous";
public static final String REMOTE_NEXT = "remote next";
private BroadcastReceiver receiver = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if (action.equals(REMOTE_PLAY)) {
System.out.println("REMOTE_PLAY");
} else if (action.equals(REMOTE_NEXT)) {
System.out.println("REMOTE_NEXT");
} else if (action.equals(REMOTE_PREVIOUS)) {
System.out.println("REMOTE_PREVIOUS");
}
}
};
@Override
public void onDestroy() {
super.onDestroy();
unregisterReceiver(receiver);
}
class MyBinder extends Binder {
MyService getService() {
return MyService.this;
}
}
@Override
public IBinder onBind(Intent intent) {
return new MyBinder();
}
public static Bitmap resource2Bitmap(Context context, int drawId) {
return BitmapFactory.decodeResource(context.getResources(), drawId);
}
}
下载
相关文章推荐
- Java 正则表达式的用法及详细介绍
- 公司十周年年会
- 爱友文章
- 在CentOS VPS上源码安装高版本git
- [转]Android开发配置,消除SDK更新时的“https://dl-ssl.google.com
- Robotruck
- <select>下拉菜单click点击事件在谷歌浏览器无法执行问题
- v7自带Actionbar 的配置
- 理论: 博弈2: 巴什博奕(Bash Game)
- iOS 本地通知
- Android开发辅助工具之GitHub
- 让程序提升Debug权限
- 1、OMD安装(check mk+nagios+...) ,版本:RHEL7+OMD1.2.4p5
- Java Calendar类
- 安卓开发——Androidstudio中如何创建shape的XML文件
- 11gR2 GI standalone环境(即:GI 单机环境)中asm spfile的位置信息
- 多路复用io接口-epoll
- mysql存储引擎
- PHP功能齐全的发送邮件类
- UEFI引导修复教程